🌍 All
About us
Digitalization
News
Startups
Development
Design
Dependency Injection in Nest.js
Viktor Kharchenko
Jan 07, 2024・5 min read
Table of Content
Understanding Dependency Injection
The Power of Dependency Injection
What Is Dependency Injection?
Injecting Dependencies into Controllers and Services
Using Custom Providers for Dependency Injection
Handling Dependency Scopes in Nest.js
Dependency Injection in Middleware and Pipes
Testing and Mocking Dependencies in Nest.js
Conclusion
Picture yourself as the architect of a grand skyscraper, and your software project is that very skyscraper. To construct it with precision and efficiency, you need the right materials at the right time. In the world of software development, "Dependency Injection" serves as your trusted blueprint for assembling components seamlessly. Welcome to the realm of Nest.js, where Dependency Injection is the cornerstone of building robust and maintainable applications.
Understanding Dependency Injection
At its core, Dependency Injection (DI) is a design pattern that promotes the decoupling of components within an application. It's like assembling a complex puzzle where each piece knows exactly where it fits without needing to understand the entire picture. In Nest.js, DI is not just a concept; it's a fundamental building block.
Imagine you're crafting a Nest.js service:
import { Injectable } from '@nestjs/common';
@Injectable()
export class ProductService {
constructor(private readonly productService: ProductService) {}
// Methods and functionality go here
Here, we use the @Injectable() decorator to signify that ProductService is an injectable component. This tells Nest.js that this class can be provided to other parts of your application.
The Power of Dependency Injection
Why is Dependency Injection in Nest.js so vital? It promotes modularity, testability, and scalability. It allows you to swap out components effortlessly, making your codebase adaptable to change. With DI, unit testing becomes a breeze, as you can inject mock dependencies for isolated testing.
In this article, we'll delve deeper into the world of Dependency Injection in Nest.js. We'll explore how it simplifies your code, improves maintainability, and elevates your development experience. So, fasten your seatbelts as we embark on this journey to master the art of Dependency Injection in Nest.js!
What Is Dependency Injection?
Imagine you're the director of a grand theatre production. Your play is a complex masterpiece, and it involves many actors, props, and behind-the-scenes crew members. To orchestrate this theatrical spectacle, you need a way to bring all the elements together seamlessly. This is precisely where Dependency Injection (DI) takes centre stage in the world of Nest.js and software development.
The Essence of Dependency Injection
Dependency Injection is a design pattern that addresses the challenge of managing dependencies in a software application. Think of dependencies as the actors and props in your theatre production. They are the pieces of code or objects that your application relies on to function correctly.
Consider this TypeScript example:
private script: Script;
constructor(script: Script) {
this.script = script;
}
startProduction() {
this.script.perform();
// Rest of the production process
In this scenario, the Director class depends on a Script to start the production. Instead of creating the Script instance within the Director class, Dependency Injection allows us to pass the Script object as a parameter to the constructor. This decouples the Director from the specific implementation of Script and makes it more flexible and maintainable.
Why Dependency Injection Matters
Dependency Injection offers several compelling benefits:
- Modularity: It promotes code modularity by breaking down complex systems into smaller, manageable components.
- Testability: Injecting dependencies makes it easy to substitute them with mock objects during testing, ensuring robust and reliable tests.
- Flexibility: It allows you to swap out dependencies or change their behaviour without altering the core code.
- Readability: By explicitly declaring dependencies, code becomes more transparent and self-documenting.
In Nest.js, Dependency Injection is a powerful tool for building scalable, maintainable, and testable applications. It fosters code that is both flexible and easy to understand, making it a valuable asset in the developer's toolkit. As we explore further, you'll discover how to harness the full potential of Dependency Injection to create efficient and elegant software solutions.
Injecting Dependencies into Controllers and Services
In the symphony of TypeScript and Nest.js, injecting dependencies into controllers and services is like orchestrating a harmonious melody. It's a fundamental concept that allows your application to seamlessly integrate different components, ensuring they work together cohesively.
Injecting Dependencies in Controllers
Controllers in Nest.js are responsible for handling incoming requests and orchestrating the flow of your application. To inject dependencies into a controller, you simply declare them in the controller's constructor. Let's explore a basic example:
import { Controller, Get } from '@nestjs/common';
import { ProductService } from './product.service';
@Controller('products')
export class ProductController {
constructor(private readonly productService: ProductService) {}
@Get()
findAll(): string {
return this.productService.findAll();
In this snippet, the ProductController depends on the ProductService. By adding private readonly productService: ProductService to the constructor, Nest.js automatically injects an instance of ProductService when a ProductController is created. This promotes modularity and code separation, making your application more maintainable.
Injecting Dependencies in Services
Services in Nest.js are the workhorses of your application. They encapsulate business logic and are often the recipients of injected dependencies. Here's an example:
import { Injectable } from '@nestjs/common';
import { LoggerService } from './logger.service';
@Injectable()
export class ProductService {
constructor(private readonly loggerService: LoggerService) {}
// Methods and functionality go here
}
In this case, the ProductService depends on the LoggerService. Just like in controllers, you declare the dependency in the constructor. This allows you to use the LoggerService within the ProductService to log messages or perform other logging-related tasks.
Using Custom Providers for Dependency Injection
In the vibrant ecosystem of Nest.js and TypeScript, the ability to wield custom providers for dependency injection is akin to crafting your own tools for a specialised task. It's a skill that grants you the freedom to inject your unique components into your application, adding a layer of personalization and adaptability.
Defining Custom Providers
Creating a custom provider is a straightforward process. You start by defining a TypeScript class and mark it with the @Injectable() decorator. This signals to Nest.js that your class can be used as an injectable dependency. Let's create an example:
import { Injectable } from '@nestjs/common';
@Injectable()
export class CustomLogger {
log(message: string) {
console.log(`CustomLogger: ${message}`);
In this case, we've crafted a CustomLogger class that's capable of logging messages in a custom format.
Injecting Custom Providers
Once you've defined your custom provider, you can inject it into various parts of your Nest.js application just like any other built-in service. Let's see how you can inject CustomLogger into a service:
import { Injectable } from '@nestjs/common';
import { CustomLogger } from './custom-logger';
@Injectable()
export class ProductService {
constructor(private readonly customLogger: CustomLogger) {}
// Methods and functionality go here
}
In this example, the ProductService depends on the CustomLogger. By declaring private readonly customLogger: CustomLogger in the constructor, Nest.js automatically injects an instance of CustomLogger when creating a ProductService. Now, you have the power to use this custom logger within your service.
The Power of Custom Providers
Custom providers for dependency injection open up a world of possibilities. They allow you to integrate your unique components and services seamlessly into your Nest.js application, tailoring it to your specific needs. Whether you're building a custom logger, data access layer, or any other specialised functionality, custom providers are your key to enhancing the flexibility and extensibility of your application.
As you continue your journey into TypeScript and Nest.js, remember that custom providers are your secret sauce for creating truly unique and powerful applications. Embrace this capability, and watch your application architecture evolve into a masterpiece of your own design. So, let's continue our exploration of Nest.js and TypeScript, armed with the knowledge of custom providers!
Handling Dependency Scopes in Nest.js
In the intricate dance of software development, managing dependency scopes is like orchestrating different acts of a theatrical performance. In the context of Nest.js and TypeScript, it's a crucial concept that allows you to control how instances of dependencies are created and shared within your application.
Understanding Dependency Scopes
In Nest.js, you have control over the scope of your dependencies. Dependency scope determines how long a particular instance of a service or provider lives and when it gets disposed of. There are two primary scopes to consider:
- Singleton Scope: In this scope, a single instance of the dependency is shared across the entire application. It's created once and reused throughout the application's lifecycle.
- Request Scope: In this scope, a new instance of the dependency is created for each incoming HTTP request. Once the request is processed, the instance is disposed of.
Configuring Dependency Scopes
Nest.js offers a straightforward way to specify the scope of your dependencies using the @Injectable() decorator. By default, dependencies are singleton-scoped. Here's an example:
import { Injectable, Scope } from '@nestjs/common';
@Injectable({ scope: Scope.REQUEST })
export class RequestScopedService {
// Methods and functionality go here
}
In this snippet, we've explicitly set the RequestScopedService to have a request scope. This means that a new instance of RequestScopedService will be created for each incoming HTTP request and disposed of when the request is completed.
Why Dependency Scope Matters?
Handling dependency scopes in Nest.js is a powerful tool for managing the lifecycle of your application's components. It offers several benefits:
- Resource Efficiency: Request-scoped dependencies ensure that resources are allocated only when needed, preventing resource leaks.
- Isolation: Singleton-scoped dependencies allow for shared state across the application, while request-scoped dependencies keep state isolated to individual requests.
- Customization: You can tailor the scope of each dependency to meet the specific requirements of your application.
Example Use Cases
Consider an authentication service in a web application. You might want to use a request-scoped dependency to keep user authentication state isolated to each request. On the other hand, a database connection service could be singleton-scoped to efficiently manage database connections across the entire application.
Understanding and effectively using dependency scopes in Nest.js is crucial for designing scalable, efficient, and secure applications. It's a key element in crafting robust and performant software, and as you delve deeper into TypeScript and Nest.js, mastering this concept will be invaluable. So, let's continue our exploration, armed with the knowledge of dependency scopes!
Dependency Injection in Middleware and Pipes
In the intricate choreography of Nest.js and TypeScript, Dependency Injection extends its reach to middleware and pipes. These essential components play a pivotal role in intercepting and processing incoming requests, and they too can benefit from the power of dependency injection.
Middleware and Pipes: The Interceptors of Request Flow
Middleware and pipes act as interceptors in the request-response cycle of your Nest.js application. Middleware functions execute before route handlers, allowing you to perform tasks such as logging, authentication, or validation. Pipes, on the other hand, transform or validate data before it reaches the route handler.
Injecting Dependencies into Middleware
Imagine you have an authentication middleware that needs to verify user credentials. Instead of manually creating instances of the services it requires, you can leverage dependency injection. Here's a simplified example:
import { Injectable, NestMiddleware } from '@nestjs/common';
import { AuthService } from './auth.service';
@Injectable()
export class AuthMiddleware implements NestMiddleware {
constructor(private readonly authService: AuthService) {}
async use(req: Request, res: Response, next: () => void) {
const token = req.headers.authorization;
const user = await this.authService.verifyToken(token);
// Handle authentication logic
next();
In this example, we've injected the AuthService into the AuthMiddleware. This allows us to use the AuthService methods to verify the authentication token seamlessly within the middleware.
Injecting Dependencies into Pipes
Pipes, like middleware, can also benefit from dependency injection. Let's say you have a pipe that validates the incoming data before it reaches your route handler. Here's how you can inject a validation service into a pipe:
import { Injectable, PipeTransform } from '@nestjs/common';
import { ValidationService } from './validation.service';
@Injectable()
export class ValidationPipe implements PipeTransform {
constructor(private readonly validationService: ValidationService) {}
transform(value: any) {
if (!this.validationService.isValid(value)) {
throw new Error('Invalid data');
}
return value;
}
}
In this example, we've injected the ValidationService into the ValidationPipe. The pipe uses this service to validate the incoming data, and if it's invalid, it throws an error.
The Power of Dependency Injection in Middleware and Pipes
By injecting dependencies into middleware and pipes, you keep your code clean, modular, and testable. It also promotes code reusability and separation of concerns. Moreover, you can easily swap out dependencies for testing or make changes without affecting the core functionality of your middleware and pipes.
In the grand symphony of Nest.js, dependency injection in middleware and pipes is the harmonious note that ensures your application's request flow remains robust, secure, and adaptable. As you delve further into TypeScript and Nest.js, remember that this powerful concept extends its reach to every corner of your application, offering flexibility and maintainability. So, let's continue our exploration, now with middleware and pipes in our repertoire!
Testing and Mocking Dependencies in Nest.js
In the world of software development, testing is the foundation of reliable and robust applications. Nest.js, with its built-in support for Dependency Injection, makes testing and mocking dependencies a breeze.
The Importance of Testing Dependencies
Testing your code is crucial to ensure it works as expected. When you use dependency injection in Nest.js, your components rely on external services and modules. In testing, you want to isolate your components from these external dependencies to focus on testing their behavior in isolation.
Mocking Dependencies for Testing
Nest.js provides a convenient way to mock dependencies during testing. You can create mock versions of your services or modules and inject them into your components for testing purposes.
Let's say you have a product service that interacts with a database. Here's how you can mock it for testing:
import { Test, TestingModule } from '@nestjs/testing';
import { ProductService } from './product.service';
// Create a mock ProductService
const productServiceMock = {
findAll: jest.fn(),
};
describe('ProductService', () => {
let service: ProductService;
beforeEach(async () => {
const module: TestingModule = await Test.createTestingModule({
providers: [
ProductService,
// Provide the mock instead of the actual service
{ provide: ProductService, useValue: productServiceMock },
],
}).compile();
service = module.get<ProductService>(ProductService);
});
it('should be defined', () => {
expect(service).toBeDefined();
});
it('should return an array of products', () => {
productServiceMock.findAll.mockReturnValue(['Product 1', 'Product 2']);
const products = service.findAll();
expect(products).toEqual(['Product 1', 'Product 2']);
});
});
In this example, we create a mock version of ProductService and provide it as a dependency when testing. This allows us to isolate and control the behavior of ProductService for testing purposes.
Benefits of Mocking Dependencies
Mocking dependencies in Nest.js testing offers several advantages:
- Isolation: You can test your components in isolation without relying on real external services or modules.
- Control: You have full control over the behavior of mocked dependencies, making it easier to cover various testing scenarios.
- Speed: Mocking can make your tests faster by avoiding time-consuming operations like database queries.
By mastering the art of testing and mocking dependencies in Nest.js, you ensure the reliability and quality of your applications. It's a fundamental skill in the world of TypeScript and Nest.js development, and it empowers you to deliver software that stands the test of time. So, let's continue our journey with testing as a reliable companion!
Conclusion
As we conclude our exploration of Dependency Injection in Nest.js, you've embarked on a journey that unraveled the power and elegance of this fundamental design pattern. We've witnessed how Dependency Injection fosters clean, modular, and maintainable code, making your applications flexible and scalable.
Throughout this journey, you've learned how to:
- Inject Dependencies: We delved into the mechanics of injecting dependencies into controllers, services, middleware, and pipes, enhancing the modularity and testability of your code.
- Create Custom Providers: You discovered the ability to craft custom providers, tailoring your application to meet specific requirements and opening doors to limitless possibilities.
- Handle Dependency Scopes: Understanding and configuring dependency scopes allows you to control the lifecycle of your components, ensuring resource efficiency and code isolation.
- Test and Mock Dependencies: Mastering the art of testing and mocking dependencies equipped you with the tools to verify the reliability of your code and its behavior in various scenarios.
Now armed with the knowledge and skills to wield Dependency Injection effectively, you're poised to build applications that are not only powerful but also maintainable and adaptable to change. As you continue your journey in TypeScript and Nest.js development, remember that Dependency Injection is your steadfast ally, guiding you toward the creation of software that stands the test of time. Embrace its principles, experiment with its possibilities, and let it be your compass in the ever-evolving landscape of modern web development.
FAQs:
1. How does Dependency Injection benefit Nest.js development?
Dependency Injection enhances code modularity, maintainability, and testability in Nest.js applications.
2. What is the role of Dependency Injection in building modular applications?
Dependency Injection promotes modularity by allowing components to request their dependencies instead of creating them.
3. Can I inject custom providers in Nest.js?
Yes, you can inject custom providers to tailor your application's behavior and functionality.
4. What are the two primary dependency scopes in Nest.js?
The two primary scopes are Singleton and Request, controlling how instances of dependencies are created and managed.
5. How can I create a custom provider for Dependency Injection?
You can create a custom provider by defining a class and marking it as injectable with @Injectable().
6. What's the purpose of handling dependency scopes in Nest.js?
Handling dependency scopes controls resource usage and isolation, ensuring efficient and secure application behavior.
7. Why is testing and mocking dependencies important in Nest.js?
Testing and mocking dependencies enable thorough testing of components in isolation, ensuring reliability.
8. Can I use dependency injection in middleware and pipes in Nest.js?
Yes, you can inject dependencies into middleware and pipes, enhancing their functionality.
9. What is the difference between middleware and pipes in Nest.js?
Middleware handles request processing, while pipes transform or validate data before reaching the route handler.
10. How do I inject dependencies into middleware in Nest.js?
You inject dependencies into middleware by defining them in the constructor, similar to controllers and services.
11. What are the benefits of mocking dependencies during testing?
Mocking dependencies isolate components for testing, allowing you to control behavior and focus on specific scenarios.
12. How can I isolate components for testing in Nest.js?
You isolate components by injecting mocked dependencies and ensuring they don't rely on real external services.
13. What is the role of custom providers in dependency injection?
Custom providers allow you to create specialized dependencies, tailoring your application's behavior.
14. How do I configure the scope of a dependency in Nest.js?
You configure the scope of a dependency by setting the scope property in the @Injectable() decorator.
15. What are some best practices for using Dependency Injection in Nest.js?
Best practices include keeping components small, focusing on single responsibilities, and favoring dependency injection over manual instantiation.
16. How does Dependency Injection enhance code maintainability?
Dependency Injection improves maintainability by promoting code separation and reusability.
17. Can I use Dependency Injection to improve code scalability?
Yes, Dependency Injection facilitates the construction of scalable applications by breaking them into smaller, interchangeable parts.
18. What is the relationship between Nest.js and TypeScript in Dependency Injection?
Nest.js leverages TypeScript's type-checking and decorators for effective Dependency Injection.
19. Are there any performance considerations when using Dependency Injection?
While Dependency Injection can introduce minor overhead, it's generally negligible and outweighed by its benefits.
20. Where can I find further resources and examples for Dependency Injection in Nest.js?
You can explore official Nest.js documentation, online tutorials, and community forums for in-depth resources and examples.
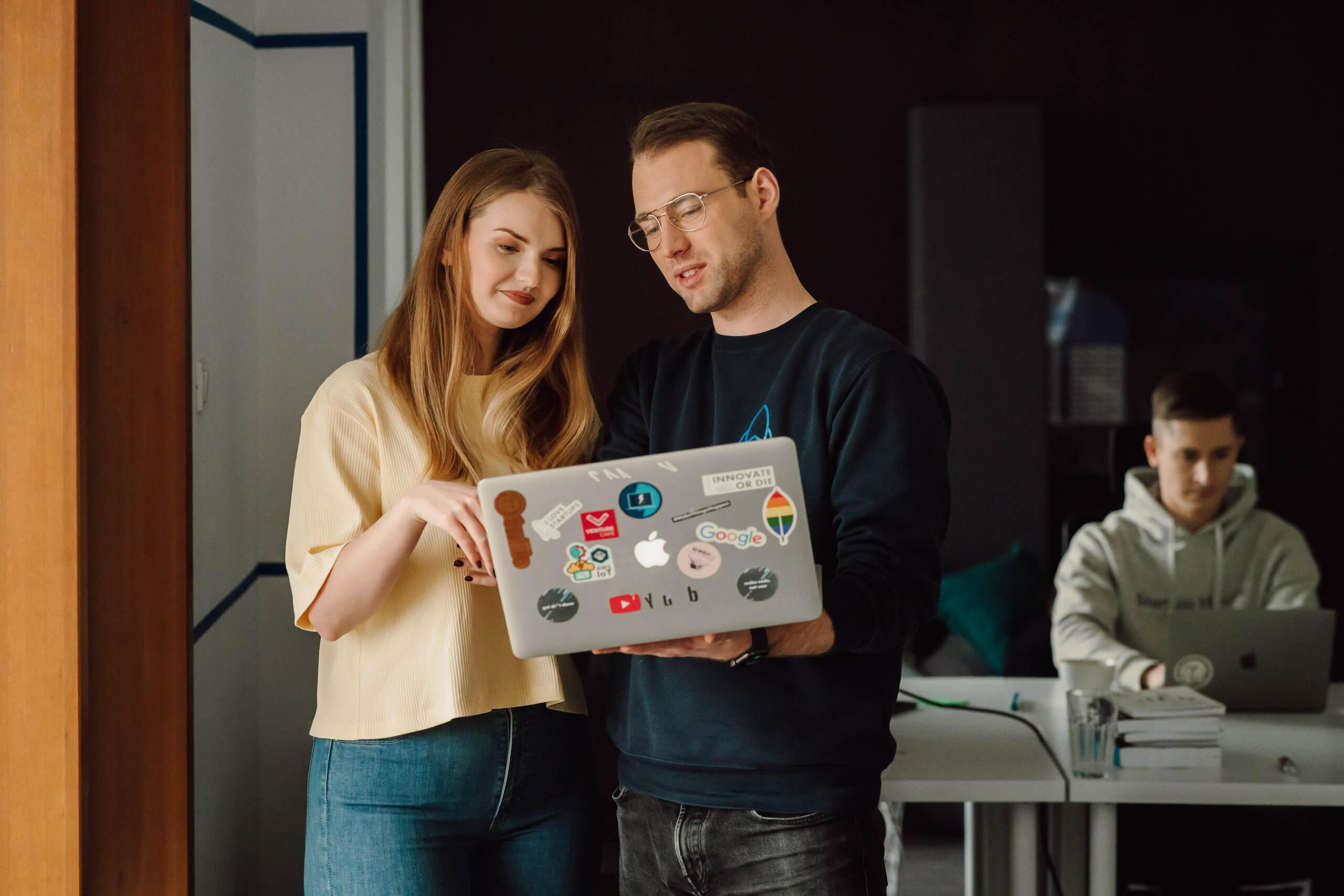
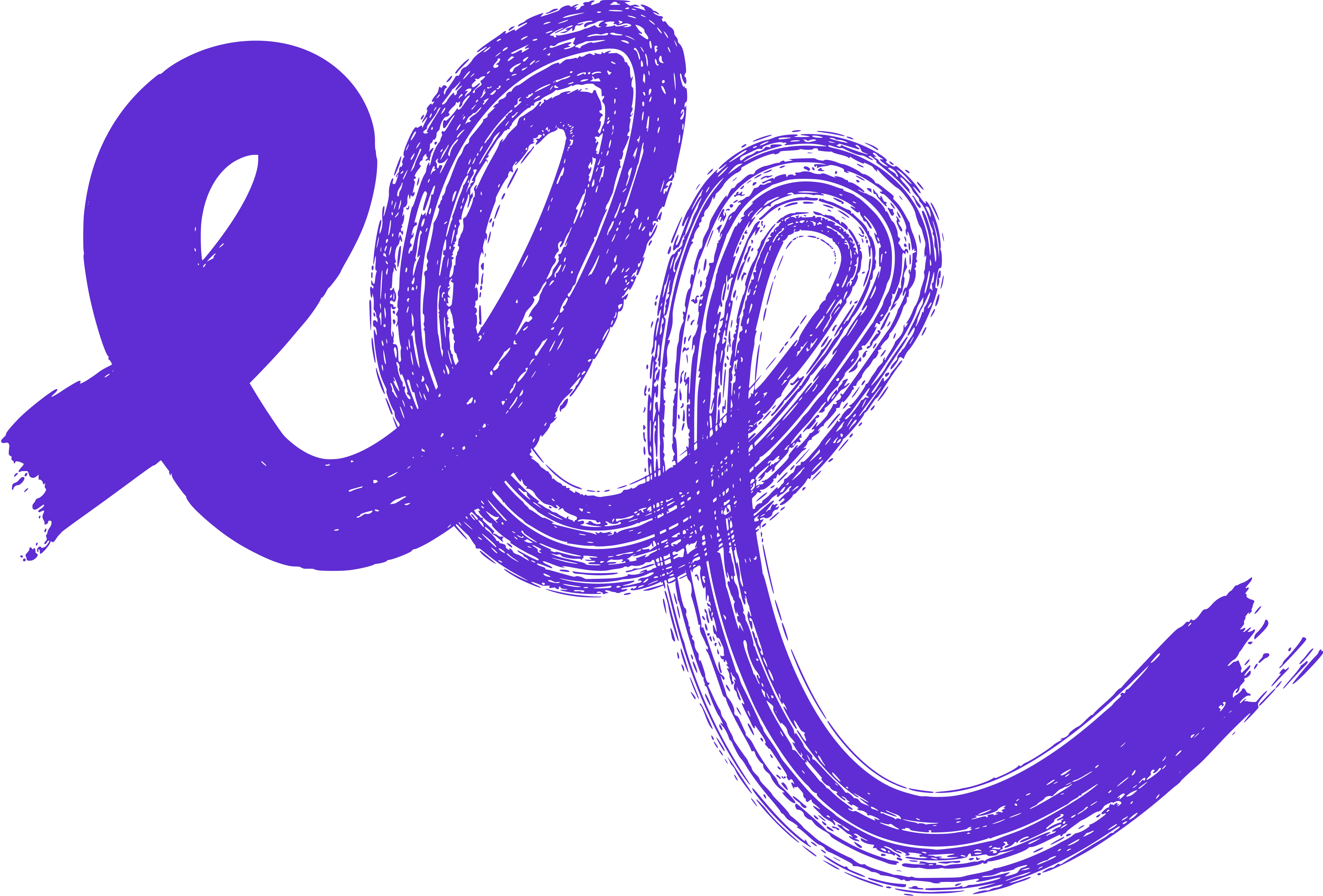