How to generate PDF from HTML in React / Node.js app
Eugene Zolotarenko
May 20, 2021・6 min read
Table of Content
Using native browser printing with CSS Print Rules
Making a Screenshot from the DOM (HTML => Canvas => Image => PDF)
Using PDF / JavaScript libraries
Using Puppeteer, Headless Chrome with Node.js
Conclusion
FAQs
Generating PDF from HTML would seem a rather simple action requiring little time and effort. However the reality is somewhat different, and finding the best solution can often be challenging.
Let’s consider this hypothetical scenario:
We have a React App through which we’d like to create a PDF either from the entire page or just in part. Our PDF document could contain charts, tables, images and/or plain text and should be structured without cuts or overlappings. We also want a button on the page allowing us to save our document.
In this article, I will walk you through some different solutions whilst outlining the pros and cons of each. We will start with the simplest method then graduate to the most complex.
Using native browser printing with CSS Print Rules
Generally speaking, a browser can already save and print PDFs from our pages: just press Ctrl/Cmd + P for the adjustable document pop-up by which you can customize its appearance.
Creating a button to perform the same action is as follows:
const SavePdfButton=()=>{
return (
<button onClick={window.print()}>Download PDF</button>
)
}
Should we wish to change its appearance, hide certain items or change the elements’ size in the PDF, we can write CSS print rules:
@media print {
.save-button {
display: none;
}
.content {
width: 100%;
}
}
We also might want to manage page breaks and/or eliminate overlappings. This can be achieved with some specific style properties as shown in this example:
@media print {
h1 {
break-before: always;
}
table, img, svg {
break-inside: avoid;
}
}
Here’s an excellent article describing more of what you can do using these print rules in CSS.
For something small and simple this is an ideal solution and one that would be over-engineered by the use of libraries. But it is not so ideal when access to code-generated documents is required.
Pros:
There are no external libraries
It is simple to implement
It does not overload the user's machine
It allows the user to select and search text
Cons:
It can be problematic rendering identical results in different browsers
Save buttons can be difficult to find owing to browser-rendering discrepancies
There is no access to code-generated PDFs
The PDF document content is dependent upon the size of the browser window
Making a Screenshot from the DOM (HTML => Canvas => Image => PDF)
Here’s another straightforward solution: just take a screenshot of the page or element and convert it to a PDF document with Canvas and image transformation:
html2canvas for creating a screenshot from HTML and generating Canvas from it
jsPDF to transform an image to PDF
We can make the Canvas => Image part with vanilla JavaScript. Accordingly, the function will look like this:
import html2canvas from 'html2canvas'
import jsPdf from 'jspdf';
function savePdf(){
const domElement=document.querySelector('#container')
html2canvas(domElement, { onclose: (document)=>{
document.querySelector('#save-button').style.visibility='hidden'
}})
.then((canvas)=>{
const img=canvas.toDataURL('image/jpeg')
const pdf=new jsPdf()
pdf.addImage(imgData, 'JPEG',0,0,width,height)
pdf.save('filename.pdf')
})
}
As you can see, it is possible to add some styles before the HTML => Canvas transformation.
However, if the HTML is lengthy you might want to relegate different elements to separate pages. To do this, create a screenshot from multiple elements and combine them into one PDF document:
import html2canvas from 'html2canvas'
import jsPdf from 'jspdf'
function savePdf(){
const domElements=document.querySelectorAll('.container')
const pdf=new jsPdf()
domElements.forEach((element,i)=>{
const img=canvas.toDataURL('image/jpeg')
pdf.addImage(imgData,'JPEG',0,0,width,height)
const isSectionLast=domElements.length===i+1;
if(isSectionLast){
pdf.save('filename.pdf')
}else{
doc.addPage()
}
}
}
This way, you can create decent PDFs looking just like the original HTML, and now have control over both the document’s appearance and the elements that can be included in it. The downside, however, is that there is still no capacity to select and search the text.
Pros:
It is highly similar to HTML
Easy implementation
It has access to generated PDF from code
Cons:
The user is unable to select and search text
The PDF document content is dependent upon the size of the browser window
External packages are required
Using PDF / JavaScript libraries
jsPDF (as mentioned), PDFKit, React-pdf are all libraries you can use to create PDF in React, however, a problem remains: that all HTML and CSS must be specifically created for your PDF document.
In our scenario, then, this solution is also insufficient since we prefer simply to copy our HTML with minor changes, not rewrite it with variations on the same design. Still, it is a useful option if you want to create a PDF from scratch using information from another source.
Here’s how it looks with React-pdf:
import {Page,Text,View,Document,StyleSheet} from "@reat-pdf/renderer';
const styles=StyleSheet.create({
page:{
backgroundColor: "#E4E4E4"
},
section:{
margin: 10,
}
})
const MyDocument=()=>{
<Document>
<Page size="A4" style={styles.page}>
<View style={styles.section}>
<Text>Section</Text>
</View>
</Page>
</Document>
}
Pros:
It gives access to generated PDF from code
The PDF document content is not dependent upon on the browser window size
The result is identical in different browsers
It is able to select and search text
Cons:
It is unable to copy the existing on-page HTML
It can be quite time-consuming.
The code is likely to contain two different variations of the same design
Using Puppeteer, Headless Chrome with Node.js
Given its code is written on the back-end, this solution is the most complex and unlike any of the fully client-side ones mentioned above.
In other words, the Puppeteer is a browser you can run from Node.js. And from the documentation, we see that it can be used to generate screenshots and PDFs of pages.
Here’s an example that navigates directly to the URL, changes some styles and generates a PDF file:
const puppeter=require('puppeteer')
async function savePdf(){
const browser=await puppeteer.launch({headless: true})
const page=await browser.newPage();
await page.goto('https://start-up.house',{waitUntil:'networkidle0'});
await page.addStyleTag({content: '#save-button {display: none}'})
const pdf=await page.pdf({format: 'A4'});
await browser.close();
return pdf;
}
Once created, the document is sent back to the front-end. On the client-side, it is then fetched, transformed to the blob and saved.
Like so:
function savePdf(){
return fetchPdfData().then((pdfData)=>{
const blob=new Blob([pdfData],{type: 'application/pdf'})
const link=document.createElement('a')
link.href=window.URL.createObjectURL(blob)
link.download='file-name.pdf'
link.click()
}
}
This seems the most comprehensive solution as it affords the greatest number of benefits and can address even the most difficult of cases. Moreover, the document it will generate allows the selecting and searching of text and can also be saved on the server without any additional API calls.
Pros:
It has access to generated PDF from code
The PDF document content is not dependent upon the size of the browser window
It allows you to select and search text
It is very similar to HTML
Does not overload the user's machine
Cons:
It requires client and server-side code
Implementation can be complicated in some cases
Conclusion
As we’ve seen, generating PDFs from HTML can be problematic. But it need not be, and the examples above are just a few options for you to consider when tackling the issue. With a bit of trial and error you’ll breach the impasse, so do try tinkering with some different options to determine which solution works best for you.
And... good luck!
If you would like to know more, contact us - hello@start-up.house
FAQs
How can I save my entire HTML page as a PDF document using React?
- Using various methods discussed in the article, you can convert your HTML page to a PDF document. One straightforward way is to use native browser printing with CSS print rules.
What libraries can assist in converting HTML to PDF in a React app?
- React PDF, PDFKit, and jsPDF are some popular libraries for this purpose. However, if you're specifically looking at the React PDF library, it's crucial to use the appropriate React PDF components to achieve the desired result.
Does exporting an HTML page to a PDF file retain the background color and custom fonts?
- It largely depends on the method you use. Some methods may not retain the background color and custom fonts, but others, especially those using Puppeteer or dedicated libraries, often do.
When I try to create a PDF document from my React app, the background color differs from my original HTML page. Why?
- The exact replication of the background color when converting an HTML page to a PDF document can be influenced by the method or library you're using. It's essential to ensure that the library or method you choose supports the preservation of background color.
Is it possible to export default app content from my React project to a PDF file?
- Yes, depending on the content and structure of your React app, you can use tools and libraries to export default app content into a PDF file seamlessly.
Can I convert an HTML string to a PDF document using jsPDF in a React app?
- Yes, with jsPDF, you can convert HTML, including HTML strings, to PDF documents. Pairing it with other tools or libraries can further streamline the conversion process in a React app.
What are the main differences between converting HTML to PDF and taking a screenshot using the DOM method in a React app?
- When converting HTML to PDF directly, the resultant PDF often allows text selection and searching. However, when using the screenshot method (HTML => Canvas => Image => PDF), the PDF is essentially an image, so text selection might not be possible.
How can I ensure consistent background color when converting my HTML page to a PDF document?
- Using dedicated libraries or tools that prioritize preserving styles, like Puppeteer or React PDF, can help ensure the background color remains consistent during the conversion.
Between creating a PDF file from scratch using React PDF and converting HTML to PDF, which method is more time-efficient?
- Converting existing HTML to PDF is generally faster as you're using existing content. Creating a PDF file from scratch using React PDF might be more time-consuming, especially if the design is intricate.
How do I handle large PDF files when converting a lengthy HTML page using React?
- If you're dealing with a lengthy HTML page, it's advisable to divide the content into sections or pages for better readability. Libraries like Puppeteer provide functionalities to manage and paginate long content effectively.
What is the 'html to pdf react' process in web development?
- The 'html to pdf react' process refers to the method of converting HTML content within a React application into a PDF document. This is typically done using libraries that capture the HTML structure and styling to create a PDF file that can be saved, printed, or shared.
Which libraries are commonly used for 'html to pdf react' conversions?
- Popular libraries for 'html to pdf react' conversions include jsPDF and html2canvas. These libraries work together to capture the HTML rendered by React components and convert it into a PDF format, maintaining the layout and styles as closely as possible.
Are there any challenges in the 'html to pdf react' conversion process?
- One of the main challenges in the 'html to pdf react' process is ensuring that the converted PDF accurately reflects the original HTML content in terms of layout, styles, and interactivity. Handling dynamic data and large documents efficiently is also a common challenge developers face.
How can I ensure high-quality output in the 'html to pdf react' process?
- To ensure high-quality output in the 'html to pdf react' process, choose reliable libraries, test the conversion with various types of content, and consider implementing custom solutions for complex layouts or dynamic data. Regular updates and optimization of the code can also contribute to better quality PDFs.
Is it possible to handle interactive content in 'html to pdf react' conversions?
- While basic interactivity can be handled in the 'html to pdf react' process, complex interactive elements like hover effects or clickable links may not be fully replicated in the PDF. The focus is usually on accurately capturing the visual presentation rather than the interactive aspects.
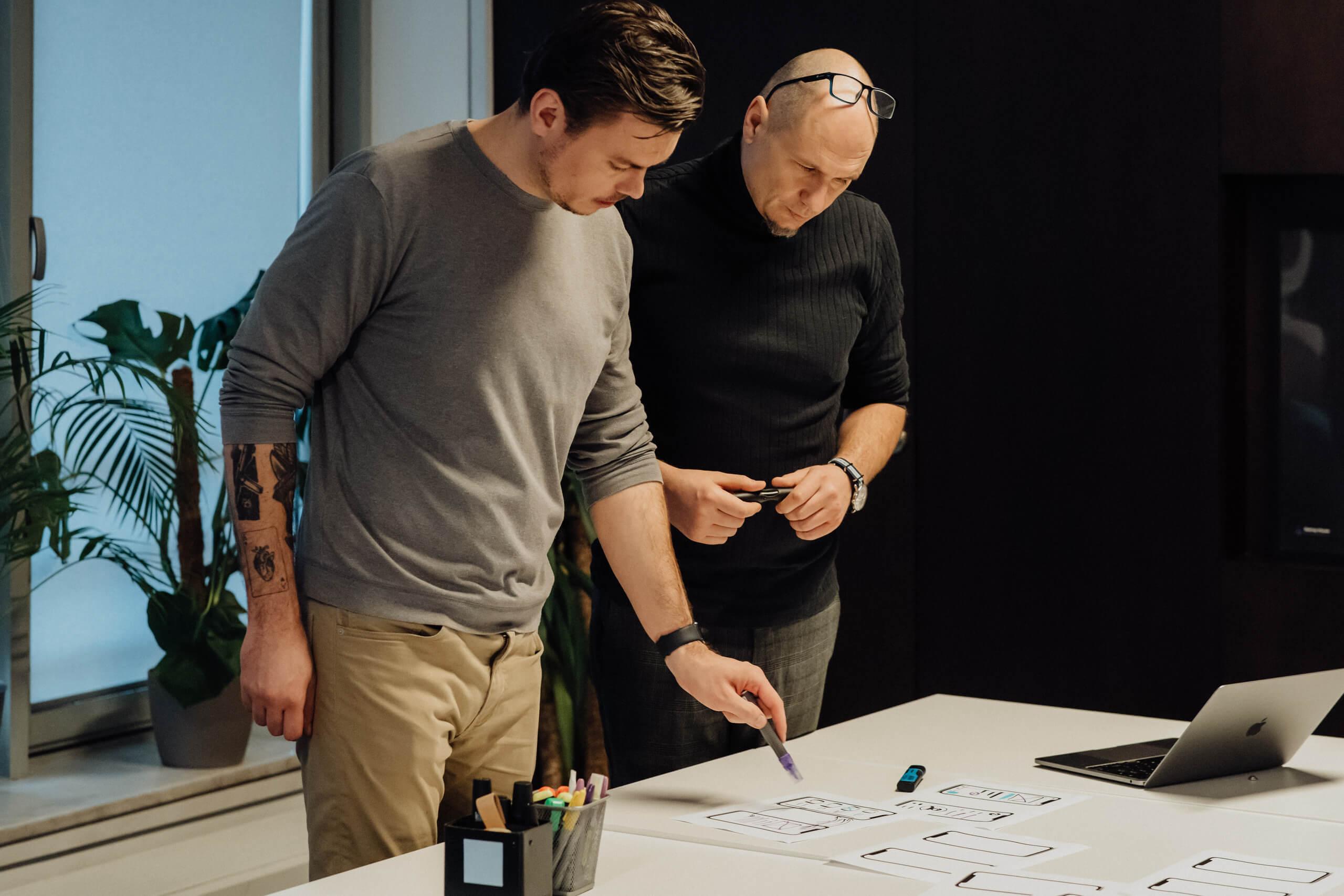
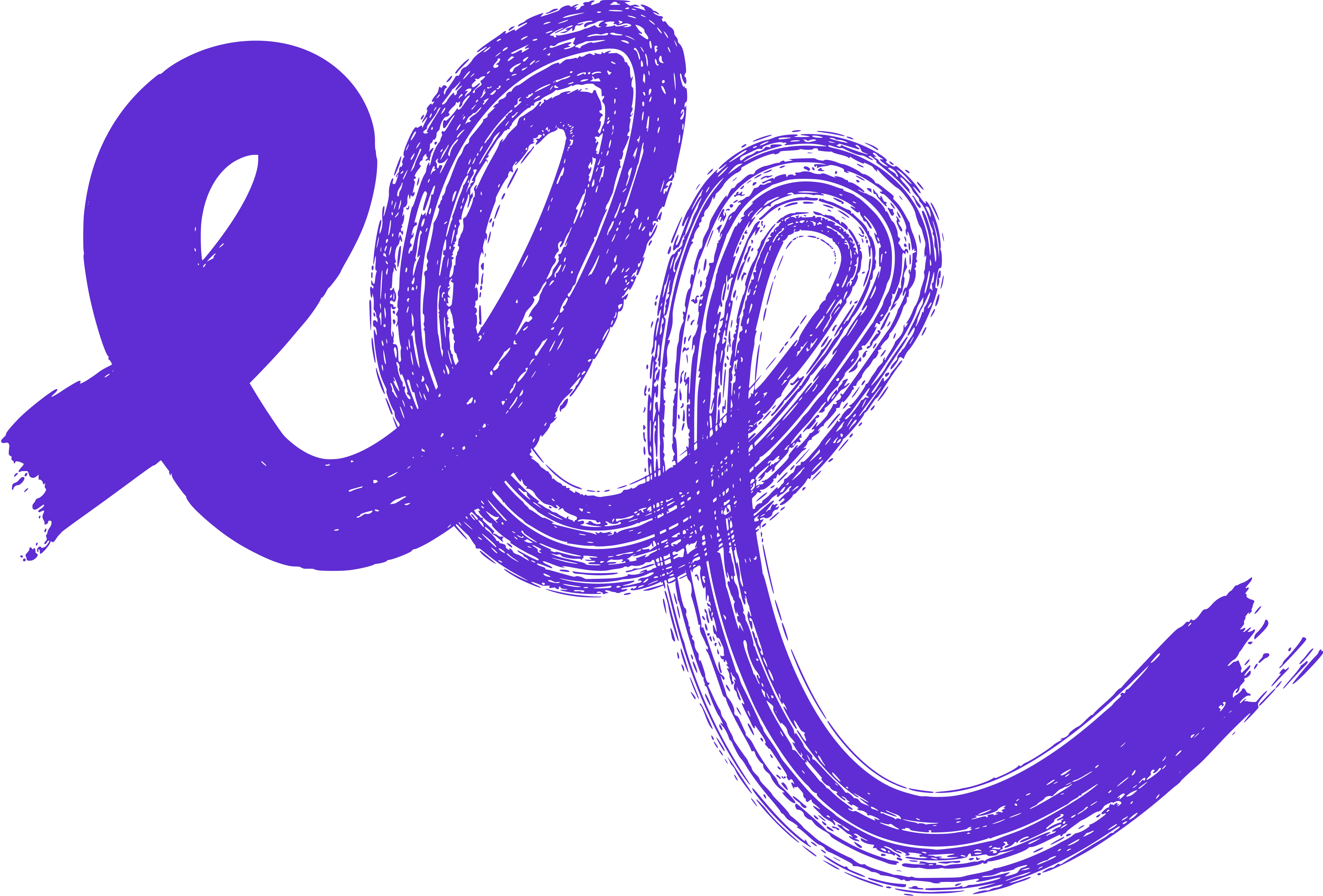
You may also
like...
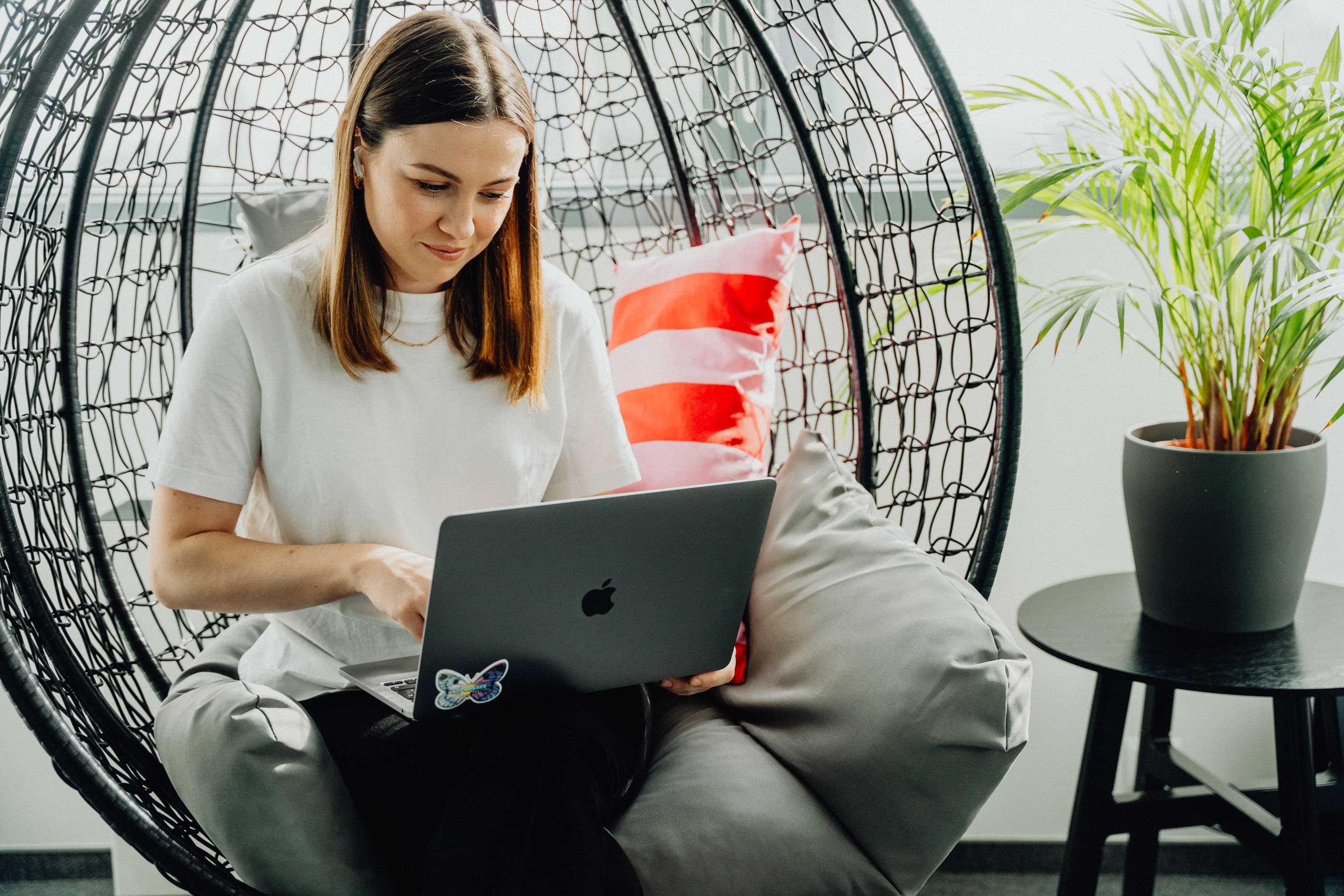
Navigating the JavaScript Jungle: A Clear Guide to React, Angular, and Vue.js
React, Angular, and Vue.js are leading JavaScript frameworks for building web applications. React excels with a component-based architecture, Angular provides a structured framework for complex apps, and Vue.js offers simplicity and flexibility. Choosing the right tool depends on project needs, scalability, and team expertise.
Marek Majdak
Nov 25, 2024・10 min read
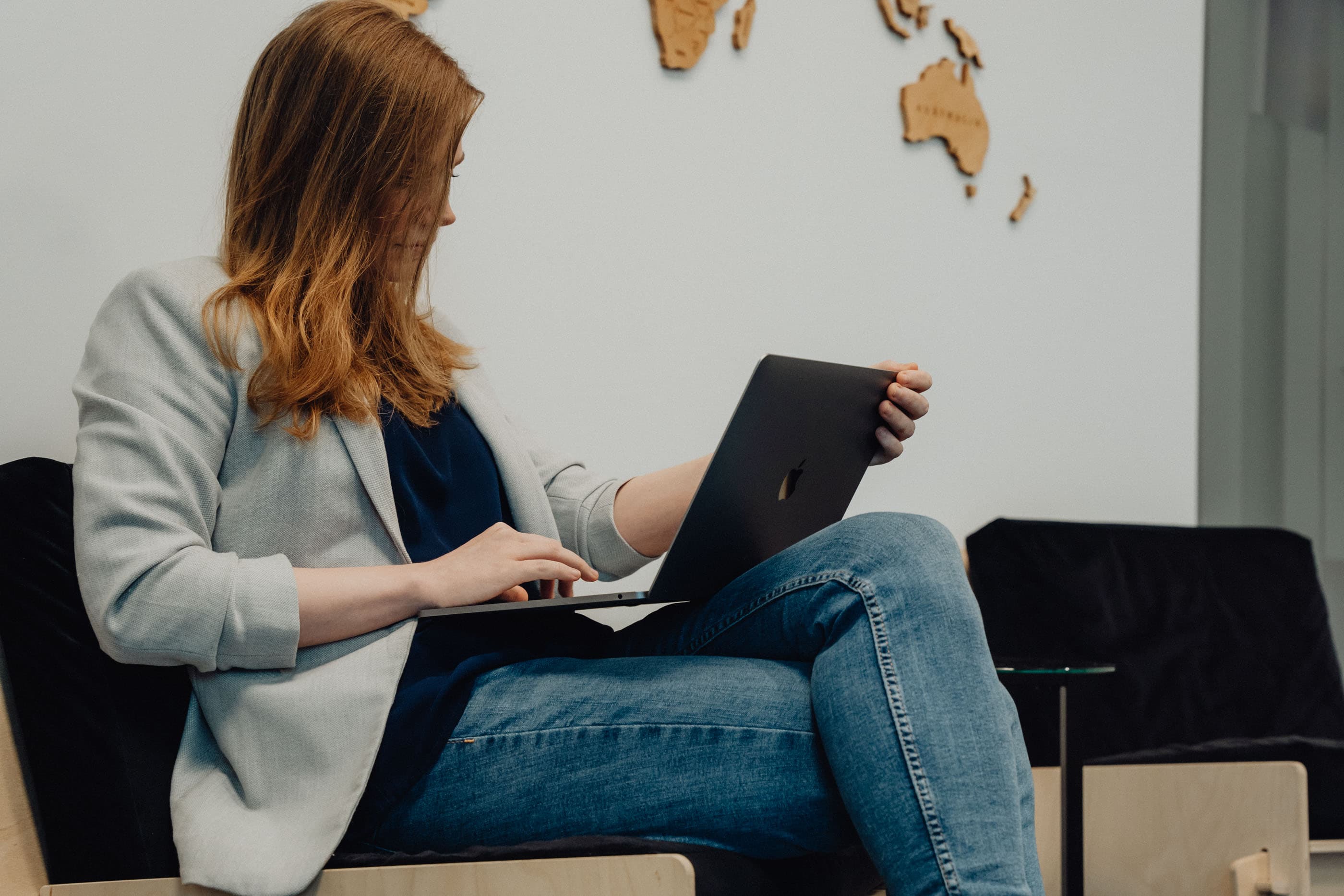
Node.js: The Backbone of Modern Web Applications
Node.js revolutionizes web development by offering a JavaScript runtime for creating high-performance, scalable server-side applications. With its non-blocking, event-driven architecture and a robust npm ecosystem, Node.js is a go-to technology for developers building modern web applications.
Alexander Stasiak
Jun 04, 2024・5 min read
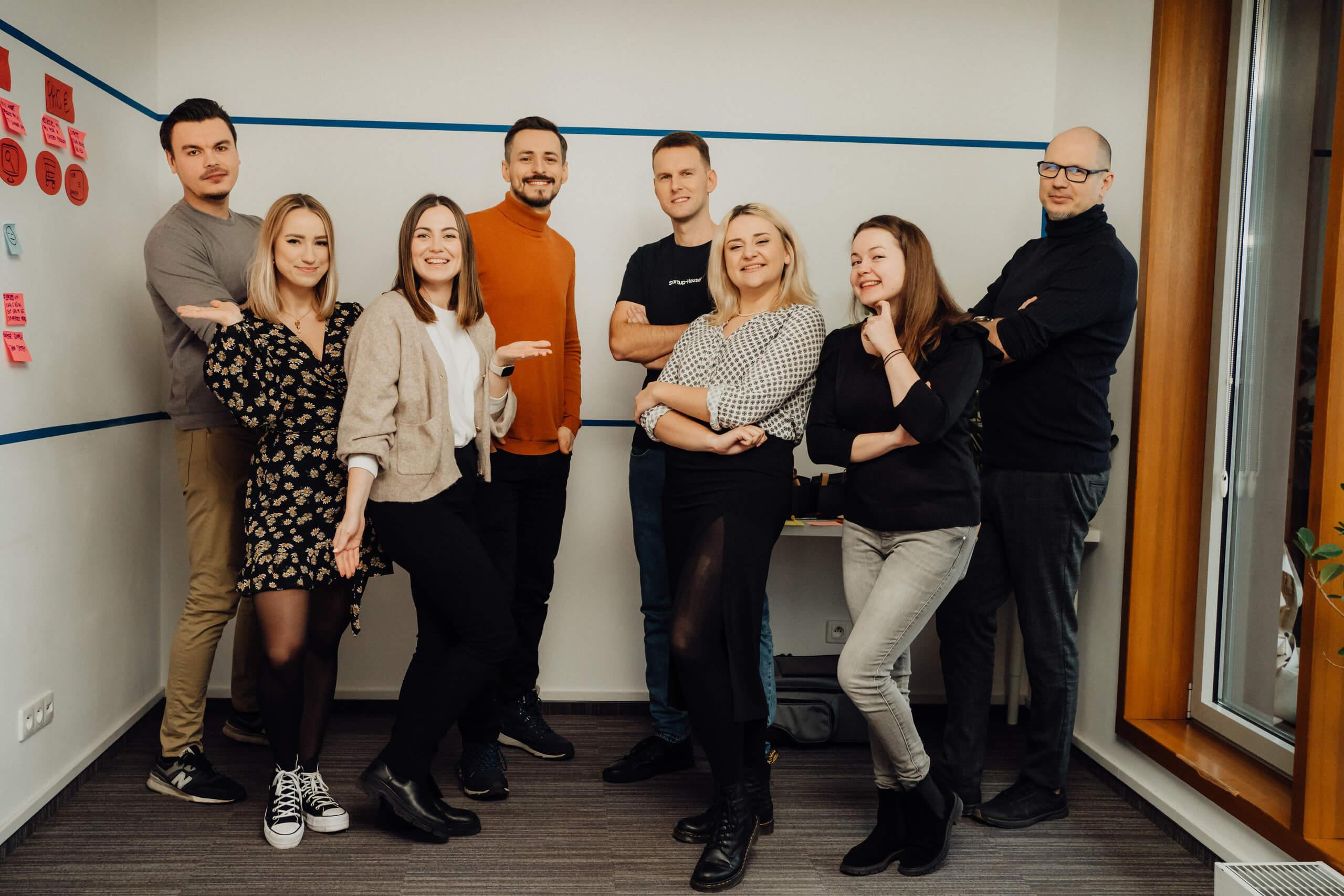
Understanding React: What is it and Why is it Important?
This article provides an introduction to React, explaining its purpose and importance in web development. It explores React's key principles, such as component-based architecture and virtual DOM, and highlights its benefits, including improved performance and scalability. The article also discusses the different types of React components and concludes with an overview of React's popularity and its role in modern web development.
Marek Majdak
May 26, 2022・9 min read