🌍 All
About us
Digitalization
News
Startups
Development
Design
Understanding React: What is it and Why is it Important?
Marek Majdak
May 26, 2022・9 min read
Table of Content
Key principles of React
Virtual DOM
Components
JSX
One-Way Data Flow
React Hooks
Performance
Scalability
Ease of Use
Getting Started with React: Step-by-Step Guide for Beginners
Step 1: Set Up Your Development Environment
Step 2: Explore the Project Structure
Step 3: Start the Development Server
Step 4: Make Changes and See Them Live
Step 5: Learn and Explore Further
React is a popular JavaScript library for building user interfaces, developed and maintained by Facebook. It is widely used in web development due to its efficiency, flexibility, and reusability. React allows developers to build interactive and dynamic user interfaces by breaking them down into reusable components.
So, what exactly is React? In simple terms, React is a JavaScript library that helps developers build user interfaces by creating reusable components. These components are like building blocks that can be combined to create complex UIs. React follows a component-based architecture, where each component has its own logic and state. This modular approach makes it easier to manage and maintain large-scale applications.
Why is React important? React revolutionized the way we build web applications by introducing the concept of a virtual DOM (Document Object Model). The virtual DOM is a lightweight copy of the real DOM, which allows React to efficiently update and render only the necessary components when changes occur. This results in faster and more efficient rendering, improving the overall performance of the application.
React's popularity can also be attributed to its strong ecosystem and community support. It has a vast collection of open-source libraries and tools that extend its functionality and simplify development tasks. Additionally, React has a large and active community of developers who contribute to its growth and provide support through forums, tutorials, and documentation.
Key principles of React
• Component-based architecture: React encourages developers to break down the user interface into reusable components, making it easier to manage and maintain complex applications.
• Virtual DOM: React uses a virtual DOM to efficiently update and render components, resulting in improved performance and faster rendering.
• Unidirectional data flow: React follows a unidirectional data flow, where data flows from parent components to child components, making it easier to track and manage data changes.
• Declarative syntax: React uses a declarative syntax, allowing developers to describe how the UI should look based on the current state, rather than imperatively defining each step.
Components are the building blocks of React applications. They can be classified into two types:
• Functional components:
These are stateless components that are defined as JavaScript functions. They accept props (properties) as input and return JSX (JavaScript XML) to define the UI.
• Class components:
These are stateful components defined as ES6 classes. They have their own state and lifecycle methods, allowing for more advanced functionality.
In conclusion, React is a powerful JavaScript library that revolutionized the way we build user interfaces. Its component-based architecture, virtual DOM, and declarative syntax make it a popular choice for building efficient and scalable web applications. Understanding React and its key principles is essential for any developer looking to excel in modern web development. Exploring the Basics: Key Concepts and Features of React
React is a popular JavaScript library for building user interfaces. It was developed by Facebook and is widely used by developers around the world. In this section, we will explore the key concepts and features of React that make it such a powerful tool for developing web applications.
Virtual DOM
One of the fundamental concepts in React is the Virtual DOM. The Virtual DOM is a lightweight representation of the actual DOM (Document Object Model) of a web page. React uses the Virtual DOM to efficiently update and render components.
When a user interacts with a React application, the Virtual DOM is updated instead of directly manipulating the actual DOM. React then compares the changes in the Virtual DOM with the actual DOM and updates only the necessary parts, minimizing the number of changes required and improving performance.
Components
React is based on the concept of reusable components. A component in React is a self-contained unit that can be composed together to build complex user interfaces. Components can be thought of as custom HTML elements with their own logic and state.
React components can be divided into two types: functional components and class components. Functional components are simple functions that return JSX (JavaScript XML), which is a syntax extension for JavaScript. Class components, on the other hand, are ES6 classes that extend the React.Component class and have additional features like state and lifecycle methods.
JSX
JSX is a syntax extension for JavaScript that allows you to write HTML-like code within your JavaScript code. It is a key feature of React and provides a concise and expressive way to describe the structure and appearance of user interfaces.
With JSX, you can write code like this:
function App() {
return (
<div>
<h1>Hello, World!</h1>
<p>This is a React application.</p>
</div>
);
}
JSX is not required to use React, but it is highly recommended as it makes the code more readable and easier to understand.
One-Way Data Flow
React follows the principle of one-way data flow, also known as unidirectional data flow. This means that data flows in a single direction, from parent components to child components.
In React, data is passed from parent components to child components through props (short for properties). Props are immutable and cannot be changed by the child components. This ensures that the data flow is predictable and helps to maintain the integrity of the application's state.
React Hooks
React Hooks are a relatively new addition to React and provide a way to use state and other React features in functional components. Hooks allow developers to write reusable logic and share stateful logic between components without using class components.
Some of the most commonly used React Hooks include useState, useEffect, useContext, and useCallback. These hooks enable developers to manage state, perform side effects, and access context in functional components.
Overall, React is a powerful and flexible library for building user interfaces. Its key concepts, such as the Virtual DOM, components, JSX, one-way data flow, and React Hooks, make it a popular choice among developers for creating dynamic and interactive web applications. 3. React vs. Other Frameworks: A Comparative Analysis
When it comes to web development, choosing the right framework can make a significant difference in the success of your project. React, a JavaScript library developed by Facebook, has gained immense popularity in recent years. In this section, we will explore how React compares to other frameworks in terms of performance, scalability, and ease of use.
Performance
One of the key advantages of React is its exceptional performance. React utilizes a virtual DOM (Document Object Model) that allows it to efficiently update and render components. This approach minimizes the number of actual DOM manipulations, resulting in faster rendering times and improved overall performance. Additionally, React's one-way data flow ensures that changes in the application's state are efficiently propagated through the component tree, further enhancing performance.
Compared to other frameworks such as Angular and Vue.js, React's performance is often considered superior. While Angular and Vue.js also offer efficient rendering mechanisms, React's virtual DOM implementation sets it apart in terms of speed and responsiveness.
Scalability
Scalability is a crucial factor to consider when choosing a framework for your project. React's component-based architecture makes it highly scalable and maintainable. By breaking down the user interface into reusable components, React allows developers to efficiently manage complex applications. Each component can be developed independently and then combined to create a cohesive and scalable user interface.
Furthermore, React's ecosystem provides a wide range of tools and libraries that facilitate the development and maintenance of large-scale applications. The availability of frameworks such as Next.js and Gatsby.js enables developers to build server-rendered and static websites, respectively, with ease.
Ease of Use
React's simplicity and ease of use have contributed to its widespread adoption. The library's declarative syntax allows developers to describe the desired outcome rather than focusing on the step-by-step process of achieving it. This approach makes React code more readable and easier to maintain.
Moreover, React's component-based architecture promotes code reusability, reducing the amount of code duplication and increasing development efficiency. The availability of a vast number of community-created components and libraries further simplifies the development process.
In conclusion, React stands out among other frameworks due to its exceptional performance, scalability, and ease of use. Its virtual DOM implementation ensures fast rendering times, while its component-based architecture enables developers to build scalable and maintainable applications. React's simplicity and extensive ecosystem make it a preferred choice for many developers.
However, it is important to note that the choice of framework ultimately depends on the specific requirements of your project. While React may be the ideal choice for some applications, other frameworks such as Angular or Vue.js may better suit different scenarios. Therefore, it is crucial to carefully evaluate your project's needs and consider the strengths and weaknesses of each framework before making a decision. ers
Getting Started with React: Step-by-Step Guide for Beginners
React is a popular JavaScript library that is widely used for building user interfaces. It was developed by Facebook and has gained immense popularity among developers due to its simplicity and efficiency. In this step-by-step guide, we will walk you through the process of getting started with React, from setting up your development environment to creating your first React application.
Step 1: Set Up Your Development Environment
Before you can start building React applications, you need to set up your development environment. The first step is to ensure that you have Node.js installed on your computer. Node.js is a JavaScript runtime that allows you to run JavaScript code outside of a web browser. You can download and install Node.js from the official website.
Once you have Node.js installed, you can use the Node Package Manager (NPM) to install create-react-app, a command-line tool that helps you create a new React application with a basic template. Open your terminal or command prompt and run the following command:
npx create-react-app my-react-app
This command will create a new directory called my-react-app and set up a basic React application inside it. Once the command finishes running, navigate to the newly created directory by running the following command:
cd my-react-app
Step 2: Explore the Project Structure
Now that you have set up your React application, let's take a look at the project structure. Inside the my-react-app directory, you will find several files and folders. The most important ones are:
• src:
This folder contains the source code of your React application. It includes JavaScript files, CSS files, and other assets.
• public:
This folder contains static files that will be served by your application, such as the HTML file that is rendered in the browser.
• package.json:
This file contains metadata about your application and the dependencies it requires.
Feel free to explore the project structure and familiarize yourself with the different files and folders. Understanding the project structure will help you navigate and work with your React application more efficiently.
Step 3: Start the Development Server
Now that you have set up your development environment and explored the project structure, it's time to start the development server and see your React application in action. In your terminal or command prompt, run the following command:
npm start
This command will start the development server and open your React application in your default web browser. You should see a Welcome to React message displayed on the screen.
Step 4: Make Changes and See Them Live
One of the great features of React is its ability to update the user interface in real-time as you make changes to your code. Open the src/App.js file in your favorite code editor and modify the content inside the <div className=App> tags. Save the file and go back to your web browser. You should see the changes reflected immediately without having to refresh the page.
Step 5: Learn and Explore Further
Congratulations! You have successfully set up your development environment, created a new React application, and made changes to the user interface. This is just the beginning of your journey with React.
To further enhance your React skills, you can explore the official React documentation, which provides detailed explanations of React's key concepts and features. Additionally, there are numerous online tutorials, courses, and communities where you can learn from experienced developers and get answers to your questions.
Remember, practice makes perfect. The more you build React applications and experiment with different features, the better you will become. So keep coding and enjoy your journey with React! React in Action: Real-World Examples and Use Cases
React, being one of the most popular JavaScript libraries, has gained immense popularity due to its flexibility and efficiency. It has been widely adopted by various companies and developers to build interactive and dynamic user interfaces. In this section, we will explore some real-world examples and use cases where React has been successfully implemented.
1. Single Page Applications (SPAs)
React is particularly suitable for building Single Page Applications (SPAs) where the entire application runs on a single web page, providing a seamless and responsive user experience. Companies like Facebook, Instagram, and Airbnb have utilized React to create highly interactive and dynamic SPAs.
2. E-commerce Websites
E-commerce websites require a smooth and efficient user interface to handle a large number of products and customer interactions. React's virtual DOM and component-based architecture make it an ideal choice for e-commerce platforms. Companies like Amazon, Walmart, and Etsy have leveraged React to enhance the shopping experience for their customers.
3. Social Media Platforms
Social media platforms heavily rely on real-time updates and user interactions. React's ability to efficiently handle and update components based on data changes makes it a perfect fit for building social media applications. Facebook, the creator of React, uses it extensively in their own platform, ensuring a seamless user experience.
4. Collaborative Tools
Collaborative tools like Google Docs, Trello, and Slack require real-time updates and synchronization between multiple users. React's ability to handle complex state management and efficiently update the user interface makes it an excellent choice for building such collaborative tools.
5. Mobile Applications
React Native, a framework built on top of React, allows developers to build native mobile applications using JavaScript. This enables code reusability and faster development cycles. Many popular mobile applications, including Instagram, Airbnb, and UberEats, have been developed using React Native.
6. Dashboards and Data Visualization
React's component-based architecture and extensive ecosystem of libraries make it a powerful tool for building dashboards and data visualization applications. Companies like Netflix, Spotify, and Airbnb utilize React to create visually appealing and interactive dashboards to present complex data in a user-friendly manner.
7. Progressive Web Applications (PWAs)
Progressive Web Applications (PWAs) combine the best of web and native applications, providing an app-like experience within a web browser. React's ability to create reusable components and manage state efficiently makes it an excellent choice for developing PWAs. Companies like Twitter and Pinterest have adopted React to build their PWAs, offering a seamless user experience across different devices.
In conclusion, React's versatility and performance have made it a preferred choice for a wide range of applications. From single page applications to mobile apps and data visualization tools, React has proven to be a powerful and reliable framework for building modern and interactive user interfaces.
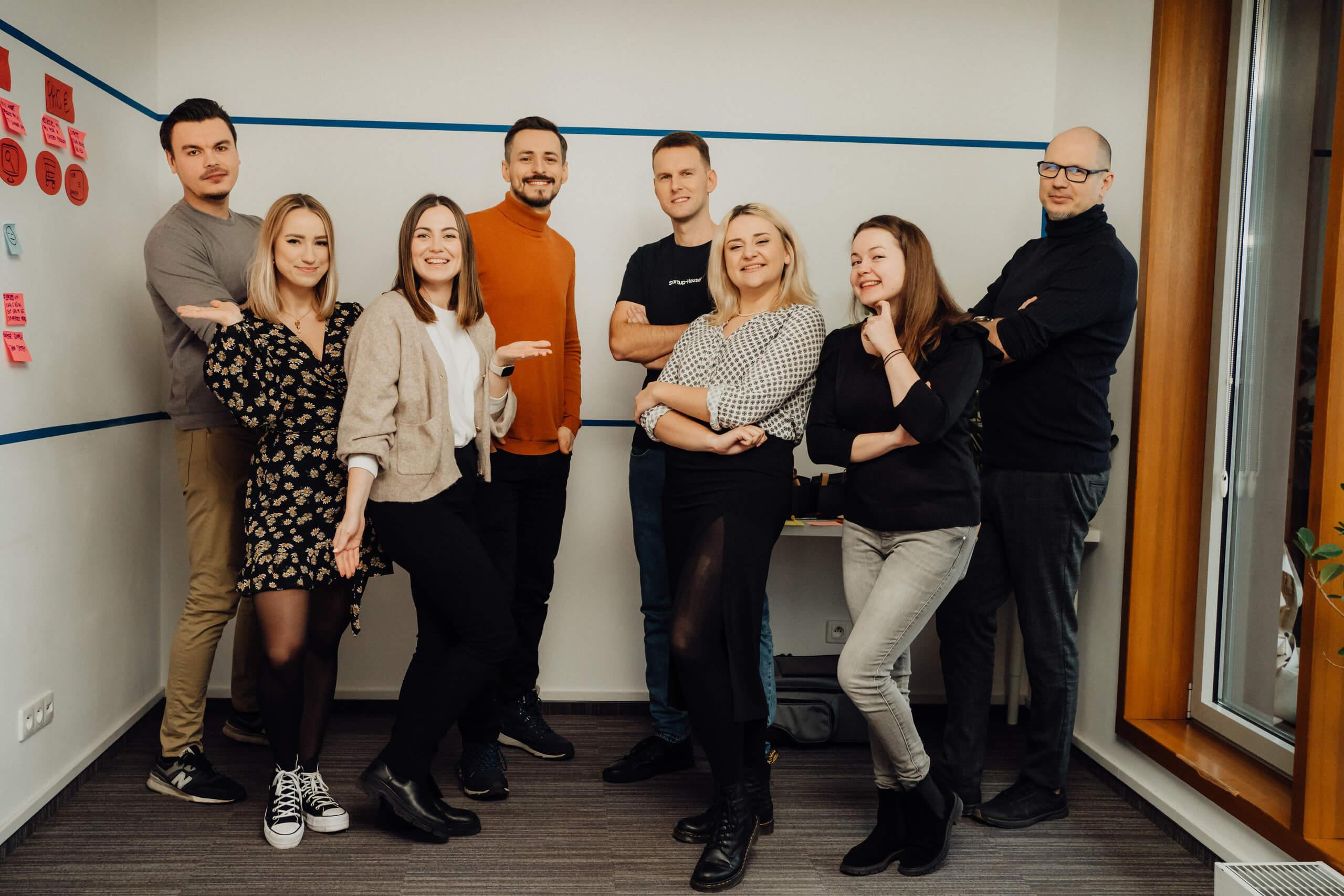
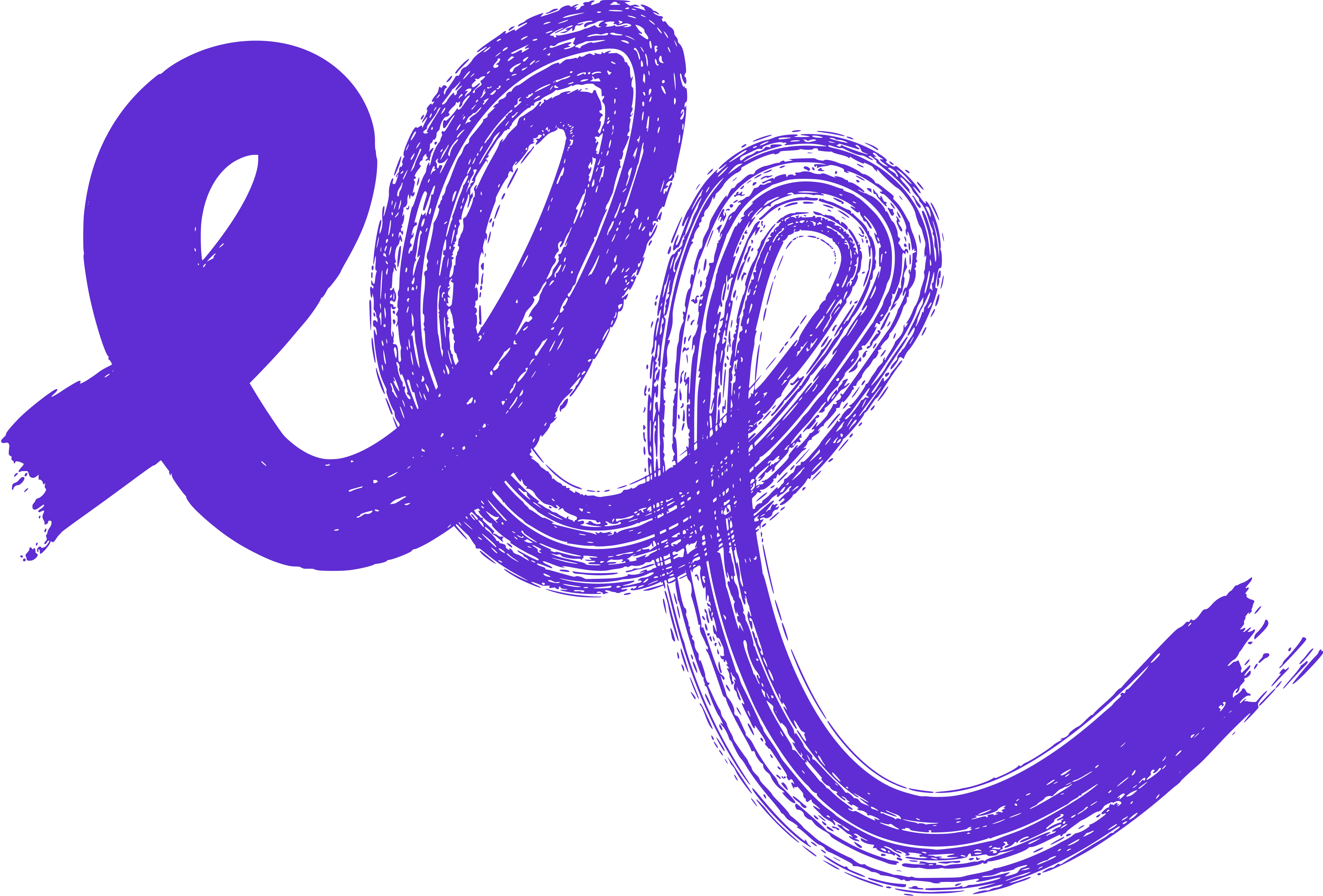
You may also
like...
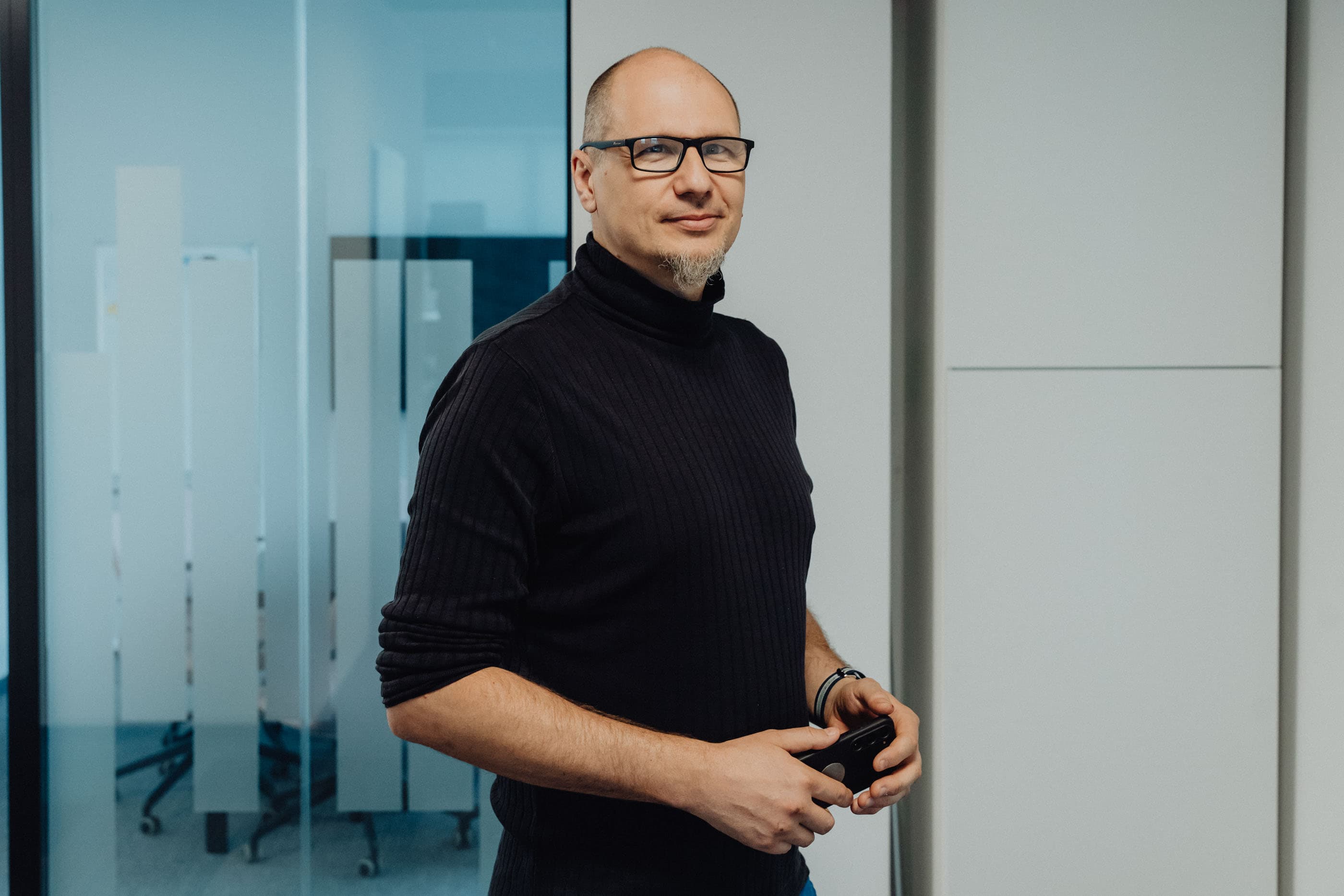
How to Hire Mobile App Developers: A Practical Guide for Businesses
Hiring the right mobile app developers is crucial for building a strong mobile presence. This guide provides a comprehensive overview of the steps involved in hiring skilled developers who can deliver on your business goals. From defining your project scope and identifying key skills to finding developers and evaluating their portfolios, we cover it all. Learn how to conduct effective interviews, assess technical skills, and evaluate cultural fit to ensure you make the right hiring decisions. Finally, discover tips on negotiating terms and successfully onboarding your new developers to set the stage for productive collaboration.
Marek Pałys
Mar 25, 2024・7 min read
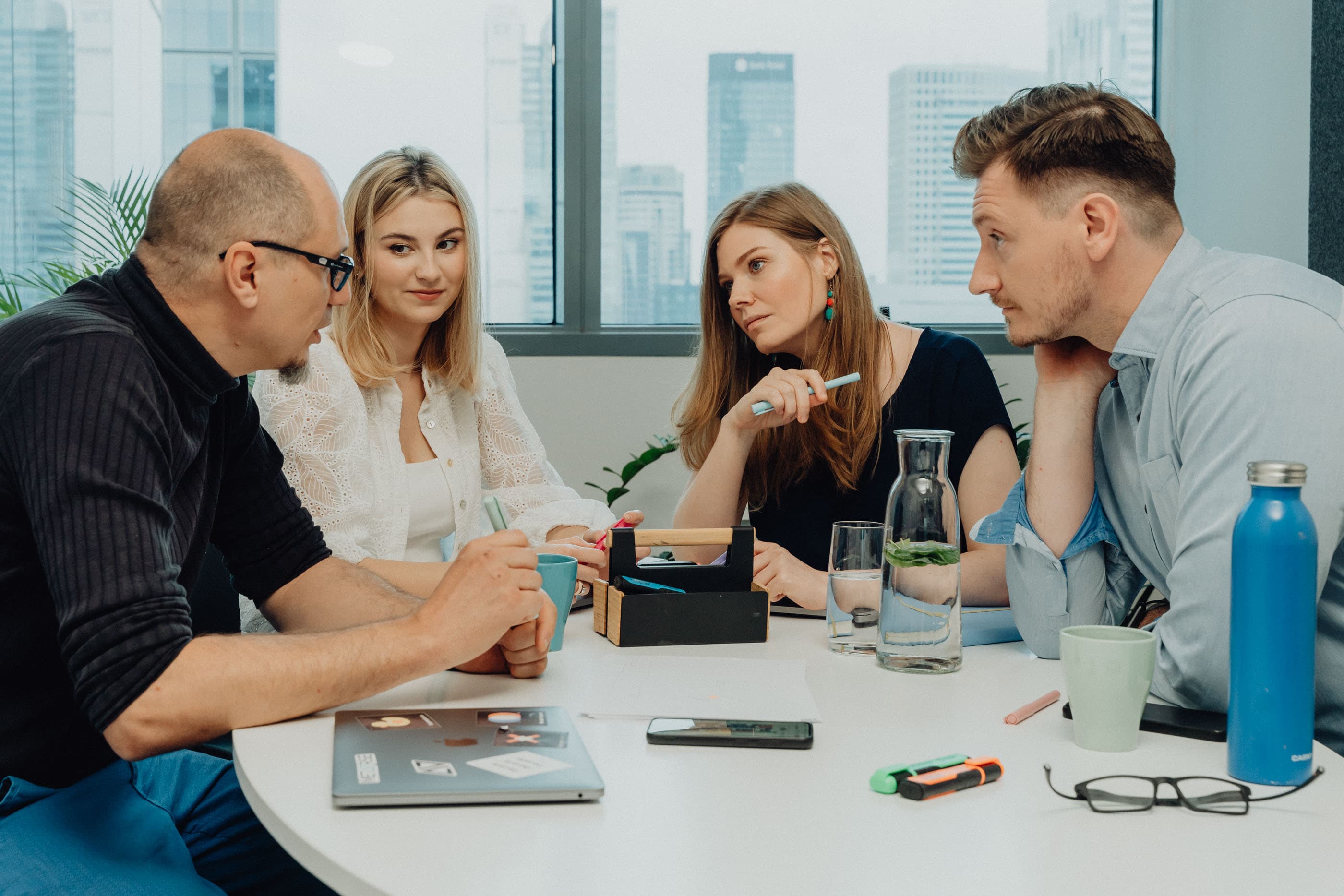
How to Hire Coders: A Straightforward Guide for Every Business
Hiring coders can be challenging in today's fast-paced digital landscape. This guide provides practical strategies to help you navigate the hiring process, from defining your project requirements and identifying essential skills to finding and evaluating candidates. Whether you're a startup building your first app or an established company enhancing your digital presence, this guide will help you make confident hiring decisions. Learn how to create competitive offers, provide necessary resources, and build a collaborative environment to ensure your new coders succeed.
Marek Pałys
Apr 02, 2024・8 min read
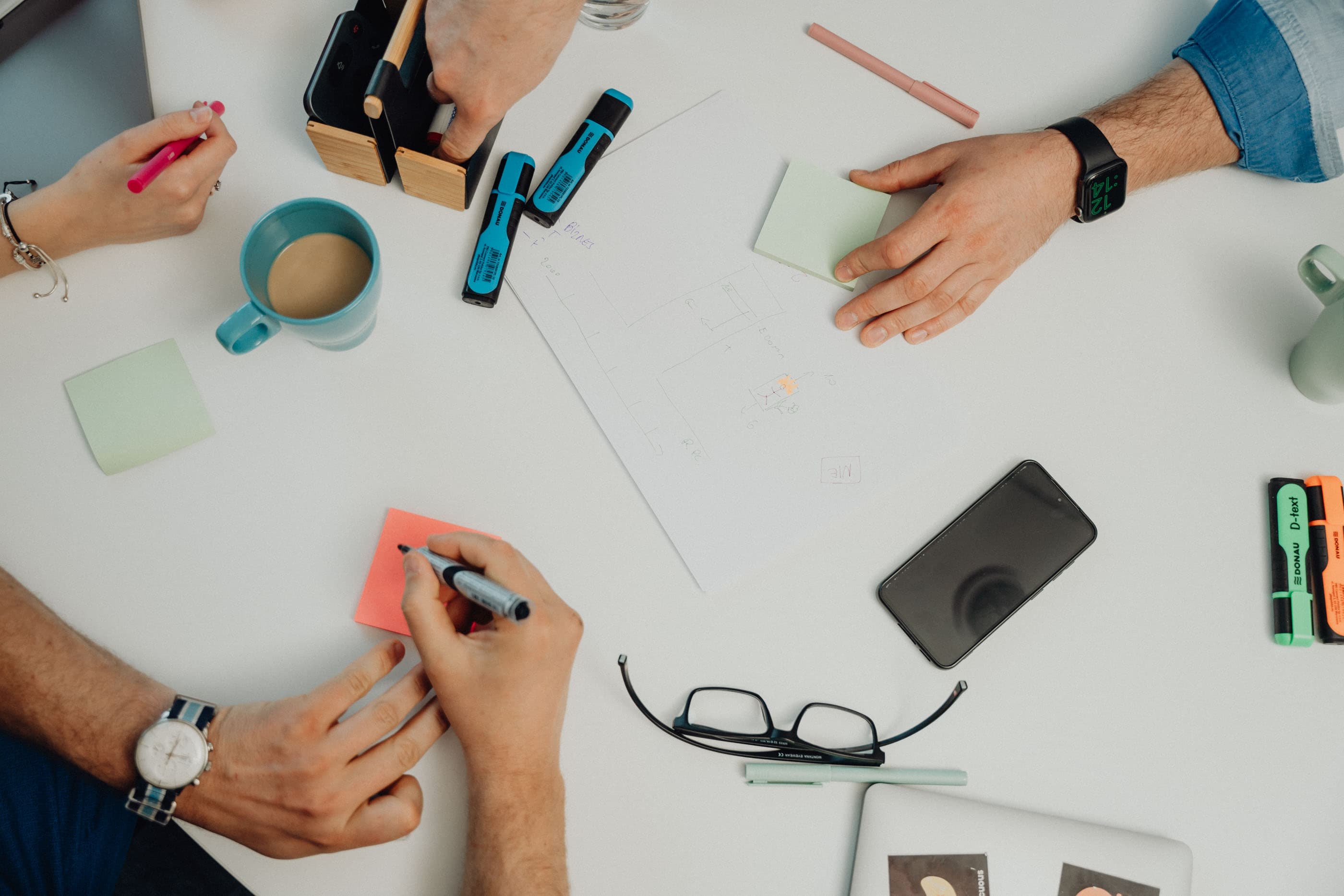
How to Hire a Software Developer: A Step-by-Step Guide for Success
Hiring a software developer is critical for the success of your project, whether you’re a startup or an established company. This guide provides a practical approach to finding and securing the right talent, from defining your project scope and identifying the necessary skills to crafting a compelling job description and conducting effective interviews. By following these steps, you can ensure a smooth hiring process and build a strong development team that meets your business goals.
Marek Pałys
Jun 28, 2024・5 min read