🌍 All
About us
Digitalization
News
Startups
Development
Design
Mastering Python Dependency Injection
Marek Majdak
Oct 14, 2023・16 min read
Table of Content
Introduction to Python Dependency Injection
Benefits of Using Dependency Injection in Python
Implementation of Dependency Injection in Python
Examples and Use Cases
Best Practices for Using Python Dependency Injection
Imagine trying to photograph a beautiful landscape…at midnight. You have the perfect camera, the perfect scenery, but without light, none of it matters. Just as light brings clarity to objects in darkness, dependency injection illuminates your Python code. It enhances modularity and flexibility, improving how software components interact within an application. Intrigued? Read ahead!
Introduction to Python Dependency Injection
As real-world systems expand and evolve continually, so does the realm of software engineering. Navigating through this digital chaos requires robust techniques that promote not just cursory designs but sustainable solutions. Enter Dependency Injection (DI), a vital concept for achieving improved code quality.
Unraveling Dependency Injection and Its Importance in Software Development
Dependency Injection(DI) essentially is a design pattern that establishes a standard mechanism for defining how different parts of your program communicate with each other. Think of it as constructing lego blocks where each block can freely connect with others based on mutual compatibility rather than being forced together.
DI decouples software modules, paving the way for successful implementation of SOLID principles:
- Single Responsibility Principle
- Open-closed Principle
- Liskov's Substitution Principle
- Interface Segregation Principle
- Dependency Inversion Principle
Embracing these principles leads to cleaner architectures interacting through simple interfaces rather than complex integrate heaps.
With meritorious benefits such as seamless module integration, easier debugging/testing operations and increased reusability - the dependency injection principle holds severe significance in modern-day software development regimes.
How Does Dependency Injection Work its Magic in Python?
Python presents programmers with flexible procedures when implementing DI ranging from simple function calls to sophisticated dependency injection pattern frameworks like PyInjector or Injector.
Primarily centered around three actors: client, interface(the dependency injector' class), and services(the dependent class), DI works by injecting dependencies(service objects) into client classes via constructors, properties(setter methods), or using interfaces. This process elicits a structural setup promoting object creation and binding outside the client's boundary, leading to profoundly modularized Python modules with limited inter-dependency.
In simpler terms, just like a syringe injected automatically injects prescribed medicine into your system reacting accordingly -dependency injection provides specific services(objects) into the main program(recipeint), serving all the requested functionalities.
Benefits of Using Dependency Injection in Python
Understanding the potential benefits of utilizing python dependency injection can usher you into a new realm of refined programming. It's like opening a door to discover an elegant and efficient approach, wherein solutions are neat, well-structured and easier to manage. Let’s unravel some notable benefits:
Improved Modularity and Reusability of Code
One pivotal advantage derived from employing python dependency injection is the enhanced modularity it offers. By disentangling dependencies or cross-connected components, each part operates independently, resulting in more modular code.
For our non-programmer readers: imagine building a house where every room can stand alone; if one room doesn't function right, you only have that specific area to fix without impacting the rest of the structure. That's essentially what modularity brings to your codebase!
Furthermore, facilitating such independency naturally gifts us reusable components. Picture having certain functions prebuilt - you use them wherever applicable with no extra configuration file modifications needed! This not only saves time but also induces consistency throughout the application.
Easier Testing and Debugging
Next, we turn towards testing - another critical stage impacted by python dependency injection. With your application broken down into smaller independent units due to improved modularity as discussed above, testing evolves into a less daunting task.
The principle revolves around unit-testing - checking each section piecemeal for bugs and slips ahead of their integration. Think about it: wouldn’t troubleshooting a snapshot became easier than scrutinizing an entire photo album? Moreover, any errors detected along this phase become markedly simpler to debug as they’re localized to confined modules rather than scattered over vast expanses of code.
Increased Flexibility and Scalability
Finally comes flexibility and scalability – key markers for modern software development practices—curveballs are common instances during coding when requirements modify overnight or complexity scales out rapidly.
But here’s how python dependency injection comes in handy: owing largely again to the modularity it promotes, developers gain the flexibility to swap out components effortlessly. Imagine switching your home furniture without disrupting any other part of the room.
On a similar note – updating or scaling applications becomes more manageable. Thanks to our modular approach, increasing system capacity by adding or upgrading certain parts doesn’t necessitate rewriting entire sections of code, making scalability an achievable goal without inflated costs.
So there you have it – improved modularity, easier testing and increased flexibility are just some of the benefits that make python dependency injection a significant chapter in Python's expansive plotline!
Implementation of Dependency Injection in Python
In my quest to help fellow developers better immerse themselves in the world of software development, I'll delve into the subject of python dependency injection. In this section, more specifically, we'll talk about different methods and how they fit snugly within various frameworks available for implementing dependency injection in Python.
Different Methods and Frameworks for Implementing Dependency Injection in Python
Python offers a myriad of straightforward techniques to achieve dependency injection. The most prominent ones include using constructors, setters or properties, and interface to implement dependency injection in.
Constructors: One way is by passing dependencies via a class constructor during initialization.
Setters or Properties: Alternatively, you can use setter methods or properties to inject dependencies after an object has been instantiated.
Interface Injection: In unique situations that require real-time updates on dependencies, interface injection method steps in as a savior.
Moreover, such processes are made convenient with existing python dependency injection frameworks providing built-in functionalities allowing smooth execution. These include popular options like PyInjector, Injector, dependable, wiring.
These tools optimize your code structure while promoting modular design principles and easier testing capability.
Comparison of Popular Dependency Injection Frameworks (e.g., PyInjector, Injector)
Comparatively speaking, understanding which framework suits best can be instrumental in building efficient applications aptly utilizing python dependency injection.
Underlined below is a quick overview comparing two highly favored selections:
- PyInjector: PyInjector revolves around simplicity and ease-of-use. Whether used in large projects or small scripts alike, it ensures convenience by emphasizing only the fundamental aspects of dependency injections such as declaring and providing dependables yet remaining flexible enough not to impose restrictive bindings or configurations on users.
- Injector: On the other hand, Injector comes packed with additional features contributing towards larger size when compared to PyInjector thus making it a potentially more potent pick for bigger projects requiring intricate system design. It supports annotations and auto-injection features mitigating the boilerplate code amount you'd have to write.
Thus, choosing between these two (or any other) relies heavily on the capability of matching a dependency injection framework's feature set against your project's specific needs, size, and complexity. By doing so, it ensures that Python dependency injection is efficiently integrated into your work yielding its desired dividends in modularity, re-useability, and robustness.
Examples and Use Cases
Delving a bit deeper into Python dependency injection, let's explore it in action through both examples and common use cases.
Example Code Demonstrating the Use of Dependency Injection in Python Projects
For a little perspective on what working with Python dependency injection looks like, consider this snippet of code that uses a bare-bones form of dependency injection:
class EmailClient(object): def init(self, mail_service): self._mail_service = mail_service class MailGun(object): # Implementation specific to MailGun pass class SendGrid(object): # Implementation specific to SendGrid pass mailgun_client = EmailClient(MailGun()) sendgrid_client = EmailClient(SendGrid())
In this example, EmailClient doesn't need to know anything about how MailGun or SendGrid works; only that it has access to an object — which we term 'a third service dependency'—capable of sending emails. We "inject" these dependencies (MailGun or SendGrid) when creating instances of our class. This process promotes significant flexibility allowing one to change or modify dependencies without affecting existing implementations.
Use Cases Where Dependency Injection Can Be Particularly Useful
Beyond simple coding exercises, you'll often find practical application for python dependency injection in real-world projects:
- Managing Database Connections: Managing database connections can be quite complex due to considerations such as connections pools or handling failures. A shared connection object injected at runtime via dependency injection simplifies the process dramatically.
- Handling API Dependencies: In modern web development, your program may interface with several APIs. By using Dependency Injection, you’re enabled to swap out actual API calls with mock objects during testing, making your tests faster and more robust.
- App Configuration: It's not uncommon for apps to behave differently depending on their environment (e.g., local development vs. production). Injecting configuration settings as dependencies allows apps to adapt accordingly without needing changes in the code itself.
- Logging: You can inject logger objects into your classes for enhanced flexibility and control over logging behavior.
It's important noting that even though dependency injection significantly adds to software design, not every situation calls for it. The key is knowing when its use will add clarity and maintainability to your code or when simpler techniques are more suitable, a skill you'll develop with experience.
Best Practices for Using Python Dependency Injection
When it comes to software architecture, the way you design your classes and dependencies matters a lot. The practical use of python dependency injection requires certain disciplines for achieving optimal results.
Guidelines for Designing Classes and Modules to Facilitate Dependency Injection
The first step in exploiting the benefits of python dependency injection is creating well-designed classes and modules. Here are few guidelines:
- Single Responsibility Principle: Each class or module should have one reason to change. This rule is easy to overlook, but ensuring each class has only one job makes dependence management considerably easier.
- Code to an Interface, Not an Implementation: Ensure that your classes depend on abstractions rather than concrete classes or instances. Concrete dependencies make code less flexible and more challenging to test.
- Provide Dependencies via Constructors: If possible, pass all dependencies into your objects at creation time (often within constructor methods). This practice allows complete object graphs can be created upfront - leading to cleaner and more predictable code.
By designing with these principles in mind, we'll ensure that our application is ready for effective dependency injection; leading not just towards better code structure but also making debugging a less tedious task.
Moving on from the design part let's talk about managing dependencies themselves because carefully defined explicitly managed dependencies lead directly toward smoother implementation.
Tips For Managing Dependencies And Resolving Conflicts
For python dependency injection handling, the key consideration is recognizing when you're introducing potential conflicts by injecting multiple versions of the same package or using packages with overlapping responsibilities.
Here are few tips on how you can manage these scenarios effectively:
- Define Clear Version Rules: Make sure you specify correct version rules for all external packages used in your project. By doing so, helps avoid unexpected breaks caused by sudden updates or changes in dependent libraries.
- Use A Package Manager: Tools like Pipenv or Poetry facilitate managing complex package dependencies efficiently.
- Isolate Dependencies: Consider using virtual environments to isolate your project dependencies. This practice helps prevent issues caused by system-wide packages or dependencies interfering with your project-specific ones.
- Explicit is better than implicit: Always ensure that it's clear and obvious where each component of your code comes from, avoiding magic tricks for dependency resolution helpers.
By following these guidelines and tips, the pitfall chances when implementing python dependency injection can be significantly reduced. An advance step in our programming journey toward streamlined, modular, test-friendly Python application design!
FAQs
What is Python Dependency Injection (DI)?
DI is a design pattern that improves code quality by decoupling software modules.
How does DI benefit Python development?
It enhances modularity, facilitates testing, and increases code reusability.
What are the key principles DI promotes in Python?
Single Responsibility, Open-closed, Liskov's Substitution, Interface Segregation, and Dependency Inversion principles.
What frameworks support Python DI?
PyInjector, Injector, dependable, and wiring are popular choices.
How does DI improve code modularity?
By separating dependencies, making each part operate independently.
Why is DI advantageous for testing?
It simplifies unit testing by isolating each code component.
Can DI increase a Python project's flexibility?
Yes, it allows easy swapping of components and adjusting to changes.
What are common methods of implementing DI in Python?
Using constructors, setters, or properties, and interface injection.
How does constructor injection work in Python DI?
Dependencies are passed via a class constructor during initialization.
What is interface injection in Python DI?
It injects dependencies in real-time, updating as needed.
How does DI facilitate easier debugging?
By localizing errors to specific, isolated modules.
What is the role of frameworks in Python DI?
They provide built-in functionalities for easy DI implementation.
How does DI promote scalability in Python apps?
Modular design enables easy updating and scaling without rewriting code.
What is the Single Responsibility Principle in DI?
Ensuring each class or module has one reason to change.
How does DI support agile software development?
By enabling quick adaptation to changes and iterative testing.
Can DI be used in small Python projects?
Yes, it's beneficial for projects of any size.
What is a common challenge in implementing DI?
Managing dependencies and resolving conflicts efficiently.
How does DI enhance reusability in Python code?
Independent modules can be reused across different parts of the application.
What best practices should be followed in Python DI?
Designing classes for single responsibility and clear dependency management.
Is DI necessary for all Python projects?
While beneficial, it's not mandatory and should be used as per project needs.
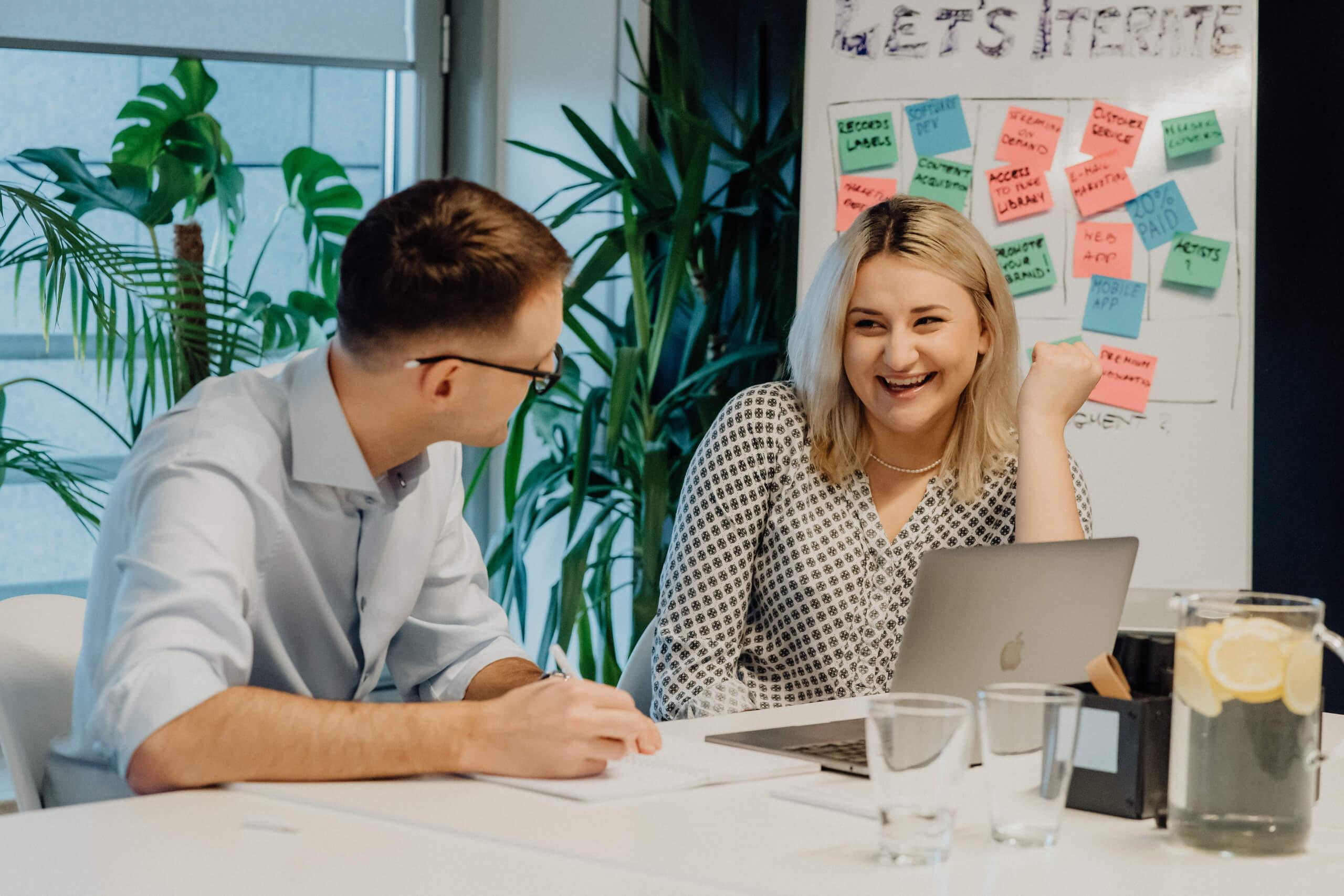
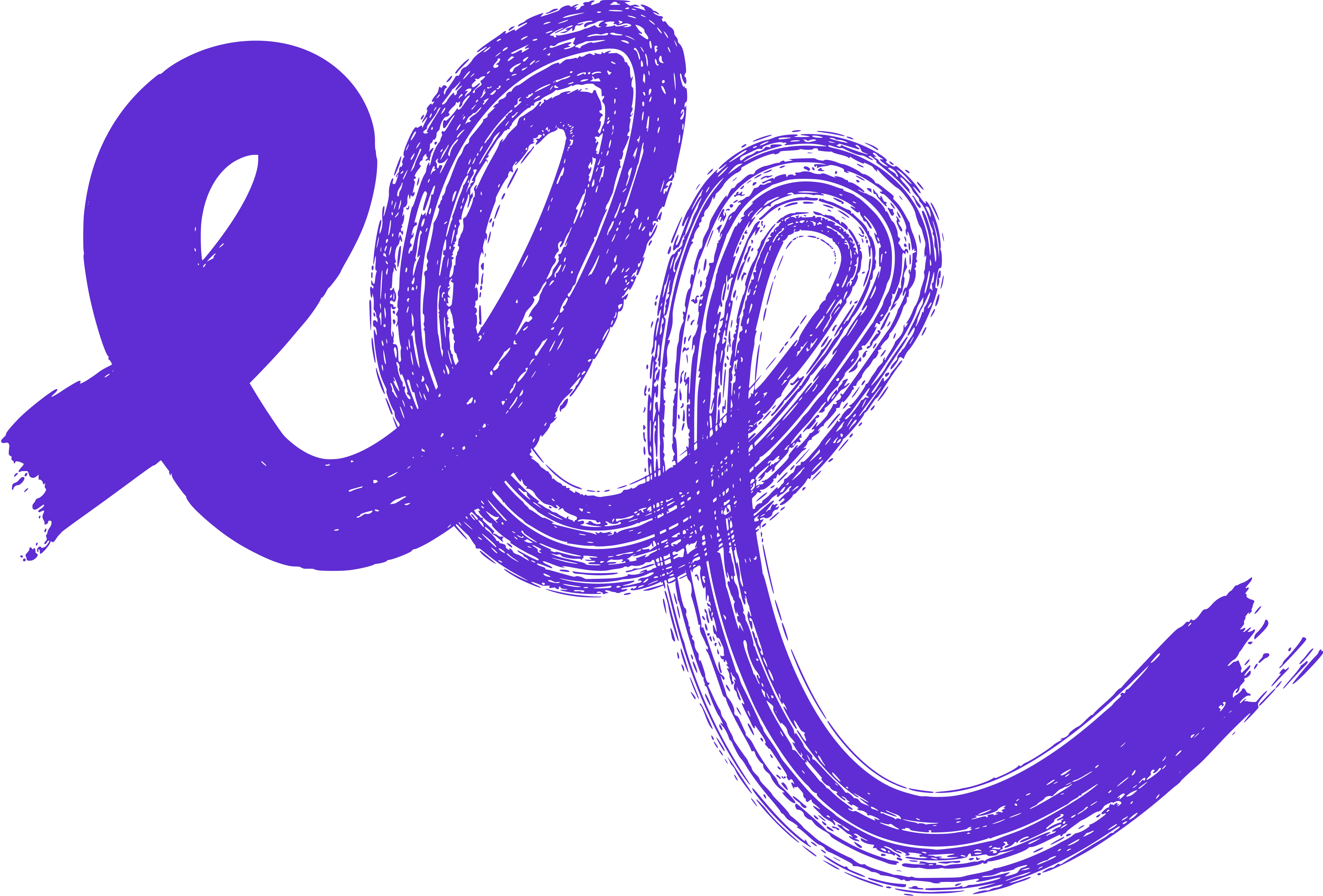
You may also
like...
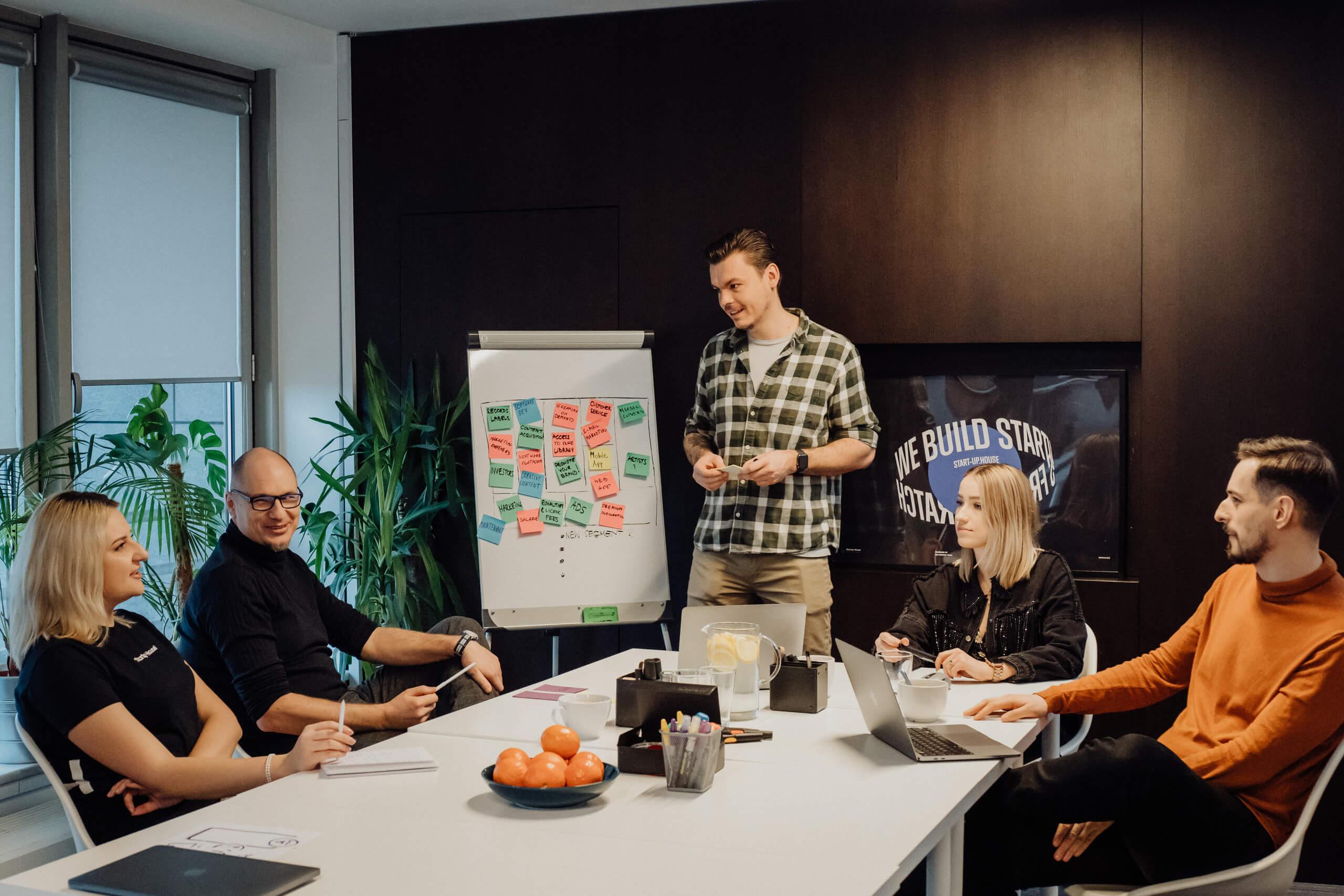
The Innovation Product Life Cycle: Phases and Strategic Insights
Mastering the innovation product life cycle—from ideation to renewal—gives businesses a competitive edge in a fast-evolving market. This guide unpacks each phase and offers strategic insights to help you plan, adapt, and thrive.
Alexander Stasiak
Jun 24, 2025・10 min read
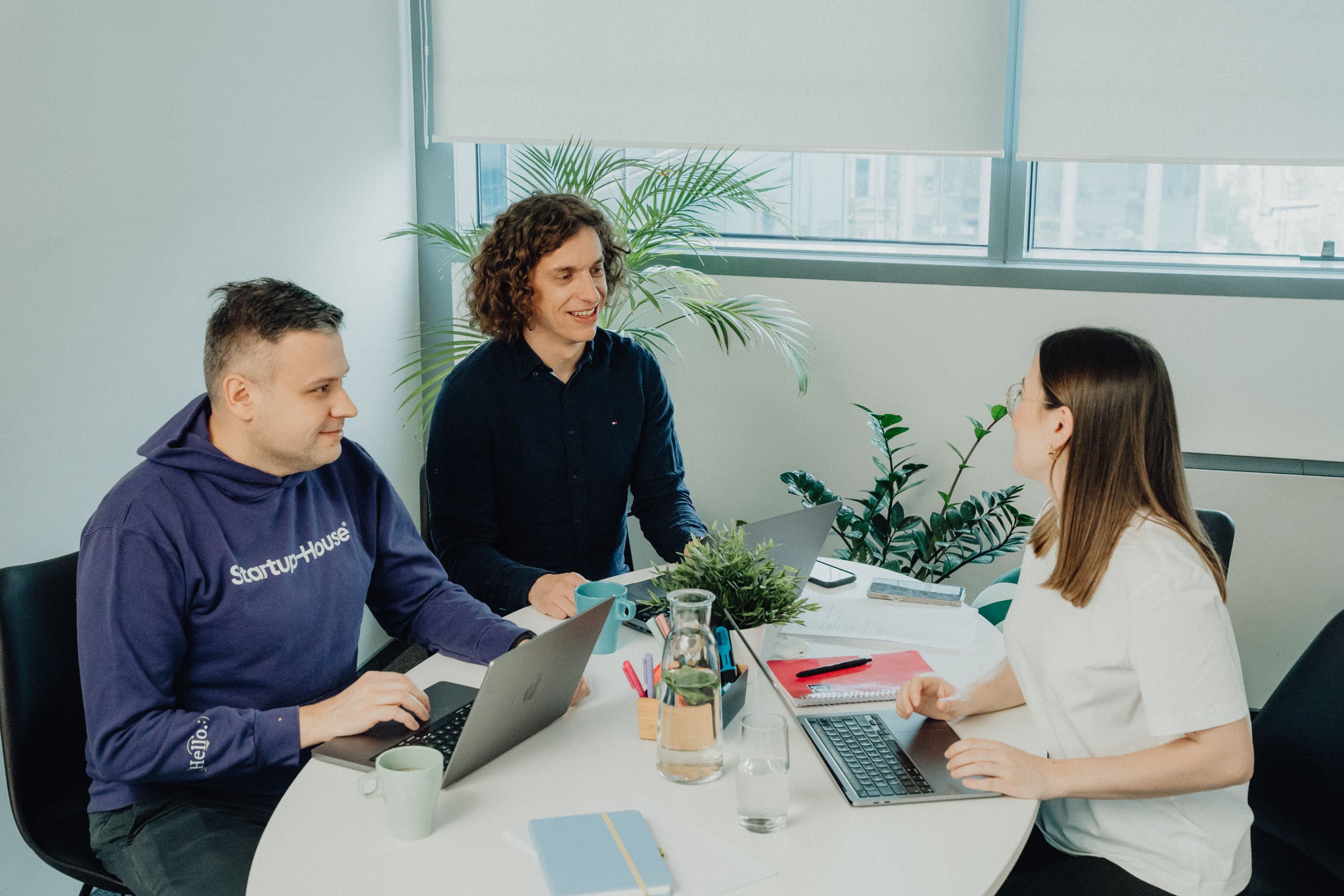
A Practical Guide to Choosing the Right BDD Framework for Your Needs
Choosing the right Behaviour-Driven Development (BDD) framework is key to enhancing collaboration and software quality. This guide explores popular frameworks, selection criteria, and tips for smooth adoption.
Alexander Stasiak
Mar 21, 2024・9 min read
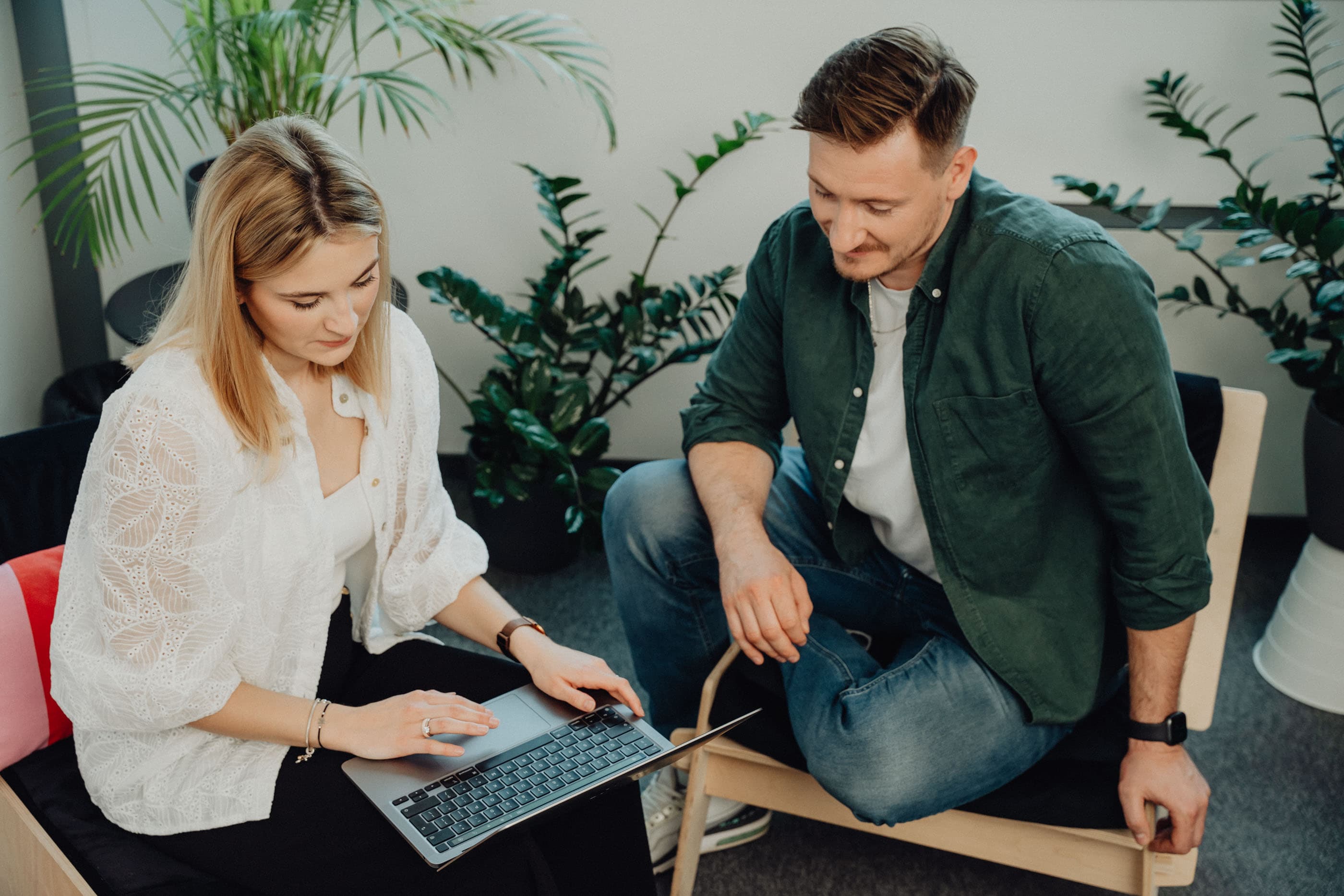
Understanding the Distinct Roles: Scrum Master vs Product Owner
Scrum Master and Product Owner roles are integral to Agile projects but serve different purposes. This guide explains their distinct responsibilities, skills, and collaborative dynamics.
Marek Pałys
Dec 09, 2024・8 min read