🌍 All
About us
Digitalization
News
Startups
Development
Design
Mastering OOP and SOLID Principles
Viktor Kharchenko
Jan 05, 2024・5 min read
Table of Content
Understanding Object-Oriented Programming (OOP)
The Fundamentals of SOLID Principles
Benefits of Using SOLID Principles in Software Development
Common Mistakes to Avoid When Implementing SOLID
Conclusion
FAQs
Welcome to the world of Object-Oriented (OO) Programming and the SOLID principles – two foundational pillars of modern software development. If you're a software enthusiast or a developer looking to enhance your coding skills, you're in the right place.
Object-Oriented Programming, often abbreviated as OOP, is a paradigm that revolutionised the way we design and structure software. It empowers developers to model real-world entities as objects, encapsulating data and behaviour into neat, reusable packages. OOP encourages modularity, making it easier to build and maintain complex applications.
But the true art of crafting software lies not only in writing code but also in writing code that is robust, flexible, and adaptable. This is where the SOLID principles come into play. Developed by Robert C. Martin and embraced by the software community, SOLID is an acronym for five fundamental design principles that guide us towards writing clean, maintainable, and scalable code.
To set the stage, let's briefly introduce these SOLID principles:
- Single Responsibility Principle (SRP): A class should have only one reason to change, meaning it should have a single responsibility.
- Open/Closed Principle (OCP): Software entities (classes, modules, functions) should be open for extension but closed for modification.
- Liskov Substitution Principle (LSP): Subtypes must be substitutable for their base types without altering the correctness of the program.
- Interface Segregation Principle (ISP): Clients should not be forced to depend on interfaces they do not use.
- Dependency Inversion Principle (DIP): High-level modules should not depend on low-level modules. Both should depend on abstractions.
Throughout this article, we'll delve deep into each SOLID principle, providing real-world examples and code snippets to illustrate their importance and practical application. By the end, you'll be armed with the knowledge and tools needed to write more maintainable, flexible, and robust software, driving your projects to success in the world of software development.
Let's embark on this exciting journey into the world of Object-Oriented Programming and SOLID principles.
Understanding Object-Oriented Programming (OOP)
Object-Oriented Programming (OOP) is a programming paradigm that has transformed the way we design and structure software. At its core, OOP is all about modeling the real world within our code by organizing data and behavior into reusable building blocks called objects. To grasp the essence of OOP, let's take a journey through its fundamental concepts and principles.
Objects: The Building Blocks
In the world of OOP, an object represents a self-contained unit that combines data (often referred to as attributes or properties) and behavior (implemented as methods). Think of objects as real-world entities: a car, a person, a bank account, or even an animal. For instance, if you were to model a car, its attributes might include making, model, and color, while its behaviors could be start, stop, and accelerate.
Here's a simple Python example illustrating the concept of objects:
class Car:
def __init__(self, make, model, colour):
self.make = make
self.model = model
self.colour = colour
def start(self):
print(f"{self.colour} {self.make} {self.model} is starting.")
def stop(self):
print(f"{self.colour} {self.make} {self.model} is stopping.")
# Creating car objects
car1 = Car("Toyota", "Camry", "Blue")
car2 = Car("Ford", "Mustang", "Red")
# Invoking object methods
car1.start() # Output: Blue Toyota Camry is starting.
car2.stop() # Output: Red Ford Mustang is stopping.
In this example, the Car class defines the blueprint for car objects. Each car object has its own set of attributes (make, model, color) and can perform actions (start and stop) defined by the class's methods.
Encapsulation: Data and Behavior Bundled
One of the core principles of OOP is encapsulation. It involves bundling an object's data and methods together while controlling access to the internal state. This encapsulation provides data hiding, preventing direct manipulation of object attributes and ensuring that the object's behavior is consistent.
Inheritance: Reusing and Extending
Inheritance allows you to create new classes (child classes) based on existing classes (parent classes). Child classes inherit attributes and methods from their parent class, which promotes code reuse and extension. This hierarchy of classes forms an "is-a" relationship.
class ElectricCar(Car):
def charge(self):
print(f"{self.color} {self.make} {self.model} is charging.")
electric_car = ElectricCar("Tesla", "Model S", "Black")
electric_car.start() # Output: Black Tesla Model S is starting.
electric_car.charge() # Output: Black Tesla Model S is charging.
Here, the ElectricCar class inherits the attributes and methods from the Car class, allowing you to add new behaviors like charge.
Polymorphism: Many Forms
Polymorphism enables objects of different classes to be treated as objects of a common superclass. This allows for flexibility and extensibility in your code. Polymorphism is often achieved through method overriding, where child classes provide their own implementation of a method inherited from a parent class.
class Animal:
def speak(self):
pass
class Dog(Animal):
def speak(self):
return "Woof!"
class Cat(Animal):
def speak(self):
return "Meow!"
# Polymorphic behaviour
def animal_sound(animal):
print(animal.speak())
dog = Dog()
cat = Cat()
animal_sound(dog) # Output: Woof!
animal_sound(cat) # Output: Meow!
In this example, animal_sound can accept any object derived from the Animal class, showcasing polymorphic behavior.
Understanding Object-Oriented Programming is a pivotal step in becoming a proficient software developer. OOP's principles of objects, encapsulation, inheritance, and polymorphism form the foundation of software design, enabling you to create modular, maintainable, and extensible code. As you continue your journey into software development, these concepts will serve as invaluable tools in your programming toolbox.
In the next sections, we'll delve into the SOLID principles, which complement OOP to help you create even more robust and scalable software solutions.
The Fundamentals of SOLID Principles
In the ever-evolving world of software development, maintaining code that is both efficient and adaptable can be a daunting challenge. That's where the SOLID principles come to the rescue. These principles, introduced by Robert C. Martin, offer a set of guidelines that, when followed, lead to more maintainable and scalable code. Let's dive into each of these principles to understand their significance and how they can revolutionise your coding practices.
Single Responsibility Principle (SRP)
The Single Responsibility Principle (SRP) dictates that a class should have only one reason to change. In other words, a class should have a single, well-defined responsibility or function within your codebase. By adhering to SRP, you create code that is easier to understand, modify, and maintain.
Consider an example where you have a ReportGenerator class responsible for both generating reports and sending email notifications:
class ReportGenerator:
def generate_report(self, data):
# Generate the report logic...
def send_email_notification(self, report):
# Send email notification logic…
Here, the ReportGenerator class has multiple responsibilities. If either the report generation or email notification logic needs to change, it could affect the other, violating SRP. A better approach would be to separate these responsibilities into distinct classes, each with a single responsibility.
class ReportGenerator:
def generate_report(self, data):
# Generate the report logic...
class EmailNotifier:
def send_email_notification(self, report):
# Send email notification logic…
Open/Closed Principle (OCP)
The Open/Closed Principle (OCP) encourages code to be open for extension but closed for modification. This means that you should be able to extend the behaviour of a module or class without changing its source code. Achieving this can be accomplished through techniques such as inheritance, interfaces, and design patterns like the Strategy Pattern.
Consider a scenario where you have a Shape class with methods to calculate area for various shapes. Instead of modifying the Shape class every time a new shape is introduced, you can extend it by creating new classes that adhere to the same interface.
class Shape:
def calculate_area(self):
pass
class Rectangle(Shape):
def calculate_area(self):
# Calculate area for a rectangle...
class Circle(Shape):
def calculate_area(self):
# Calculate area for a circle…
By following OCP, you can easily add new shapes without altering the existing Shape class.
Liskov Substitution Principle (LSP)
The Liskov Substitution Principle (LSP) emphasises that objects of a derived class should be substitutable for objects of the base class without affecting the correctness of the program. In simpler terms, if you have a base class, you should be able to use any of its derived classes interchangeably.
class Bird:
def fly(self):
pass
class Sparrow(Bird):
def fly(self):
# Sparrow-specific flying behavior...
class Ostrich(Bird):
def fly(self):
# Ostriches cannot fly, so this method is overridden…
Here, both Sparrow and Ostrich are derived from the Bird base class and can be used interchangeably in contexts where a Bird object is expected. The LSP ensures that this substitution does not lead to unexpected behavior.
Interface Segregation Principle (ISP)
The Interface Segregation Principle (ISP) states that clients should not be forced to depend on interfaces they do not use. In essence, it promotes creating smaller, specific interfaces rather than large, monolithic ones.
Imagine a scenario where you have a Worker interface with a method work, and you want to create two classes, Engineer and Manager. Instead of forcing both classes to implement the same method, you can create separate interfaces tailored to their specific needs.
class Workable:
def work(self):
pass
class Manageable:
def manage(self):
pass
class Engineer(Workable):
def work(self):
# Engineer-specific work behaviour...
class Manager(Manageable):
def manage(self):
# Manager-specific management behaviour…
By adhering to ISP, you ensure that classes only depend on the methods they need, reducing unnecessary coupling.
Dependency Inversion Principle (DIP)
The Dependency Inversion Principle (DIP) emphasises that high-level modules should not depend on low-level modules. Both should depend on abstractions. In other words, it advocates the use of interfaces or abstract classes to decouple high-level and low-level components.
Consider a scenario where a high-level Report class depends on a low-level Database class:
class Report:
def generate_report(self):
data = Database().fetch_data()
# Generate report logic...
class Database:
def fetch_data(self):
# Fetch data from the database…
To adhere to DIP, you can introduce an abstraction (an interface or abstract class) that both Report and Database depend on, reducing the direct dependency.
from abc import ABC, abstractmethod
class DataSource(ABC):
@abstractmethod
def fetch_data(self):
pass
class Report:
def __init__(self, data_source):
self.data_source = data_source
def generate_report(self):
data = self.data_source.fetch_data()
# Generate report logic...
class Database(DataSource):
def fetch_data(self):
# Fetch data from the database…
By following DIP, you create more flexible and maintainable code where changes in one component do not ripple through the entire system.
Understanding the SOLID principles is a crucial step toward writing clean, maintainable, and adaptable code. Each principle serves as a guiding light, helping you design software that is robust and extensible. As you implement these principles in your projects, you'll find that they not only improve code quality but also enhance collaboration among team members. In the next section, we'll explore practical examples of how to apply SOLID principles to real-world software development scenarios.
Benefits of Using SOLID Principles in Software Development
The SOLID principles are more than just guidelines; they are powerful tools that can elevate your software development projects to new heights. By embracing these principles, you unlock a multitude of benefits that enhance code quality, maintainability, and scalability. Let's explore the tangible advantages of adhering to SOLID principles in your software development journey.
1. Improved Code Maintainability
One of the most significant benefits of SOLID principles is improved code maintainability. When you follow these principles, your code becomes modular, with each component having a clear, single responsibility. This makes it easier to understand, update, and extend over time.
Consider a scenario where you need to add new features to an existing system. With SOLID principles in place, you can confidently make changes to specific modules without worrying about unintended consequences in other parts of the codebase.
# Before SOLID principles
class MonolithicClass:
def complex_method(self):
# A long, complex method...
# After SOLID principles
class SeparateResponsibilityClasses:
def method1(self):
# Specific responsibility...
class AnotherClass:
def method2(self):
# Another specific responsibility…
2. Increased Code Reusability
SOLID principles encourage the creation of small, focused classes with well-defined interfaces. This promotes code reusability, as these components can be easily integrated into other parts of your project or shared across different projects.
For example, if you have a well-designed NotificationService class that adheres to SOLID principles, you can effortlessly use it in multiple applications without modification.
class NotificationService:
def send_notification(self, message):
# Notification logic...
# Reused in another project
notification_service = NotificationService()
notification_service.send_notification("Hello, world!")
3. Enhanced Testability
Writing tests for your code becomes more manageable when SOLID principles are applied. The single responsibility principle (SRP) ensures that each class has a clear purpose, making it easier to isolate and test specific functionalities.
With SOLID principles, you can confidently write unit tests for individual classes, ensuring that changes or updates to one class do not inadvertently break other parts of your application.
class PaymentProcessor:
def process_payment(self, amount):
# Payment processing logic...
# Unit test for PaymentProcessor
def test_payment_processor():
payment_processor = PaymentProcessor()
result = payment_processor.process_payment(100)
assert result == "Payment successful"
4. Facilitated Collaboration
SOLID principles promote a clean and organised codebase that is easy for team members to understand and collaborate on. When developers follow consistent design patterns and adhere to these principles, it becomes simpler for multiple team members to work on different parts of the codebase simultaneously.
This collaboration is particularly valuable in larger projects, as it minimises the chances of conflicts and streamlines development efforts.
5. Easier Debugging and Troubleshooting
In a codebase structured around SOLID principles, identifying and fixing issues is more straightforward. When a problem arises, you can quickly isolate the responsible component, thanks to the principle of single responsibility.
Moreover, since the code is less entangled and more modular, debugging becomes less of a headache. You can focus on the specific module where the problem resides, reducing the time and effort required to resolve issues.
The benefits of using SOLID principles in software development extend far beyond mere guidelines. They empower you to write code that is not only efficient but also adaptable to change, reusable across projects, and easier to collaborate on. With SOLID principles as your foundation, you'll find yourself better equipped to tackle complex projects, maintain legacy codebases, and ultimately deliver higher-quality software.
Common Mistakes to Avoid When Implementing SOLID
While the SOLID principles offer invaluable guidance for creating well-structured and maintainable software, developers often encounter common pitfalls when applying them. Being aware of these mistakes can help you navigate the path to SOLID code more effectively. Let's explore some of the typical errors to avoid.
1. Violating the Single Responsibility Principle (SRP)
One of the most common mistakes is creating classes that try to do too much. When a class has multiple responsibilities, it becomes harder to maintain and test. Avoid the temptation to overload a class with unrelated functions.
class ReportGenerator:
def generate_report(self, data):
# Report generation logic...
def send_email_notification(self, report):
# Email notification logic…
To rectify this, split the responsibilities into separate classes adhering to SRP.
2. Over-Abstracting Code
Over-abstraction can lead to excessive complexity. While abstraction is essential, creating numerous interfaces and abstract classes for every possible scenario can make your codebase challenging to understand.
class IEmailService(ABC):
@abstractmethod
def send_email(self, message):
pass
class EmailService(IEmailService):
def send_email(self, message):
# Email service logic…
It's important to strike a balance between abstraction and simplicity, only introducing abstractions when they genuinely enhance the design.
3. Misapplying the Open/Closed Principle (OCP)
Misunderstanding OCP can result in unnecessary abstractions and complexity. Developers may attempt to make every part of the codebase extensible, even when it's not required.
class PaymentProcessor:
def process_payment(self, payment_method, amount):
# Payment processing logic...
def process_refund(self, payment_method, amount):
# Refund processing logic…
Not every class needs to be open for extension. Ensure that you apply OCP judiciously, focusing on areas where future extensions are likely.
4. Neglecting the Liskov Substitution Principle (LSP)
Failing to adhere to LSP can lead to incorrect assumptions about derived classes' behaviour. Always ensure that derived classes are true substitutes for their base classes, without violating the expected contract.
class Bird:
def fly(self):
pass
class Penguin(Bird):
def fly(self):
raise Exception("Penguins can't fly.")
If a base class has a method that derived classes can't implement, reconsider the class hierarchy.
5. Creating Bloated Interfaces
When implementing the Interface Segregation Principle (ISP), avoid creating overly comprehensive interfaces. It's better to have smaller, focused interfaces that match the specific needs of implementing classes.
class IWorker(ABC):
@abstractmethod
def work(self):
pass
@abstractmethod
def manage(self):
pass
Instead, design interfaces tailored to individual roles.
6. Violating the Dependency Inversion Principle (DIP)
One common mistake with DIP is not properly decoupling high-level and low-level modules. Dependency injection can help achieve this decoupling, but if it's done incorrectly, it can lead to unnecessary complexity.
class Report:
def __init__(self):
self.data_source = Database() # Direct instantiation
def generate_report(self):
data = self.data_source.fetch_data()
# Report generation logic…
Instead, use dependency injection to pass the data source to the Report class.
By understanding and avoiding these common mistakes, you can effectively implement the SOLID principles in your projects, leading to code that is more maintainable and adaptable while avoiding unnecessary complexity.
Conclusion
In the world of software development, mastering Object-Oriented Programming (OOP) and embracing the SOLID principles is akin to constructing a solid foundation for a grand architectural masterpiece. These principles are not just theoretical concepts; they are the building blocks that enable you to craft software solutions that stand the test of time.
As you've journeyed through this article, you've explored the essence of OOP, where real-world entities become objects with data and behaviours, encapsulating complexity in elegant packages. You've delved into the SOLID principles, each with its own unique role in shaping code that is maintainable, flexible, and scalable.
Yet, it's important to remember that SOLID principles are not rigid rules but rather guiding principles. They provide a framework for sound decision-making in the ever-evolving landscape of software development.
As you embark on your coding journey, keep in mind that the pursuit of excellence is an ongoing endeavour. Strive to apply these principles with intention, adapt them to your project's unique needs, and always seek opportunities for improvement.
With OOP and SOLID principles as your companions, you possess the tools to create software that not only meets the demands of today but also adapts gracefully to the challenges of tomorrow. As you continue to refine your craft, you'll find that these principles are your allies in the pursuit of software excellence, ensuring that your code stands as a testament to your skill and dedication.
Happy coding!
FAQs
1. What is Object-Oriented Programming (OOP)?
Object-Oriented Programming (OOP) is a programming paradigm where software is organized around objects, which are instances of classes that encapsulate data and behavior.
2. What are the core principles of SOLID?
SOLID stands for Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion principles, which guide software design and development.
3. Why is OOP important in software development?
OOP promotes code reusability, modularity, and easier maintenance, making it a fundamental concept in modern software development.
4. How does the Single Responsibility Principle (SRP) improve code?
SRP ensures that each class has a single responsibility, making code more focused, maintainable, and less prone to bugs.
5. Can you provide an example of the Open/Closed Principle (OCP)?
OCP encourages extending code without modifying existing classes. For instance, you can add new payment methods to a system without changing the payment processing class.
6. What is the Liskov Substitution Principle (LSP) and why is it essential?
LSP ensures that derived classes can be used interchangeably with their base class, maintaining expected behavior throughout the program.
7. How does Interface Segregation Principle (ISP) benefit large projects?
ISP encourages smaller, specialized interfaces, reducing unnecessary dependencies and making code more maintainable in extensive projects.
8. Why is Dependency Inversion Principle (DIP) crucial for decoupling code?
DIP decouples high-level modules from low-level modules, promoting flexibility and making code more resistant to changes.
9. What are the practical advantages of applying SOLID principles?
Applying SOLID principles improves code maintainability, reusability, testability, collaboration, and debugging, leading to higher-quality software.
10. Can SOLID principles be adapted to different programming languages?
Yes, SOLID principles are language-agnostic and can be applied in various programming languages.
11. How can I start implementing SOLID principles in my projects?
Start by understanding each principle, refactoring existing code, and gradually applying them in new development tasks.
12. Are there any tools or frameworks that facilitate SOLID implementation?
While there are no specific tools, code analysis and review tools can help identify violations and areas for improvement.
13. What challenges do developers face when applying SOLID principles?
Common challenges include over-abstraction, misunderstanding of principles, and resistance to change in existing codebases.
14. Are SOLID principles suitable for both small and large projects?
Yes, SOLID principles are beneficial for projects of all sizes, as they enhance code quality and maintainability, irrespective of project scale.
15. What are some real-world examples of companies benefiting from SOLID principles?
Companies like Netflix, Amazon, and Adobe have leveraged SOLID principles to enhance software quality and agility.
16. Can SOLID principles be applied in web development?
Yes, SOLID principles can be applied in web development for creating more maintainable and scalable web applications.
17. How can SOLID principles contribute to agile software development?
SOLID principles support agile development by making code more adaptable to changing requirements and reducing technical debt.
18. What are some recommended resources for learning more about SOLID principles?
Books like "Clean Code" by Robert C. Martin and online tutorials and courses offer valuable insights into SOLID principles.
19. Can you provide examples of open-source projects that follow SOLID principles?
Projects like Django, Ruby on Rails, and Spring Framework are known for adhering to SOLID principles.
20. How can I assess the SOLID compliance of my codebase?
Code review, static analysis tools, and automated testing can help evaluate SOLID compliance and identify areas for improvement.

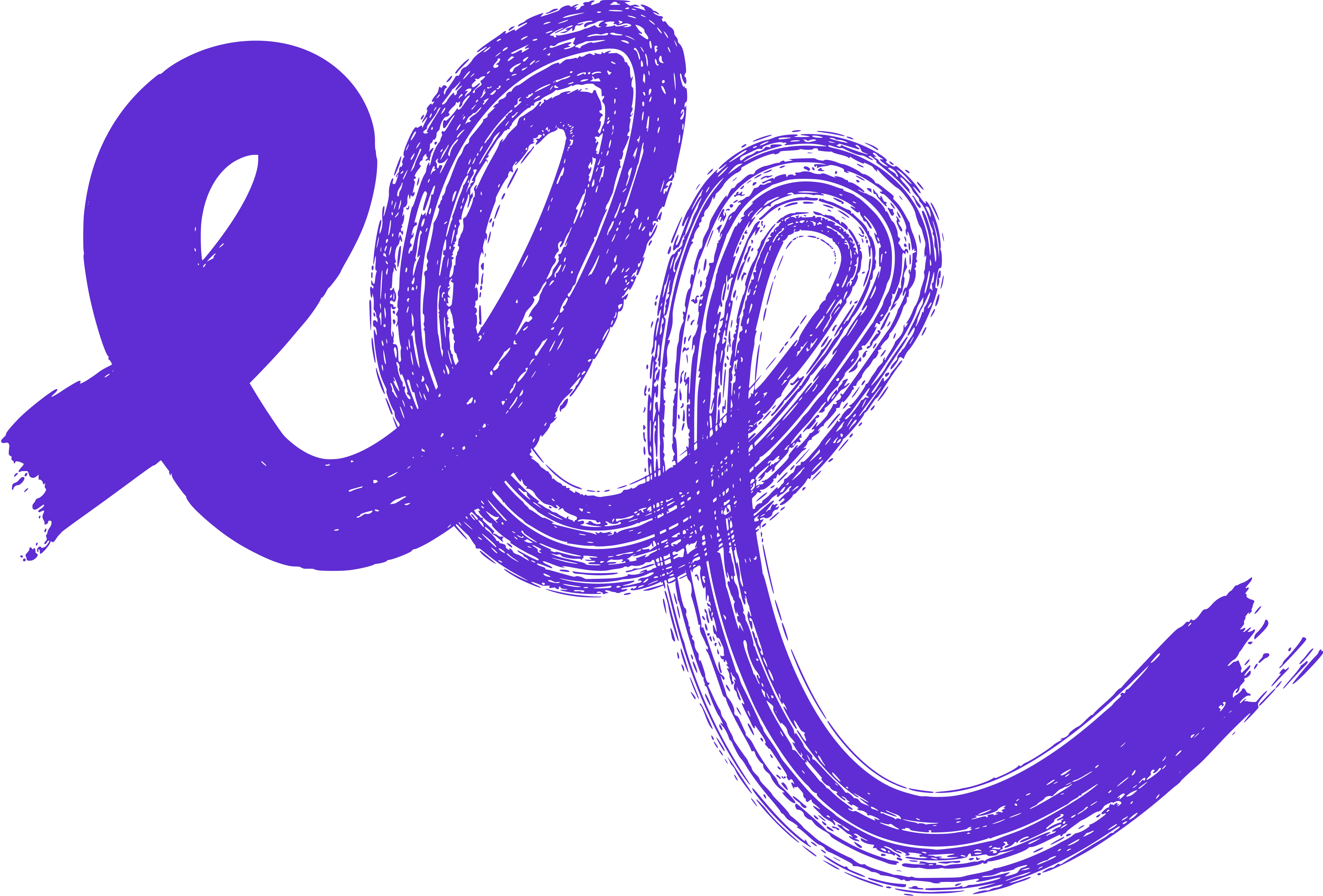
You may also
like...
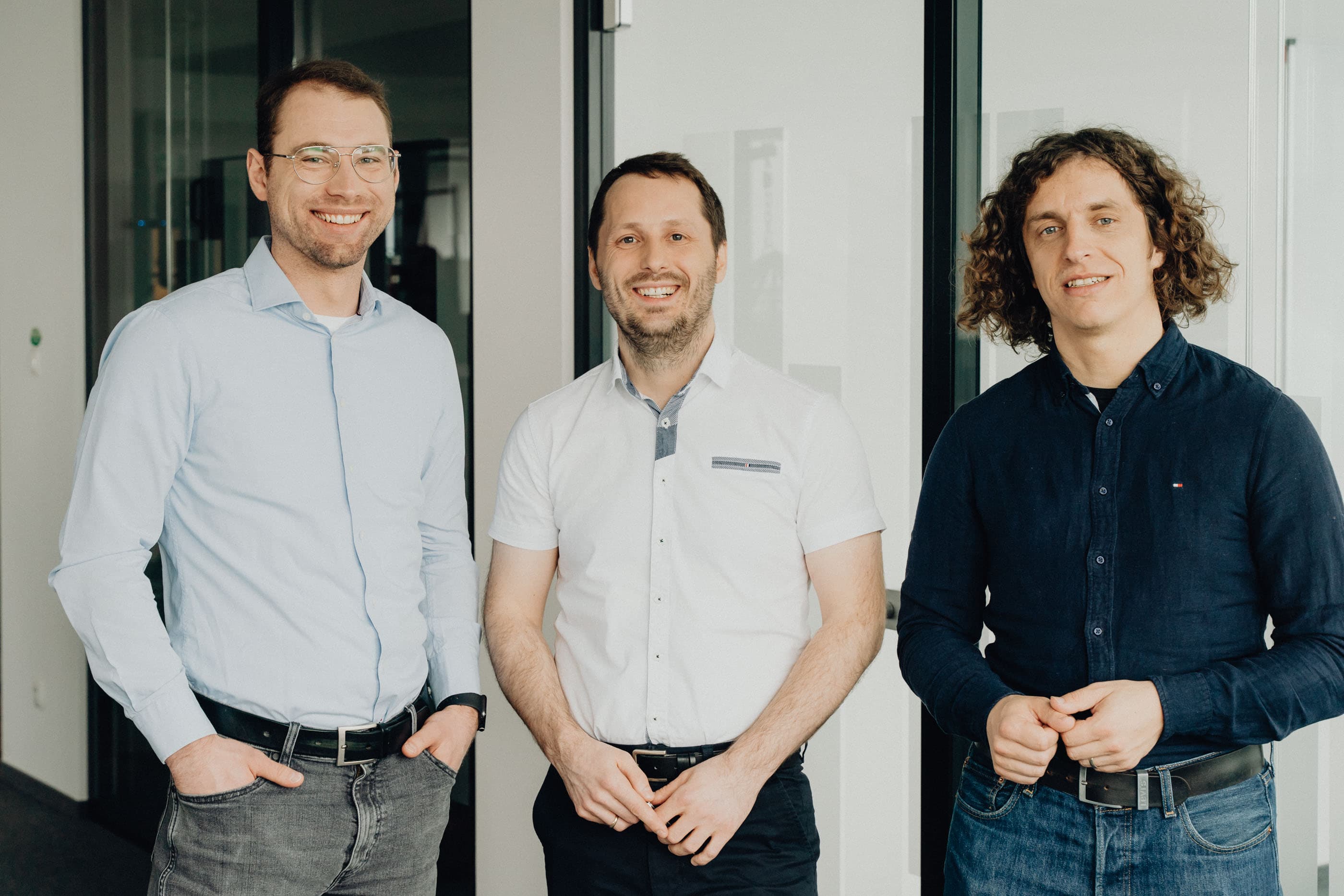
Python vs Scala: Choosing the Right Language for Your Project
Python and Scala are powerful programming languages with distinct strengths. Python excels in data science and machine learning with its simplicity, while Scala shines in big data and high-performance systems, leveraging its static typing and JVM integration.
Alexander Stasiak
Apr 17, 2024・5 min read
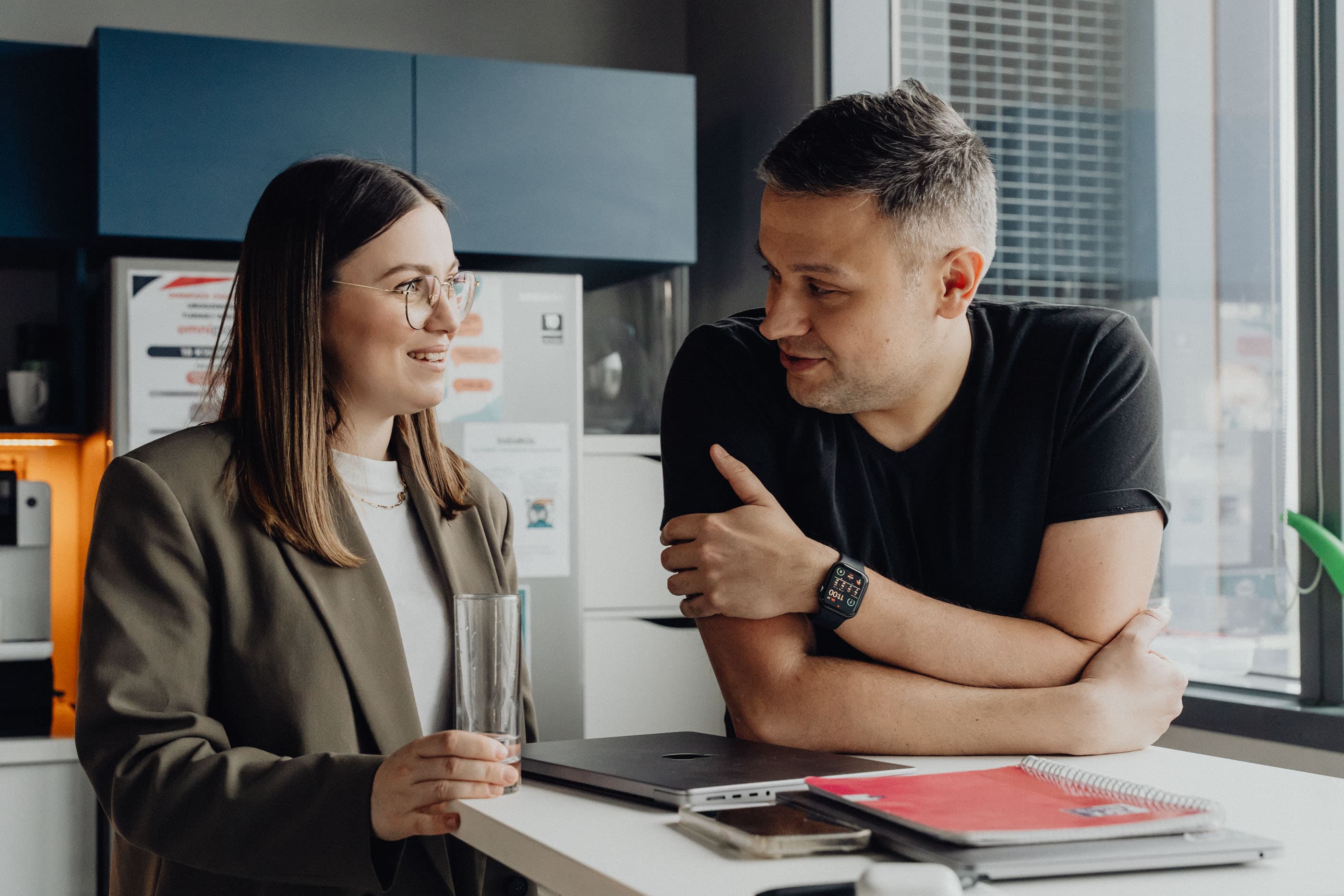
Top Python IDEs: Best Tools for Python Developers
Choosing the right Python IDE can enhance coding efficiency, debugging, and project management. This article reviews the top Python IDEs, including PyCharm, Visual Studio Code, and Jupyter Notebook, highlighting their key features and benefits for Python development.
Alexander Stasiak
Jul 09, 2024・5 min read
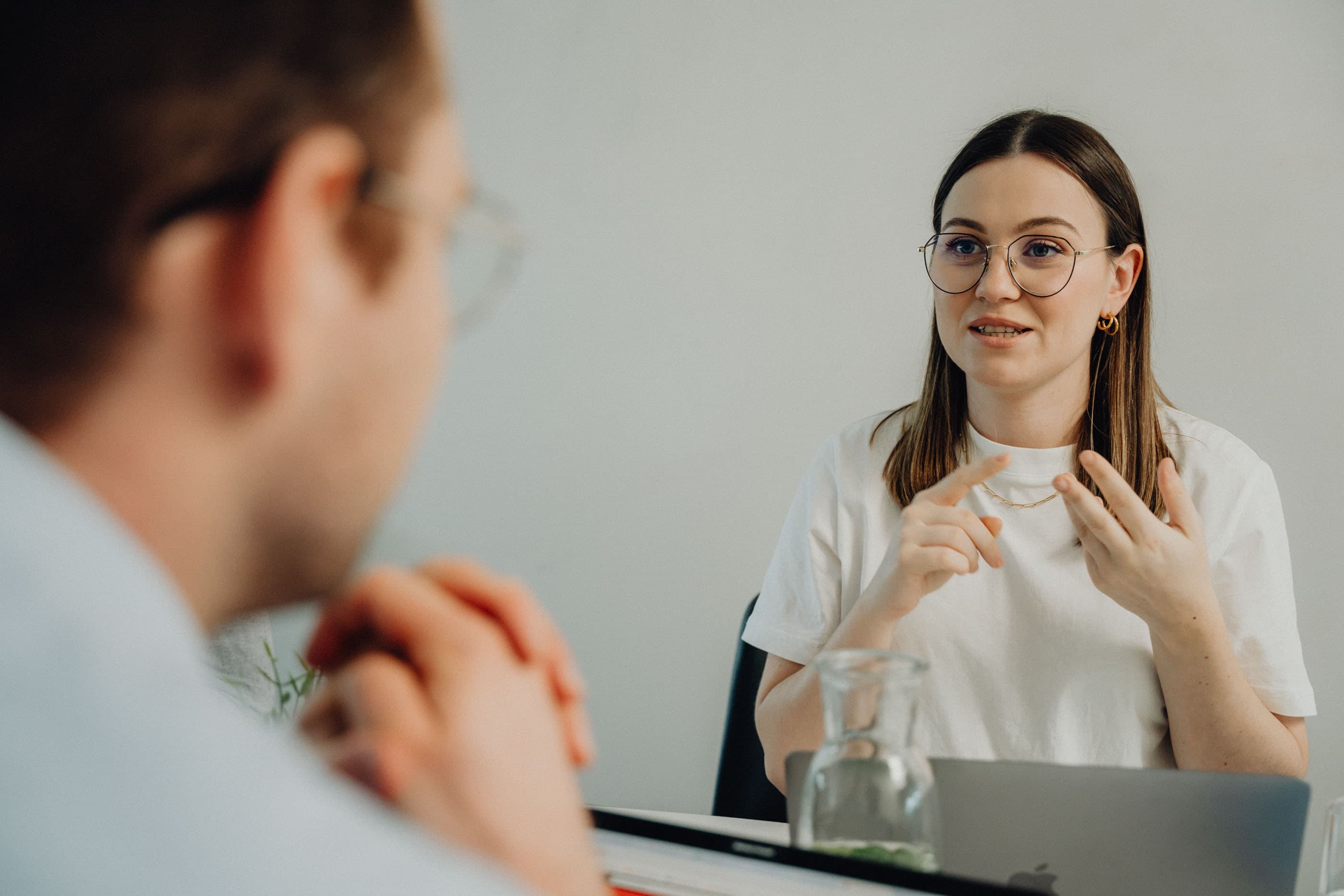
Python vs. C#: Comparing Two Powerful Programming Languages
Python and C# are versatile, powerful programming languages with unique strengths. Python excels in data science and rapid development due to its dynamic typing, while C# offers consistent syntax and robust support for game and enterprise development. This article explores their key differences and use cases.
Alexander Stasiak
May 01, 2024・4 min read