🌍 All
About us
Digitalization
News
Startups
Development
Design
Node.js Event Loop and Asynchronous Programming Tools
Viktor Kharchenko
Nov 23, 2023・5 min read
Table of Content
Understanding the Power of Node.js
Event Loop Demystified
Asynchronous Programming in Node.js
Conclusion
FAQ
Node.js is an open-source, cross-platform JavaScript runtime built on the Chrome V8 JavaScript engine. It allows developers to run JavaScript code on the server side, enabling them to build scalable, high-performance applications. Unlike traditional server-side languages like Python or Ruby, Node.js leverages non-blocking, event-driven architecture, making it particularly suitable for handling I/O-intensive tasks.
Node.js has gained immense popularity in recent years as a runtime environment for server-side JavaScript applications. To appreciate its power, it's essential to grasp the fundamental concepts that underlie Node.js and why it's a preferred choice for efficient backend development.
Understanding the Power of Node.js
Event-Driven Architecture
At the core of Node.js lies its event-driven, asynchronous nature. This means that Node.js can handle multiple concurrent operations without blocking the execution of other tasks. When an asynchronous operation is initiated, Node.js registers a callback function to be executed once the operation is completed. This non-blocking approach enables Node.js to efficiently manage numerous I/O-bound tasks, making it well-suited for applications like real-time chat, streaming, and APIs.
const fs = require('fs');
// Asynchronous file reading
fs.readFile('example.txt', 'utf-8', (error, data) => {
if (error) {
throw error;
}
console.log(data);
});
console.log('Reading file...');
In the code snippet above, the fs.readFile function initiates an asynchronous file reading operation. While Node.js reads the file, it doesn't block the execution of the console.log('Reading file...') statement, demonstrating its non-blocking nature. We will take a closer look at the asynchronous nature of Node.js in the next section.
Single-Threaded Event Loop
Node.js operates on a single-threaded event loop, allowing it to efficiently manage thousands of concurrent connections. The event loop constantly checks for events, such as I/O operations or timers, and executes their associated callback functions when events occur. This architecture minimises the overhead of thread management and context switching, making Node.js highly performant.
Use Cases
Node.js shines in scenarios that demand real-time communication and high concurrency, however it is far from the best choice when it comes to CPU intensive tasks. It's an excellent choice for building web applications, RESTful APIs, microservices, and IoT applications. Popular frameworks like Express.js simplify web development with Node.js by providing a robust set of tools and middleware.
Node.js's power lies in its event-driven, asynchronous architecture and single-threaded event loop. It's an ideal choice for developers looking to build scalable and efficient backend applications, especially those that require handling a large number of concurrent connections and I/O operations. As we delve deeper into advanced techniques in this article, you'll discover how to harness the full potential of Node.js for your backend development needs.
Event Loop Demystified
The event loop is a fundamental concept in Node.js, and understanding how it works is key to harnessing the full power of asynchronous programming. In this section, we'll demystify the event loop, explore its inner workings, and see how it enables Node.js to handle concurrent tasks efficiently.
What is the Event Loop?
At its core, the event loop is a mechanism that allows Node.js to perform non-blocking I/O operations and handle multiple tasks concurrently within a single-threaded environment. It's the secret sauce that makes Node.js highly performant.
Imagine the event loop as the conductor of an orchestra. It manages the execution of tasks (events) in a way that ensures everything plays in harmony, without one task blocking the entire process. This orchestration is what gives Node.js its ability to handle thousands of concurrent connections and I/O-bound operations efficiently.
Event Loop Phases
The event loop consists of several phases, each responsible for handling different types of events. Understanding these phases can help you make better decisions when writing asynchronous code.
- Timers Phase: This phase handles callbacks scheduled by setTimeout() and setInterval(). It checks if any timers are ready to execute and runs their callback functions.
- I/O Callbacks Phase: In this phase, callbacks for I/O operations that have completed are executed. For example, when a file read or a network request finishes, the associated callback is processed here.
- Idle, Prepare Phases: These phases are typically not used in most applications. They are used for internal housekeeping by the event loop.
- Poll Phase: The poll phase is where most of the action happens. It checks for I/O events, such as data arriving on a socket or a user interaction with the application. If any callbacks are waiting to be executed, they are processed in this phase.
- Check Phase: The check phase allows you to execute callbacks scheduled via setImmediate(). It runs these callbacks immediately after the poll phase, thus the name.
- Close Callbacks Phase: In this final phase, any callbacks registered with close events (e.g., when a server or a socket is closed) are executed.
When the event loop runs, it goes through each of these phases one by one - in a loop. Each cycle is called a tick and if we have something to be done right before the next tick executes, right before anything else besides synchronous code, we can do so with process.nextTick(). Whatever passed inside will be executed right at the beginning of the next tick, just as the name suggests.
The Event Loop in Action
To get a sense of how the event loop operates, consider the following example:
console.log('Start');
setTimeout(() => {
console.log('Timeout callback');
}, 0);
setImmediate(() => {
console.log('Immediate callback');
});
process.nextTick(() => {
console.log('Running at next tick');
});
console.log('End');
Output
Start
End
Running at next tick
Immediate callback
Timeout callback
In this code, the event loop goes through the following steps:
The console.log('Start') and console.log('End') statements are executed immediately.
Right after synchronous code, the event loop fires up whatever we passed inside process.nextTick().
The setTimeout() callback is scheduled to run after a minimal delay (0 milliseconds). It goes into the timer phase.
The setImmediate() callback is scheduled to run in the check phase.
The event loop first enters the poll phase, where it waits for events.
Once the poll phase is complete and there are no I/O events to handle, it enters the check phase and executes the setImmediate() callback.
Finally, the event loop returns to the timer phase and executes the setTimeout() callback.
So the output of the above code snippet would be as follows.
Understanding the event loop is crucial for writing efficient Node.js applications. It enables you to make informed decisions about when to use timers, setImmediate(), and other asynchronous constructs. As you continue your journey into advanced Node.js techniques, keep in mind that the event loop is the conductor that orchestrates the magic of asynchronous programming.
Asynchronous Programming in Node.js
Asynchronous programming is at the heart of Node.js, enabling it to handle multiple tasks simultaneously without blocking the execution of others. In this section, we'll delve into the concept of asynchronous programming, why it's crucial in Node.js, and how to work effectively with asynchronous operations.
Understanding Asynchronous Operations
In traditional synchronous programming, tasks are executed one after the other in a sequential manner. This approach can lead to bottlenecks when dealing with I/O-bound operations like file reading, network requests, or database queries. Asynchronous programming, on the other hand, allows Node.js to initiate multiple tasks and continue executing code without waiting for their completion.
Imagine you have to fetch data from a remote server, perform some calculations, and then send a response to a client. With asynchronous programming, you can initiate the data retrieval, work on other tasks, and handle the data when it becomes available, ensuring that your application remains responsive.
Callbacks: The Foundation of Asynchrony
In Node.js, callbacks are a common mechanism for handling asynchronous operations. A callback is a function passed as an argument to another function and is executed when a specific event or operation is complete. It allows you to define what should happen once an asynchronous task finishes.
Here's an example of using a callback for reading a file asynchronously from a previous section:
const fs = require('fs');
fs.readFile('example.txt', 'utf-8', (error, data) => {
if (error) {
throw error;
}
console.log(data);
});
console.log('Reading file...');
In this code, the readFile function initiates an asynchronous file reading operation, and the provided callback function is executed when the operation is finished. Meanwhile, the console.log('Reading file...') statement is executed immediately, showcasing Node.js's non-blocking behaviour. And we already know that the reason behind this behaviour is the orchestrator - the Event Loop.
Callback Hell and the Need for Better Solutions
While callbacks are essential, they can lead to a problem known as "callback hell" or "pyramid of doom" when dealing with deeply nested asynchronous operations. Code readability and maintainability can suffer. To address this issue, developers have adopted techniques like Promises and async/await.
Promises
Promises provide a more structured way to work with asynchronous operations. They represent a future value or error and allow you to attach callbacks for success and failure. Here's the same file reading example using Promises:
const fs = require('fs').promises;
fs.readFile('example.txt', 'utf-8')
.then((data) => {
console.log(data);
})
.catch((error) => {
console.error(error);
});
console.log('Reading file...');
Promises make the code more readable by chaining .then() and .catch() methods for handling success and error cases, respectively.
async/await
Async/await is a modern JavaScript feature that simplifies asynchronous code even further. It allows you to write asynchronous code in a more synchronous style, improving readability. Here's the same file reading example using async/await:
const fs = require('fs').promises;
async function readFileAsync() {
try {
const data = await fs.readFile('example.txt', 'utf-8');
console.log(data);
} catch (error) {
console.error(error);
}
}
readFileAsync();
console.log('Reading file...');
Async/await makes asynchronous code look similar to synchronous code, making it easier to understand and maintain.
Asynchronous programming is a cornerstone of Node.js, enabling efficient handling of I/O-bound tasks and concurrent operations. Understanding callbacks, Promises, and async/await is crucial for writing clean and maintainable Node.js applications. In the next sections, we'll explore advanced techniques that leverage asynchronous programming to build efficient backend systems.
Conclusion
In this comprehensive article, we've explored the fundamental concepts of Node.js, including its event-driven architecture and single-threaded event loop. We've also demystified the event loop's inner workings, discussed asynchronous programming with callbacks, Promises, and async/await. Armed with this knowledge, you're better equipped to harness the full power of Node.js for efficient backend development.
FAQ
What is Node.js and why is it popular?
Node.js is an open-source, cross-platform JavaScript runtime known for its event-driven, asynchronous architecture. It's popular for building scalable, high-performance server-side applications.
How does Node.js handle asynchronous operations?
Node.js uses callback functions, Promises, and async/await to handle asynchronous operations, ensuring that it remains non-blocking and responsive.
What is the role of the event loop in Node.js?
The event loop is the core mechanism in Node.js that manages asynchronous operations, allowing it to handle multiple tasks concurrently within a single-threaded environment.
Why is understanding the event loop crucial for Node.js developers?
Understanding the event loop helps developers write efficient code and make informed decisions about when to use asynchronous constructs like timers and setImmediate().
What are the different phases of the Node.js event loop?
The event loop consists of phases such as Timers, I/O Callbacks, Poll, Check, and Close Callbacks, each responsible for handling specific types of events.
How can I profile my Node.js application to identify performance bottlenecks?
You can use tools like the --inspect flag and Chrome Developer Tools for profiling your code and identifying performance issues.
What are some tips for optimizing JavaScript code in Node.js?
To optimize JavaScript code, avoid blocking the event loop, minimize I/O operations, optimize loops, and consider using object pooling.
What are some common challenges developers face when working with asynchronous code in Node.js?
Developers often face challenges like callback hell, managing complex error handling in asynchronous operations, and coordinating the execution of multiple asynchronous tasks. These challenges can be mitigated with techniques like Promises, async/await, and proper error handling practices.
Is Node.js suitable for building real-time multiplayer games?
Node.js can be used to build real-time multiplayer games thanks to its event-driven architecture and non-blocking I/O. However, the suitability depends on the complexity of the game and the need for real-time synchronization. For highly demanding and performance-critical games, specialized game engines may be more appropriate, but Node.js can be used effectively for simpler real-time games and game-related services.
Why is Node.js not the best choice for CPU-intensive tasks?
Node.js's single-threaded nature and event-driven architecture make it less suitable for CPU-bound tasks that require extensive computation.
What is callback hell, and how can it be mitigated?
Callback hell, or pyramid of doom, occurs when dealing with deeply nested callbacks. It can be mitigated by using Promises or async/await for cleaner and more readable code.
What are Promises in Node.js?
Promises are a structured way to handle asynchronous operations in Node.js, representing a future value or error and allowing you to chain callbacks for success and failure.
How does async/await simplify asynchronous code in Node.js?
Async/await is a modern JavaScript feature that allows you to write asynchronous code in a more synchronous style, improving code readability and maintainability.
What is the difference between setImmediate() and setTimeout() in Node.js?
Both setImmediate() and setTimeout() schedule callbacks, but setImmediate() executes its callbacks immediately after the current phase of the event loop, while setTimeout() schedules its callbacks for a specific delay.
Can you explain the concept of process.nextTick() in Node.js?
process.nextTick() allows you to schedule a callback to run immediately after the current operation but before the next tick of the event loop, making it suitable for tasks that should be prioritized.
What are some common use cases for Node.js?
Node.js is well-suited for building web applications, RESTful APIs, microservices, IoT applications, and real-time communication systems like chat applications.
How can I ensure that my Node.js application remains responsive and scalable?
To ensure responsiveness and scalability, avoid blocking the event loop, use asynchronous programming, and optimize code performance.
What are some best practices for managing I/O operations in Node.js?
Best practices for managing I/O operations include using streams for efficient data handling, caching data when possible, and delegating heavy work to worker threads or child processes.
Can I use Node.js for CPU-bound tasks if I optimize my code?
While you can optimize Node.js code, it may not be the best choice for CPU-bound tasks due to its single-threaded nature. Consider other languages or tools designed for CPU-intensive tasks.
How often is the V8 engine updated, and why should Node.js developers stay informed about its features?
The V8 engine is continuously updated to improve performance and add new features. Node.js developers should stay informed about these updates to benefit from the latest optimizations and enhancements.
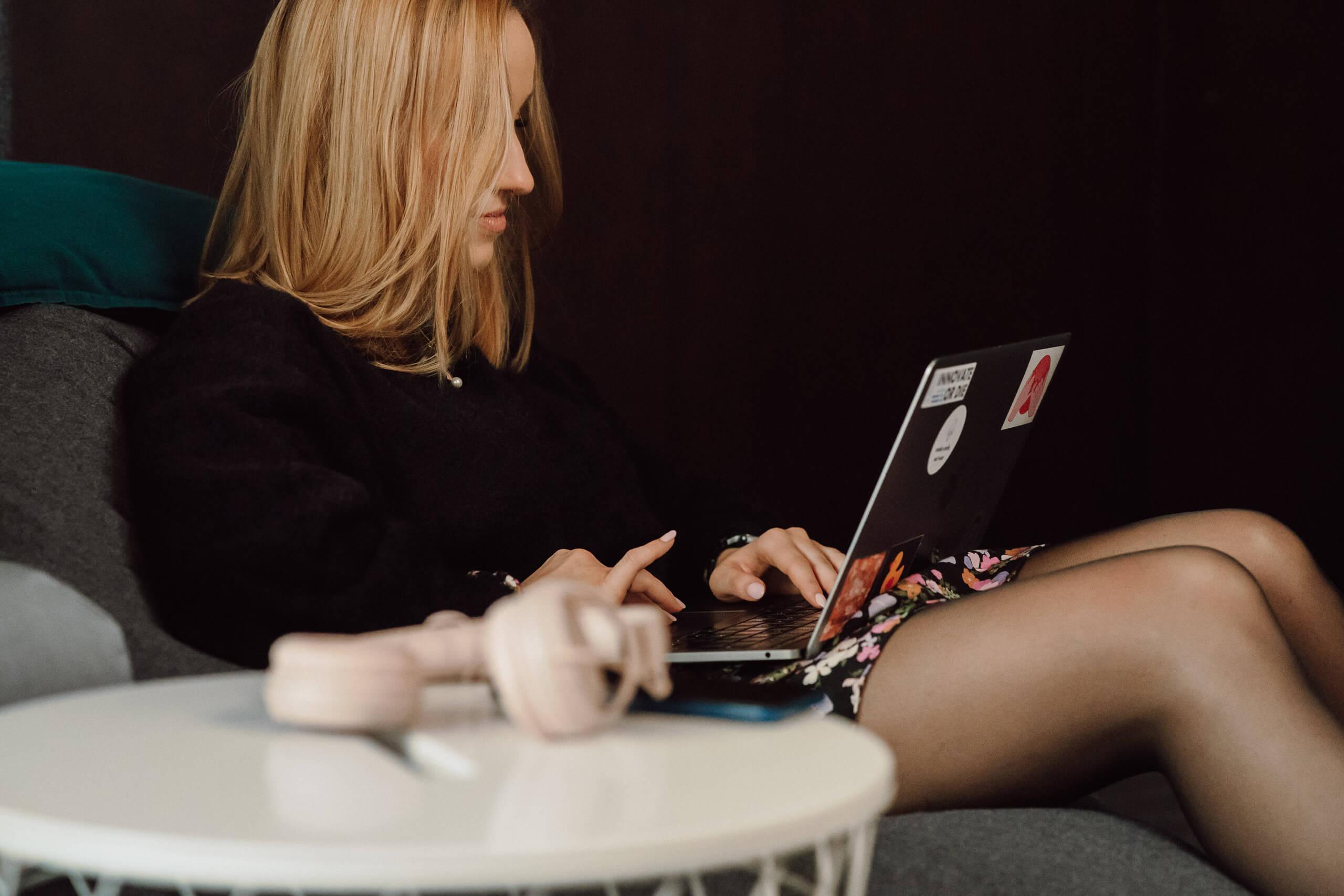
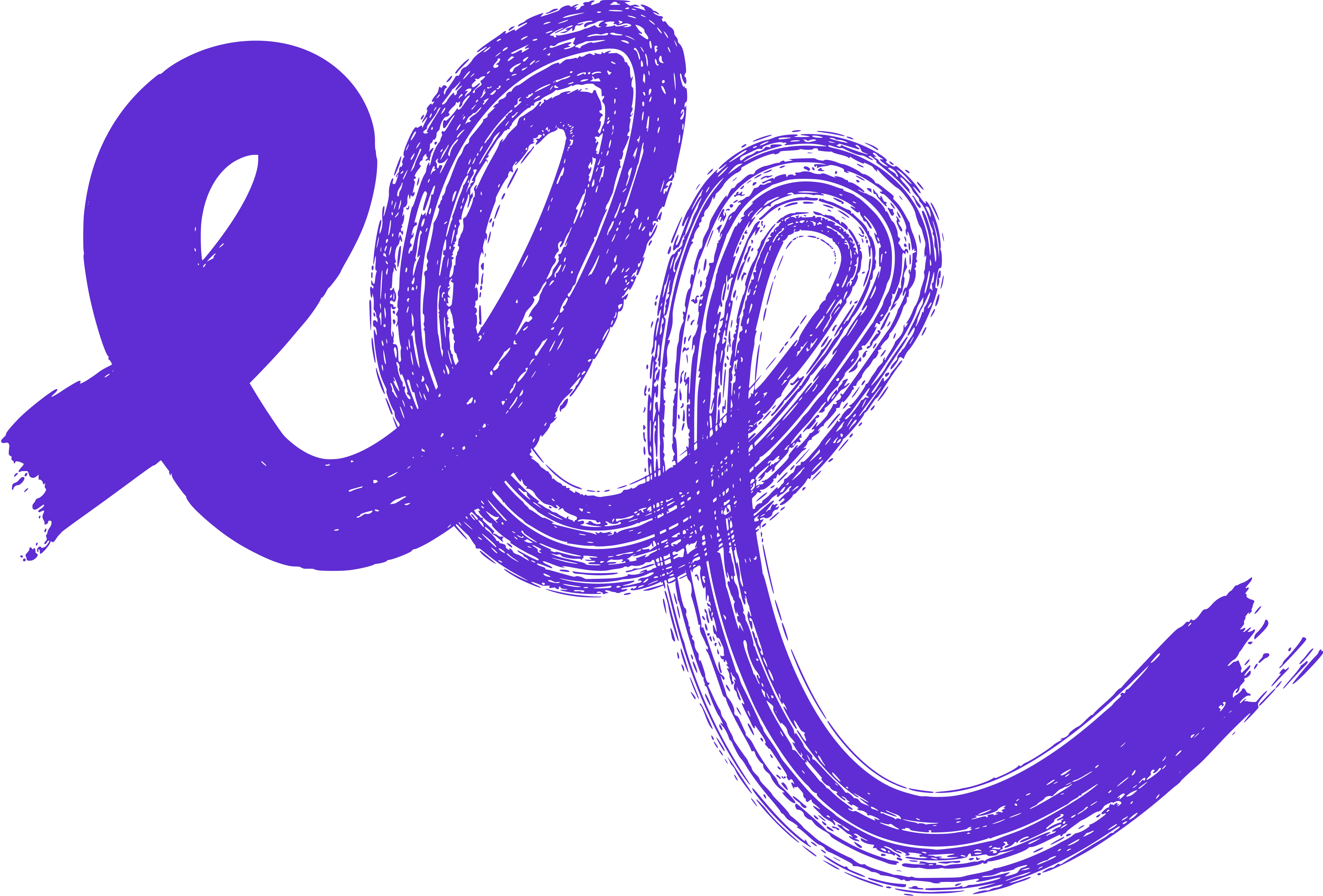
You may also
like...
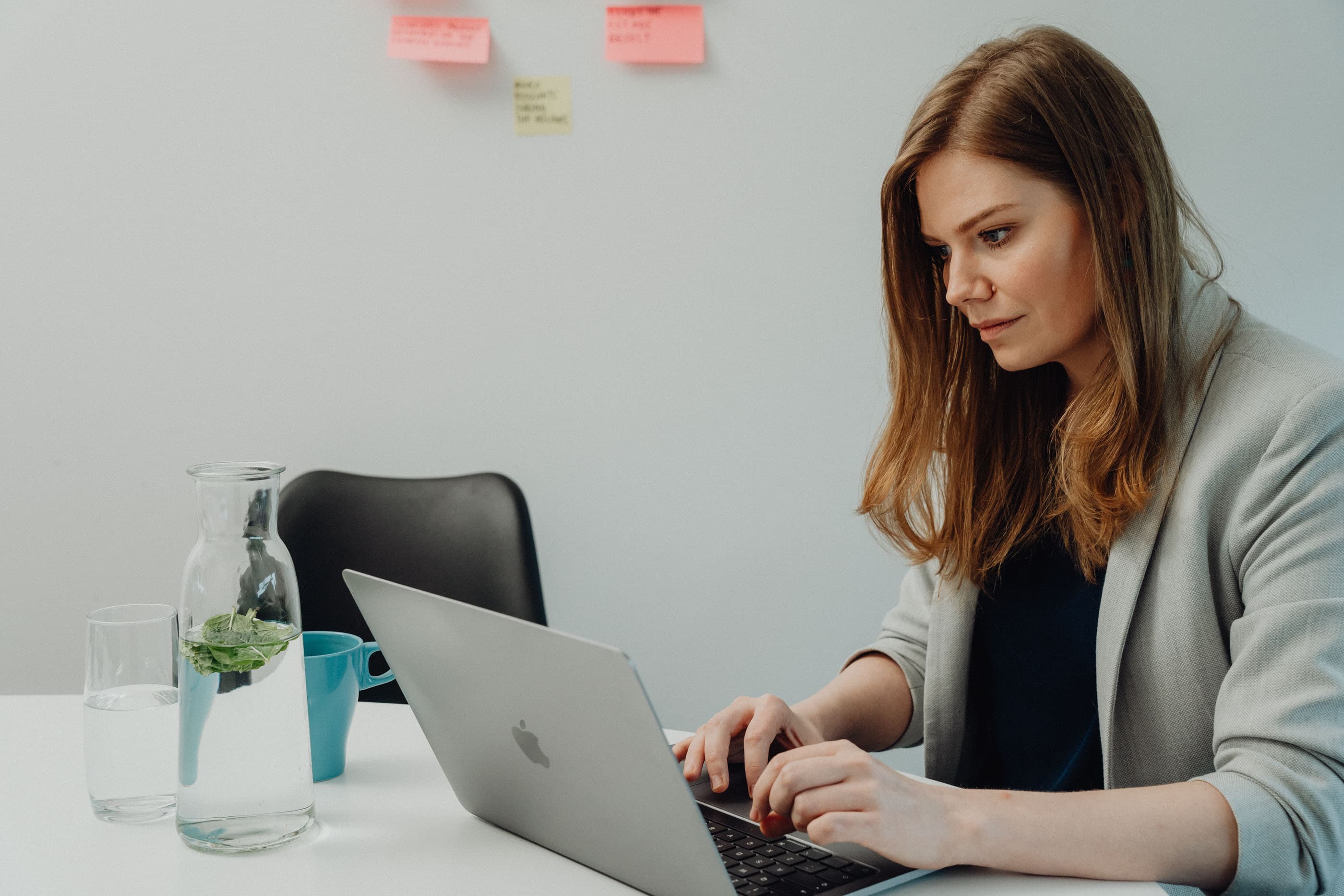
Understanding Event-Driven Programming: A Simple Guide for Everyone
Explore the essentials of event-driven programming. Learn how this responsive paradigm powers interactive applications with real-world examples and key concepts.
Marek Pałys
Apr 30, 2024・9 min read
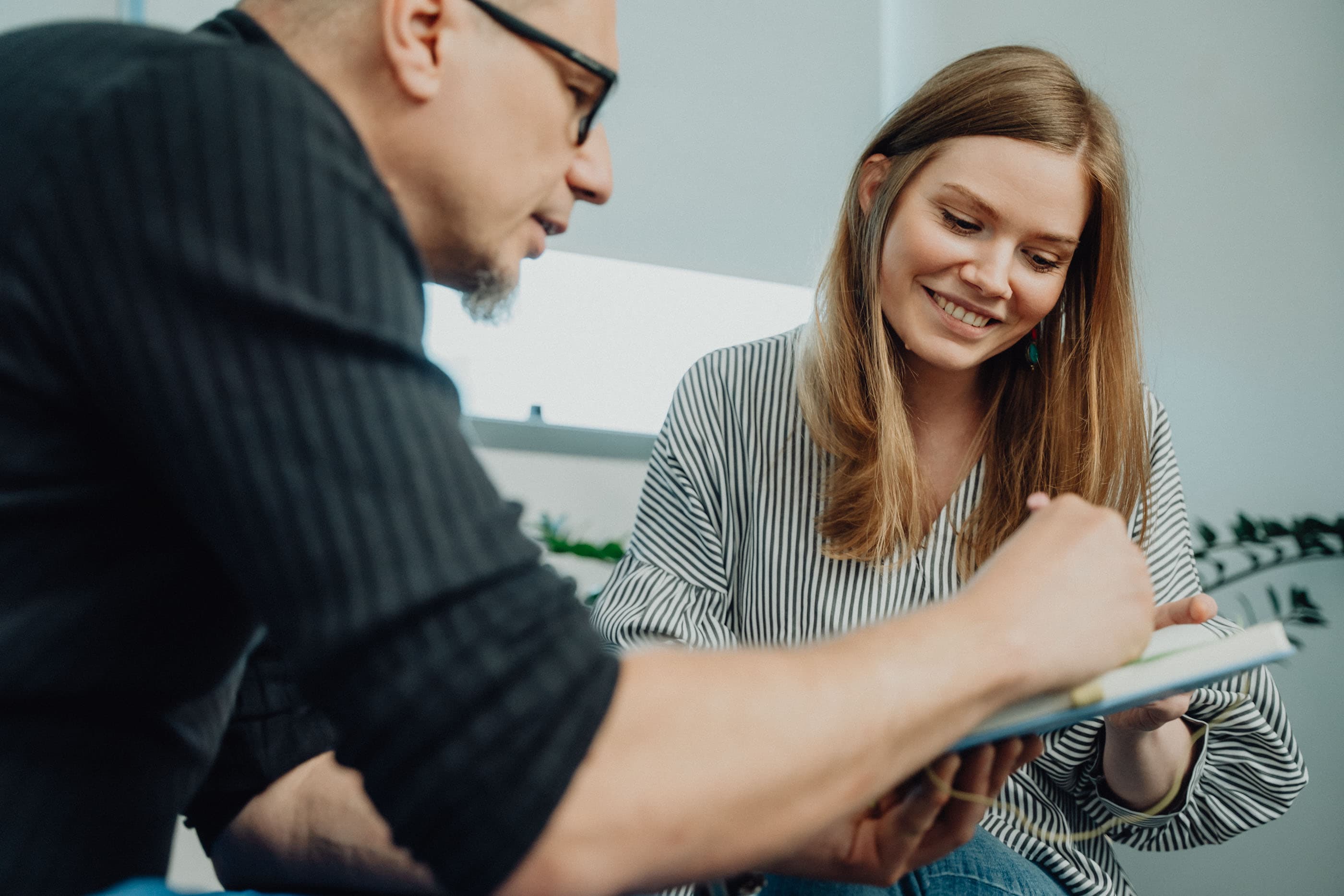
Navigating the Cloud: Understanding SaaS, PaaS, and IaaS
Discover the differences between SaaS, PaaS, and IaaS in cloud computing. This guide explains each model, their benefits, real-world use cases, and how to select the best option to meet your business goals.
Marek Pałys
Dec 12, 2024・11 min read
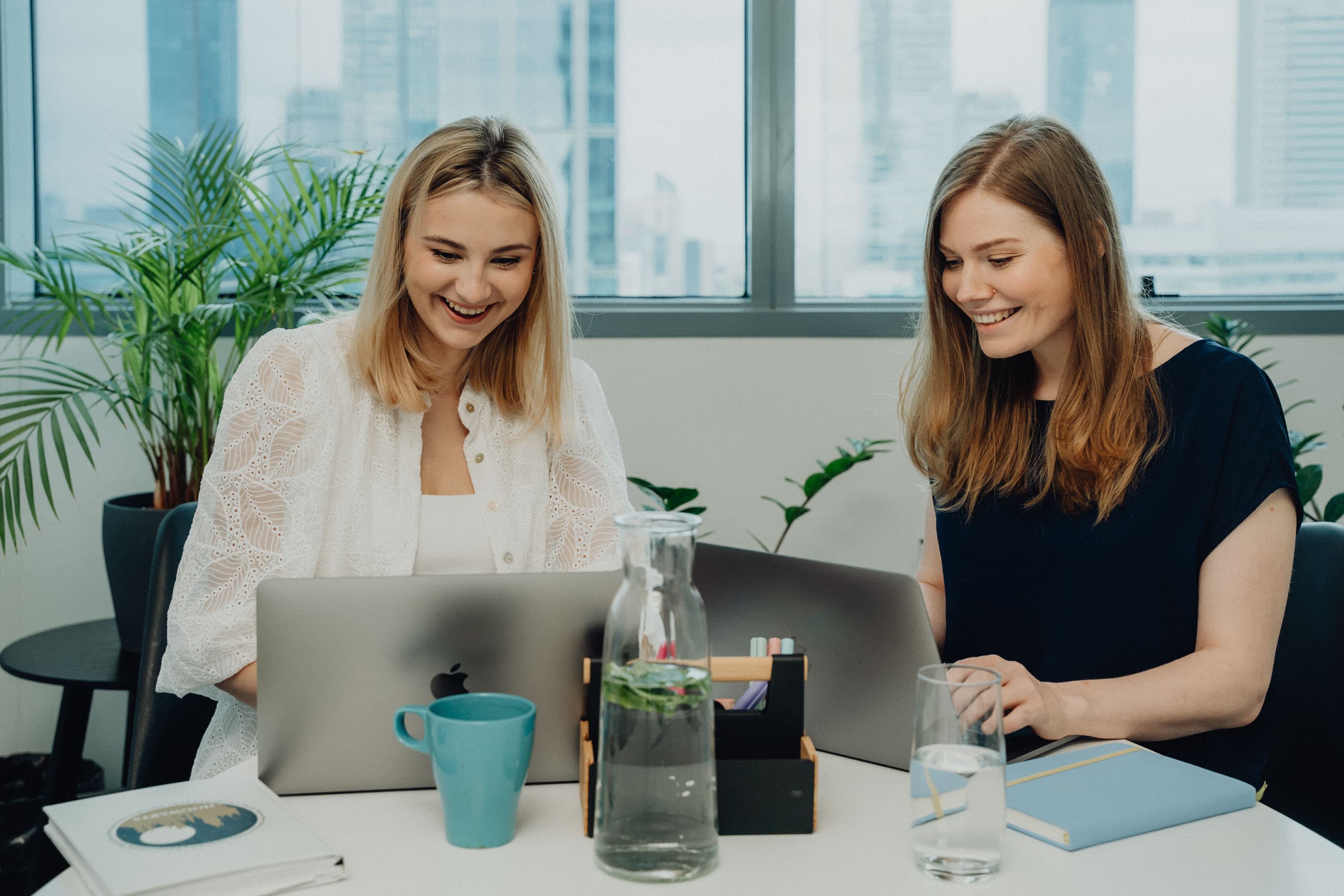
Cypress or Selenium: Making the Right Choice for Your Testing Needs
Cypress and Selenium are leading automated testing tools for web applications. Cypress offers speed, real-time feedback, and ease of setup, while Selenium supports multiple languages, browsers, and platforms for broader testing. Choosing the right tool depends on your project scope, testing needs, and environment.
Alexander Stasiak
Nov 26, 2024・5 min read