🌍 All
About us
Digitalization
News
Startups
Development
Design
Introduction to NestJS and RESTful APIs
Viktor Kharchenko
Nov 21, 2023・5 min read
Table of Content
What is NestJS?
Why NestJS?
RESTful APIs with NestJS
Understanding RESTful API Principles
Designing Your API Endpoints
Handling Data with NestJS Controllers
Middleware and Request Validation
Authentication and Authorization
Error Handling and Validation
Testing Your API with Unit Tests
Documentation with Swagger and NestJS
Deployment and Scaling Strategies
Conclusion and Next Steps
Welcome to the world of NestJS, a framework that brings structure and efficiency to building robust RESTful APIs with Node.js. Whether you're a seasoned developer or just starting your journey in web development, NestJS is a powerful tool to have in your arsenal.
What is NestJS?
NestJS is a progressive Node.js framework for building scalable and maintainable server-side applications. It combines the best features of modern web development with the power of TypeScript, a statically typed superset of JavaScript. This marriage of technologies brings order to the sometimes chaotic world of backend development.
Why NestJS?
Modular and Scalable: NestJS encourages a modular approach to building applications. You can create reusable modules, making it easier to scale your application as it grows.
TypeScript: With TypeScript, you get the benefits of static typing, code autocompletion, and better tooling. This leads to fewer bugs and more robust code.
Decorator-Based Syntax: NestJS uses decorators to define routes, controllers, and providers, making your codebase clean and readable. Here's a simple example:
// Define a basic controller
import { Controller, Get } from '@nestjs/common';
@Controller('hello')
export class HelloController {
@Get()
sayHello(): string {
return 'Hello, NestJS!';
}
}
Built-in Support for RESTful APIs: NestJS is designed with RESTful APIs in mind. It provides features like routing, request handling, and serialisation out of the box.
RESTful APIs with NestJS
REST (Representational State Transfer) is an architectural style for designing networked applications. RESTful APIs follow certain principles, such as statelessness and the use of HTTP methods (GET, POST, PUT, DELETE) to perform operations on resources.
NestJS simplifies the creation of RESTful APIs by providing decorators like @Get, @Post, @Put, and @Delete to define your routes. It also offers tools for request validation, error handling, and data serialisation.
In the example above, the @Get() decorator defines a GET endpoint for the /hello route, and the sayHello() method handles the incoming request and returns a "Hello, NestJS!" response.
Now that we've laid the foundation, we're ready to embark on a journey through the world of NestJS and build RESTful APIs step by step.
Understanding RESTful API Principles
Before we start building RESTful APIs with NestJS, let's establish a solid understanding of the principles that govern RESTful architecture. REST, which stands for Representational State Transfer, is an architectural style that forms the backbone of modern web services. So, what exactly are these principles, and why are they important?
RESTful Resource-Based Architecture
At the core of REST is the concept of resources. In the context of web services, a resource is any piece of data that can be identified by a unique URL. Resources can represent objects, data, or even processes. For example, a blog post, a user profile, or a payment transaction can all be considered resources.
Stateless Communication
RESTful APIs are designed to be stateless, which means that each request from a client to a server must contain all the information necessary to understand and process that request. The server should not rely on any previous requests or sessions. This statelessness simplifies scaling and makes APIs more robust and predictable.
HTTP Methods
HTTP methods, also known as HTTP verbs, play a crucial role in RESTful API design. The four primary HTTP methods used in REST are:
GET: Used to retrieve data from the server. For example, fetching a list of products or reading a specific article.
POST: Used to create new resources on the server. It's typically used for actions like creating a new user account or submitting a form.
PUT: Used to update existing resources or create them if they don't exist. For example, updating a user's profile information.
DELETE: Used to remove resources from the server. It might be used to delete a user account or remove a product from a catalogue.
Resource Representation
Resources in REST are represented in a format, often JSON or XML, which contains both the data and the metadata about that resource. The format should be self-descriptive, allowing clients to understand the resource's content and its relationships.
Here's a simplified example of a JSON representation of a user resource:
{
"id": 1,
"name": "John Doe",
"email": "john@example.com"
}
Uniform Interface
A key principle of REST is a uniform and consistent interface. This means that clients can interact with different resources using a small set of well-defined methods (HTTP verbs) and standard conventions.
By understanding these fundamental principles of REST, you'll be better prepared to design and implement RESTful APIs with NestJS that are both efficient and user-friendly. In the next sections, we'll put these principles into action as we dive deeper into building RESTful APIs.
Designing Your API Endpoints
Now that we've grasped the fundamental principles of RESTful architecture, it's time to dive into the exciting task of designing your API endpoints. The way you structure your endpoints can significantly impact the usability and maintainability of your API.
Resource-Centric Design
In RESTful API design, endpoints should be centred around resources, representing the entities you want to manipulate. Each resource should have a unique URI (Uniform Resource Identifier) that clearly identifies it. For example:
/users: Represents a collection of user resources.
/users/1: Represents a specific user with ID 1.
Using HTTP Methods
HTTP methods play a crucial role in determining the action to be performed on a resource. Let's explore how to use these methods effectively:
GET: Use it to retrieve data. For instance, a GET /users request fetches a list of users, while GET /users/1 retrieves the details of a specific user.
POST: Employ it to create a new resource. A POST /users request might create a new user.
PUT: Use it for updating an existing resource. For example, a PUT /users/1 request could update user information for the user with ID 1.
DELETE: Utilise it to remove a resource. A DELETE /users/1 request would delete the user with ID 1.
Versioning Your Endpoints
As your API evolves, it's essential to maintain backward compatibility for existing clients while introducing new features. One way to achieve this is through versioning. You can include the version number in the URI, like /v1/users, to ensure that clients can choose which version of the API to use.
Query Parameters
Sometimes, you need to provide additional information to your endpoints. This is where query parameters come into play. For example, you can use ?page=2&limit=10 in a GET /users request to paginate results.
Request and Response Formats
Ensure that your API follows a consistent request and response format, typically in JSON or XML. This makes it easier for clients to understand and work with your API. Here's an example of a JSON request and response:
Request:
{
"email": "john@example.com"
}
Response:
{
"id": 1,
"name": "John Doe",
"email": "john@example.com"
}
By adopting these principles and best practices for designing your API endpoints, you'll create a well-structured and user-friendly API that developers will appreciate working with. In the next sections, we'll delve into implementing these designs using NestJS to bring your RESTful API to life.
Handling Data with NestJS Controllers
NestJS controllers are the heart of your API. They handle incoming HTTP requests, process data, and send back responses. Think of them as the traffic cops of your application, directing requests to the right places.
Creating a Controller
To create a controller in NestJS, you simply decorate a class with the @Controller decorator, specifying the base route that this controller will handle. Here's a basic example:
import { Controller, Get } from '@nestjs/common';
@Controller('users')
export class UsersController {
@Get()
findAll(): string {
return 'This endpoint retrieves a list of users.';
}
}
In this example, we've created a UsersController that handles requests to the /users route.
Request Handling
Controllers use methods to handle HTTP requests. The @Get(), @Post(), @Put(), and @Delete() decorators define which HTTP method each method should handle. Here's an example of handling a GET request:
@Get()
findAll(): string {
return 'This endpoint retrieves a list of users.';
}
Dependency Injection
NestJS leverages dependency injection to manage dependencies in your controllers. For instance, you can inject services into your controllers to interact with data sources or perform business logic.
import { Controller, Get } from '@nestjs/common';
import { UsersService } from './users.service';
@Controller('users')
export class UsersController {
constructor(private readonly usersService: UsersService) {}
@Get()
findAll(): string {
const users = this.usersService.findAll();
return `Found ${users.length} users.`;
}
}
In this example, we inject the UsersService into the UsersController to fetch user data.
NestJS controllers offer a powerful and organised way to handle incoming requests, making your API development more structured and maintainable. In the next sections, we'll dive deeper into middleware, request validation, error handling, and other essential topics to build a complete RESTful API.
Middleware and Request Validation
Middleware plays a crucial role in the request-response cycle of your NestJS application. It allows you to preprocess requests, perform validation, and execute code before it reaches your controller methods. Additionally, request validation ensures that incoming data meets your API's requirements.
Understanding Middleware
Middleware functions are executed in the order they are defined in your application. You can use them for tasks like logging, authentication, and request parsing. NestJS offers built-in middleware and allows you to create custom middleware as well.
Here's an example of a simple middleware that logs incoming requests:
import { Injectable, NestMiddleware } from '@nestjs/common';
import { Request, Response, NextFunction } from 'express';
@Injectable()
export class LoggerMiddleware implements NestMiddleware {
use(req: Request, res: Response, next: NextFunction) {
console.log(`Request received: ${req.method} ${req.url}`);
next();
}
}
Request Validation
Request validation ensures that the data sent to your API adheres to predefined rules. NestJS simplifies this process using decorators and class validator. For instance, you can validate incoming POST data:
import { Controller, Post, Body } from '@nestjs/common';
import { CreateUserDto } from './dto/create-user.dto';
@Controller('users')
export class UsersController {
@Post()
create(@Body() createUserDto: CreateUserDto) {
// createUserDto contains validated data
return 'User created successfully';
}
}
In this example, the @Body() decorator uses the createUserDto class to validate the request body against predefined rules.
Middleware and request validation are essential for maintaining the integrity of your API and ensuring that it handles data securely and efficiently. In the following sections, we'll explore more about authentication and error handling in the context of building RESTful APIs with NestJS.
Authentication and Authorization
Authentication and authorization are vital aspects of any secure RESTful API. They ensure that only authorised users can access certain resources and perform specific actions.
Authentication
Authentication is the process of verifying the identity of a user or system. NestJS provides various strategies for authentication, including Passport.js integration. You can use strategies like JWT (JSON Web Tokens) or OAuth2 to authenticate users.
Here's an example of setting up JWT-based authentication:
import { Strategy, ExtractJwt } from 'passport-jwt';
import { PassportStrategy } from '@nestjs/passport';
import { Injectable } from '@nestjs/common';
@Injectable()
export class JwtStrategy extends PassportStrategy(Strategy) {
constructor() {
super({
jwtFromRequest: ExtractJwt.fromAuthHeaderAsBearerToken(),
secretOrKey: 'your-secret-key',
});
}
async validate(payload: any) {
return { userId: payload.sub, username: payload.username };
}
}
Authorization
Authorization comes into play after authentication. It defines what an authenticated user can or cannot do within the application. You can use decorators like @Roles and @AuthGuard to implement authorization logic.
import { Controller, Get, UseGuards } from '@nestjs/common';
import { RolesGuard } from './roles.guard';
@Controller('admin')
@UseGuards(RolesGuard)
export class AdminController {
@Get()
findAll(): string {
return 'Admin-only resource';
}
}
In this example, the AdminController is restricted to users with specific roles, thanks to the RolesGuard.
Implementing robust authentication and authorization mechanisms is crucial for protecting your RESTful API and ensuring that only authorised users can access sensitive resources. In the following sections, we'll delve deeper into data persistence, error handling, and other essential topics in building RESTful APIs with NestJS.
Error Handling and Validation
Error handling and validation are critical components of any reliable RESTful API. They ensure that your API responds gracefully to errors and enforces data integrity.
Error Handling
NestJS provides robust error handling mechanisms, allowing you to gracefully handle errors and return meaningful responses. You can use built-in exception filters to catch and format errors for clients.
Here's an example of handling a not-found error:
import { NotFoundException, Controller, Get } from '@nestjs/common';
@Controller('users')
export class UsersController {
@Get(':id')
findOne(@Param('id') id: string) {
const user = findUserById(id);
if (!user) {
throw new NotFoundException(`User with ID ${id} not found`);
}
return user;
}
}
In this example, we throw a NotFoundException when a user is not found, which NestJS automatically translates into an appropriate HTTP response.
Request Validation
Request validation ensures that incoming data meets the expected criteria. You can use the class-validator library to define validation rules for your DTOs (Data Transfer Objects). For instance, validating an email address:
import { IsEmail } from 'class-validator';
export class CreateUserDto {
@IsEmail()
email: string;
}
By decorating the email property with @IsEmail(), you ensure that it contains a valid email address.
Implementing effective error handling and request validation in your NestJS API enhances its reliability and usability. In the next sections, we'll explore data persistence, documentation, and testing to further refine our RESTful API.
Testing Your API with Unit Tests
Testing is a crucial aspect of building a reliable RESTful API with NestJS. It helps you catch and prevent issues early in the development process and ensures that your API behaves as expected.
Unit tests focus on individual parts of your application, such as services or controllers, in isolation. NestJS provides built-in testing utilities, making it easy to write unit tests.
Here's a basic example of a unit test for a service:
import { Test, TestingModule } from '@nestjs/testing';
import { UsersService } from './users.service';
describe('UsersService', () => {
let service: UsersService;
beforeEach(async () => {
const module: TestingModule = await Test.createTestingModule({
providers: [UsersService],
}).compile();
service = module.get<UsersService>(UsersService);
});
it('should be defined', () => {
expect(service).toBeDefined();
});
it('should return an array of users', () => {
const users = service.findAll();
expect(users).toBeInstanceOf(Array);
});
});
By writing thorough unit tests you can ensure that your NestJS RESTful API functions correctly and reliably. In the next sections, we'll explore documentation, deployment, and best practices to bring your API to production.
Documentation with Swagger and NestJS
Creating clear and comprehensive documentation for your RESTful API is essential for both developers and users. NestJS makes this task effortless with Swagger, a powerful tool for API documentation.
What is Swagger?
Swagger is an open-source framework that allows you to describe, document, and visualise RESTful APIs. With NestJS, you can generate Swagger documentation automatically from your code, ensuring that it stays up-to-date as your API evolves.
Setting up Swagger in NestJS
To get started, you'll need to install the @nestjs/swagger package and configure it in your NestJS application. Here's a simplified example:
import { DocumentBuilder, SwaggerModule } from '@nestjs/swagger';
const config = new DocumentBuilder()
.setTitle('Your API')
.setDescription('API description')
.setVersion('1.0')
.addTag('users')
.build();
const document = SwaggerModule.createDocument(app, config);
SwaggerModule.setup('api', app, document);
This code sets up Swagger with basic information about your API and tags for grouping routes.
Viewing Swagger Documentation
Once configured, you can access your API's Swagger documentation by visiting /api in your application's URL. This interactive interface allows developers to explore your API, test endpoints, and understand how to use it effectively.
Swagger simplifies the process of keeping your API documentation up-to-date and makes it more accessible to your development team and API consumers.
In the next sections, we'll explore deployment strategies and best practices to ensure your NestJS RESTful API is ready for production.
Deployment and Scaling Strategies
After you've built your NestJS RESTful API, the next critical step is deploying it to a production environment and preparing for potential scalability challenges. Deployment and scaling are key aspects of making your API accessible, reliable, and capable of handling increased traffic.
Deployment
1. Choose a Hosting Environment:
Select a hosting environment that suits your needs. Popular options include cloud providers like AWS, Azure, Google Cloud, or platform-as-a-service providers like Heroku.
2. Configuration Management:
Use configuration management tools or environment variables to manage application configurations in different environments (development, staging, production).
3. Deployment Pipeline:
Implement a deployment pipeline that automates the deployment process. Tools like Jenkins, Travis CI, or GitLab CI/CD can help streamline this.
Scaling Strategies
1. Vertical Scaling:
Increase the resources (CPU, RAM) of a single server. This approach is useful when you want to handle more traffic on a single machine.
2. Horizontal Scaling:
Add more servers or instances to distribute the load. NestJS, with its modular architecture, is well-suited for horizontal scaling.
3. Load Balancing:
Implement load balancers to evenly distribute incoming requests among multiple server instances. Tools like Nginx or cloud load balancers can help achieve this.
4. Containerization:
Use containerization technologies like Docker to package your application and its dependencies. Container orchestration platforms like Kubernetes or Docker Swarm facilitate the management and scaling of containers.
Here's a simplified example of a Docker Compose file for scaling a NestJS application:
version: '3'
services:
app:
image: your-api-image
ports:
- "3000:3000"
deploy:
replicas: 3 # Scale to 3 instances
Effective deployment and scaling strategies ensure that your NestJS API remains reliable and performs well as it grows. Always monitor your application's performance and make adjustments as needed to meet increasing demands.
Conclusion and Next Steps
Congratulations! You've journeyed through the comprehensive guide to building RESTful APIs with NestJS, gaining insights into the fundamental concepts and practical techniques that make NestJS a powerful choice for API development.
What You've Accomplished
Understanding RESTful Principles: You've grasped the core principles of REST architecture, enabling you to design APIs that are efficient, scalable, and user-friendly.
NestJS Controllers: You've learned how to create controllers to handle HTTP requests and manage your API's logic.
Middleware and Validation: You've explored middleware and request validation, ensuring the security and integrity of incoming data.
Authentication and Authorization: You've implemented authentication and authorization to secure your API, allowing only authorized users to access specific resources.
Error Handling and Testing: You've discovered error handling techniques and testing strategies to ensure the reliability and correctness of your API.
Documentation with Swagger: You've set up Swagger for API documentation, making your API more accessible and understandable.
Deployment and Scaling: You've explored deployment options and scaling strategies to prepare your API for production and handle increased traffic.
What's Next?
Your journey doesn't end here. Building RESTful APIs is an ongoing process that can continually evolve and improve. Consider the following next steps:
Optimization: Fine-tune your API for performance, and explore caching strategies, database optimization, and query optimization.
Logging and Monitoring: Implement robust logging and monitoring solutions to track and troubleshoot issues in your production environment.
Security Audits: Regularly conduct security audits to identify and mitigate potential vulnerabilities.
API Versioning: As your API matures, think about versioning to maintain backward compatibility for existing clients while introducing new features.
Community Engagement: Join the NestJS community, participate in discussions, and share your knowledge and experiences with others.
Remember, building RESTful APIs is not just about code but also about providing value to your users. Keep the user experience in mind and focus on delivering reliable, well-documented, and secure APIs. With NestJS as your foundation, you're well-equipped to embark on your API development journey with confidence. Best of luck, and happy coding!
FAQs
How do I start building a RESTful API with NestJS?
To begin, create a NestJS project using the Nest CLI, define controllers, routes, and services, and set up your API endpoints.
What is the role of middleware in NestJS?
Middleware in NestJS intercepts and processes incoming requests. It's used for tasks like authentication, logging, and request preprocessing.
How can I secure my NestJS API with authentication?
Implement authentication using strategies like JWT (JSON Web Tokens) or OAuth2 to verify the identity of users.
What is Swagger, and how does it help with API documentation?
Swagger is an open-source framework that simplifies the generation of API documentation. In NestJS, it automatically generates interactive documentation based on your code, making it easy for users to understand and test your API.
What is the difference between unit testing and end-to-end testing in NestJS?
Unit testing focuses on testing individual units of code, like functions or modules, in isolation. End-to-end testing, on the other hand, tests the entire application or API, simulating real user interactions.
What are some best practices for deploying a NestJS API?
Select a suitable hosting environment (e.g., AWS, Heroku), automate deployment with CI/CD tools, and use environment variables for configuration.
How can I scale my NestJS API to handle increased traffic?
You can scale vertically by upgrading server resources (CPU, RAM) or horizontally by adding more server instances to distribute the load.
What is load balancing, and why is it important for scaling?
Load balancing distributes incoming requests evenly among multiple server instances, ensuring efficient resource utilization and high availability.
What is containerization, and how does it relate to NestJS?
Containerization, using tools like Docker, packages your NestJS application and its dependencies into containers. It simplifies deployment and scaling.
How can I optimise the performance of my NestJS API?
Optimise performance through techniques like caching, database indexing, query optimization, and minimising unnecessary computations.
Why is logging and monitoring important for a production-ready API?
Logging and monitoring help you identify and resolve issues in real-time, ensuring the reliability and availability of your API in production.
What security measures should I consider for my NestJS API?
Regularly conduct security audits, implement input validation, protect against common vulnerabilities (e.g., SQL injection, XSS), and use secure authentication methods.
When should I consider API versioning?
API versioning becomes necessary when introducing changes while maintaining backward compatibility for existing clients. It ensures a smooth transition for users.
How can I engage with the NestJS community?
Engage with the community by participating in discussions on forums, attending meetups or conferences, and contributing to open-source projects related to NestJS.
What's the significance of user experience in API development?
A good user experience in API development includes providing well-documented, secure, and reliable APIs. This fosters user satisfaction and encourages adoption.
Can I use NestJS for microservices development?
Yes, NestJS's modular architecture makes it well-suited for building and scaling microservices, allowing you to develop complex, distributed systems.
How do I handle file uploads in NestJS?
Handle file uploads by integrating libraries like multer into your NestJS application. These libraries facilitate file handling and storage.
What tools can I use for automated testing in NestJS?
Commonly used tools for automated testing in NestJS include Jest for unit testing and Supertest for end-to-end testing.
Are there any NestJS plugins or extensions to enhance API development?
NestJS boasts a rich ecosystem of plugins and extensions that streamline API development. Explore these extensions to add features and functionality to your API.
What are some common pitfalls to avoid in NestJS API development?
Avoid overcomplicating your code, neglecting error handling and validation, and failing to thoroughly document your API. These practices can lead to maintenance challenges and usability issues.
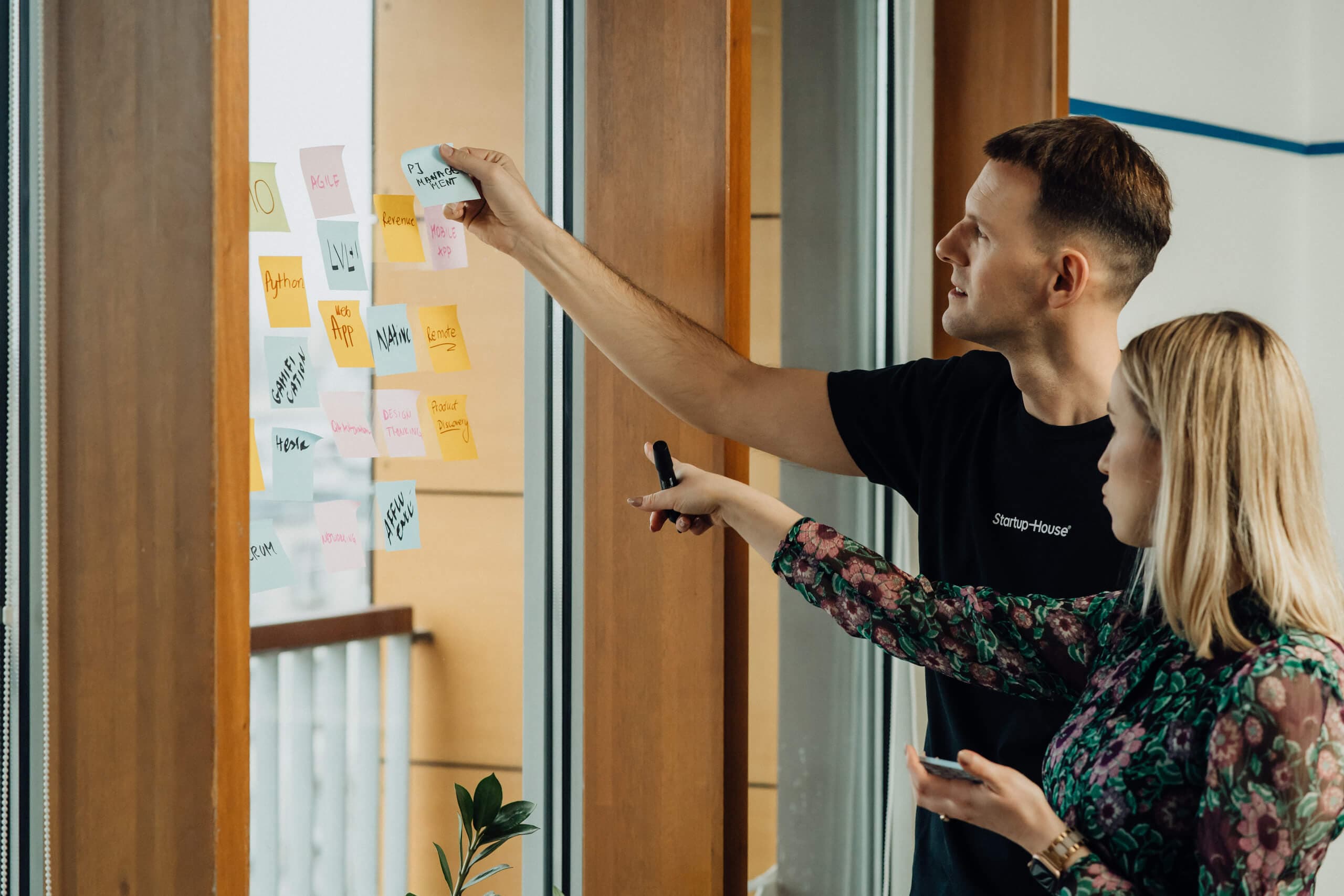
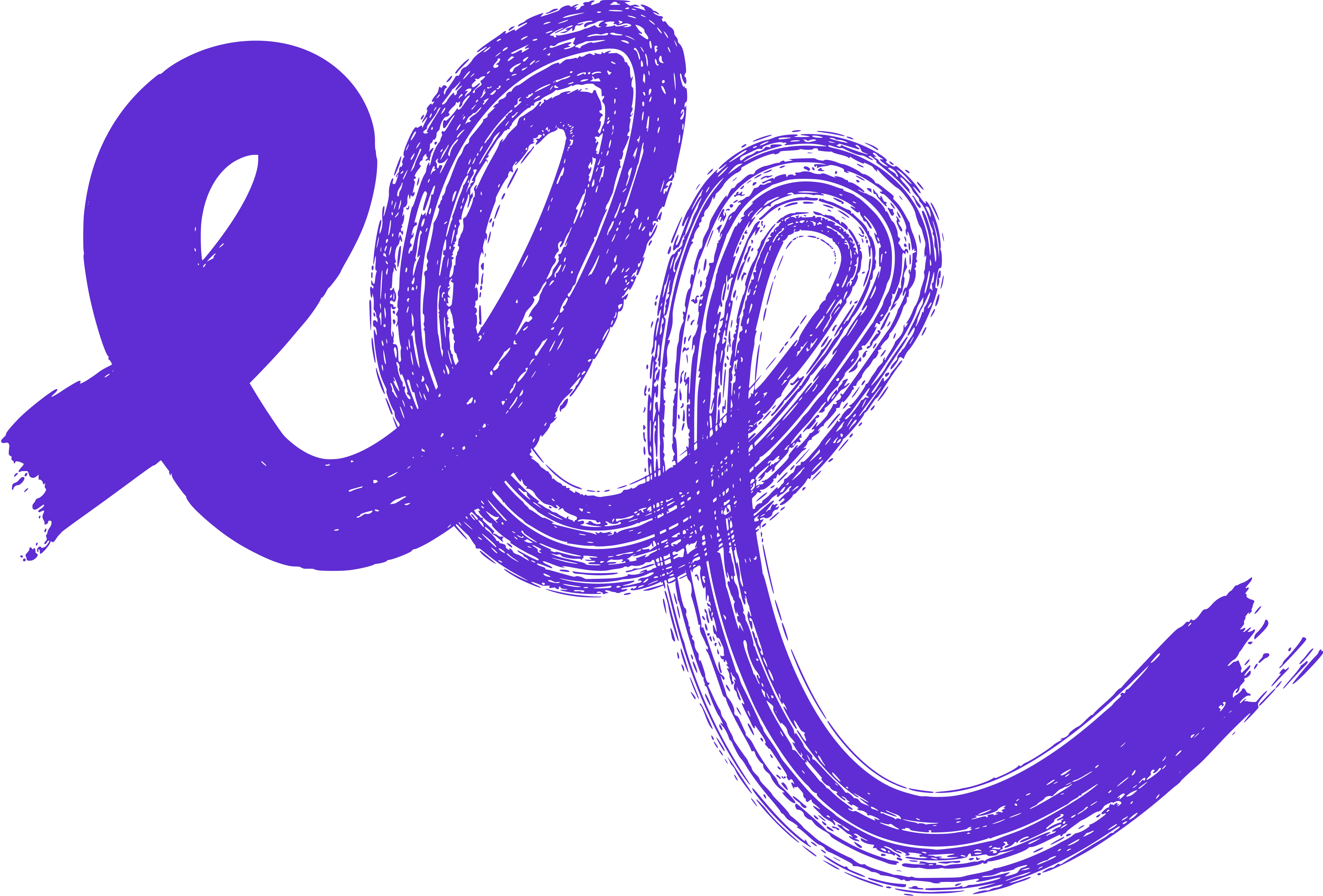
You may also
like...
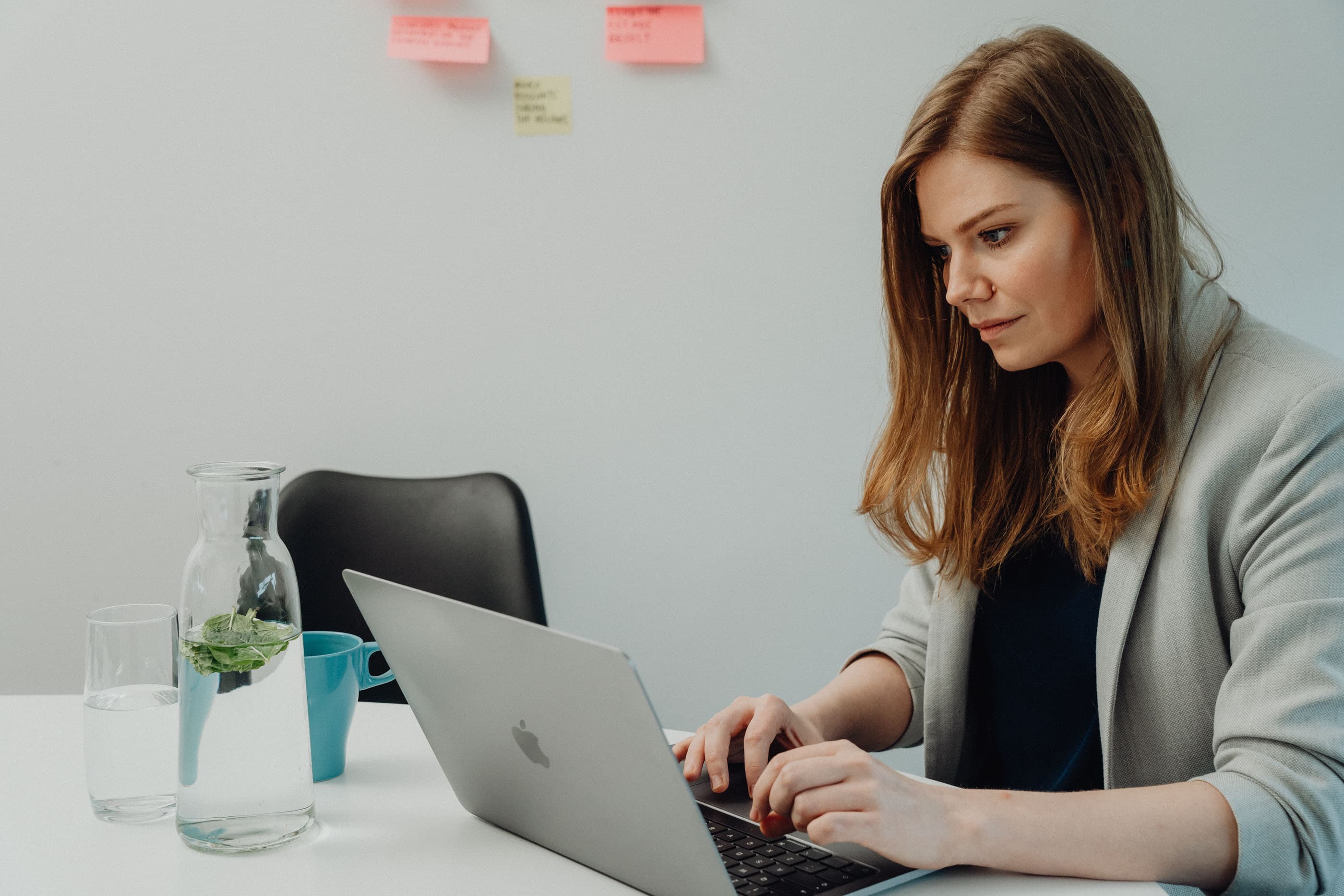
Understanding Event-Driven Programming: A Simple Guide for Everyone
Explore the essentials of event-driven programming. Learn how this responsive paradigm powers interactive applications with real-world examples and key concepts.
Marek Pałys
Apr 30, 2024・9 min read
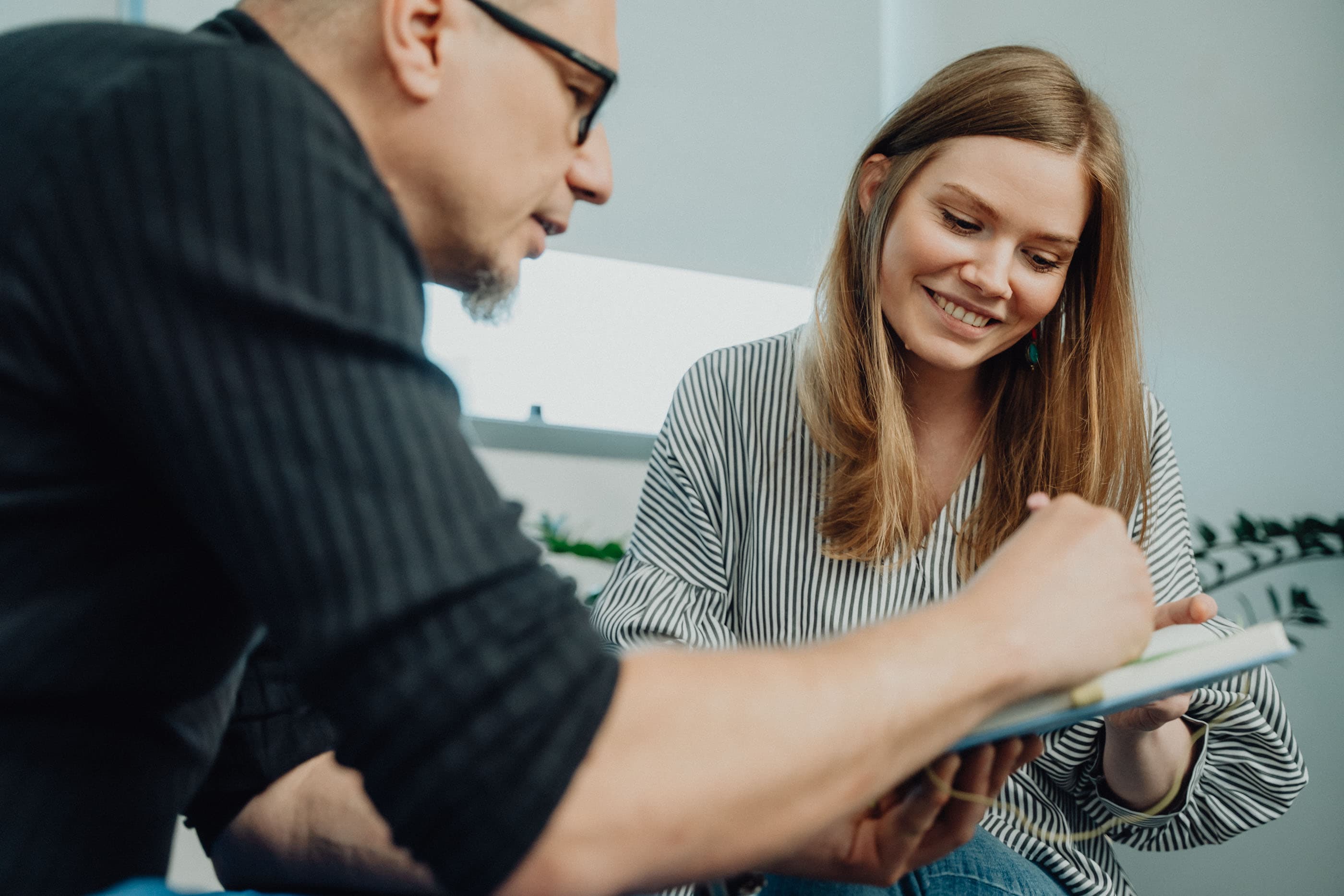
Navigating the Cloud: Understanding SaaS, PaaS, and IaaS
Discover the differences between SaaS, PaaS, and IaaS in cloud computing. This guide explains each model, their benefits, real-world use cases, and how to select the best option to meet your business goals.
Marek Pałys
Dec 12, 2024・11 min read
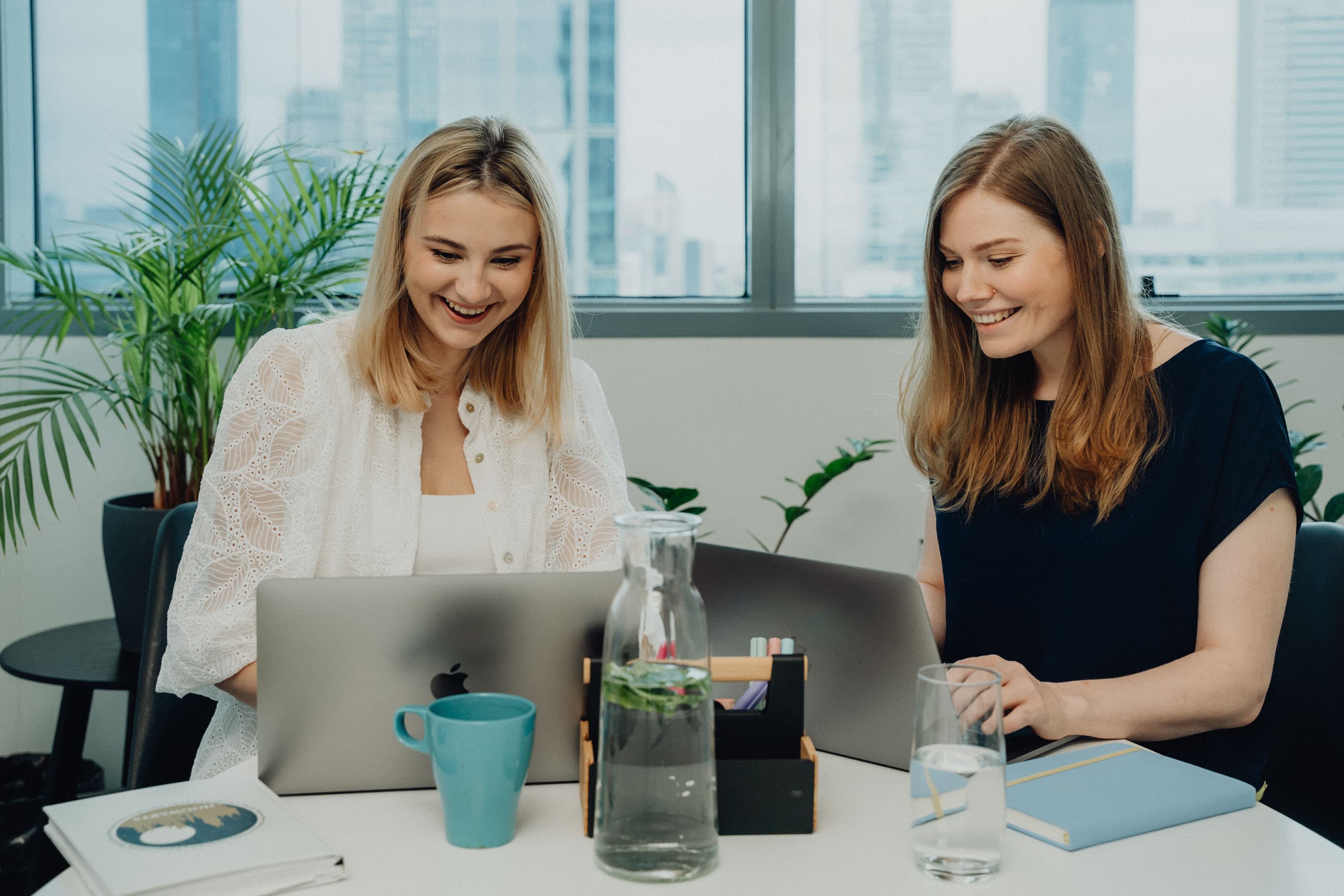
Cypress or Selenium: Making the Right Choice for Your Testing Needs
Cypress and Selenium are leading automated testing tools for web applications. Cypress offers speed, real-time feedback, and ease of setup, while Selenium supports multiple languages, browsers, and platforms for broader testing. Choosing the right tool depends on your project scope, testing needs, and environment.
Alexander Stasiak
Nov 26, 2024・5 min read