🌍 All
About us
Digitalization
News
Startups
Development
Design
Monolith vs Microservices: Choosing Wisely
Viktor Kharchenko
Jan 09, 2024・5 min read
Table of Content
Monolithic architecture
Microservices architecture
Monolithic Architecture Explained
When to Choose Monolithic Architecture
Microservices Architecture Explained
Scalability: Monolith vs. Microservices
Conclusion
FAQs:
In the ever-evolving landscape of software development, choosing the right architectural approach is akin to deciding the foundation for a skyscraper. It's a pivotal choice that will significantly impact your project's success. Enter the age-old debate: Monolith vs. Microservices Architecture.
Monolithic architecture
Our first contender resembles a single, massive block of code—a monolith. Here, all components of an application are bundled together, tightly intertwined like a well-knit scarf. This architectural style provides simplicity, with all functionality within arm's reach. Consider a simple web application in Python, where everything is housed in a single file:
def main():
initialize_database()
start_server()
handle_requests()
if __name__ == "__main__":
main()
Microservices architecture
On the flip side, we have Microservices architecture, a paradigm shift that favours breaking down your software into smaller, independent services, each with its unique functionality. Think of it as a team of specialised experts working in harmony. Take, for instance, an e-commerce platform. The catalogue, shopping cart, and payment processing are all distinct microservices, each serving a specific purpose.
# Catalog Service
def get_product_details(product_id):
# ...
# Cart Service
def add_to_cart(user_id, product_id):
# ...
# Payment Service
def process_payment(user_id, cart_items):
# …
But when do you choose one over the other? What are the benefits and trade-offs? Throughout this article, we will embark on a journey into the intricacies of these architectural giants. We'll explore their strengths and weaknesses, dissect real-world case studies, and equip you with the knowledge to make an informed decision for your next software endeavor. So, whether you're building a small application or architecting a digital empire, join us as we unravel the mysteries of Monolith vs. Microservices Architecture.
Monolithic Architecture Explained
Imagine constructing a grand mansion, where every room, from the living area to the kitchen and bedrooms, is merged into a single, colossal structure. This is the essence of monolithic architecture in the realm of software development. In a monolith, all components of an application are tightly bound together within a single codebase, much like the rooms of a house under one roof.
To illustrate this concept, let's delve into a simplified Python web application:
# Monolithic Web Application
# Import required libraries
from flask import Flask, request, render_template
from flask_sqlalchemy import SQLAlchemy
# Create a Flask application
app = Flask(__name__)
# Configure the database
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///myapp.db'
db = SQLAlchemy(app)
# Define a data model
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(80), unique=True, nullable=False)
# Define a route and view function
@app.route('/')
def index():
users = User.query.all()
return render_template('index.html', users=users)
# Run the application
if __name__ == '__main__':
app.run(debug=True)
In this example, all aspects of the web application, including the web server, database handling, and user interface, are tightly coupled within a single codebase. While this approach simplifies development and initial setup, it can present challenges as your project evolves.
As your application grows, maintaining a monolith becomes increasingly complex. Any modification may have ripple effects, and scaling the application horizontally can be cumbersome. However, for small to medium-sized projects or when rapid development is a priority, the monolithic architecture remains a viable choice.
Understanding monolithic architecture is the first step in the "Monolith vs. Microservices" debate. In the next sections, we'll explore the alternative approach, microservices architecture, and delve into the intricacies of choosing the right architecture for your software project.
When to Choose Monolithic Architecture
In the intricate world of software architecture, the choice between monolith and microservices isn't a one-size-fits-all decision. There are scenarios where opting for a monolithic architecture is not only practical but advantageous.
Simplicity in the Beginning: When you're embarking on a small-scale project, simplicity is your ally. A monolith offers a straightforward path. Imagine building a simple blogging platform in Python:
# Monolithic Blog Platform
# Define a list to store blog posts
blog_posts = []
# Create a function to add a new post
def create_post(title, content):
blog_posts.append({"title": title, "content": content})
# Create a function to list all posts
def list_posts():
return blog_posts
# Run the application
if __name__ == '__main__':
while True:
print("1. Create a new post")
print("2. List all posts")
choice = input("Enter your choice: ")
if choice == '1':
title = input("Enter post title: ")
content = input("Enter post content: ")
create_post(title, content)
elif choice == '2':
posts = list_posts()
for post in posts:
print(f"Title: {post['title']}")
print(f"Content: {post['content']}")
else:
print("Invalid choice. Try again.")
Resource Constraints: When you have limited resources, both in terms of budget and team size, a monolithic approach can be more cost-effective. It reduces the complexity of deployment and management.
Prototyping and Rapid Development: Monolithic architecture can be a great choice for prototyping or quickly bringing an idea to life. You can focus on building features without the overhead of managing multiple services.
However, it's essential to remember that while monolithic architecture has its merits, it may not be the best choice for large, complex applications. As your project grows, you might need to consider transitioning to a microservices architecture to maintain scalability and manageability. The key is to evaluate your project's current needs and future potential before making the architectural leap.
Microservices Architecture Explained
In the world of software development, where change is constant and adaptability is paramount, microservices architecture stands as a beacon of flexibility and scalability. Unlike the monolithic approach we explored earlier, microservices architecture is akin to constructing a city with interconnected neighbourhoods, each serving a specific purpose.
Imagine building a modern e-commerce platform using Python with microservices:
# Product Service
# Handles product information
class ProductService:
def get_product_details(self, product_id):
# Retrieve and return product details
# Cart Service
# Manages shopping cart operations
class CartService:
def add_to_cart(self, user_id, product_id):
# Add product to user's cart
# Payment Service
# Deals with payment processing
class PaymentService:
def process_payment(self, user_id, cart_items):
# Process payment and fulfil the order
In this example, we've split the e-commerce platform into distinct microservices—Product, Cart, and Payment. Each service specialises in a specific area of functionality, allowing for independent development, scaling, and maintenance.
Microservices advantages
- Scalability: You can scale individual microservices based on their demand, ensuring optimal resource utilisation.
- Flexibility: Teams can work on and deploy microservices independently, promoting agility and reducing coordination overhead.
- Fault Isolation: If one microservice encounters an issue, it's less likely to impact the entire system, enhancing fault tolerance.
- Technology Diversity: Microservices enable the use of different technologies and programming languages, allowing you to choose the right tool for each job.
However, microservices architecture isn't without its challenges. It introduces complexities in terms of communication, data consistency, and deployment orchestration. The decision to adopt microservices should be influenced by the size and complexity of your project, your team's expertise, and your willingness to embrace the intricacies of distributed systems.
As we continue our exploration of Monolith vs. Microservices Architecture, we'll delve deeper into the practical aspects of implementing and managing microservices, helping you make informed decisions for your software projects.
When to Choose Microservices Architecture?
Microservices architecture is not a one-size-fits-all solution, but it shines in specific scenarios where its characteristics align with your project's needs.
Complexity at Scale: If your project is vast and likely to expand, microservices offer a way to manage complexity. Consider a social media platform like Instagram. It encompasses user profiles, image uploads, comments, and more. In Python, you could structure it like this:
# User Service
class UserService:
def create_user(self, user_data):
# Create a new user
# Image Service
class ImageService:
def upload_image(self, user_id, image_data):
# Upload and process images
# Comment Service
class CommentService:
def add_comment(self, user_id, image_id, comment_text):
# Add a comment to an image
Each service handles a specific aspect, making it easier to manage, scale, and update as the platform evolves.
- Independent Development Teams: If you have multiple development teams, each focusing on different parts of your application, microservices facilitate independent development. Each team can manage its microservice without stepping on each other's toes.
- Technology Diversity: Microservices allow you to choose the best technology stack for each service. For instance, you might use Django for user management, Flask for image processing, and FastAPI for comments, all within the same application.
- Frequent Changes: When your project requires rapid updates and deployments, microservices are advantageous. Changes to one microservice don't necessarily disrupt others, promoting agility.
However, the transition to microservices is not without its challenges. It introduces complexities in terms of inter-service communication, data consistency, and deployment orchestration. Ensure that your team has the necessary expertise and resources to manage a distributed architecture effectively.
Ultimately, the decision between monolith and microservices architecture hinges on your project's current and future requirements. Understanding when to choose microservices is crucial to harnessing the benefits of this architectural style effectively.
Scalability: Monolith vs. Microservices
Scalability is a pivotal consideration in software architecture, as it determines your system's ability to handle increased loads and user demands. Let's delve into how monolithic and microservices architectures differ in terms of scalability.
Monolithic Scalability:
In a monolithic architecture, scaling can be a challenging task. Imagine a single, colossal engine powering your entire application. To scale, you often need to replicate the entire monolith, which can be resource-intensive.
Consider an e-commerce platform where all components, including product catalogue, shopping cart, and payment processing, are tightly knit within a monolith. To handle increased traffic during a sale event, you might need to duplicate the entire monolithic stack:
# Monolithic Application Scaling
class MonolithApp:
def __init__(self):
self.database = Database()
self.server = Server()
def start(self):
self.database.initialize()
self.server.start()
def handle_requests(self):
while True:
request = self.server.receive_request()
self.database.process(request)
# Scaling Monolith
monolith_instances = [MonolithApp() for _ in range(10)]
This approach can be costly and resource-inefficient, as it replicates all components, even those that may not require additional capacity.
Microservices Scalability:
In contrast, microservices architecture offers a more granular approach to scalability. Each microservice represents a specific function, allowing you to scale only the services that need additional resources.
For instance, in the same e-commerce scenario, if the payment processing service experiences a surge in demand during a sale event, you can independently scale that service:
# Microservices Scaling - Payment Service
class PaymentService:
def process_payment(self, user_id, cart_items):
# Process payment and fulfil the order
# Scaling Payment Service
payment_service_instances = [PaymentService() for _ in range(50)]
This fine-grained scalability minimises resource wastage and ensures efficient allocation of resources to handle increased loads.
In summary, when it comes to scalability, microservices architecture offers a more flexible and efficient approach compared to monolithic architecture. Microservices allow you to scale individual components independently, ensuring that your system remains responsive and cost-effective as your user base grows.
Testing and Deployment Strategies
In the realm of software development, effective testing and deployment strategies are the pillars of a reliable and maintainable system. Let's explore how these strategies differ between monolithic and microservices architectures.
Monolithic Testing and Deployment:
In a monolithic architecture, testing often involves comprehensive end-to-end testing of the entire application. This means testing all interconnected components together. While it ensures that the system functions as a whole, it can be time-consuming and complex.
Deployment in a monolith typically involves updating the entire application, even if you've made changes to only a small part. This all-or-nothing approach can lead to downtime during deployments and requires careful planning to minimise service disruptions.
Microservices Testing and Deployment:
In contrast, microservices architecture promotes a more modular and focused approach to testing. Each microservice can be tested independently, allowing for quicker feedback and easier identification of issues. For example, testing a user authentication microservice in Python might look like this:
# Microservices Testing - User Authentication Service
def test_user_authentication_service():
# Set up test environment
auth_service = UserAuthenticationService()
# Test user registration
user_data = {"username": "testuser", "password": "password123"}
user_id = auth_service.register_user(user_data)
assert user_id is not None
# Test user login
result = auth_service.login("testuser", "password123")
assert result == True
# Clean up test environment
auth_service.cleanup()
Deployment in microservices can also be more agile. You can update and deploy individual services as needed without affecting the entire system. This minimises downtime and allows for continuous delivery.
However, microservices deployment introduces new challenges, such as service discovery, load balancing, and version management. Tools like container orchestration platforms (e.g., Kubernetes) and service meshes (e.g., Istio) help address these complexities.
In summary, while monolithic architectures demand comprehensive end-to-end testing and can involve all-or-nothing deployments, microservices architecture enables modular and independent testing and deployment of individual services. The choice between the two should align with your project's testing and deployment requirements and the level of agility you need to maintain a robust and responsive system.
Conclusion
In the dynamic world of software architecture, choosing between a monolithic and microservices approach is akin to selecting the foundation for your digital empire. Each has its merits and trade-offs.
Monolithic architecture offers simplicity in development and a unified codebase but may struggle with scalability and maintenance as projects expand.
On the other hand, microservices architecture provides flexibility, scalability, and independent development but introduces complexities in orchestration and communication.
The right choice hinges on your project's specific needs. For smaller, straightforward applications or when rapid development is crucial, a monolith may suffice. For large-scale, complex projects with scalability demands and diverse development teams, microservices offer a path to agility.
In the end, understanding these architectural paradigms empowers you to make an informed decision—one that aligns with your project's goals, resources, and future aspirations. So, choose wisely, and embark on your software development journey with confidence.
FAQs:
1. What is monolithic architecture in software development?
Monolithic architecture is an approach where all components of an application are tightly integrated into a single codebase.
2. What is microservices architecture?
Microservices architecture is a design pattern where an application is divided into small, independent services that communicate with each other.
3. When should I choose a monolithic architecture?
Consider a monolith for smaller projects, limited resources, or rapid development needs.
4. When should I opt for microservices architecture?
Microservices are suitable for large-scale projects, independent development teams, and scalability requirements.
5. What are the advantages of monolithic architecture?
Simplicity, unified codebase, and ease of initial development.
6. What are the disadvantages of monolithic architecture?
Challenges in scalability and maintenance as the project grows.
7. What are the advantages of microservices architecture?
Scalability, flexibility, and independent development and deployment.
8. What are the disadvantages of microservices architecture?
Complex orchestration, communication challenges, and potential overhead.
9. How does testing differ in monolithic and microservices architectures?
Monolithic testing involves end-to-end testing, while microservices allow for more modular and focused testing.
10. What is the key difference in deployment strategies between monoliths and microservices?
Monoliths often require all-or-nothing deployments, whereas microservices enable independent and agile deployments.
11. Is it possible to transition from a monolithic to a microservices architecture?
Yes, but it can be complex and requires careful planning and execution.
12. How do monolithic architectures handle fault tolerance?
Fault tolerance is limited in monolithic architectures, as a failure can affect the entire system.
13. How do microservices ensure fault tolerance?
Microservices can implement fault tolerance strategies independently, reducing the impact of failures.
14. Which approach is more suitable for a rapidly changing project?
Microservices are better suited for rapidly evolving projects due to their agility.
15. Can microservices use different technologies and programming languages?
Yes, microservices allow for technology diversity, enabling each service to use the most suitable stack.
16. What are some common challenges in transitioning from monoliths to microservices?
Challenges include data migration, communication protocols, and breaking dependencies.
17. How do microservices handle database management compared to monoliths?
Microservices often use separate databases for each service, while monoliths typically have a single database.
18. What role does container orchestration play in microservices architecture?
Container orchestration tools like Kubernetes help manage the deployment and scaling of microservices.
19. Are there any specific security considerations for microservices?
Yes, microservices introduce additional attack surfaces, making robust security practices crucial.
20. Which architecture is best for a startup with limited resources?
A monolithic architecture is a pragmatic choice for startups aiming for rapid development with limited resources.
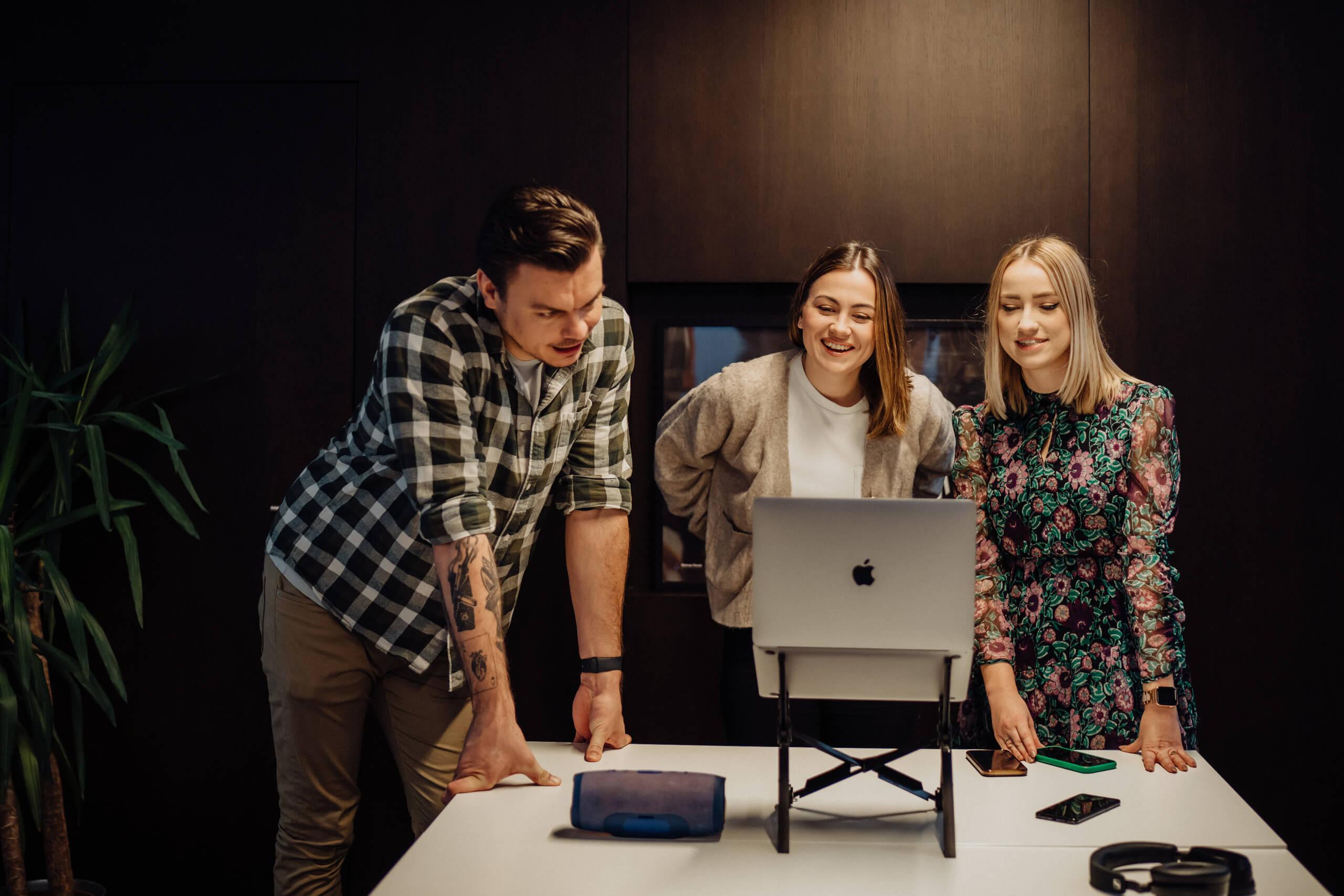
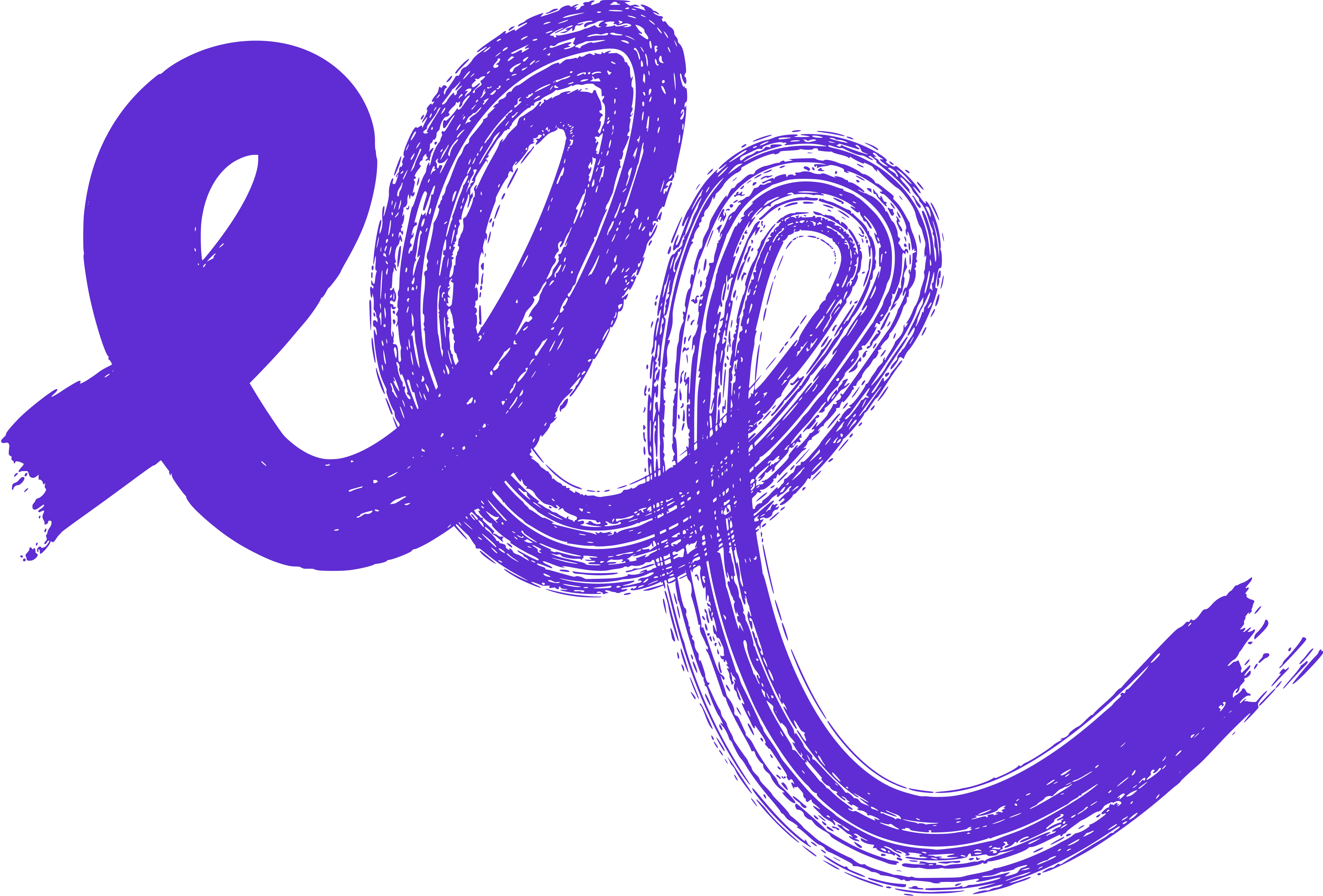
You may also
like...
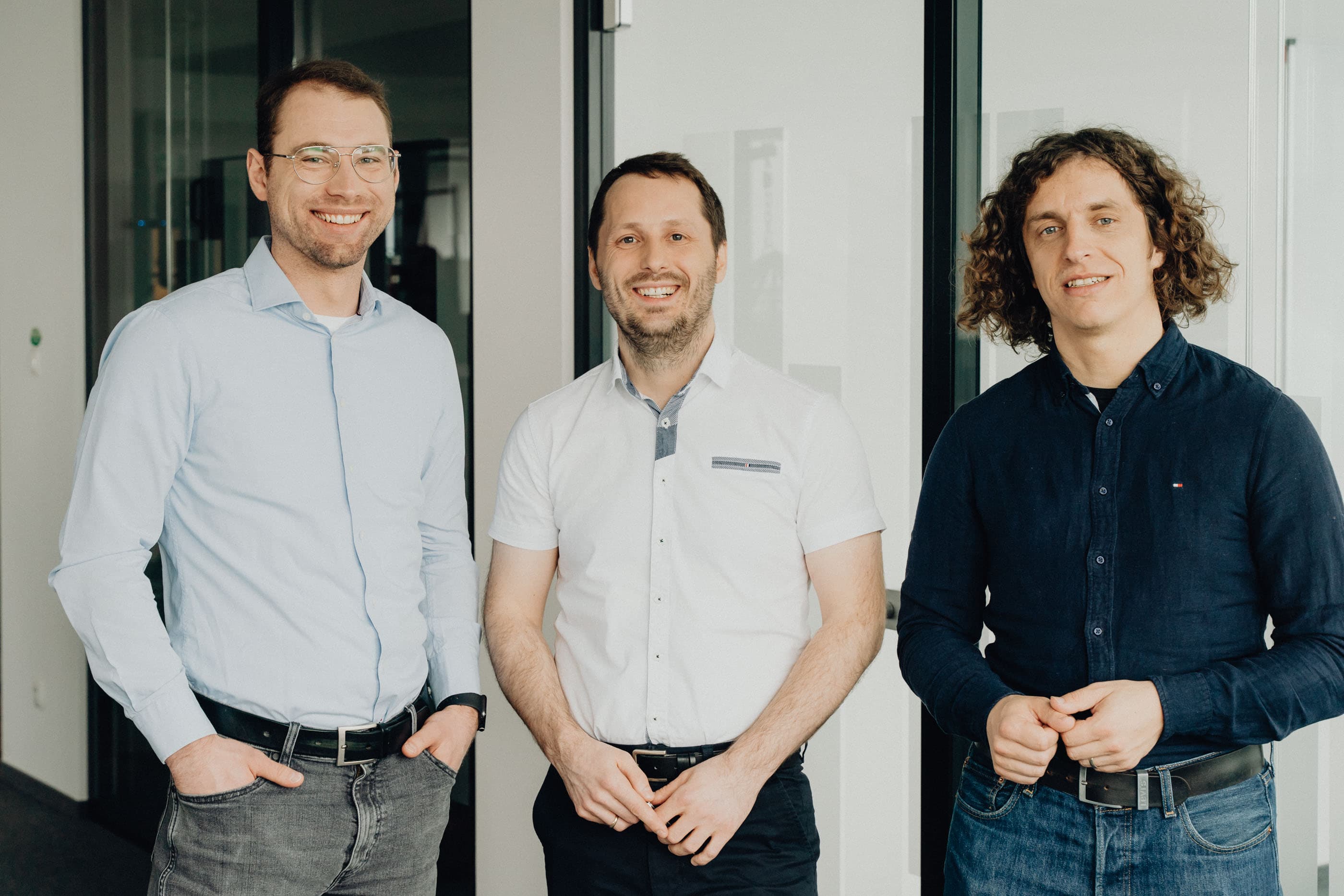
Python vs Scala: Choosing the Right Language for Your Project
Python and Scala are powerful programming languages with distinct strengths. Python excels in data science and machine learning with its simplicity, while Scala shines in big data and high-performance systems, leveraging its static typing and JVM integration.
Alexander Stasiak
Apr 17, 2024・5 min read
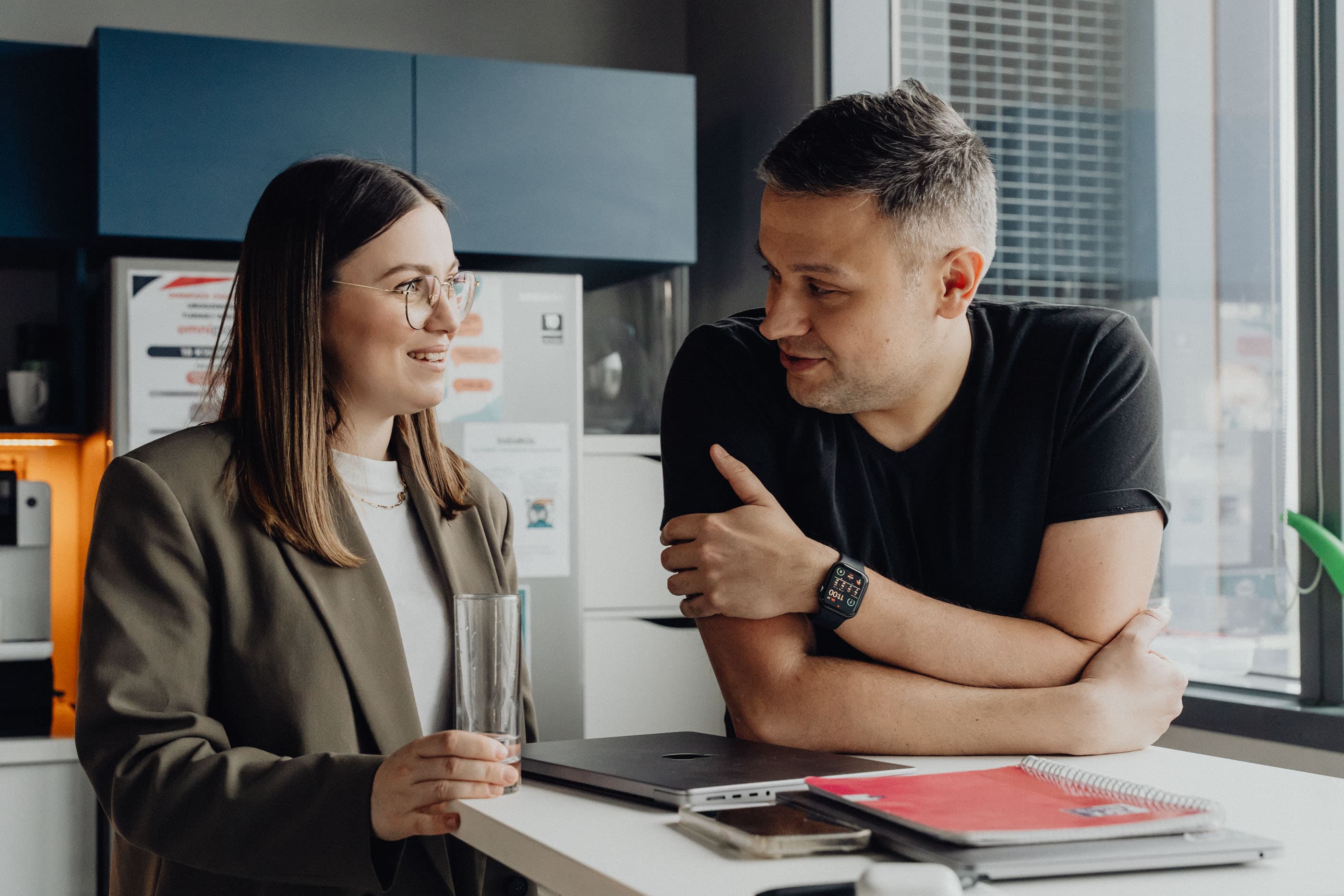
Top Python IDEs: Best Tools for Python Developers
Choosing the right Python IDE can enhance coding efficiency, debugging, and project management. This article reviews the top Python IDEs, including PyCharm, Visual Studio Code, and Jupyter Notebook, highlighting their key features and benefits for Python development.
Alexander Stasiak
Jul 09, 2024・5 min read
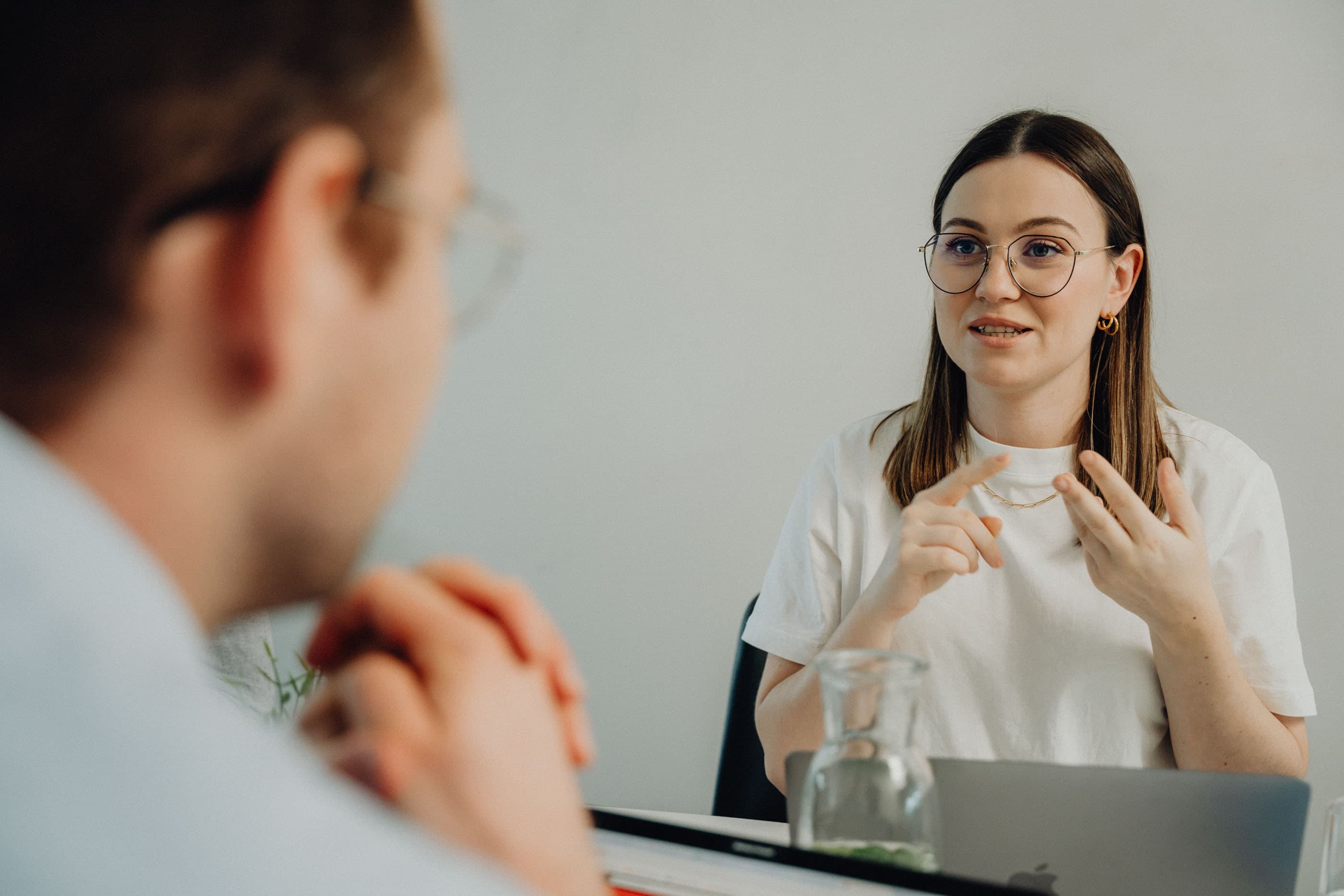
Python vs. C#: Comparing Two Powerful Programming Languages
Python and C# are versatile, powerful programming languages with unique strengths. Python excels in data science and rapid development due to its dynamic typing, while C# offers consistent syntax and robust support for game and enterprise development. This article explores their key differences and use cases.
Alexander Stasiak
May 01, 2024・4 min read