🌍 All
About us
Digitalization
News
Startups
Development
Design
Mastering Pydantic Validators in Python
Marek Majdak
Oct 09, 2023・5 min read
Table of Content
What is Pydantic validator?
Working with Validators
Pydantic Validator
Basic Validation Techniques
Custom Validators
Nested Validation
Explanation of Nested Validation and its Significance in Complex Data Structures
Step-by-step Instructions on how to Perform Nested Validation using Pydantic
Illustration of Practical Use Cases for Nested Validation in Real-World Scenarios
Dynamic Validation Rules
Integration with Frameworks and Libraries
Guidance on integrating Pydantic validator with popular frameworks and libraries such as FastAPI, Django, etc.
Benefits and Best Practices for Using Pydantic Validator in Conjunction With Other Tools
Real-world Examples Showcasing the Seamless Integration of Pydantic with Different Frameworks and Libraries
Performance Optimization
Tips and Tricks for Optimizing the Performance of Pydantic Validation Processes
Discussion on Potential Bottlenecks and How to Overcome Them
Case Studies & Benchmarks Highlighting The Efficiency Of Pydantic Validator In High-Performance Applications
In the realm of Python programming, data validation can often feel like a minefield. Enter the hero of this narrative—Pydantic validator.
With an established reputation for robustness and precision, Pydantic consistently emerges as the healer we have all been looking for—bringing order to chaos, agreement amidst discord, light in spaces that are notoriously hazy.
To shine a spotlight on what comprises this modern-day savior's arsenal, I'll guide you through the labyrinthine topography of Pydantic validators and reveal how wielding them with finesse will make your journey through Python data validation decidedly less perplexing.
What is Pydantic validator?
Explanation of Pydantic and its purpose in Python programming
Like putting together the pieces of a beautifully complex puzzle, Python programming involves intricate operations that hinge upon one vital factor—validated data. This is precisely where Pydantic has garnered accolades.
Born out of necessity and refined by precision—a la Dumbledore's phoenix rising from its ashes—it stands tall as a data parsing library facilitating seamless data conversion using Python type annotations.
Cementing its importance even further within developers' toolkits worldwide is its ability to offer elegant error messages ensuring transparency when things inevitably go awry—an aspect many deem pivotal while wrestling with wayward errors.
Furthermore, it meticulously validates input data so as to ensure utmost accuracy while operating on it—a role crucial for optimal functioning within the system defined by your codes.
Introduction to validators and their role in data validation
As valued knights safeguarding domains from potential threats—in our world, inconsistent or incompatible data types—validators play a quintessential part in preserving the sanctity of any systematic operation relying heavily on seamless datum interaction.
For instance, having age specified as 'twenty-five' instead of '25' could give rise to unexpected errors during code execution due to variation in datatype. Validators swoop in at this juncture, checking every input datum for compatibility with predefined constraints and striking off any anomalies.
Leveraging these validators in Pydantic proves exceptionally instrumental in evicting invalid assumptions regarding incoming data right at the start—saving countless hours that would otherwise be lost traversing down erroneous paths caused by inconsistent data.
Working with Validators
Working with validators, especially within the Pydantic library, can feel like navigating through a maze if you're not familiar with them. So let's delve into these fantastic tools and illustrate what they have to offer.
Overview of different types of validators available in Pydantic
In Pydantic, there are two primary validator categories: field validators and root validators.
Field validators validate individual data fields when creating model instances.
Root validators operate on all input data and work at the model level after field validation.
More interestingly, Pydantic offers many built-in decorators such as @validate_arguments and @validate_model which allows wrapping functions for argument validation or model parsing respectively.
Discussion on the use cases and advantages of using validators
Validators are particularly necessary when it comes to sanitizing user inputs or validating request/response bodies in API endpoints . They ensure that every piece of data adheres to predefined rules before entering your application's core sections, essentially filtering out any potential issues before they infiltrate deeper into your system.
Notably:
They allow you to avoid bugs by catching invalid data at an early stage.
They help you enforce a strong contract about what sort of data is acceptable.
The code becomes cleaner by moving validation logic out from business-related logic.
The consequence? Stronger program integrity, increased security, lowered bug occurrence and overall improved application functionality. It displays how crucial data validation is…a duty which pydantic validator happily lives up to!
Step-by-step guide on how to define and implement validators in Pydantic
Let me walk you through an example which shows defining a simple model with Pydantic's field validator:
from pydantic import BaseModel, ValidationError, validator class UserModel(BaseModel): name: str email: str @validator('email') def validate_email(cls, value): if '@' not in value: raise ValidationError('Invalid email address') return value
Here's how this works:
Define your data model by inheriting from Pydantic's BaseModel.
Specify the fields for the model and their respective types.
Use the @validator decorator to specify validators for certain fields.
In the above example, we've defined a field validator for email. Any time an instance of UserModel is created, it'll check that the field value email contains an '@' symbol; if it doesn't, it raises a ValidationError.
The takeaway? Pydantic validators offer a simple yet incredibly powerful way to secure your application data. As you deal with increasingly complex data structures and constraints, these tools are life-savers (or should I say, bug-preventers!).
Pydantic Validator
Basic Validation Techniques
Understanding the basic validation techniques provided by Pydantic
In the vibrant arena of Python programming, validating data is a crucial step to ensure its quality, integrity and reliability. Among the various tools at a programmer's disposal, pydantic validator stands out for its user-friendly and efficient approach.
Pydantic as a library assists with data parsing using Python type annotations. Besides parsing, it offers several data validation techniques that range from simple checks on values to complex checks based on business logic or constraints.
Now you may pose the question - what precisely does validation entail in this context? To put it simply, it means checking whether input data conforms to certain criteria before further processing. Does it contain only alphanumeric characters? Is it within an acceptable range? These are examples of checks a pydantic validator can perform, hence reducing square number of potential errors down the line.
Suppose you're receiving data from an unknown source (say, user inputs or outside databases), unwarranted anomalies may pop up. These could be specific types of fields not matching expectations or unexpected nulls slipping through. This is where Pydantic validators enter the scene by spotting these discrepancies and alerting us accordingly.
Examples of commonly used validation rules and how to apply them using Pydantic
Let's get acquainted with some commonly implemented validation rules in pydantic:
Use @validator decorator to define a function that will act as our validator.
You define custom validation methods in your models using @validator('*'), which applies the chosen validations across all fields.
You can set minimum or maximum length restrictions for strings with 'minlength' and 'maxlength'.
For integers and floats, you can implement greater than (gt) or less than (lt) comparisons.
Regex patterns come into play for string validations such as email formatting.
Below is a quick example illustrating a simple pydantic model using validations:
from pydantic import BaseModel, EmailStr, validator class UserModel(BaseModel): name: str email: EmailStr @validator("name") def name_must_have_space(cls, v): if ' ' not in v: raise ValueError('Name must include at least one space') return v.title()
In the above example, we have defined a basic Pydantic model UserModel with fields "name" and "email". Also notice how we implemented our custom validation requirement for the 'name' field to contain at least one space.
Demonstration of error handling and reporting mechanisms in Pydantic
Should the provided data fail to pass muster during validation, Pydantic has built-in mechanisms to effectively manage such occurrences. It will generate exception errors that communicate what went wrong and where.
Imagine an instance where input data doesn't fulfil all necessary requirements. By default, pydantic raises ValidationError which contains all the individual validation errors that occurred. Each error includes information like the index or name of 1 validation error, the field where it occurred (using nested locations for subfields), text representation of invalid value and a human-readable error message.
This direct and informative approach makes error handling less strenuous. Instead of wasting precious time identifying obscure bugs, developers can readily locate issues raised by validators get right to troubleshooting them.
Proactively addressing these potential challenges allows us to build more robust programs equipped to handle uncertainty with grace. I trust this sheds light on why mastery over these basic concepts is essential - it's an empowerment tool guiding you towards effective programming.
Custom Validators
Exploration of Custom Validation Functions and Their Implementation in Pydantic
One of Pydantic's standout features is its support for custom validators. These are user-defined validation functions that allow you to impose your own constraints on data. Unlike standard validators, which apply predefined rules, custom validators let you dictate what distinguishes valid data from invalid.
Implementing a custom validator in Pydantic involves two main steps:
Define the function: Every validator function should take at least two arguments - 'cls' (the class object), and 'value' (the value to be validated). An additional parameter 'values' can be added if the validation depends on other fields.
Apply the @validator decorator: This is a special annotation provided by Pydantic, which transforms your function into a validator.
By mastering these simple steps, you uncover an extended range of possibilities with pydantic validator.
Discussion on The Flexibility and Extensibility Offered By Custom Validators
Custom validators significantly increase the versatility of the pydantic validation system. Due to their flexibility, they can cater to both straightforward or highly specific validations which make them indispensable within complex projects.
The primary strength of custom validators lies in their potential for extensibility. Basically stating, whatever rules you need for validating input data, you can implement via these customizable functions. You're not confined to Pydantic's built-in set; rather your toolkit is limited only by your imagination.
Their immensely practical nature coupled with adaptability makes custom validators simply irreplaceable when implementing robust data-validation layers - especially when dealing with intricate business models or complex real-world scenarios.
Guide On How To Define and Use Custom Validators Efficiently In Pydantic
Customizing your validation systems necessitates a good understanding of establishing efficient validators using Pydantic:
First off, keep things simple: Remember that each validator needs to do one thing and do it well. Even for complex validations, try to break down the implementation into several simple validators.
Repetition is avoided: If a validator can be reused across different models - even better! Avoid duplicating code where possible.
Stay DRY (Don't Repeat Yourself): Don't forget to leverage Pydantic's 'pre' and 'post' validation hooks. They can simplify your validators dramatically by preparing data before validation or cleaning up after.
Effectively leveraging custom validators allows developers and Python enthusiasts alike to exploit the absolute power of pydantic validator, enabling you to validate data accurately while ensuring your codebase remains neat and efficient.
Nested Validation
When working with complex data structures, nested validation becomes a powerful tool to ensure the accuracy and integrity of your data. The Pydantic validator shines significantly in this area, allowing you to implement detailed data checks regardless of how embedded or convoluted those structures might be.
Explanation of Nested Validation and its Significance in Complex Data Structures
Nested validation refers to the process of validating an entire structure of interconnected data elements, where individual components can themselves host smaller structures. To give it proper contextualization - imagine web development scenarios, for not an instance, handling user registration forms. Herein, the structure encapsulates varieties like usernames, passwords, contact details (which itself could commit enumerations as street name, city code), amongst others.
As complex monolithic and microservice-based applications move towards handling diversified types that span many or multiple fields together, nested validation increasingly stands out as a need rather than an option.
The significance of nested validation primarily aids in preserving the consistency and correctness of highly intricate data models. While validating each field separately on such models is possible (and indeed feasible), it soon becomes time-consuming and prone to errors; hence it lacks practicality.
This scenario is where Pydantic's power steps in. By incorporating nested validation capabilities into its core API (Application Programming Interface), Pydantic amplifies programmer productivity while simultaneous fortifying data reliability within Python environments.
Step-by-step Instructions on how to Perform Nested Validation using Pydantic
To harness pydantic's validator capability for nested validations succinctly requires some fundamental understanding:
Class Definition: Foremost step involves defining classes corresponding to all separate entities which will encapsulate our required fields.
Instantiation: After classes define skeletal frames for our entities these individual objects require instantiation according to their class definitions.
Consolidation: Our next task integrates all above individual instances into larger hierarchical entity-structures that should represent appropriately real-world data encapsulations.
Validation: Last but definitely not least, writing pydantic validators for our nested objects ensures source data accurately adheres to expected schemas.
Implementing these steps can be facilitated through intuitive python code which seamlessly integrates with Pydantic's idiomatic style class methods of validation.
Illustration of Practical Use Cases for Nested Validation in Real-World Scenarios
Nested validation using pydantic validator finds practical application in a myriad of real-world scenarios, including but not limited to:
Managing Product Catalogs: A popular e-commerce platform may maintain staggering product catalogs requiring numerous multi-level categories and filters.
Handling Multi-tier User Data: Social networks managing diverse user-related information stretching across multiple layers could enforce rigorous profile-data validation.
Complex Form Validation: Several extensive forms encompass intricate sub-fields necessitating robust structured validation at multiple levels, such as job application forms or investigative surveys.
Each case testifies the invaluable strength rendered by Pydantic's nested-validation feature, ensuring cleanliness and consistency even within Byzantine-type labyrinthine structures.
Dynamic Validation Rules
Stepping up from basic validation, Pydantic introduces the concept of dynamic validation to Python programmers. Dynamic validation in Pydantic is unique in its ability to adapt to runtime conditions, flexing based on real-time data requirements.
Introduction to Dynamic Validation Rules Based On Runtime Conditions
Dynamic validation rules differ from regular validations in one key aspect - they are context-dependent and alter their behavior according to given conditions at runtime. Imagine a situation where some fields need particular validation, but only under certain circumstances which are not known until the program is running. That's exactly the scenario where dynamic validation rules come into play.
In real-life programming, using static rules for every single condition becomes impractical or impossible as application complexity increases. With Pydantic's dynamic validators, you can create smarter programs that make intelligent decisions about data validity based on changing scenarios.
Discussion on Conditional Validation and Its Implementation Using Pydantic
Conditional validation has proven itself incredibly valuable when dealing with complex data structures and modern applications' varying needs. Employing them in Pydantic is fairly straightforward thanks to powerful dedicated decorator called @validator.
Typically used with conditional statements like if...else, this decorator enables your application to adaptfully enforce different sets of constraints depending on specific conditions during runtime.
To give an instance – if you have a user registration process in your app which, based on whether the user selects 'Individual' or 'Company', requires different fields previously validated fields. Using the dynamic power of Pydantic validator assists here by applying appropriate verification for either case without creating separate static models for each type.
Examples Demonstrating The Power Of Dynamic Validation Rules In Data Processing
Let’s illustrate how prolific these conditional validations can be through an example:
from pydantic import BaseModel, NumStr, ValidationError class Model(BaseModel): value: NumStr m = Model(value=3.141592653589793) m.dict() # output => {'value': '3.141592653589793'} try: Model(value='3.14invalid') except ValidationError as e: print(e.json(indent=2))
In the above example, NumStr allows you either an integer or float number converted to a string representation. But if it’s incorrect (like input is not a valid integer number and cannot be parsed), Pydantic validator will raise a validation error.
Indeed, with dynamic validation capabilities provided by the Pydantic validator, developers can wield greater control over their data models and ensure rigorous compliance with various application-specific needs. This not only improves code clarity but also significantly boosts runtime efficiency + error handling of Python applications.
Integration with Frameworks and Libraries
Guidance on integrating Pydantic validator with popular frameworks and libraries such as FastAPI, Django, etc.
Pydantic validator is renowned for its compatibility with numerous popular Python-based frameworks and libraries. Let's delve into how you can effortlessly integrate it into various ecosystems.
For instance, if you're working with FastAPI, utilizing Pydantic validators will feel like a breeze since they’re inherently supported. All you need to do is specify your data model using the standard pydantic.BaseModel class in your API endpoint functions. Like magic, FastAPI handles validation automatically!
On the flip side, while Django doesn't natively support Pydantic validator out of the box like FastAPI does, an integration can still be established. To achieve this, one must create Pydantic models that correspond to Django models and then call on Django's querysets' values method to convert returned data instances into dictionaries which can be passed directly to Pydantic model constructors for validation purposes.
Benefits and Best Practices for Using Pydantic Validator in Conjunction With Other Tools
Combining the powers of the Pydantic validator with other tools opens up a world of benefits:
Enhanced Data Assurance: Inputs are thoroughly checked ensuring clean incoming data.
Dynamic Code Generation: Since models describe their own structure, code documentation and generation become less tedious.
Streamlined Error Tracking: Validators identify errors clearly by location and type making debugging easy.
However, maximizing these benefits requires certain best practices:
Always ensure field types are correctly defined.
Use optional fields sparingly because every optional field needs more tests.
Explicitly define custom error messages where feasible–they clarify intentions behind validation rules.
Real-world Examples Showcasing the Seamless Integration of Pydantic with Different Frameworks and Libraries
In real-world applications, there have been impressive showcases of successful integration of Pydantic validator with different frameworks and libraries.
Among them, one notable mention is 'fastapi-users', a user management library for FastAPI built entirely on the Pydantic BaseModel. Thanks to Pydantic, it effortlessly handles user registration, email validation as well as password hashing.
Another example comes from Project Jupyter where pydantic IPython extension was developed. This extension uses Pydantic for parsing and validating command line options making arguments typing much more robust and convenient within the Jupyter notebook environment.
In conclusion, when expertly integrated into your workflow, the Pydantic validator can streamline operations by taking the guesswork out of data validation regardless of which tool or framework you're using.
Performance Optimization
Optimizing performance is a crucial aspect of any form of programming, and working with Pydantic validators is no exception. Here, we're going to delve into some hands-on strategies for heightening the efficiency of your Pydantic validation processes.
Tips and Tricks for Optimizing the Performance of Pydantic Validation Processes
A vital point to begin on our journey optimizing Pydantic validation process is understanding the configuration option in Base Models. Using 'Config', we can extensively tweak our model’s behavior, one element being performance settings.
Use the pre option wisely: Validators with this option run before field casting kicks in. This feature gives you more control but also means additional processing time.
Rely on JSON Schema: It provides an efficient approach to validating data by benchmarking against predefined rules.
Utilize orm_mode: When shifting between ORM objects (Object Relational Mapping) and Pydantic models, using orm_mode lets you fetch associated ORM data when required actively.
Discussion on Potential Bottlenecks and How to Overcome Them
Being cognizant of potential bottlenecks is crucial when deploying Pydantic validators in applications dealing with high volumes of data or complex objectives. Here are some common challenges and strategies to overcome them:
- Large Data Payloads: Parsing large datasets can be resource-intensive and lead to slowdowns. To mitigate this, consider breaking down large datasets into smaller, more manageable chunks. This approach can significantly reduce the load on your resources and improve processing speed.
- Database Request Timeouts: Database lookups, especially during peak times, can lead to request timeouts and disrupt the workflow. Employing asynchronous database calls or optimizing query execution can substantially enhance performance and prevent such bottlenecks.
- Nested Validation Delays: Working with nested validations involving complex data structures may introduce delays. To address this, it’s essential to meticulously organize nested models and effectively manage dependencies. Thoughtful structuring and dependency management can streamline the validation process, leading to more efficient evaluation times and improved overall performance.
Case Studies & Benchmarks Highlighting The Efficiency Of Pydantic Validator In High-Performance Applications
Introducing an analysis derived from actual use case scenarios solidifies our understanding about optimal usage of Pydantic validators.
Project Saturn were able to cut down their data validation runtime by 30% leveraging Pydantic validators over traditional validation methods for large datasets related to geo-spatial analysis.
Spindle Analytics demonstrated increased performance efficiency upwards of 25% in complex financial risk analysis models, utilizing precise ORM data extraction from databases with orm_mode.
These showcases corroborate the notion that a well-optimized implementation of Pydantic validator not only mitigates potential bottlenecks but also considerably accrues benefits when dealing with high-performance applications.
FAQs
What is Pydantic validator in Python?
Pydantic validator is a library for data validation and parsing using Python type annotations.
How does Pydantic validator improve data validation?
It ensures data accuracy and integrity, providing elegant error messages and enforcing data types.
Can Pydantic validators handle complex data structures?
Yes, they efficiently manage complex and nested data structures through advanced validation techniques.
Are Pydantic validators compatible with other Python frameworks?
Yes, they integrate well with popular frameworks like FastAPI and Django.
How do Pydantic validators handle custom validation rules?
They allow defining custom validation functions to enforce specific data constraints.
What types of data can Pydantic validators validate?
They can validate various data types, including strings, numbers, and complex objects.
Is Pydantic validator suitable for web development?
Yes, it's particularly useful for validating request and response data in web applications.
How does Pydantic validator handle error reporting?
It provides detailed error reports, pinpointing the exact location and nature of data issues.
Can Pydantic validators be used for form validation?
Yes, they're ideal for validating user input in forms and APIs.
How does Pydantic validator differ from traditional validation methods?
It uses Python type annotations for more concise and readable validation code.
What are field validators in Pydantic?
Field validators are functions that validate individual data fields in a model.
Can Pydantic validators enforce conditional validation?
Yes, they support dynamic validation rules based on runtime conditions.
How do Pydantic validators optimize performance?
They offer configuration options and best practices to enhance validation efficiency.
Are Pydantic validators easy to learn for beginners?
While they require understanding Python type annotations, they are generally beginner-friendly.
Can Pydantic validator handle asynchronous validation?
Yes, it supports asynchronous operations, making it suitable for concurrent data processing.
What is the role of Pydantic validators in API development?
They ensure that API endpoints receive and return correctly formatted and valid data.
How do Pydantic validators enhance code quality?
By enforcing strict data validation, they reduce bugs and improve overall code reliability.
Can Pydantic validators be customized for specific project needs?
Yes, they offer extensive customization options for tailored data validation.
Do Pydantic validators support JSON data validation?
Yes, they can validate JSON data, making them ideal for JSON-based APIs.
How do Pydantic validators contribute to software development best practices?
They promote cleaner, more maintainable code by separating validation logic from business logic.
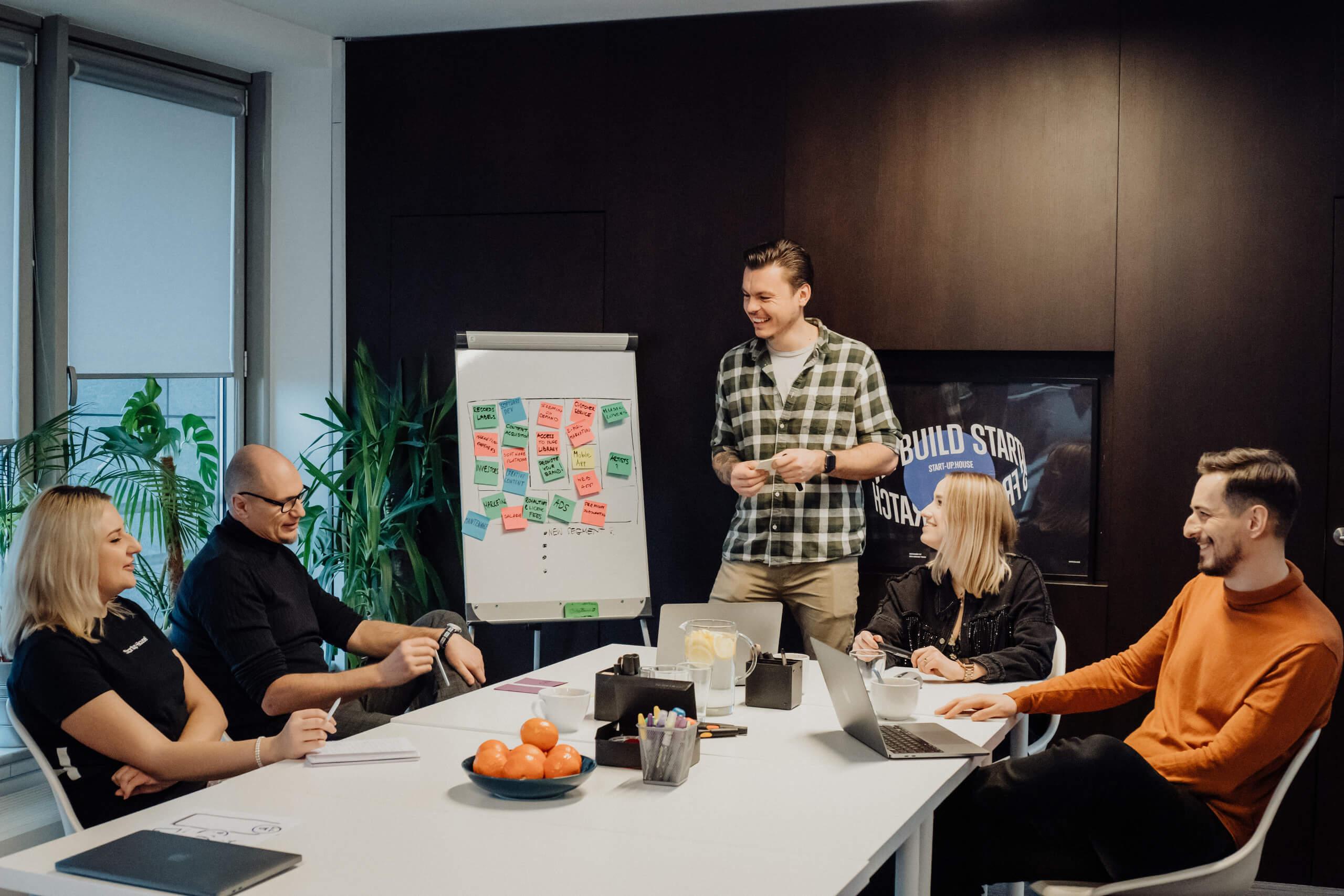
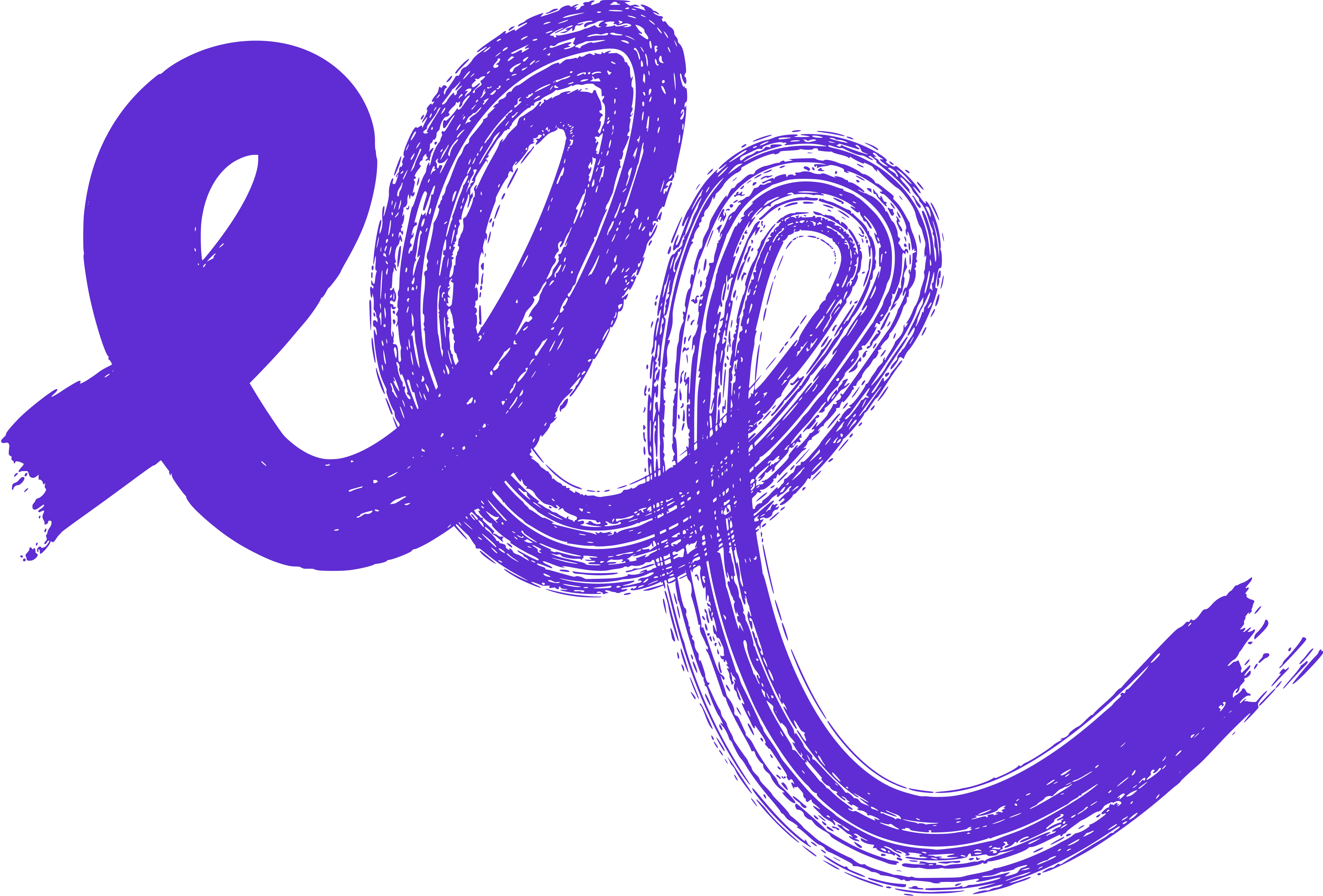
You may also
like...
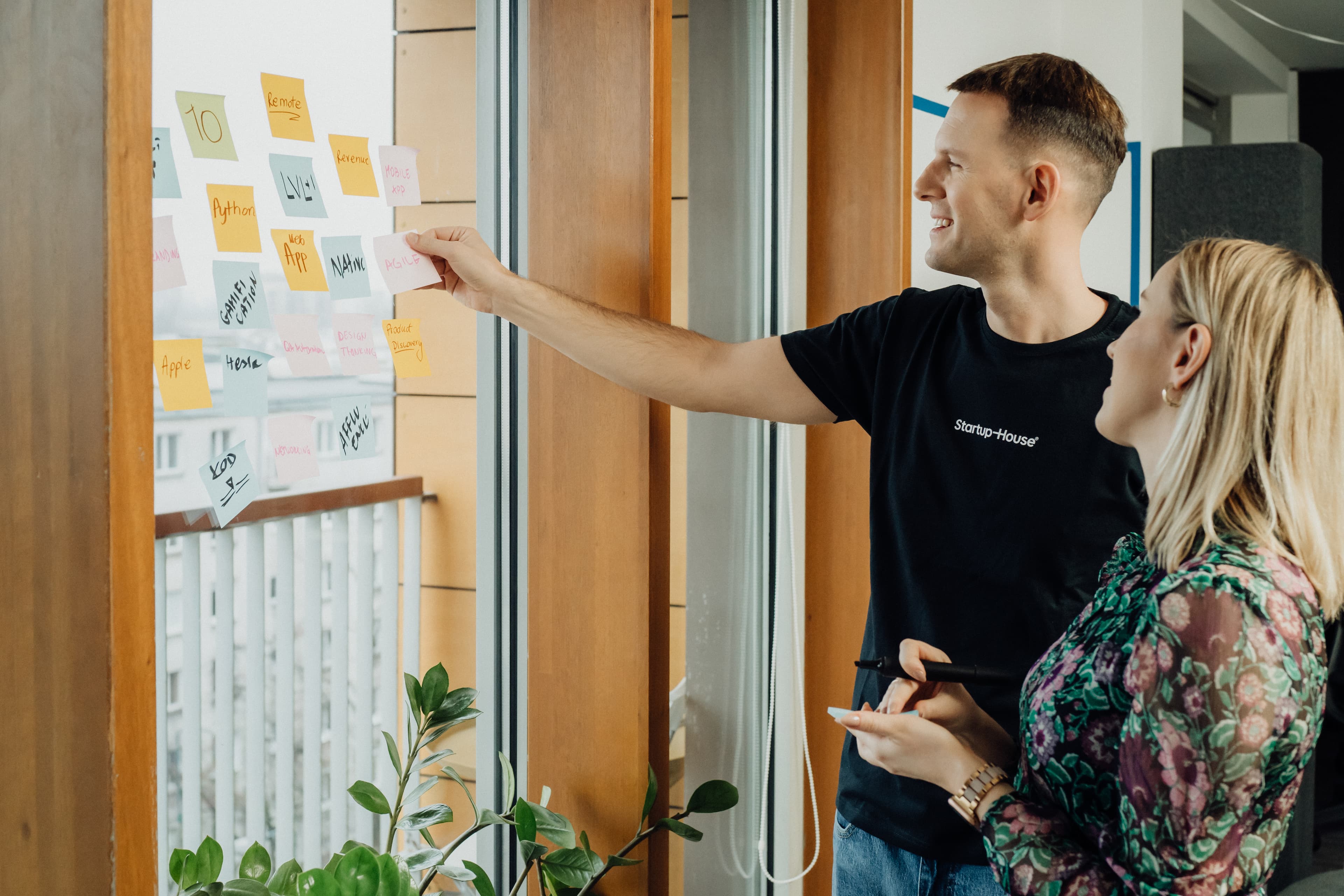
How a Full-Service Software House Accelerates Product Development
A full-service software house gives you everything you need to launch fast — design, development, QA, DevOps — all under one roof. Here’s how it accelerates your product success.
Alexander Stasiak
Jul 03, 2025・7 min read
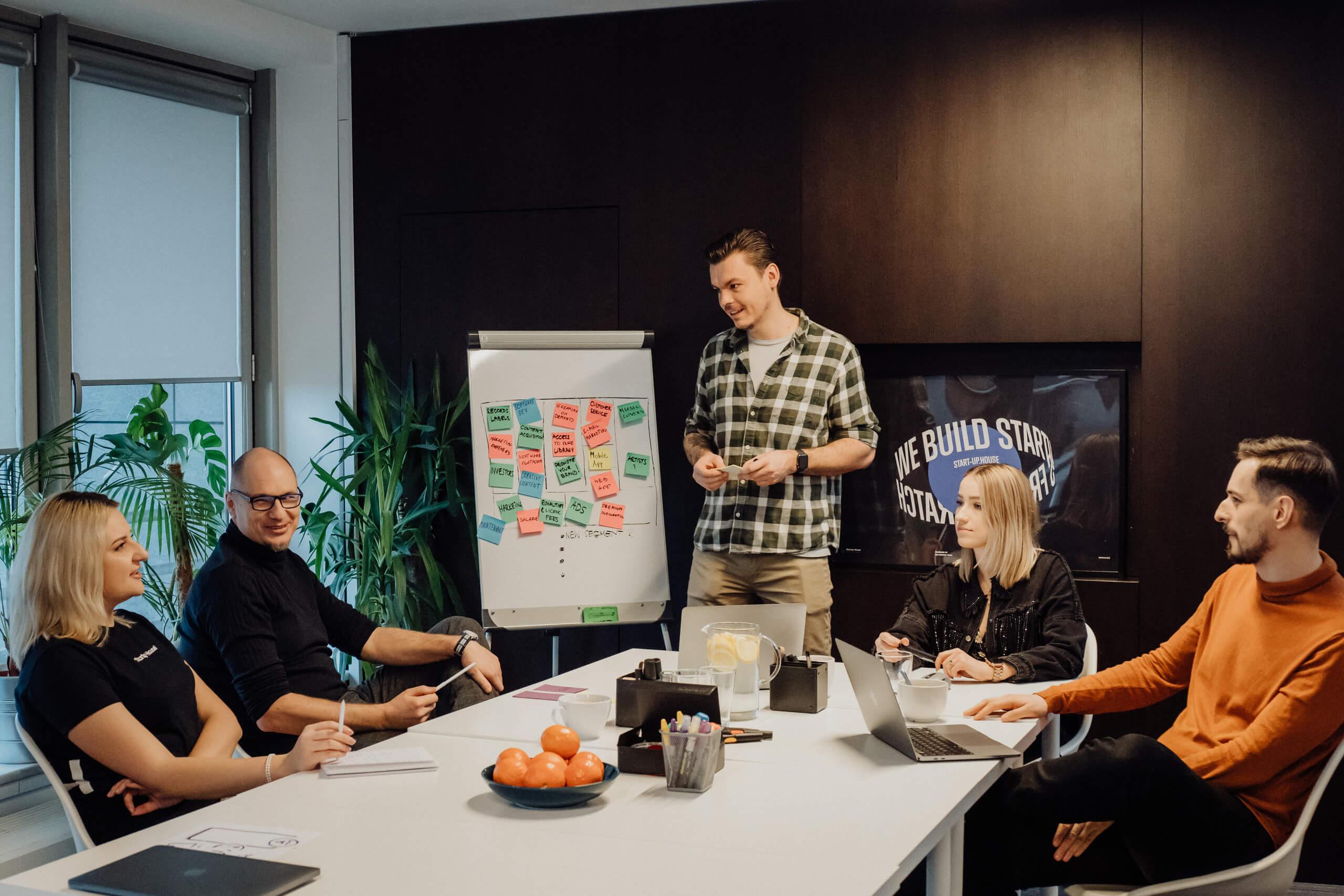
The Innovation Product Life Cycle: Phases and Strategic Insights
Mastering the innovation product life cycle—from ideation to renewal—gives businesses a competitive edge in a fast-evolving market. This guide unpacks each phase and offers strategic insights to help you plan, adapt, and thrive.
Alexander Stasiak
Jun 24, 2025・10 min read
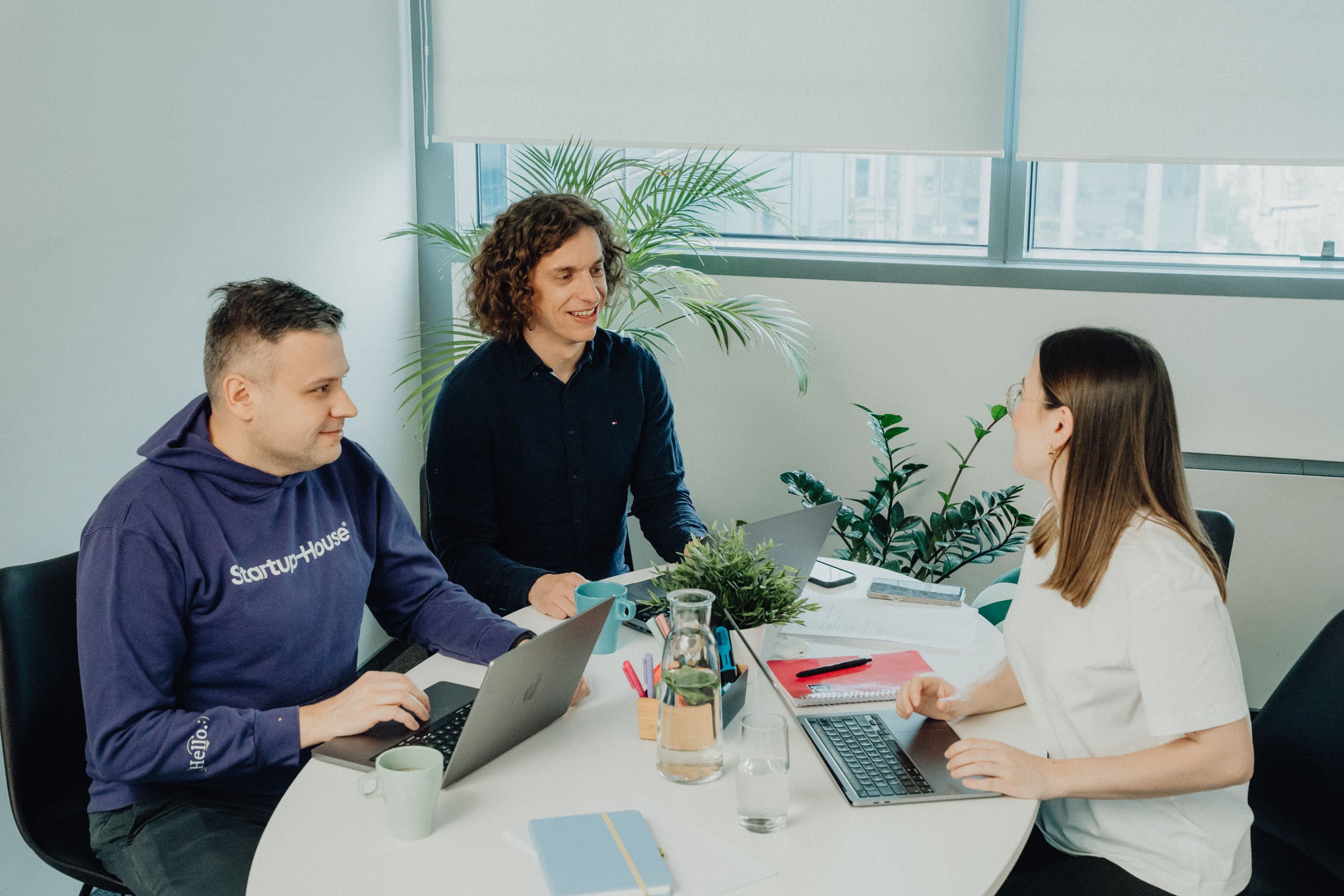
A Practical Guide to Choosing the Right BDD Framework for Your Needs
Choosing the right Behaviour-Driven Development (BDD) framework is key to enhancing collaboration and software quality. This guide explores popular frameworks, selection criteria, and tips for smooth adoption.
Alexander Stasiak
Mar 21, 2024・9 min read