🌍 All
About us
Digitalization
News
Startups
Development
Design
Mastering HTML to PDF Conversion in Node.js: A Simple Guide
Marek Majdak
Jun 03, 2024・5 min read
Table of Content
Introduction to HTML to PDF Conversion
Setting Up Your Node.js Environment
How to Convert HTML to PDF in Node.js
Advanced Techniques and Tips
Conclusion and Next Steps
FAQs
Converting HTML to PDF in Node.js has become an essential skill for developers seeking to create static documents from dynamic web content. Whether you are a novice in programming or an experienced developer, mastering this process can be both practical and rewarding. This guide aims to provide straightforward, step-by-step instructions on how to convert HTML to PDF in Node.js, empowering you to generate professional-looking documents easily and efficiently. By the end of this guide, you will have the confidence to tackle HTML to PDF document conversion in your own projects with ease.
Introduction to HTML to PDF Conversion
Importance of PDF Format
The Portable Document Format (PDF) stands as a universally accepted format, offering numerous advantages in today's digital landscape. Firstly, PDFs preserve the layout font size, and content of a document across all devices and platforms, ensuring consistency and professionalism. This makes them ideal for official documents, reports, and presentations. Secondly, PDFs are highly secure, allowing for password protection and encryption, which helps safeguard sensitive information. Additionally, PDFs support interactive elements like hyperlinks and forms, enhancing their functionality. The format's compatibility with various operating systems and devices further cements its importance. For developers working in Node.js, the ability to convert HTML to PDF is invaluable, as it enables the creation of static, accessible documents from dynamic web content. By understanding the significance of PDFs, developers can utilise this format effectively, ensuring their documents are both visually appealing and secure.
Use Cases in Web Development
In web development, converting an HTML template to PDF serves a variety of practical applications. One common use case is the generation of invoices and receipts. Businesses can automatically generate PDFs from HTML templates, ensuring documents are consistent and professional. Another important application is the creation of reports and data sheets. By converting dynamic web content into static PDFs, developers can provide users with easily shareable and printable documents that maintain their intended format. Additionally, educational websites often utilise HTML to PDF conversion to offer downloadable resources, such as study materials or user manuals. This conversion process is also beneficial for archiving purposes, allowing websites to store content in a format that is not easily altered. By integrating HTML to PDF conversion in their projects, developers can enhance user experience and functionality, providing versatile and reliable document solutions that cater to various needs.
Setting Up Your Node.js Environment
Installing Necessary Packages
Getting started with HTML to PDF conversion in Node.js requires installing essential packages. Begin by ensuring that Node.js and npm (Node Package Manager) are installed on your machine. Open your command line interface and run node -v and npm -v to confirm their installation. Once verified, you'll need specific packages for the conversion process. A popular choice is the puppeteer package, which leverages the Chromium browser to generate PDFs. Install it by running npm install puppeteer in your project directory. Another widely used package is html-pdf, which offers a straightforward interface for converting HTML content. You can install it with npm install html-pdf. Both packages have their own features and strengths, so choose based on your project's requirements. With these packages installed, you'll be equipped to start converting HTML to generate PDF in, setting the groundwork for your development journey in Node.js.
Configuring the Project
Once the necessary packages are installed, the next step is configuring your Node.js project for HTML to PDF conversion. Start by creating a new directory for your project and navigate into it using the command line. Run npm init to initialise a new Node.js project, following the prompts to set up your package.json file. This file will manage your project dependencies and scripts. Next, create a new JavaScript file, for example, index.js, where you'll write your conversion code. In index.js, require the installed packages by adding const puppeteer = require('puppeteer'); or const pdf = require('html-pdf'); at the top of template file, depending on your choice. You should also organise your HTML content, either by embedding it directly within the script or storing it in a separate HTML file. Proper project configuration not only streamlines the development process but also ensures your conversion tasks are efficiently managed and executed.
How to Convert HTML to PDF in Node.js
Implementing Basic Conversion
With your Node.js environment set up, it's time to implement basic HTML to PDF conversion. If you're using the puppeteer package, begin by launching the browser with const browser = await puppeteer.launch();. Then, create a new page using const page = await browser.newPage();. Load your HTML content with await page.setContent(htmlContent);, where htmlContent is your HTML string or file. To generate the PDF, use await page.pdf({ path: 'output.pdf', format: 'A4' });. This command saves the generated PDF as 'output.pdf' in A4 format. Remember to close the browser with await browser.close(); once the conversion is complete. For the html-pdf package, simply call pdf.create(htmlContent).toFile('output.pdf', (err, res) => { ... }); to perform the conversion and handle any errors. These basic steps provide a foundational understanding of how to convert HTML to PDF in Node.js, allowing you to create simple yet effective document conversions.
Handling Styles and Images
Incorporating styles and images into your PDF conversion process is crucial for maintaining the aesthetic integrity of your documents. When using puppeteer, styles can be managed by embedding CSS directly in your HTML or linking to external stylesheets. Ensure that any linked stylesheets are accessible by the browser. Similarly, images should be referenced by absolute URLs or embedded as Base64 strings to ensure they are rendered correctly in the PDF. Use await page.goto('file://' + path.join(__dirname, 'your-html-file.html')) to load HTML files with styles and images. For the html-pdf package, images and styles need to be embedded within the HTML content, as it can struggle with relative paths. Verify that all paths are correct and resources are accessible. By carefully handling styles and images, your PDFs will reflect the intended design and functionality of web page, providing users with a visually consistent and professional document.
Managing Large Documents
When converting large HTML documents to PDF files in Node.js, several considerations can help manage performance and output quality. First, ensure your HTML is well-structured and optimised, minimising unnecessary elements and using efficient CSS. Large documents can strain memory, especially when using packages like puppeteer. To mitigate this, consider splitting the document into smaller sections and converting them individually, then merging the resulting PDFs. Adjusting the page size and margins can also help maintain document clarity. Use the format and margin options in puppeteer or html-pdf to customise these settings. Additionally, keep an eye on image resolutions; high-resolution images can significantly increase file size and processing time. Compress images beforehand to balance quality with performance. By adopting these strategies, you can effectively manage large document conversions in Node.js, ensuring that your PDFs are both efficient to produce and easy to distribute.
Advanced Techniques and Tips
Customising PDF Output
Customising the output of your PDFs allows you to tailor documents to meet specific requirements and preferences. With puppeteer, you have a range of options to control the your PDF file's appearance. For instance, you can modify the page size, orientation, and margins using the pdf() method's options like format, landscape, and margin. Additionally, you can add headers and footers by specifying displayHeaderFooter: true, then customising the content via headerTemplate and footerTemplate. For dynamic content such as page numbers, JavaScript expressions within these templates can be utilised. Meanwhile, the html-pdf package provides similar customisation through its options object, where you can set configurations for page dimensions and styles. By leveraging these customisation features, you can create PDFs that not only look professional but also align with your branding or client specifications, delivering a polished end product every time.
Enhancing Performance
Optimising performance during HTML to PDF conversion in Node.js is crucial, especially when dealing with large-scale applications or high volumes of data. One effective strategy is to manage resource loading efficiently. For instance, disable unnecessary JavaScript and images if they are not required for PDF generation, using the page.setRequestInterception(true) and filtering requests in puppeteer. This can significantly reduce load times. Additionally, running headless Chromium instances in batches can prevent file system call overload and improve processing speed. Consider increasing the concurrency of your Node.js application by utilising asynchronous operations and promises, which can help handle multiple conversion requests simultaneously. Moreover, optimising your HTML and CSS by minimising code and using lightweight frameworks can enhance loading and rendering speed. By applying these techniques, you can improve the performance of your conversion processes, ensuring that your application remains responsive and efficient under various conditions.
Debugging Common Issues
When converting HTML pages to PDF in Node.js, developers might encounter several common issues. A frequent problem is missing styles or images in the output PDF. This often arises from incorrect file paths or issues with network resources. Double-check that all CSS files and images are correctly linked and accessible. Another issue is incorrect page formatting, which can usually be resolved by verifying PDF dimension settings and ensuring the HTML structure is well-defined. If the conversion process fails entirely, inspect the Node.js console for error messages and review your code for syntax errors or misconfigurations. Additionally, enabling debug mode in packages like puppeteer by setting the environment variable DEBUG to puppeteer:* can provide insightful logs to pinpoint issues. Addressing these common problems methodically can streamline the debugging process, allowing you to generate accurate and visually pleasing PDFs with greater consistency.
Conclusion and Next Steps
Recap of Key Points
In this guide, we've covered the essentials of how to convert HTML to PDF in Node.js, equipping you with the skills needed to create professional documents from dynamic content. We began by understanding the importance and use cases of PDF format in web development. Then, we set up the Node.js environment by installing necessary packages and configuring the project. We moved on to implementing basic conversion techniques and handling styles and images to ensure the visual integrity of your PDFs. For more advanced needs, we discussed managing large documents, customising generating PDFs output, enhancing performance, and debugging common issues. Each step is aimed at providing you with practical knowledge to tackle HTML to PDF conversion confidently. With these foundational and advanced techniques, you are now well-prepared to integrate robust PDF generation into your Node.js projects, enhancing both functionality and user experience.
Further Reading and Resources
To deepen your knowledge of HTML to PDF conversion in Node.js, several resources can be particularly beneficial. The official documentation for puppeteer and html-pdf provides comprehensive guides and examples that can help you understand advanced features and troubleshooting techniques. Websites like MDN Web Docs and W3Schools offer valuable insights into HTML and CSS, which are foundational for web pages and creating well-structured documents. Additionally, community forums such as Stack Overflow and GitHub Discussions are excellent platforms for seeking advice and sharing solutions with other developers. For those who prefer interactive learning, online courses on platforms like Udemy and Coursera can provide structured learning paths and practical exercises. Lastly, exploring blogs and tutorials from experienced developers can offer real-world applications and tips. By leveraging these resources, you can continually enhance your skills and stay updated with the latest techniques in HTML to PDF conversion in Node.js.
FAQs
What is the best package for converting HTML to PDF in Node.js?
The best packages for converting HTML to PDF in Node.js are Puppeteer and html-pdf. Puppeteer is known for its Chromium-based rendering, while html-pdf offers a simpler interface for basic tasks.
How do I install Puppeteer in Node.js?
To install Puppeteer, run npm install puppeteer in your Node.js project directory. This will download and configure Puppeteer for use in your application.
What are the use cases for HTML to PDF conversion in Node.js?
Common use cases include generating invoices, reports, data sheets, and downloadable resources like study materials. It is also useful for archiving web content.
How do I convert HTML to PDF using Puppeteer?
To convert HTML to PDF using Puppeteer, first install it, then write a script that launches Puppeteer, opens a new page, sets the HTML content, and generates a PDF with the page.pdf() method.
How do I manage styles and images in HTML to PDF conversion?
In Puppeteer, ensure that your styles are either inline or linked with accessible URLs, and images are either absolute paths or Base64 encoded to render correctly in the PDF.
How can I handle large HTML files when converting to PDF in Node.js?
For large documents, split the HTML into smaller sections or optimise the content and resources. Compress images and simplify the HTML structure to improve performance during conversion.
Can I customise the PDF output in Puppeteer?
Yes, Puppeteer allows extensive customisation of the PDF output. You can set page size, orientation, margins, headers, footers, and even include page numbers using JavaScript templates.
How do I troubleshoot missing styles or images in my PDF?
Check that the paths to CSS files and images are correct and accessible. Ensure that the resources are fully loaded before starting the PDF conversion process.
What is the difference between Puppeteer and html-pdf for HTML to PDF conversion?
Puppeteer uses a Chromium rendering engine and supports more advanced features like JavaScript-heavy pages. html-pdf is simpler and better suited for basic conversion needs without dynamic content.
How do I improve the performance of HTML to PDF conversion in Node.js?
Disable unnecessary resources like JavaScript and images that are not required for the PDF output. Use asynchronous functions and split large tasks into smaller, manageable operations.
Can I automate HTML to PDF conversion in Node.js?
Yes, you can automate the process by running the conversion scripts in scheduled tasks or using background services. This is ideal for generating recurring documents like reports or invoices.
How do I add page numbers to a PDF using Puppeteer?
To add page numbers in Puppeteer, use the displayHeaderFooter: true option in the pdf() method and define a footerTemplate with JavaScript for dynamic page numbering.
How do I convert dynamic HTML content to PDF in Node.js?
Puppeteer is ideal for converting dynamic content like JavaScript-heavy web pages. It fully renders the page, including dynamic elements, before generating the PDF.
What security measures can I implement when converting HTML to PDF?
You can add security measures such as password protection and encryption to the generated PDFs using additional tools or libraries integrated with Node.js.
Is it possible to convert HTML to PDF from a URL in Node.js?
Yes, both Puppeteer and html-pdf allow you to load a webpage from a URL and convert it to PDF by fetching the page content and applying the conversion methods.
Can I generate PDFs with headers and footers in Node.js?
Yes, in Puppeteer, you can add custom headers and footers by enabling displayHeaderFooter and using headerTemplate and footerTemplate for personalised content.
What is the advantage of using Node.js for HTML to PDF conversion?
Node.js allows real-time handling of web pages, making it ideal for converting dynamic, server-rendered HTML content to PDFs. It also supports asynchronous operations, improving performance.
How do I handle errors in HTML to PDF conversion in Node.js?
Use error handling techniques like try-catch blocks in your conversion scripts. Enable debugging in Puppeteer or html-pdf for more detailed error messages and logs.
How can I compress images for HTML to PDF conversion?
Before converting, compress images using tools like TinyPNG or image compression libraries to reduce file size without sacrificing quality. This improves performance during PDF generation.
How do I handle timeouts or slow conversion in Puppeteer?
To manage timeouts, increase the timeout parameter in Puppeteer settings. For slow conversions, optimise HTML, disable unnecessary resources, or process documents in smaller batches.
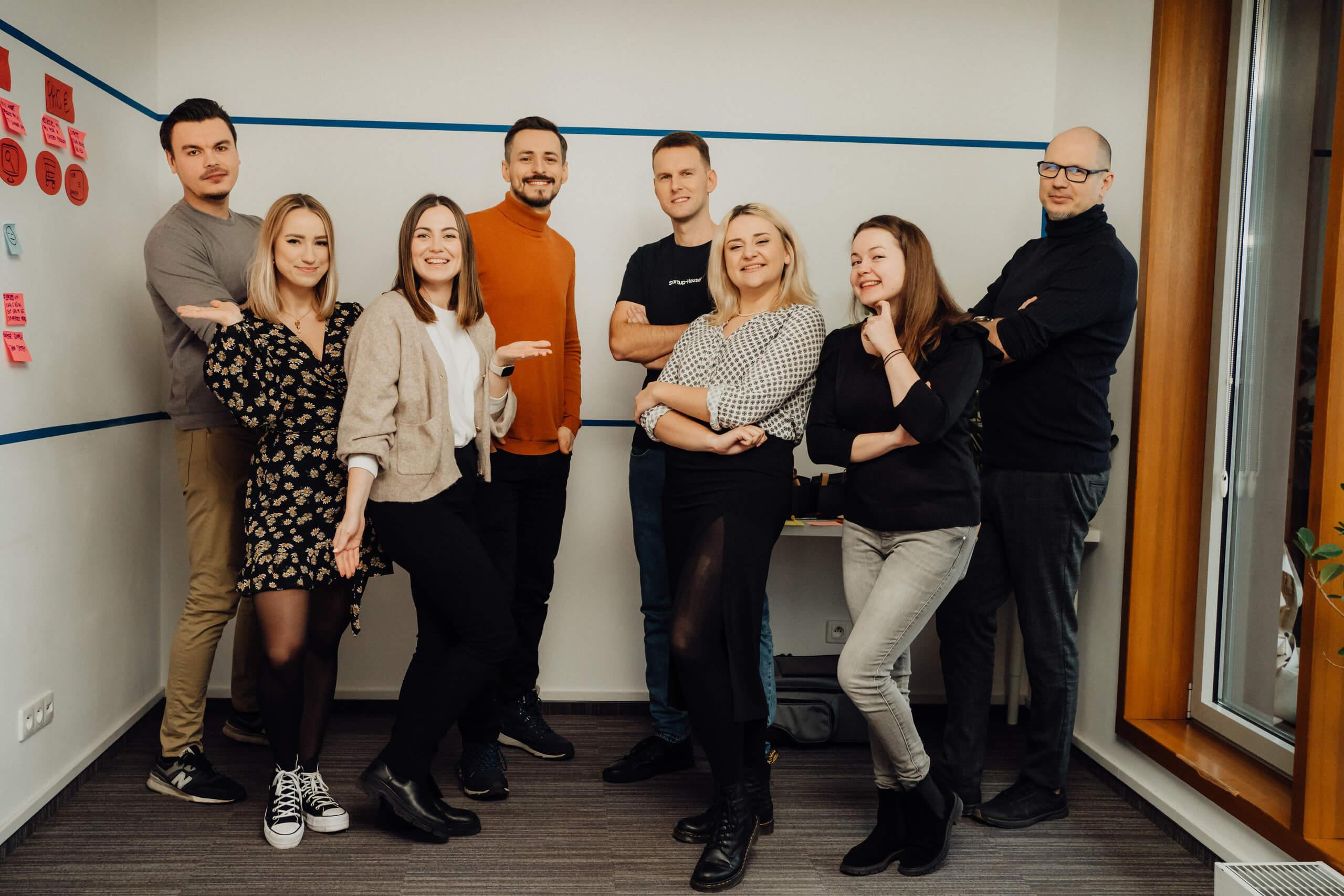
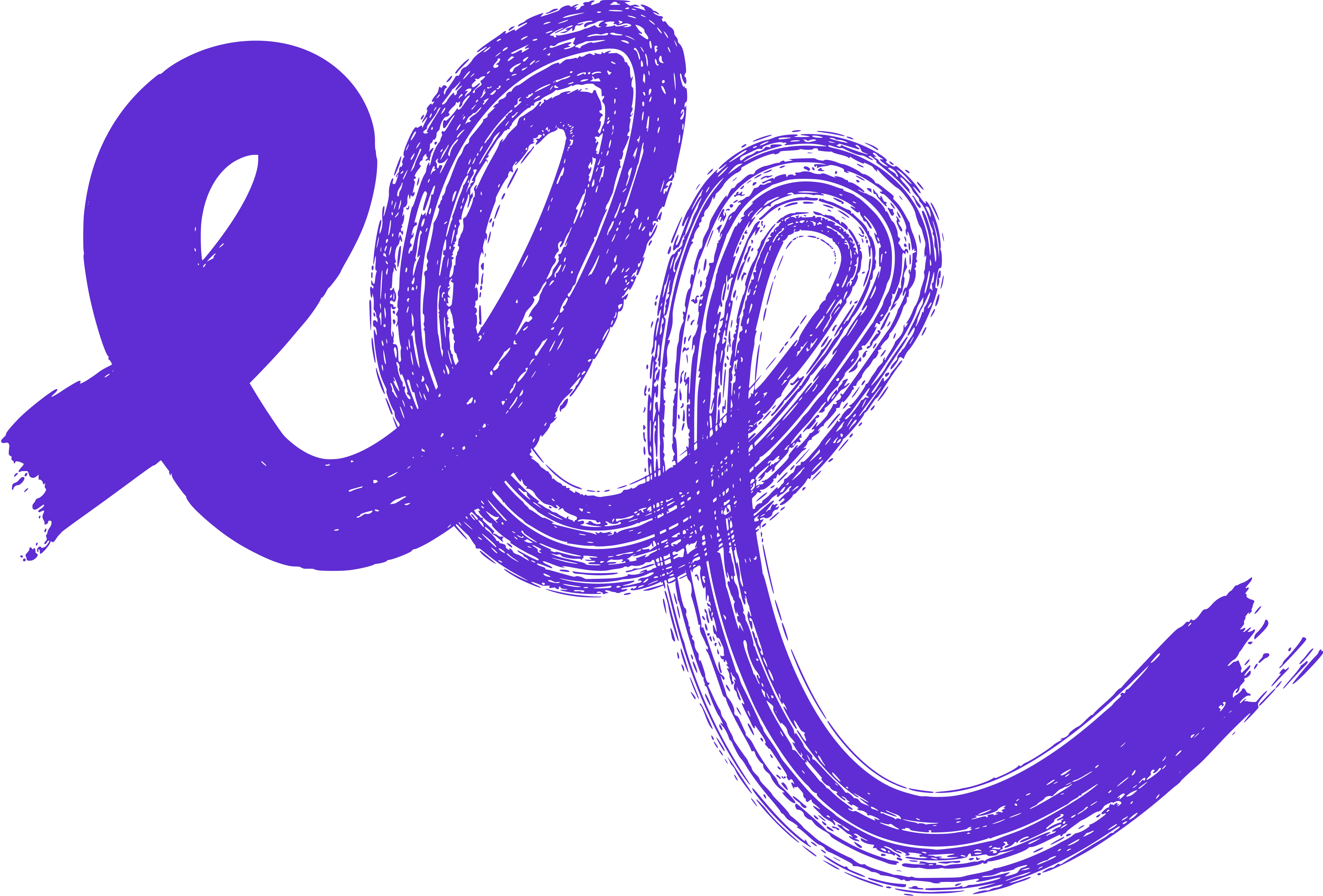
You may also
like...
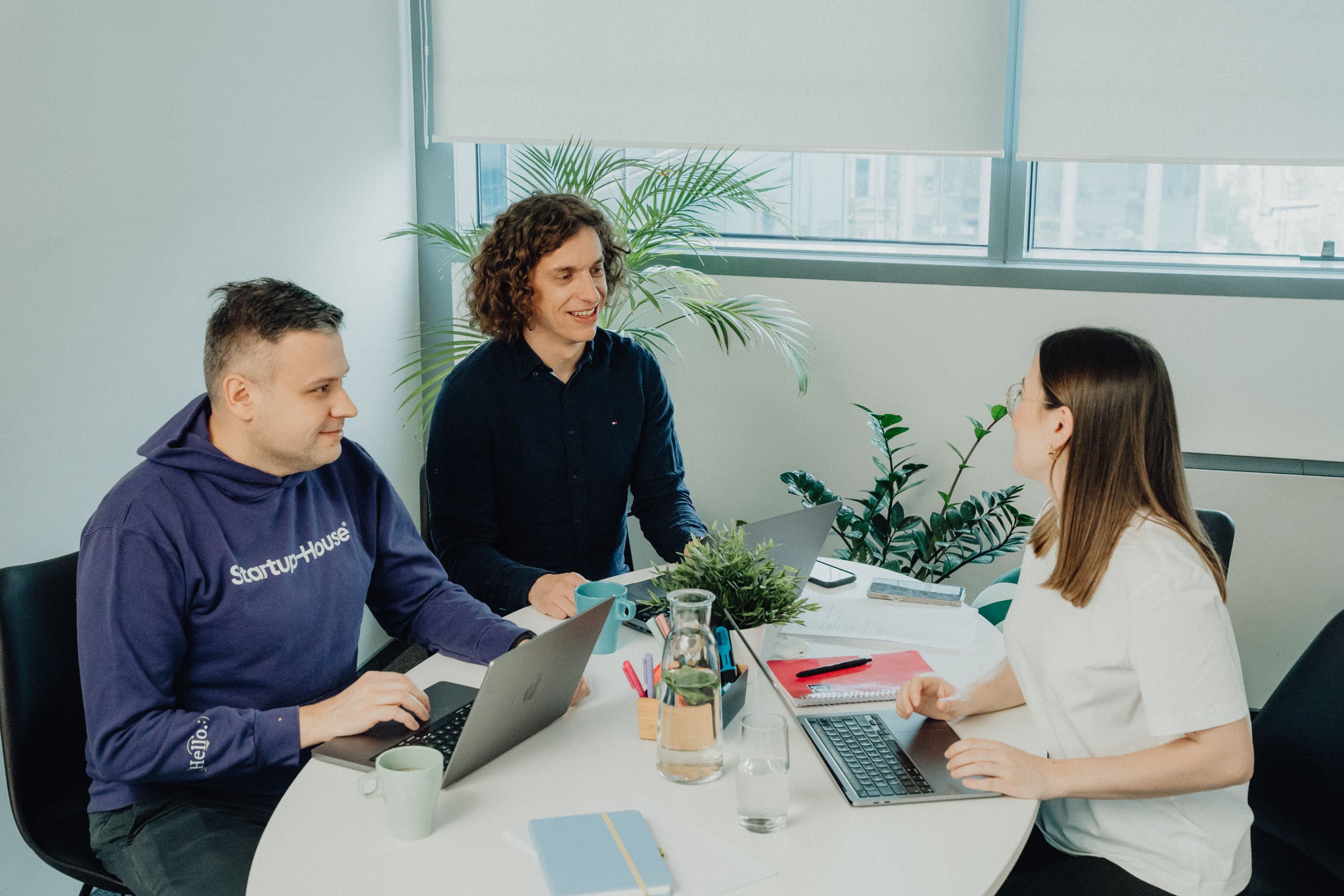
A Practical Guide to Choosing the Right BDD Framework for Your Needs
Choosing the right Behaviour-Driven Development (BDD) framework is key to enhancing collaboration and software quality. This guide explores popular frameworks, selection criteria, and tips for smooth adoption.
Alexander Stasiak
Mar 21, 2024・9 min read
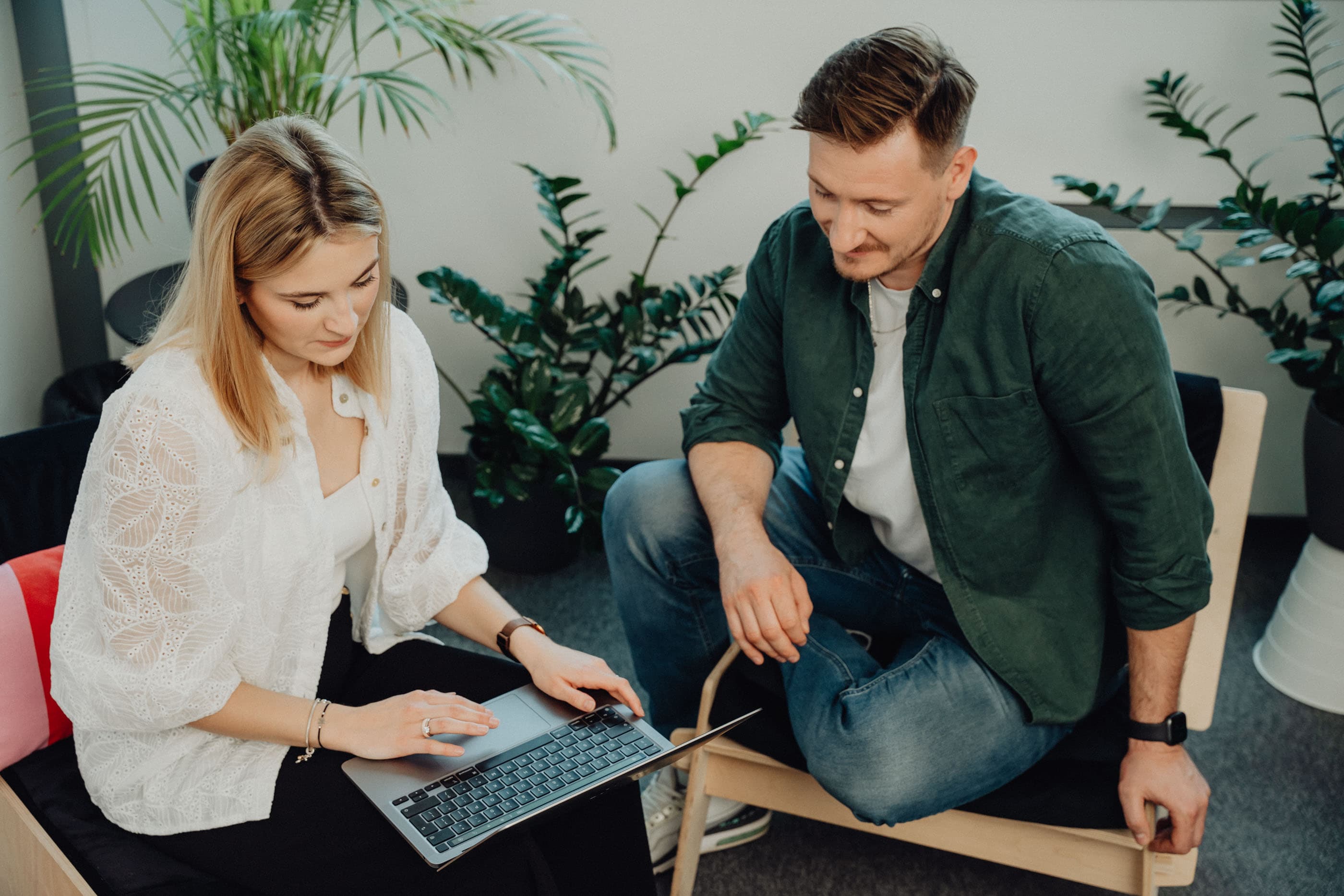
Understanding the Distinct Roles: Scrum Master vs Product Owner
Scrum Master and Product Owner roles are integral to Agile projects but serve different purposes. This guide explains their distinct responsibilities, skills, and collaborative dynamics.
Marek Pałys
Dec 09, 2024・8 min read
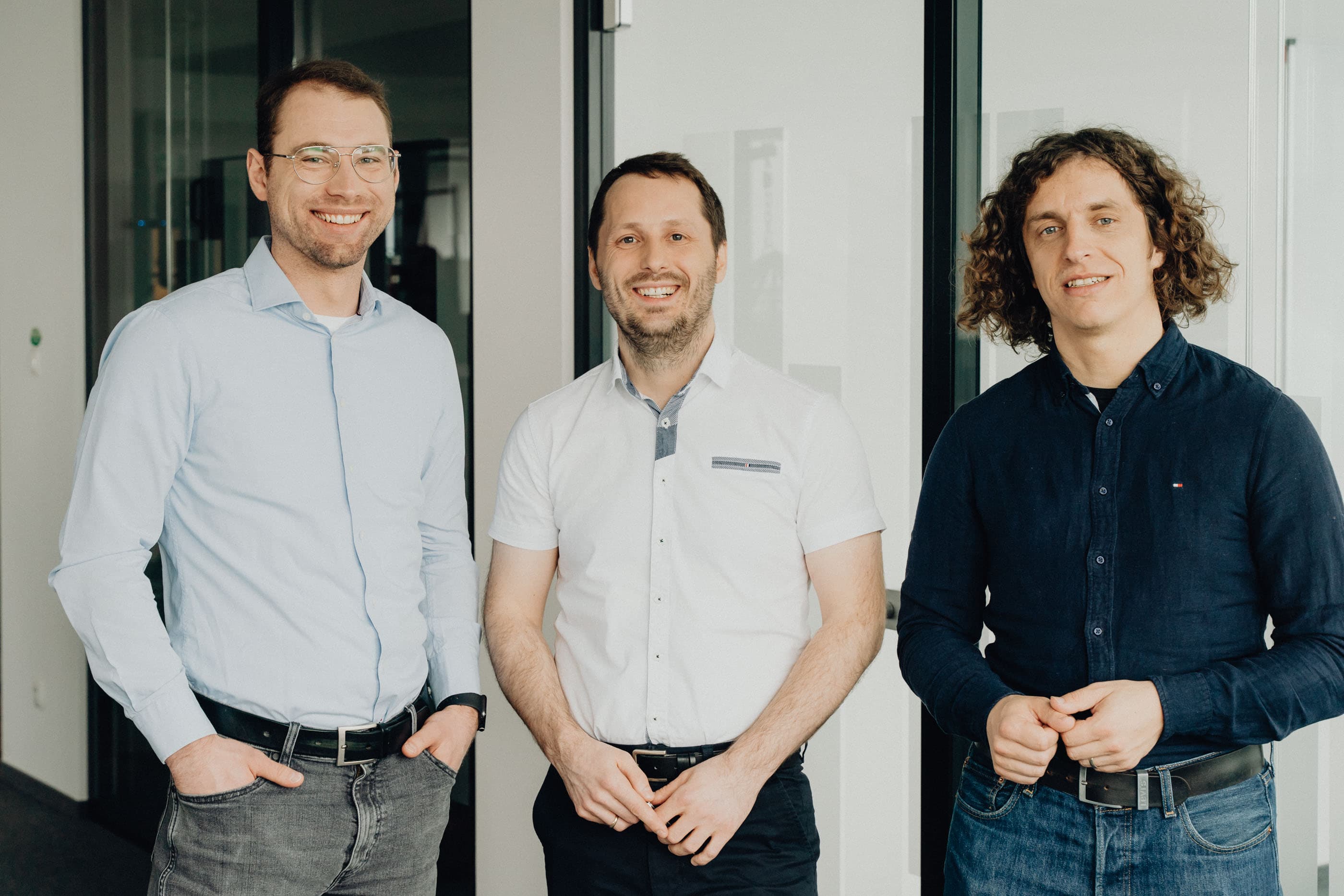
Private vs Public Cloud: A Clear Guide to Making the Right Choice for Your Business
Discover the key differences between private and public clouds to make an informed choice for your business. This guide explains their benefits, cost implications, security, and performance to help you find the ideal cloud solution.
Marek Majdak
Sep 17, 2024・9 min read