🌍 All
About us
Digitalization
News
Startups
Development
Design
Demystifying the Knockout Framework: A Simple Guide for All Levels
Marek Majdak
Sep 17, 2024・8 min read
Table of Content
Demystifying the Knockout Framework: A Simple Guide for All Levels
Understanding the Basics
Setting Up Your Environment
Core Concepts Explained
Advanced Techniques and Tips
Real-World Applications
Demystifying the Knockout Framework: A Simple Guide for All Levels
The Knockout framework is a powerful tool designed to simplify the process of building dynamic web applications. Known for its ability to bind HTML elements to JavaScript models effortlessly, it allows developers to create rich, responsive user interfaces with ease. Whether you are a seasoned developer or just starting out, understanding the core principles of Knockout can significantly enhance your programming toolkit. In this guide, we will break down the essentials of the Knockout web framework itself, providing a clear and practical approach to leveraging its capabilities. By the end, you'll be equipped with the knowledge to implement this framework in your projects confidently.
Understanding the Basics
What is the Knockout Framework?
The Knockout framework is a JavaScript library that helps developers create dynamic and interactive web applications. It uses a Model-View-ViewModel (MVVM) pattern, which is instrumental in separating concerns within an application. This separation allows developers to manage complex interfaces more efficiently. The primary feature of Knockout is its declarative bindings, which allow you to connect your HTML elements directly to your data model. This connection means that any changes in your data or view model automatically reflect in the user interface without the need for additional code. By leveraging observables and dependency tracking, Knockout ensures that your UI remains in sync with your underlying data, providing a seamless and responsive user experience. This makes it an invaluable tool for modern web development.
Key Features to Know
Knockout offers several key features that make it a preferred choice for many developers. First, its declarative bindings allow you to associate DOM elements with model data seamlessly, reducing the need for manual updates. This makes your code cleaner and more manageable. Second, Knockout's observables are crucial. They enable automatic data updates in the UI whenever the underlying model data changes. This ensures that your application remains consistent and responsive. Third, Knockout's dependency tracking intelligently updates only those parts of the UI that are affected by a change, enhancing performance. Finally, Knockout's templating capabilities allow for dynamic rendering of data-bound templates, making it easier to handle complex data presentations. Together, these features provide a robust framework for building scalable, maintainable web applications. Understanding these features is essential for leveraging Knockout effectively in your projects.
Why Choose Knockout?
Choosing Knockout as your framework offers several advantages, particularly for those looking to build feature-rich web applications. For starters, its simplicity and ease of use make it an excellent option for developers at all levels. The framework's clear syntax and minimal setup requirements mean you can get up and running swiftly. Additionally, Knockout excels in creating responsive user interfaces. Its automatic UI updates, driven by observables, ensure that any change in your data model's state is instantly reflected on the screen, providing a seamless experience for users. Furthermore, Knockout is highly flexible and can be easily integrated with other libraries and frameworks, allowing you to tailor your development environment to your specific needs. Finally, its open-source nature and active community mean there's plenty of support and resources available for troubleshooting and learning. These benefits make Knockout a compelling choice for modern web development.
Setting Up Your Environment
Installation Steps
Installing Knockout is a straightforward process that can be accomplished in a few simple steps. First, you need to download the Knockout library. This can be done by visiting the official Knockout website and downloading the latest version. Alternatively, you can include Knockout in your project via a content delivery network (CDN). Simply add the <script> tag referencing the CDN link in your HTML file. This method ensures you always have the latest version without manual updates. If you are using package managers like npm, you can install Knockout by running the command npm install knockout in your terminal. Once installed, you can start using Knockout by including the necessary <script> tag in your HTML file or by requiring it in your JavaScript file. With these steps, you’re ready to begin building dynamic applications with the Knockout framework.
Essential Tools and Resources
To maximise your efficiency with the Knockout framework, it's beneficial to have a set of essential tools and resources at your disposal. Firstly, an integrated development environment (IDE) such as Visual Studio Code or WebStorm can significantly streamline your coding process with features like syntax highlighting and error detection. Secondly, browser developer tools, especially those in Chrome or Firefox, are indispensable for debugging and inspecting your application in real-time. Additionally, Knockout's official documentation is a crucial resource, offering detailed explanations and examples that cover a wide array of use cases. For community support and more nuanced queries, platforms like Stack Overflow provide a wealth of shared knowledge and troubleshooting tips. Lastly, consider exploring online tutorials and courses that delve into advanced Knockout topics to further enhance your understanding. Together, these tools and resources form a comprehensive support system for developing robust applications with Knockout.
Troubleshooting Common Issues
When working with the Knockout framework, it's not uncommon to encounter a few hiccups. One frequent issue is data not updating in the UI. This often stems from forgetting to declare a property as an observable, which is necessary for automatic UI updates. Ensure your data properties are set up correctly as ko.observable. Another common problem is with bindings not working as expected, which can result from syntax errors in your data-bind attribute. Double-check your bindings for typos or incorrect syntax. Additionally, ensure your Knockout library is correctly linked in your HTML file. A missing or incorrect script reference can prevent Knockout from functioning altogether. If the problem persists, use browser developer tools to inspect and debug your application. Console logs can provide valuable insights into what's going wrong. By systematically checking these areas, you can resolve most common issues and ensure your Knockout application runs smoothly.
Core Concepts Explained
Observables and Bindings
Observables and bindings are fundamental concepts in the Knockout framework. Observables are special JavaScript objects that allow you to track changes in data and automatically update the UI. You create an observable by using the ko.observable function. For example, var myObservable = ko.observable('initial value');. When the value of myObservable changes, any UI element bound to it will automatically reflect the new value.
Bindings, on the other hand, are used to connect your HTML elements to your observables. The data-bind attribute in HTML is where you specify these bindings. For instance, data-bind="text: myObservable" will ensure that the text content of an element is always in sync with myObservable. There are different types of bindings such as text, value, visible, and foreach, each serving specific purposes. Mastering observables and bindings is crucial for creating dynamic, responsive applications with Knockout.
Dependency Tracking
Dependency tracking is a powerful feature of the Knockout framework that ensures your user interface stays in sync with the underlying data model. It works by automatically identifying which observables a computed observable depends on. Whenever any of these observables change, the computed observable is recalculated, and the UI updates accordingly. This mechanism removes the need for manual tracking of dependencies, simplifying the development process.
For example, consider a scenario where you have a list of items and you want to compute the total price. By using a computed observable, Knockout automatically tracks each item's price observable. If any item's price changes, the total price recalculates without extra coding. This automatic management of dependencies leads to cleaner code and reduces the likelihood of errors. Understanding dependency tracking is essential for leveraging the full potential of Knockout, as it plays a critical role in maintaining a responsive and efficient application.
Using Computed Observables
Computed observables in Knockout are a vital tool for creating dynamic calculations based on other observables. They function like a combination of a function and an observable, automatically recalculating whenever their dependencies change. To create a computed observable, you use the ko.computed function, passing in a function that contains your logic. For instance, if you have observables for quantity and price, a computed observable can calculate the total cost.
var totalCost = ko.computed(function() { return this.quantity() * this.price(); }, this);
Here, totalCost will automatically update whenever quantity or price changes, ensuring the UI is always displaying accurate information. This feature is particularly useful when you need to maintain a clean separation between your full model view view model and view, as it reduces the need to manipulate DOM directly. By understanding and utilising computed observables, you can build more efficient and maintainable applications with Knockout.
Advanced Techniques and Tips
Custom Bindings
Custom bindings in Knockout allow you to create your own binding handlers, extending the framework's capabilities to meet specific needs of given project. This is particularly useful when you need functionality not covered by Knockout's built-in bindings. To create a custom binding, you define a new handler in ko.bindingHandlers. Each handler can include init and update functions, which determine how the binding should behave during different stages of the DOM element lifecycle.
For example, you might create a custom binding to format a date:
ko.bindingHandlers.dateString = { update: function(element, valueAccessor) { var value = ko.unwrap(valueAccessor()); var formattedDate = new Date(value).toLocaleDateString(); element.innerText = formattedDate; } };
In this example, the dateString binding takes a date value and formats it for display. By employing custom bindings, you can encapsulate complex logic within a single, reusable binding, simplifying your HTML and enhancing code clarity. This approach is a powerful way to tailor Knockout to suit unique application requirements.
Extending Functionality
Extending functionality in Knockout involves enhancing or adding new features to the framework to better fit your application needs. This can be done through custom bindings, plugins, or modifying the existing framework. One common method is to create custom functions that extend observables or computed observables, allowing them to perform additional operations. This can help encapsulate repeated logic and keep your code DRY (Don't Repeat Yourself).
For instance, you might extend an observable with a custom function that validates user input before updating its value. This ensures consistent data integrity across your application. Another way to extend functionality is by integrating third-party libraries or plugins that complement Knockout's capabilities, like adding rich UI components or enhancing performance.
By carefully extending the framework, you can tailor Knockout to meet specific requirements, improve efficiency, and maintain the adaptability of your application, all while keeping your source codebase organised and maintainable.
Performance Optimisation
Optimising performance in Knockout applications is crucial for ensuring a smooth user experience, especially as your application scales. One effective strategy is to minimise the number of observables in your ViewModel. Each observable introduces overhead for dependency tracking, so using plain JavaScript objects where reactivity is unnecessary can improve performance. Similarly, be judicious with computed observables, ensuring they are only recalculated when absolutely necessary.
Another approach is to limit DOM updates. Using Knockout's deferred updates feature can batch multiple updates into a single UI refresh cycle, reducing unnecessary redraws. Additionally, for large data sets, consider using virtualisation techniques to render only the visible items in a list, greatly enhancing responsiveness.
Lastly, profiling and monitoring tools can help identify bottlenecks within your application. Tools like browser developer tools or specific Knockout extensions can provide insights into rendering times and update cycles, allowing you to pinpoint and address performance issues effectively. These optimisations ensure your Knockout application remains efficient and responsive.
Real-World Applications
Practical Use Cases
Knockout is widely used in various practical applications due to its ability to manage complex user interfaces efficiently. One common use case is in form-heavy applications, such as those found in customer relationship management (CRM) systems, where real-time data validation and dynamic updates are crucial. Knockout's observables and bindings ensure that data is always up-to-date, providing immediate feedback to users.
Another example is in e-commerce platforms, where Knockout can manage shopping cart interactions, dynamically updating item quantities and totals as users interact with the site. This real-time responsiveness enhances the shopping experience by providing instantaneous updates without page reloads.
Additionally, Knockout is useful in dashboard applications, where it can handle the dynamic rendering of data visualisations and reports. Here, its dependency tracking ensures that visual components are automatically refreshed as underlying data changes. These use cases demonstrate Knockout's versatility and effectiveness in building responsive, interactive web applications.
Integrating with Other Technologies
Knockout's design allows for seamless integration with a wide range of other technologies, making it a flexible choice for various development environments. For instance, it can be paired with server-side technologies like ASP.NET or Node.js to have other frameworks handle data management and business logic, while Knockout manages the client-side user interface. This separation of concerns helps streamline development and maintainability.
Moreover, Knockout can be integrated with front-end frameworks such as Bootstrap, providing a polished, responsive design to complement its dynamic capabilities. It also works well alongside other JavaScript libraries, like jQuery, for DOM manipulations and Ajax requests, enhancing the interactivity of web applications.
Additionally, integrating Knockout with RESTful APIs allows developers to create single-page applications that fetch and update data asynchronously, providing a smoother user experience. This compatibility with various technologies enables developers to leverage Knockout's strengths while benefiting from the unique advantages of other tools in a new version of their tech stack.
Success Stories and Examples
Many organisations have successfully leveraged Knockout to have webflow developers build robust web applications, showcasing its versatility and effectiveness. For example, Microsoft adopted Knockout in their Hotmail and Outlook.com projects to manage complex, data-driven interfaces, enabling real-time email updates and notifications. The framework's ability to handle dynamic content efficiently contributed significantly to the user experience.
Another success story is the online learning platform Pluralsight, which uses Knockout to have designers create interactive course interfaces. The platform benefits from Knockout's seamless data binding and reactivity, allowing learners to engage with course materials dynamically and receive instant feedback on exercises.
Additionally, the financial services firm Morgan Stanley has utilised Knockout to build internal applications that require real-time data updates and complex user interactions. The framework's observables and computed observables help maintain data consistency and responsiveness, which are critical in financial applications.
These examples demonstrate Knockout's capability to support diverse and demanding applications, making it a reliable choice for modern web development.
FAQs
What is the Knockout framework? Knockout is a JavaScript library that helps build dynamic web applications using a Model-View-ViewModel (MVVM) architecture.
How does Knockout simplify data binding? Knockout uses declarative bindings to connect HTML elements with JavaScript models, automating UI updates.
What are observables in Knockout? Observables are objects that track changes in data and automatically update the UI when data changes.
How does Knockout's dependency tracking work? Dependency tracking automatically updates only the parts of the UI affected by data changes, improving performance.
Can Knockout handle large-scale applications? Yes, Knockout can manage complex interfaces and data structures, making it suitable for large-scale applications.
What are computed observables in Knockout? Computed observables dynamically calculate values based on other observables and automatically update when dependencies change.
What are Knockout custom bindings? Custom bindings allow developers to create their own handlers to extend Knockout’s default functionality.
How does Knockout ensure responsive UI updates? Knockout ensures responsive updates through observables and bindings, automatically syncing UI with underlying data models.
Can Knockout integrate with other frameworks? Yes, Knockout integrates easily with other JavaScript libraries and frameworks, enhancing its flexibility.
How do you install Knockout? You can install Knockout by downloading the library from its official website or via a CDN or npm.
Is Knockout compatible with modern browsers? Yes, Knockout is compatible with all mainstream browsers, ensuring wide usability.
Can Knockout be used in single-page applications (SPAs)? Yes, Knockout is highly effective in building responsive single-page applications due to its dynamic data binding.
What are the advantages of Knockout over other frameworks? Knockout offers simplicity, seamless data binding, and ease of integration, making it a developer-friendly choice.
How does Knockout manage complex data models? Knockout uses observables and dependency tracking to efficiently manage and synchronize complex data models.
What are the best practices for using Knockout? Best practices include limiting the number of observables, using deferred updates, and optimizing dependency tracking for performance.
Can Knockout work with third-party plugins? Yes, Knockout can integrate with third-party plugins to extend its functionality and enhance UI components.
How does Knockout improve development speed? Knockout simplifies code structure and automates data binding, which reduces the amount of manual coding required.
Is Knockout open-source? Yes, Knockout is open-source and has an active community that provides resources and support.
Can Knockout be used for real-time data applications? Yes, Knockout is effective for real-time data applications, keeping the UI updated without reloading the page.
Does Knockout support mobile web development? Yes, Knockout can be used to build responsive web applications that work seamlessly across mobile devices.
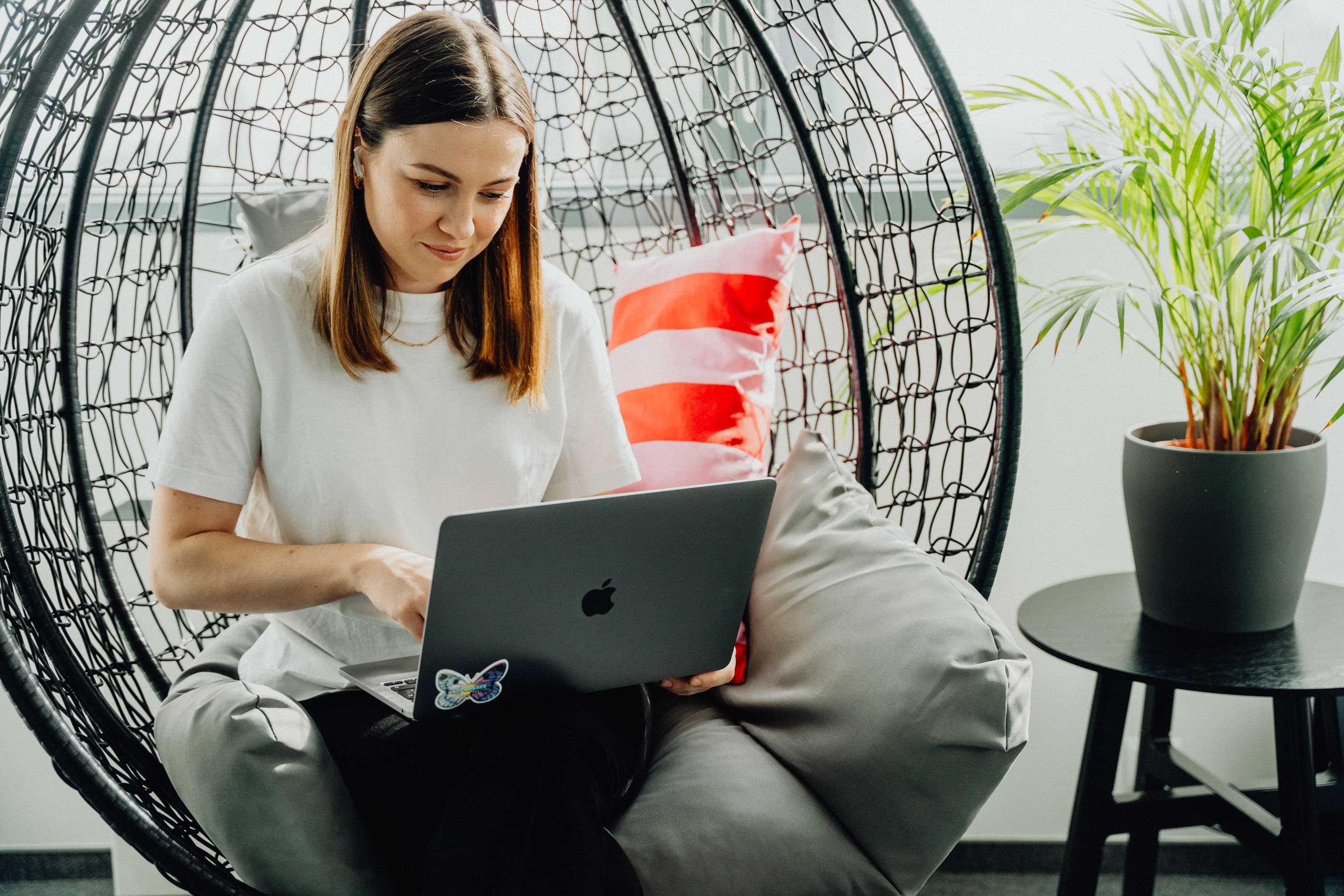
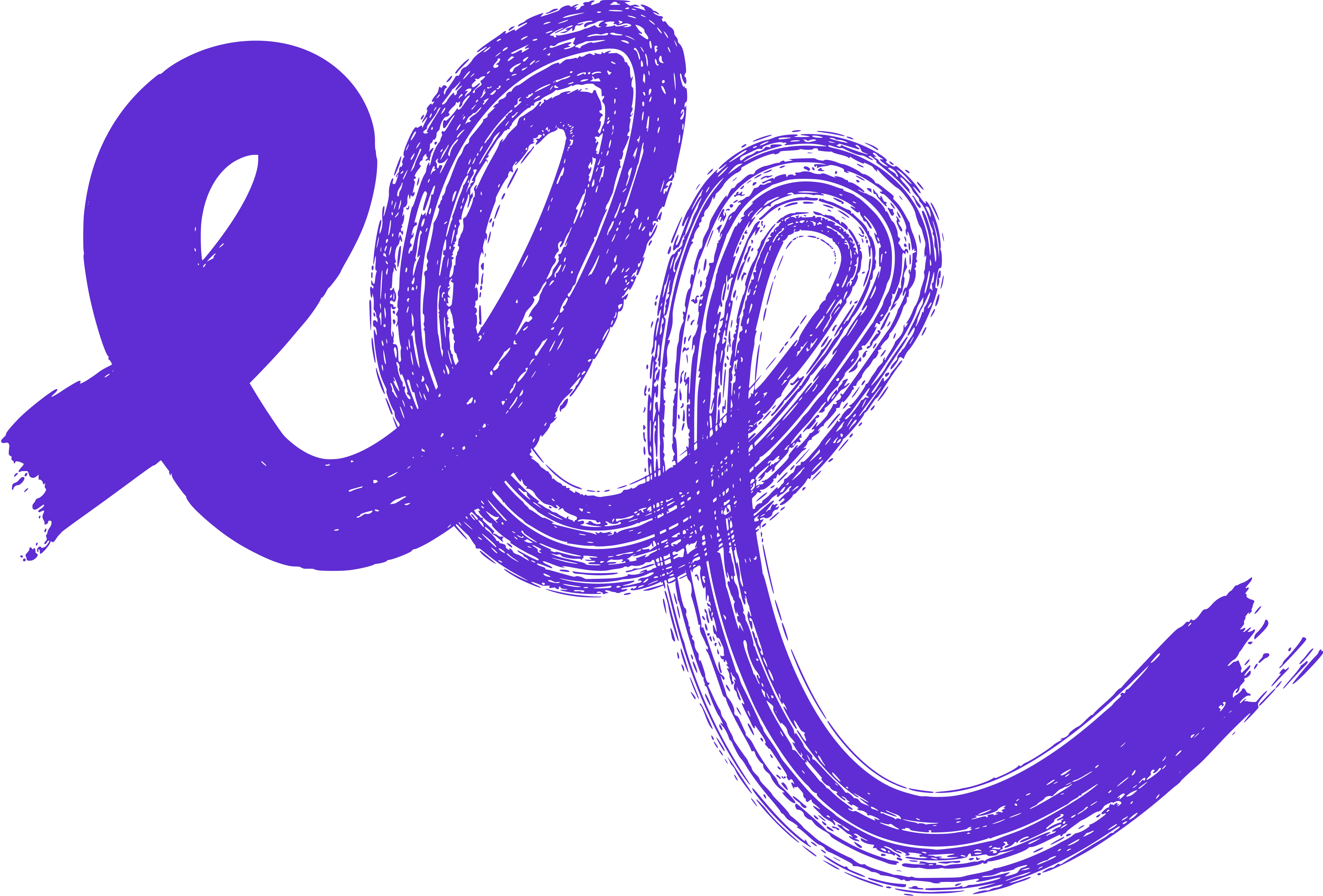
You may also
like...

Understanding Why Your App Rendered More Hooks Than During the Previous Render
This article explores the reasons why your application might render more hooks than during the previous render, a common issue faced by developers. By understanding the mechanics of React hooks and their rendering process, you'll gain practical insights to diagnose and resolve this problem. Enhancing your app's performance and stability is crucial for delivering a seamless user experience.
Marek Majdak
Apr 29, 2024・12 min read
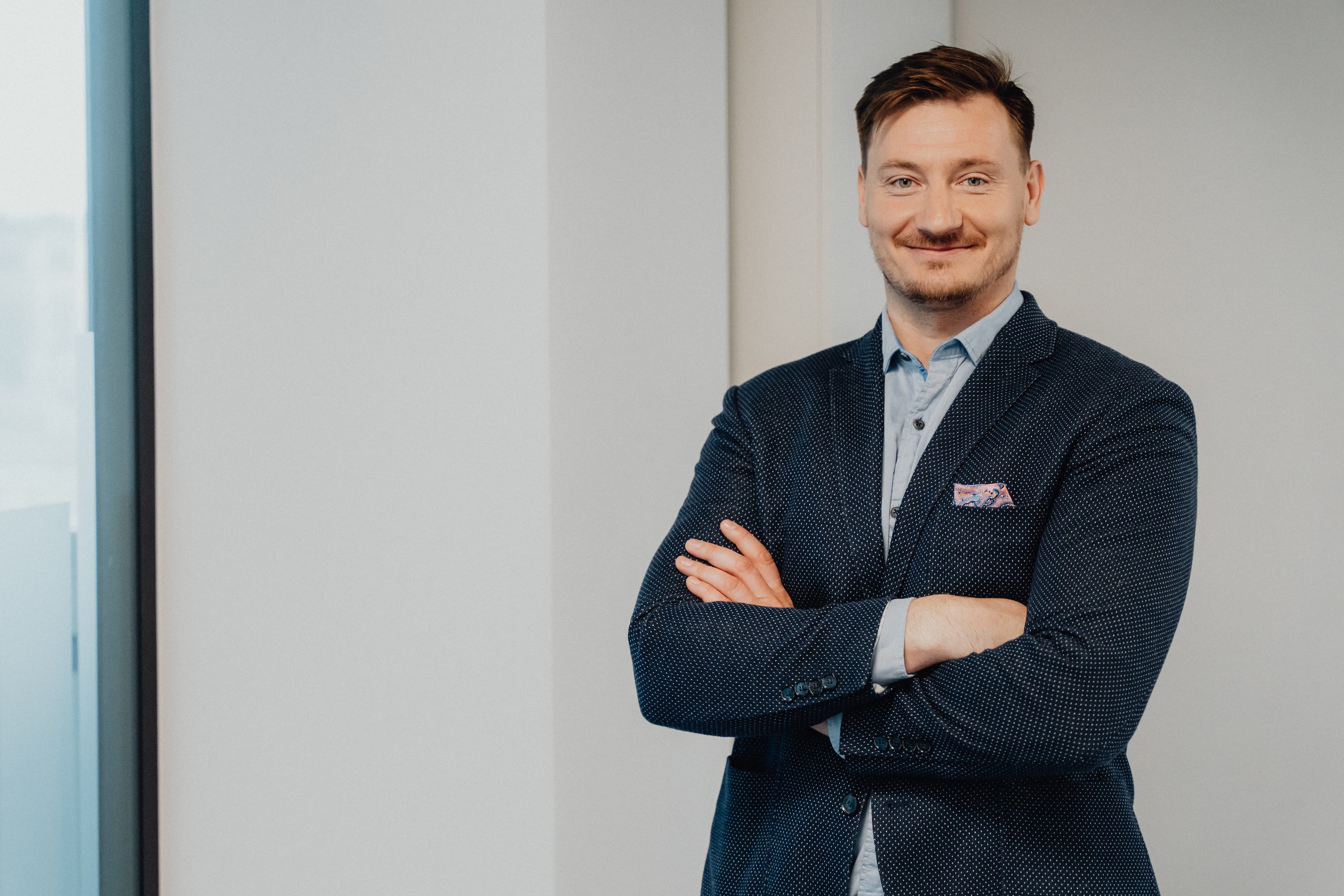
A Clear Perspective: The Python Language Advantages and Disadvantages
Python has emerged as one of the most popular programming languages, valued for its simplicity and versatility. This article examines its key advantages, such as ease of learning and extensive libraries, alongside potential drawbacks, including performance limitations and challenges in mobile computing. Understanding these factors can help developers make informed choices about using Python for their projects.
Marek Majdak
Jul 16, 2024・7 min read
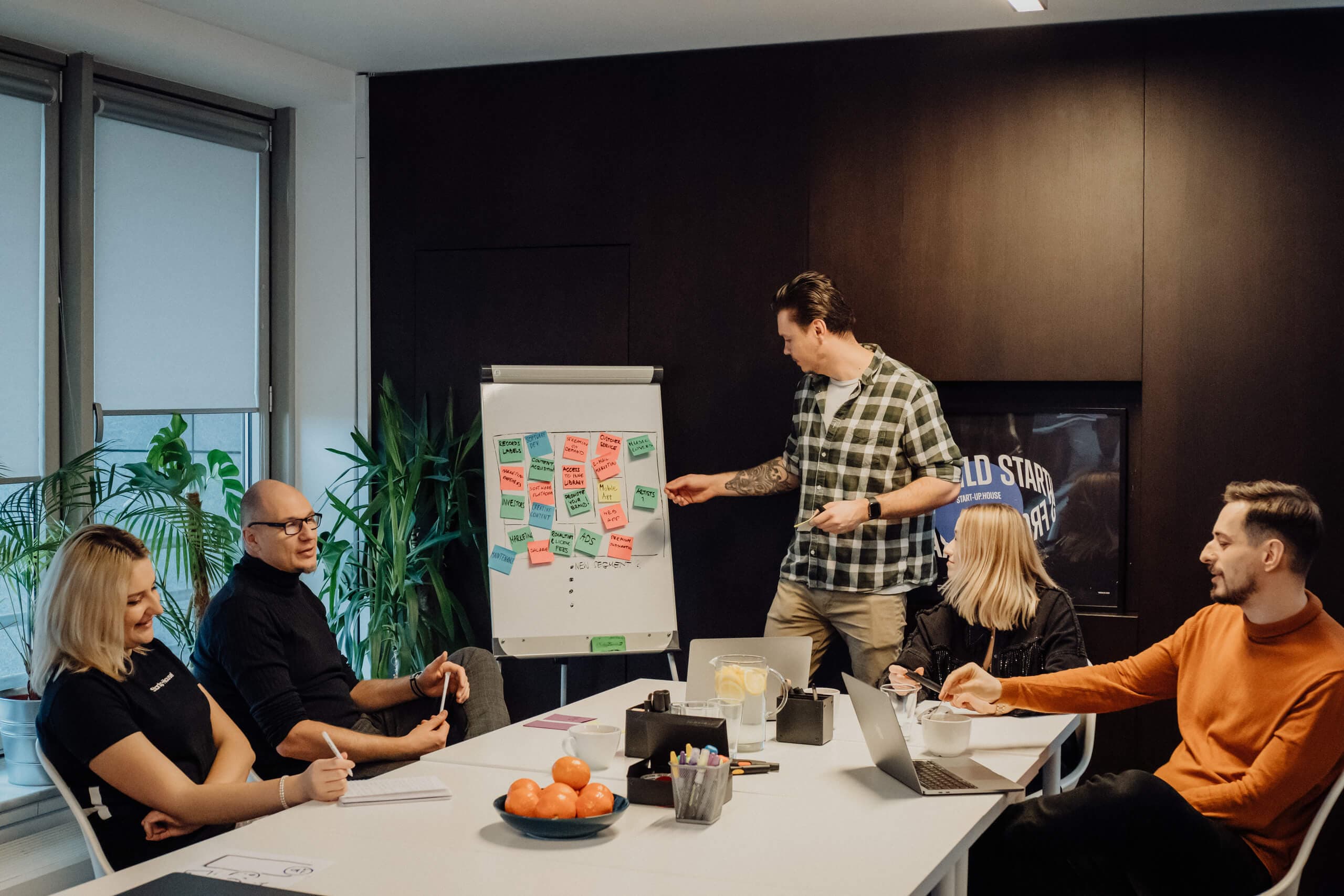
In-house vs Outsourcing Software Development: Making the Right Choice for Your Business
Choosing between in-house and outsourcing software development is a crucial decision that can significantly influence your business’s success. This article explores the distinct advantages and challenges of each approach, helping you assess your specific needs and make an informed choice. Whether you prioritize control and team cohesion or seek specialized skills and cost efficiency, understanding these factors is essential for strategic planning.
Alexander Stasiak
Aug 20, 2024・11 min read