🌍 All
About us
Digitalization
News
Startups
Development
Design
Is JavaScript Single-Threaded? Understanding the Basics
Marek Majdak
Apr 29, 2024・8 min read
Table of Content
Understanding JavaScript's Single-Threaded Nature
The Event Loop Explained
JavaScript's Asynchronous Capabilities
Common Misconceptions
Practical Implications for Developers
JavaScript is a powerful and versatile programming language that is widely used for web development, and one of its fundamental characteristics is its single-threaded nature. But what does it mean for JavaScript to be single-threaded? In straightforward terms, it implies that JavaScript operates on a single call stack, executing one task at a time. This design simplifies the language, making it easier for developers to manage tasks without the complexities of multi-threading. However, it also raises questions about performance and how JavaScript handles multiple operations efficiently. In this discussion, we will delve into the core concept of JavaScript being single-threaded, exploring how it manages to maintain smooth and responsive applications.
Understanding JavaScript's Single-Threaded Nature
The Core Concepts of Single-Threading
At its core, JavaScript's single-threaded nature means it uses a single call stack to execute code. This call stack is like a to-do list that processes one task at a time in the order they are called. When a function is invoked, it gets added to the call stack, and once it completes, it is removed, allowing the next function to be processed. This model avoids the complexities of dealing with multiple threads, such as race conditions and deadlocks. However, it can also mean that long-running tasks might block the execution of subsequent code. JavaScript mitigates this through asynchronous programming techniques, ensuring the application remains responsive even when performing complex operations. Understanding this mechanism is crucial for effectively managing performance and developing efficient web applications.
How JavaScript Executes Code
JavaScript executes code using a combination of the call stack and the event loop. When a script runs, functions and operations are added to the call stack in the order they are called. The JavaScript engine processes each item on the call stack sequentially. If an operation involves waiting, like a network request or timer, it is handled by the browser's Web APIs, and the main thread continues processing other tasks. Once the waiting operation completes, the event loop places its callback in the task queue. The event loop constantly checks the call stack and, if it's empty, moves the next task from the queue to the stack. This approach allows JavaScript to handle asynchronous operations efficiently while maintaining a single-threaded execution model, ensuring that the application remains responsive. Understanding this process is vital for developers to optimise code performance and manage asynchronous tasks effectively.
Comparing Single vs Multi-threaded Languages
Single-threaded and multi-threaded languages have distinct execution models that affect how they manage tasks. JavaScript, being single-threaded, processes one task at a time, which simplifies development by eliminating the need for complex thread management. This is advantageous for web applications where responsiveness is key, and where asynchronous operations can be used to handle tasks that might otherwise block execution.
In contrast, multi-threaded languages like Java or C++ can execute multiple threads simultaneously. This allows for parallel processing, where different parts of a program can run concurrently, enhancing performance for CPU-intensive tasks. However, it also introduces challenges such as synchronisation issues and potential deadlocks, which require careful management.
The choice between single-threaded and multi-threaded depends on the application's needs. For tasks involving heavy computations, multi-threading can offer performance benefits. Meanwhile, single-threaded models like JavaScript are well-suited for applications where simplicity and responsiveness are priorities.
The Event Loop Explained
How the Event Loop Works
The event loop is a fundamental component of JavaScript's concurrency model, allowing it to handle asynchronous operations effectively. When a JavaScript program runs, synchronous code is executed first, filling the call stack with tasks to be processed. Meanwhile, asynchronous tasks, such as timers or network requests, are handled by the browser's Web APIs. Once these tasks complete, their callbacks are placed in the task queue.
The event loop continuously monitors the call stack and task queue. When the call stack is empty, the event loop takes the first task from the queue and pushes it onto the stack for execution. This ensures that asynchronous tasks are processed as soon as the call stack is clear, allowing the application to remain responsive.
By managing asynchronous operations in this manner, the event loop helps JavaScript maintain its single-threaded nature while efficiently performing multiple tasks. Understanding the event loop is crucial for developers aiming to optimise performance and responsiveness in web applications.
Role of Callbacks and Promises
Callbacks and promises are integral to managing asynchronous operations in JavaScript. A callback is a function passed as an argument to another function, designed to be executed once an asynchronous operation completes. This pattern allows developers to specify the actions to be taken after tasks like network requests or file operations finish. However, extensive use of callbacks can lead to "callback hell," where nested callback functions become difficult to manage and read.
Promises provide a more structured approach to handling asynchronous tasks, representing a value that may be available now, in the future, or never. A promise can be in one of three states: pending, fulfilled, or rejected. It allows developers to attach handlers using .then() and .catch() methods, promoting cleaner and more readable code.
Both callbacks and promises work in tandem with the event loop, ensuring that asynchronous operations don't block the execution of other code, thus maintaining a smooth and responsive user experience.
JavaScript's Asynchronous Capabilities
Non-blocking I/O Operations
Non-blocking I/O operations are a key feature in JavaScript, enabling efficient management of tasks like file reading, network requests, and database interactions without hindering the execution of other code. In traditional blocking I/O, operations must complete before the program proceeds, often leading to unresponsive applications during lengthy tasks. JavaScript, however, utilises non-blocking I/O to ensure these operations run in the background.
When a non-blocking I/O operation is initiated, JavaScript delegates the task to the system's Web APIs or Node.js runtime. This allows the main thread to continue executing other code. Once the I/O operation completes, a callback or promise is used to handle the result. This approach is crucial for maintaining the responsiveness of applications, particularly in environments like web browsers where user interaction is constant.
By leveraging non-blocking I/O, JavaScript maximises performance and responsiveness, allowing developers to build applications that handle multiple operations seamlessly.
Utilising Async/Await Functions
Async/await is a syntactic feature in JavaScript that simplifies working with promises, making asynchronous code appear more like synchronous code. By using the async keyword before a function declaration, that function is set up to return a promise. Within this function, the await keyword can be used to pause execution until a promise is resolved, returning the result or throwing an error.
This approach offers several benefits over traditional promise chaining or callbacks. It enhances code readability and maintainability by reducing the need for nested .then() or callback structures. With async/await, developers can write code that flows more naturally, making it easier to debug and understand.
However, async/await requires careful error handling, as any errors thrown within the async function need to be caught using try/catch blocks. Utilising async/await effectively allows developers to manage asynchronous tasks more efficiently, leading to cleaner and more robust JavaScript applications.
Common Misconceptions
Debunking Multi-threading Myths
A common misconception is that JavaScript cannot handle multiple tasks efficiently due to its single-threaded nature. While it is true that JavaScript executes code on a single thread, its architecture, including the event loop and asynchronous capabilities, allows it to manage multiple concurrent operations effectively. This design is often misunderstood as a limitation; however, it is a strength in scenarios where simplicity and responsiveness are crucial.
Another myth is that multi-threading is always superior to single-threading. While multi-threading can enhance performance for CPU-intensive tasks, it introduces complexities like synchronisation issues and potential race conditions. JavaScript's model avoids these pitfalls by using non-blocking I/O and the event loop to maintain application responsiveness without the overhead of managing multiple threads.
Moreover, modern JavaScript environments offer solutions like Web Workers, enabling background processing on separate threads for tasks that require heavy computation. Understanding these nuances helps developers appreciate JavaScript's robust concurrency model.
Understanding Concurrency in JavaScript
Concurrency in JavaScript often leads to confusion, especially given its single-threaded execution model. While it processes one task at a time on the call stack, JavaScript handles multiple operations concurrently through its event-driven architecture. This means JavaScript can manage multiple tasks by delegating long-running operations to the system's Web APIs or Node.js, which operate independently of the main thread.
Asynchronous operations such as network requests, timers, or file I/O are handled in the background, allowing the main thread to continue executing other code. Once these operations complete, their results are queued to be processed when the call stack is clear.
This concurrency model ensures high responsiveness and efficiency, making it ideal for web applications where user interaction cannot be blocked. Developers often misconstrue JavaScript's concurrency capabilities as a limitation, but in reality, it is a powerful feature that, when properly understood and utilised, offers robust solutions for handling multiple operations simultaneously without the need for multi-threading.
Practical Implications for Developers
Best Practices for Writing Efficient Code
Writing efficient JavaScript code involves leveraging its asynchronous capabilities while maintaining clarity and performance. One key practice is to minimise blocking operations on the main thread. Long-running tasks should be handled using asynchronous techniques, such as promises or async/await, to keep applications responsive.
Additionally, developers should optimise DOM manipulation, as it can be expensive in terms of performance. Batch DOM updates and use document fragments to reduce reflows and repaints. Utilising tools like debouncing and throttling can also help manage the frequency of function calls, particularly in response to user events.
Another best practice is to take advantage of caching strategies to avoid redundant data fetching. This can significantly reduce load times and server requests. Moreover, profiling tools can be used to identify bottlenecks in the code, allowing developers to focus on areas that need optimisation.
By adopting these practices, developers can ensure their JavaScript applications are both efficient and scalable, providing a smooth user experience.
Tools and Techniques for Optimisation
Optimising JavaScript code requires a combination of effective tools and techniques to enhance performance and maintainability. One essential tool is the browser’s built-in developer tools, which offer features like the JavaScript profiler to analyse execution time and identify bottlenecks. Using these insights, developers can pinpoint and optimise inefficient code segments.
Minification and bundling are techniques that reduce file size and improve load times by removing unnecessary characters and combining multiple files into one. Tools like Webpack and Babel automate this process, ensuring a streamlined codebase.
Another technique is lazy loading, which defers the loading of non-essential resources until they are needed. This can significantly improve initial load times and overall performance.
Developers should also consider using service workers to cache assets for offline use and faster loading. By combining these tools and techniques, developers can optimise JavaScript applications, ensuring they are fast, efficient, and responsive to user interactions.
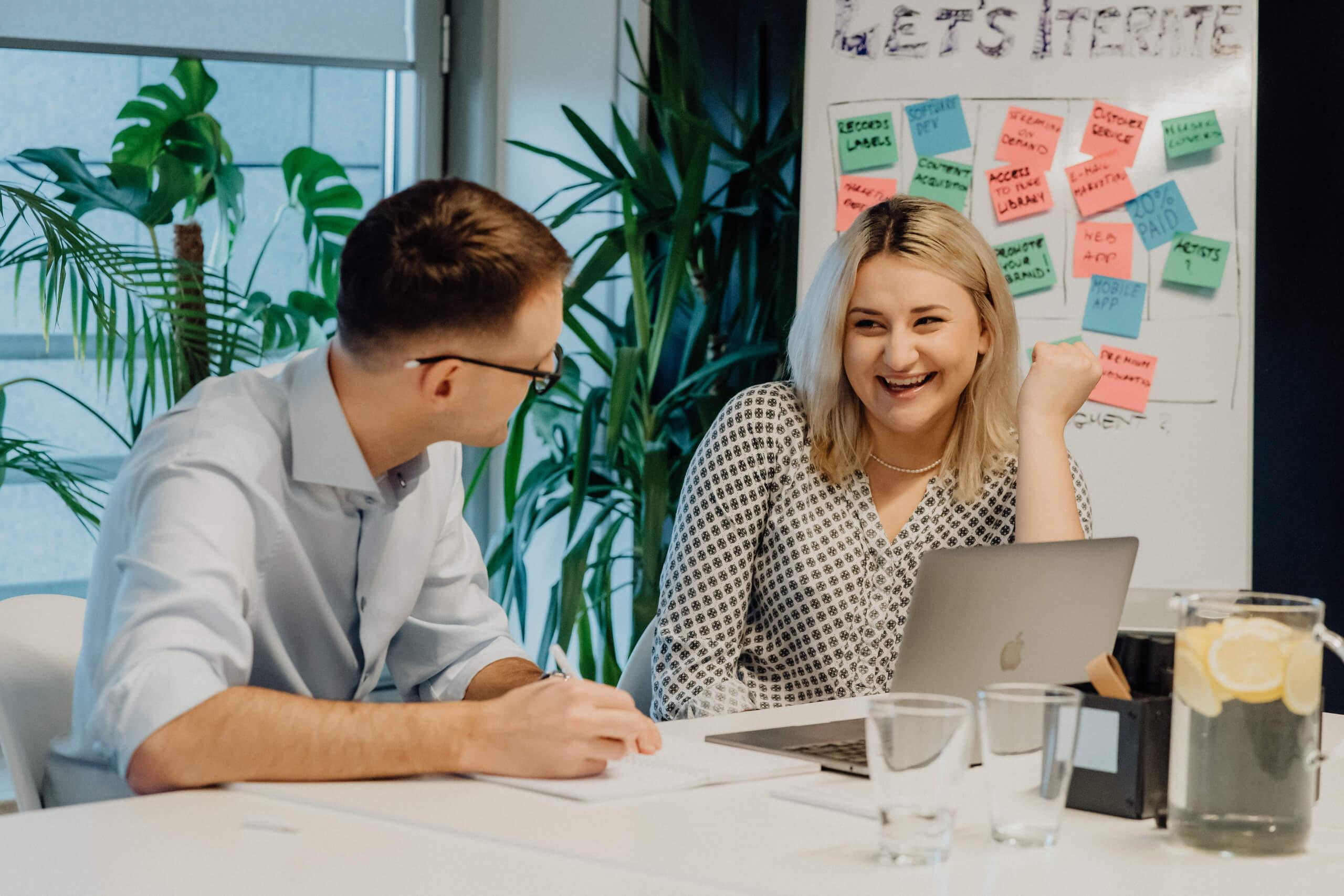
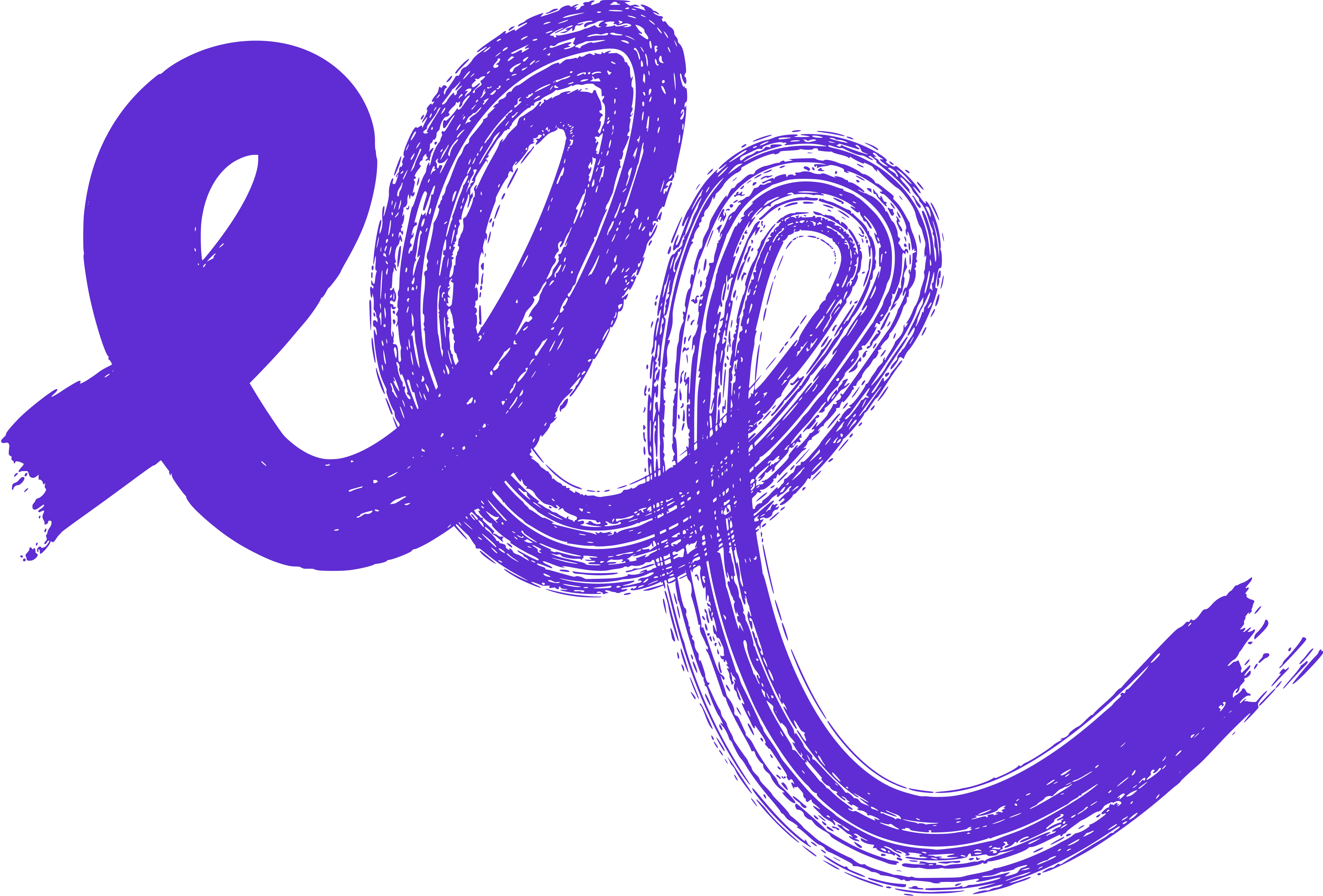
You may also
like...
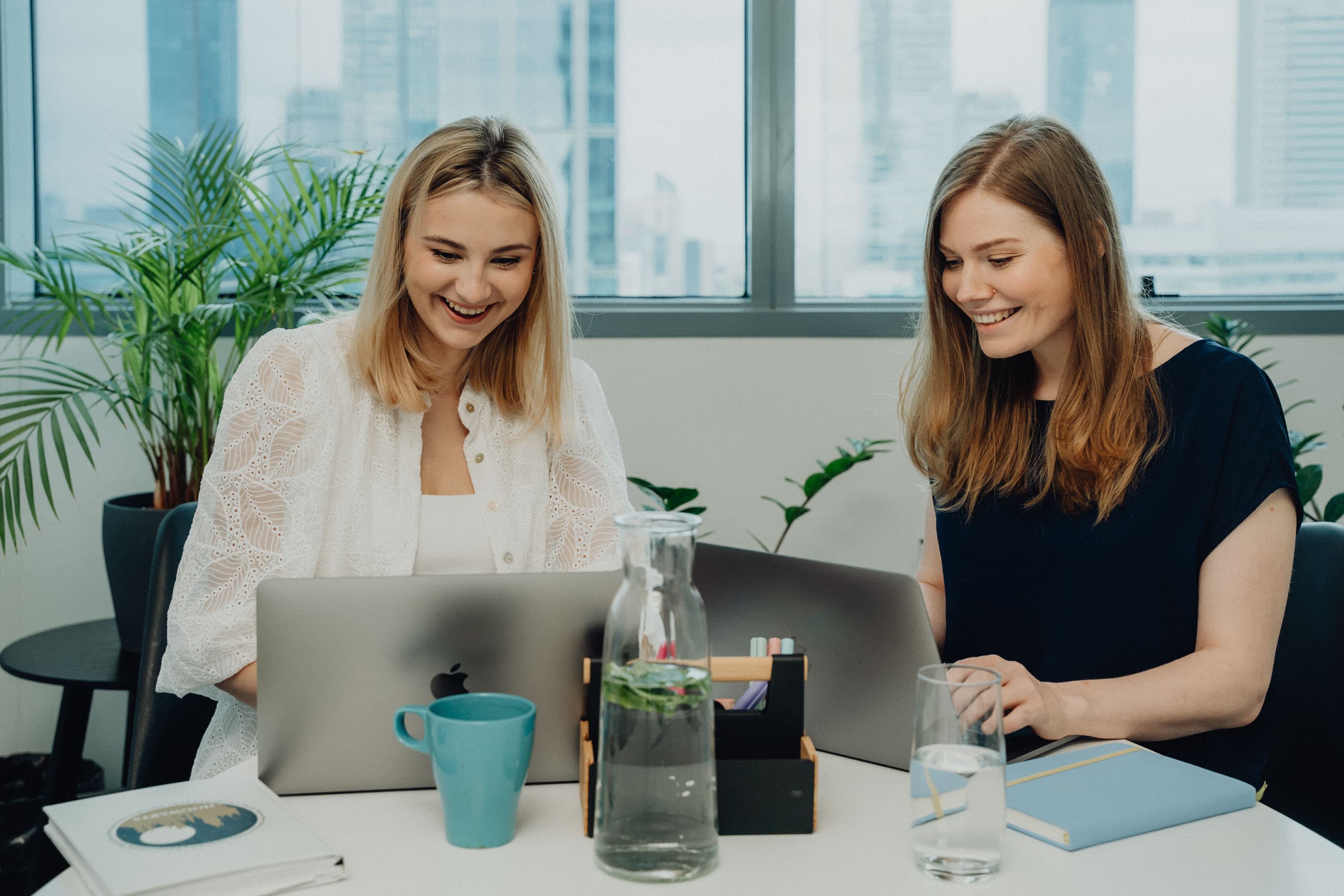
Mastering Declarative Programming: Essential Practices for Every Developer
Discover declarative programming essentials. This guide covers principles, tools, and best practices to simplify coding, enhance readability, and improve scalability.
Marek Pałys
Apr 16, 2024・11 min read
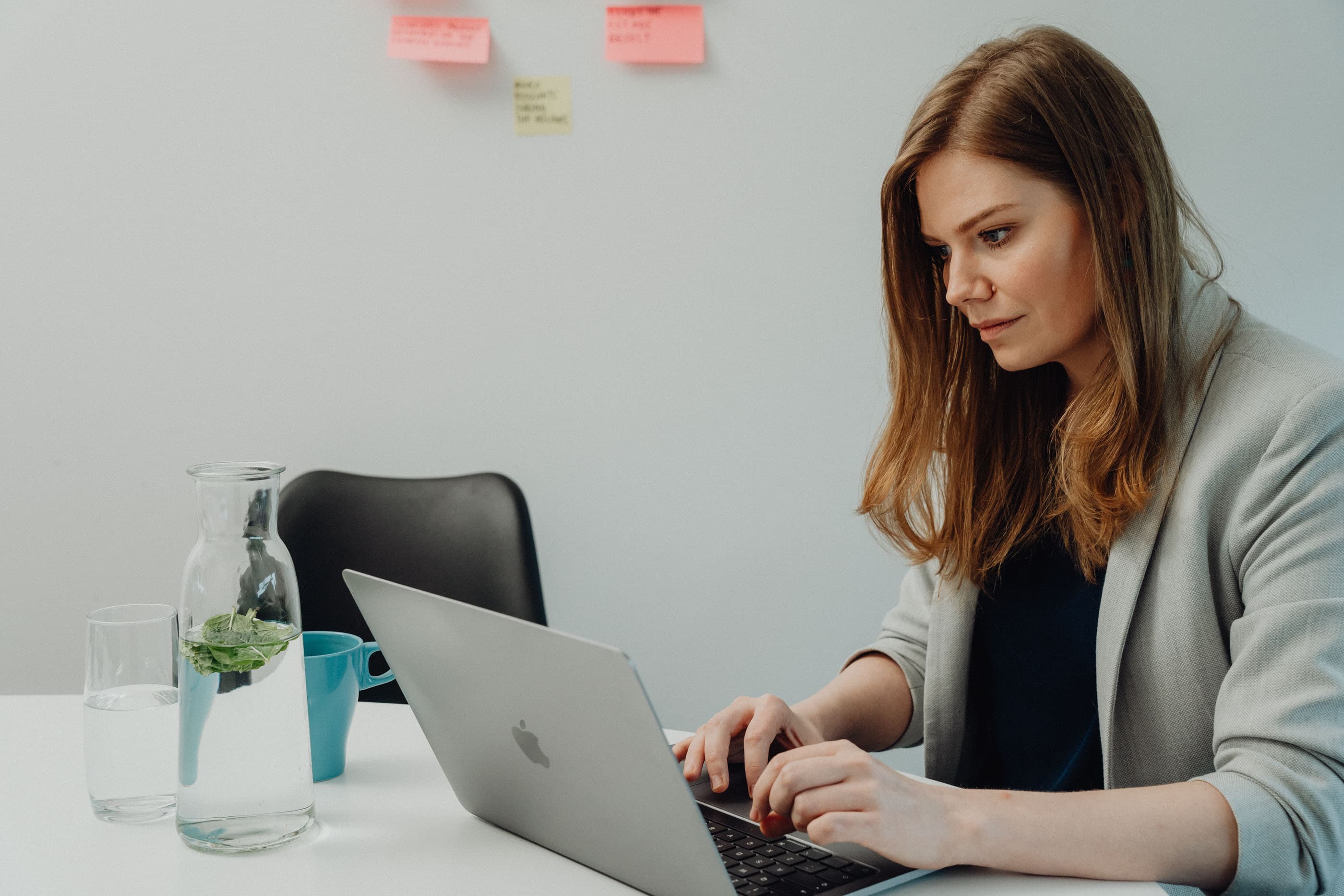
Understanding Event-Driven Programming: A Simple Guide for Everyone
Explore the essentials of event-driven programming. Learn how this responsive paradigm powers interactive applications with real-world examples and key concepts.
Marek Pałys
Apr 30, 2024・9 min read
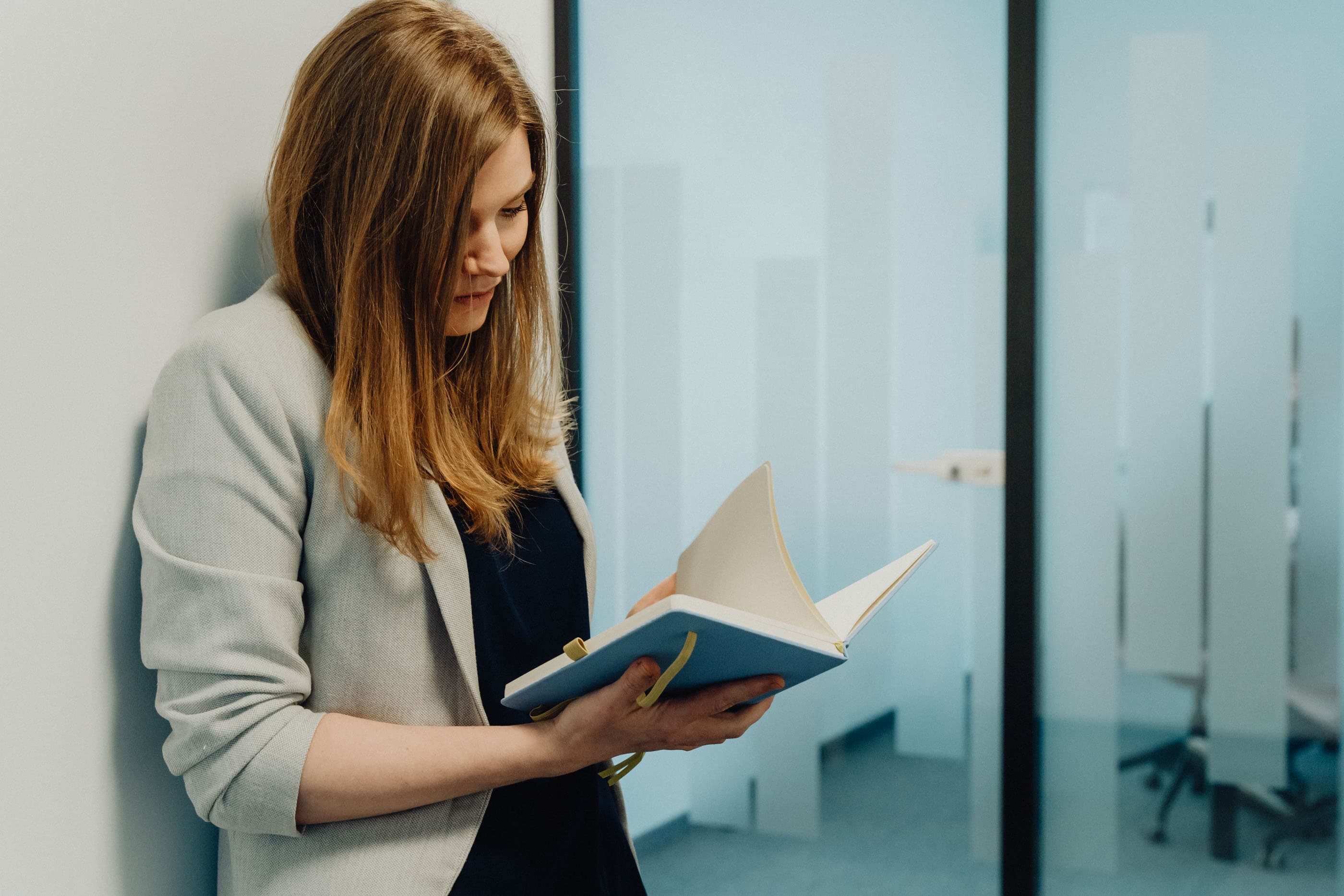
Demystifying Procedural Programming: Simple Examples for All
Explore procedural programming with easy-to-follow examples and insights into its core principles. Learn how this step-by-step approach forms the basis of many programming paradigms.
Marek Pałys
Jul 05, 2024・10 min read