🌍 All
About us
Digitalization
News
Startups
Development
Design
Tutorial on how to install Ruby, Ruby on Rails and how to use Ruby Gems
Jan Grela
Mar 20, 2020・6 min read
Table of Content
How to install Ruby on Mac?
How to install Ruby on Windows?
What is RubyGems?
How to use Ruby Gems?
Ruby Bundler
How to use Ruby on Rails?
How to set up a Ruby on Rails development environment?
Where to go next?
You already heard about Ruby on Rails and how easy it is to quickly develop working applications. However, the process of creating new software requires a few building blocks and understanding how to handle them efficiently.
The first step is, of course, installing Ruby itself. As the number of your projects will surely quickly increase, managing multiple variants of Ruby can become quite unpleasant. To help with that, the open source community prepared multiple version managers, with most popular ones being rbenv and rvm.
How to install Ruby on Mac?
Both rvm and rbenv are available for MacOS and popular Linux distributions, so the whole process comes down to pasting a few command lines into the console.
rbenv
1. With Homebrew installed, run init in your Terminal (and if you don’t have it, you can install rbenv with GitHub checkout):
$ brew install rbenv
2. After it is done, type:
$ rbenv init
3. This will prompt a guide on how to set up the shell. Usually, it’s enough to just add one line to .zshrc or .bash_profile:
$ echo 'eval "$(rbenv init -)"' >> ~/.zshrc
4. Now everytime Terminal is opened, rbenv should be up and ready to work.
$ source ~/.zshrv
5. Finally, it’s time to install actual Ruby. Now it’s as simple as the providing version number after the install command:
$ rbenv install 2.7.0
$ rbenv global 2.7.0
6. To set the local version to use, just type in:
$ rbenv local 2.7.0
rvm
If you have already installed Ruby using rbenv, your work is complete. Rvm is an alternative tool to do the same thing and generally it’s not a good idea to use them both at the same time — besides, there really is no point.
Installing rvm is quite similar to rbenv, and again, there are only few commands that need to be run in the console:
1. Let’s start with adding PGP keys:
gpg --keyserver hkp://keys.gnupg.net --recv-keys 409B6B1796C275462A1703113804BB82D39DC0E3
2. Then fetching rvm and installing the latest version of Ruby just after that:
$ curl -sSL https://get.rvm.io | bash -s stable --ruby
3. Different versions of Ruby can be quickly downloaded and installed with the install command, just like so:
$ rvm install 2.7.0
It’s important to mention that in your project directory, you can create a .ruby-version file (simple text file with the Ruby version number). Based on this file both rbenv and rvm will try to use the proper Ruby version or will prompt installing the missing one.
How to install Ruby on Windows?
If you are using Windows, the best option is to use RubyInstaller. Just get the .exe file for the desired Ruby version and start the installation. RubyInstaller can be combined with MSYS2 (a port of the Unix development toolset) and MinGW libraries, providing a great development environment.
Ruby installation check
After installation, you can check version with:
$ ruby -v
And see where the interpreter is located:
$ which ruby
Ruby is an interpreted programming language. To run the code, it’s enough to just point to the .rb file and hit execute:
$ ruby app.rb
Along with the interpreter comes the Interactive Ruby Shell (irb) which executes Ruby commands as you type them in real time:
$ irb
irb(main):001:0> 2+2
=> 4
What is RubyGems?
The standard Ruby installation comes with Ruby Core API and Standard Library API, which are used to write Ruby applications. A simple application could be written from scratch using those APIs, but when it comes to something more complex, it is best to use Ruby packages provided in the Package Manager. Ruby has one called RubyGems and the packages are called gems.
RubyGems comes with a command-line utility called a gem. It installs gems from specified public or private repositories. Another thing worth mentioning is https://rubygems.org/, which hosts many gems from the Ruby community. Typically, a gem delivers new libraries with particular functionalities and, sometimes, command-line tools.
RubyGems comes with the standard Ruby installation, so there’s no need to install anything else.
How to use Ruby Gems?
Let’s see how to work with gems. This command will print the RubyGems settings with info where the gems are installed:
$ gem env
Gems install / uninstall
To install and uninstall gems, type:
$ gem install gem_name (-v version to specify gem version)
$ gem uninstall gem_name
Gems update
To update specific gem, run:
$ gem update gem_name
Or all gems:
$ gem update
Gems list
Gems are installed in a local repository. To list installed gems type:
$ gem list
While installing gems all dependencies are installed along with the gem.
Ruby Bundler
During project development you can discover that your project requires more and more gems. Installing them all manually with the gem command would be difficult if not impossible to match all dependencies. There is a smart tool that is used to manage all gems in a project — the Bundler.
Install Bundler and specify gems for the project
It comes as a gem, so first install it:
$ gem install bundler
Then create a Gemfile in your project root directory with the content:
source ‘https://rubygems.org’ gem ‘rails’, ’6.0.2.1’ gem ‘rspec’
Install gems with Bundler
To install, type:
$ bundle install
It will install all the gems with the specified version and their dependencies. If you want to use another gem in your project, then add it to the Gemfile and run the bundle install again.
Update gems with Bundler
If you want to update some gems for your project, change the version in the Gemfile and again, run:
$ bundle install
To update specific gem, run:
$ bundle update gem_name
Or for all gems:
$ bundle update
In either case, Bundler will resolve the dependencies.
How to use Ruby on Rails?
After all the installations and settings it’s time to start a new Ruby on Rails project and set up a couple of things.
Install Rails
No surprise here — Rails comes with a gem:
$ gem install rails
It will install the newest Rails with some other gems Rails depends on. Rails itself has a command-line utility which could be used to create a new Ruby on Rails application.
Check the version and help messages which describe options for creating a new app.
$ rails -v
$ rails -h
New Rails application
To create a Rails project type with default options:
$ rails new my_amazing_rails_app
That command will generate the whole project structure under my_amazing_rails_app directory. You will see the output of creating application files and then Bundler will install all dependencies from the newly created Gemfile.
Next, enter my_amazing_rails_app directory and start a Rails server.
$ cd my_amazing_rails_app
$ rails server
This will run a web server Puma, which is used by Rails applications by default. The default port is 3000. Go to your browser and enter http://localhost:3000 and you should see Rails welcome page.
How to set up a Ruby on Rails development environment?
The default database used by Rails is SQLite. If you want to use different DB from the beginning, you can provide the DB from the following option:
$ rails new my_amazing_rails_app —database postgresql
The database configuration is located in the config/database.yml file. Rails runs the application within the specified environment (for starting app locally environment called “development” is used as default). To provide your DB settings, edit the development settings in config/database.yml.
Another often used option is api, which configures the application with limited middleware, only for API-like applications.
$ rails new my_amazing_rails_app —api
Rails generates a lot of files. In the app directory, you will find the main files for the application. As Rails was created following the MVC (Model-View-Controller) pattern, you will find there are files for models, controllers and views in appropriate directories. In the config directory, you will find configuration files for the application, environments, the database, routes, etc. In the db directory, you will find the DB schema and migration files.
Where to go next?
There are many of Rails tutorials and guides. Just to mention one https://guides.rubyonrails.org/, which describes most of Rails features with examples. Ruby and Rails APIs are well documented. Just create a new app, create a model, a controller and a view for it, and you might fall in love with Rails!
If you are interested in Ruby on Rails development, read our article to find out why it’s worth learning Ruby on Rails.
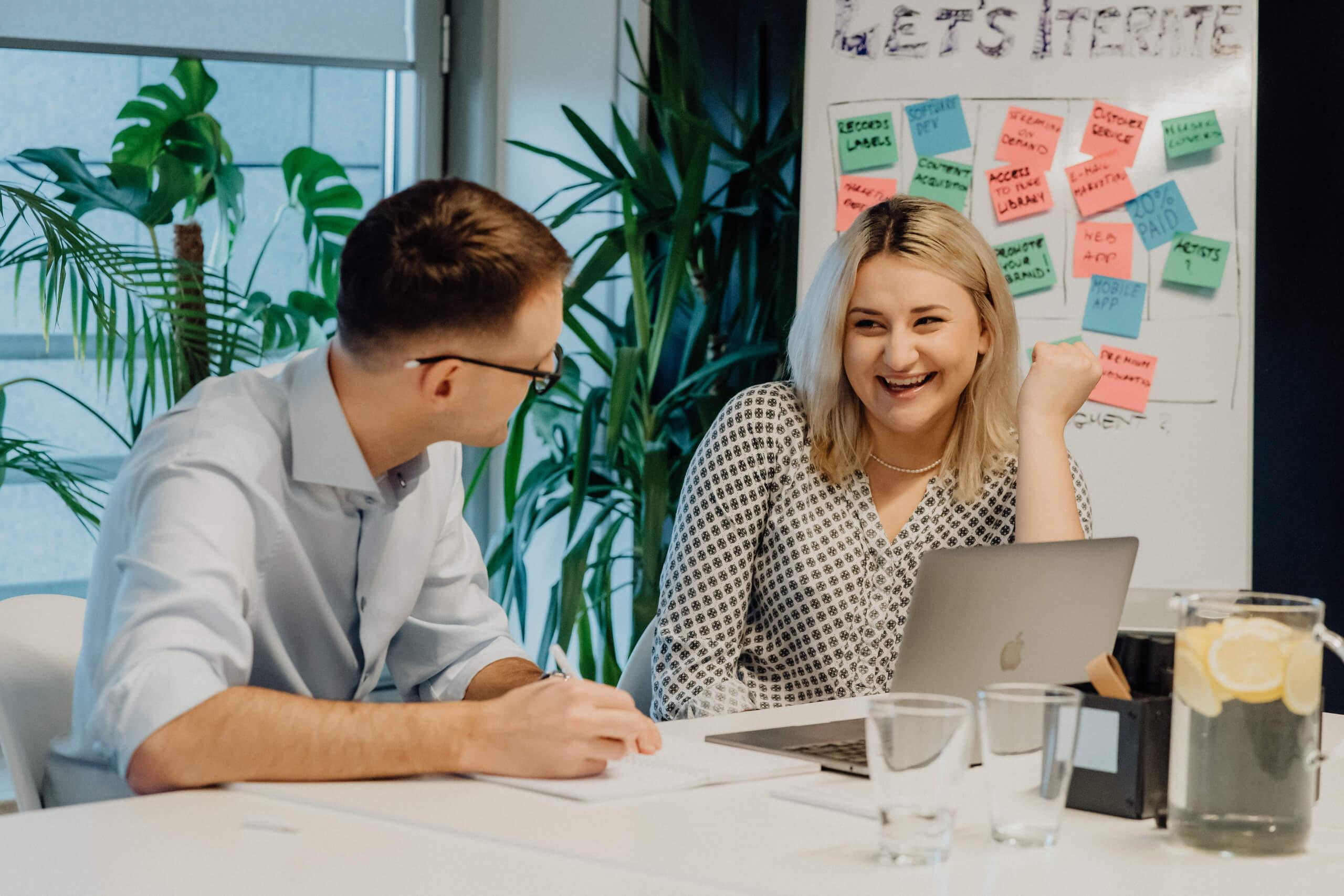
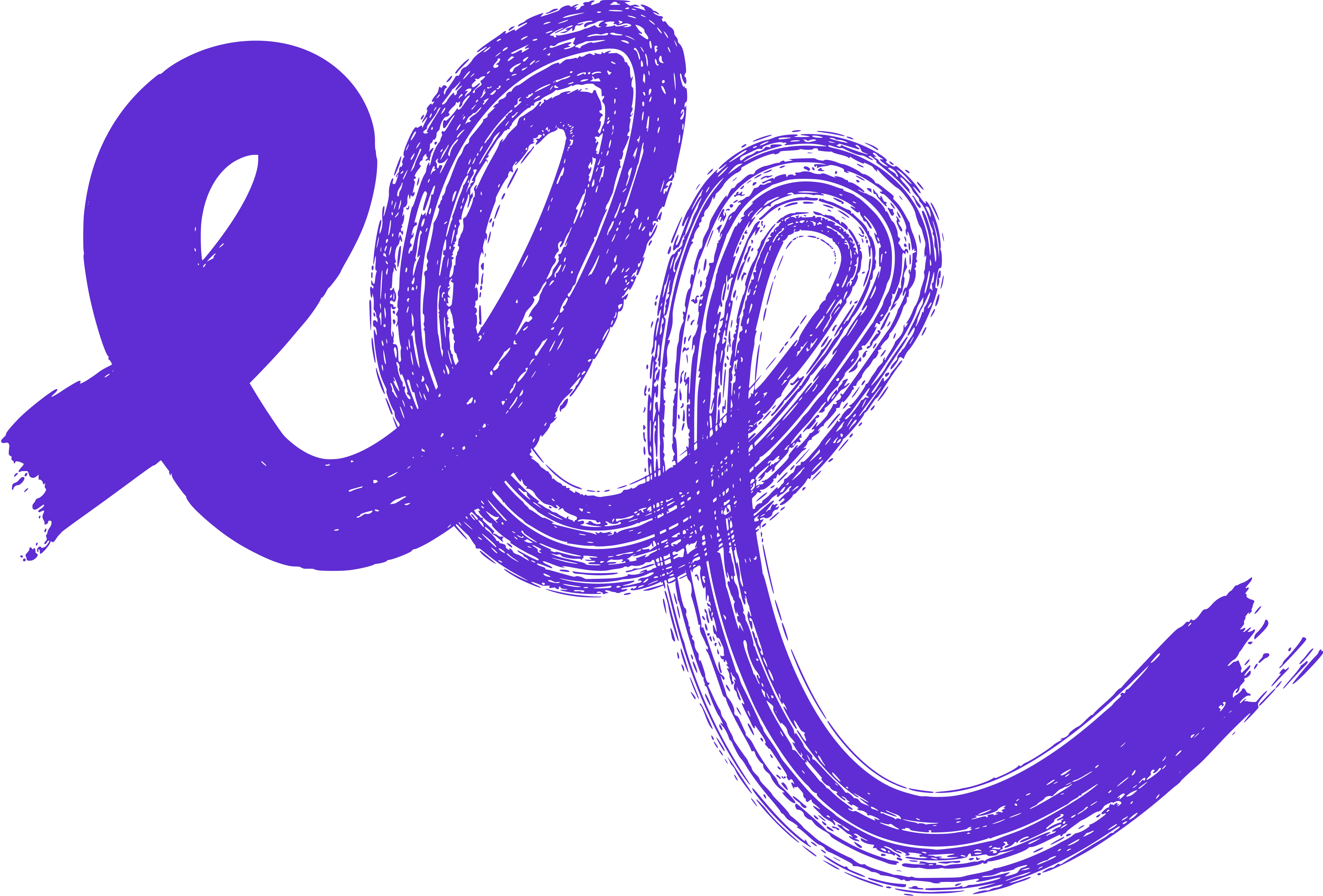
You may also
like...
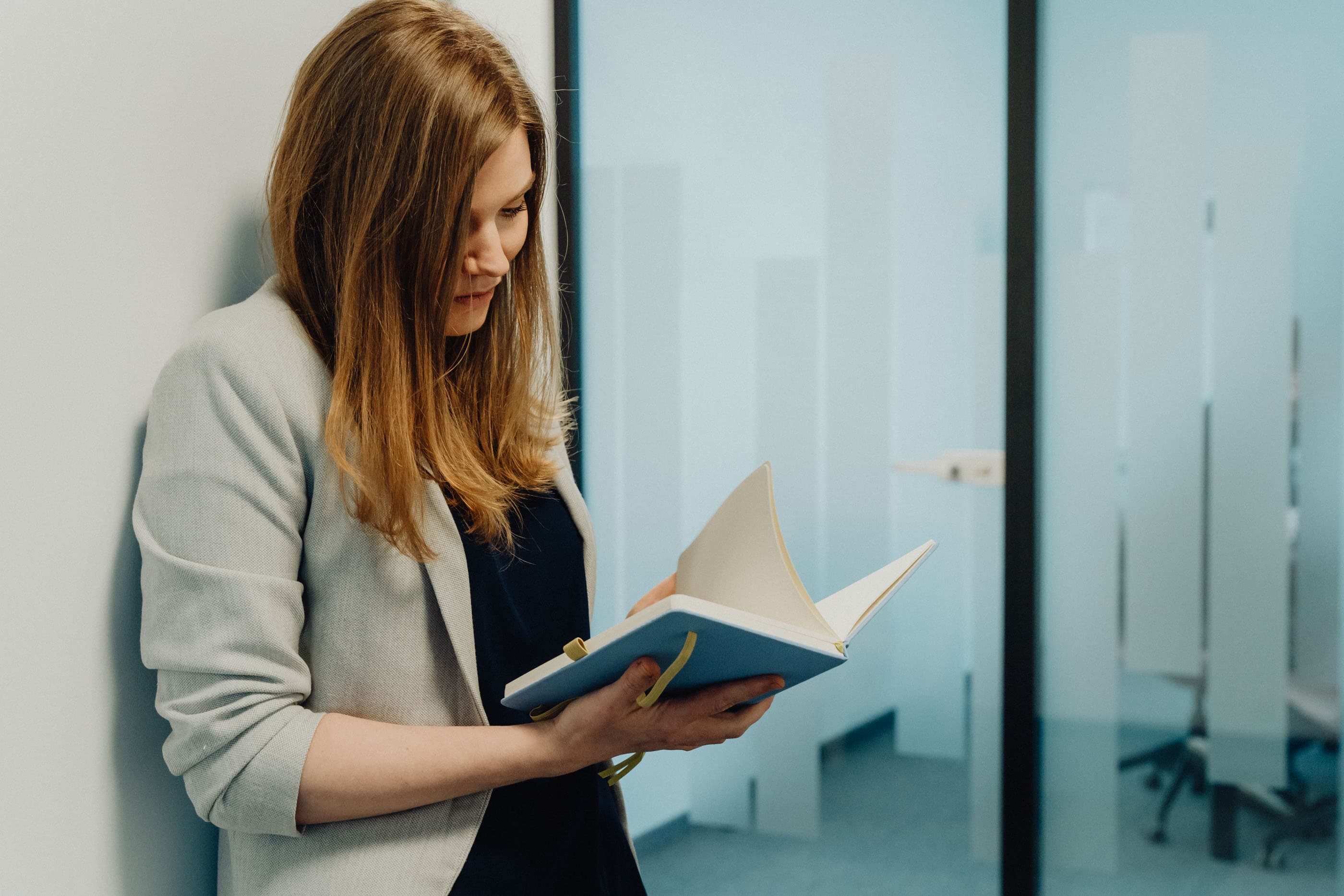
Demystifying Procedural Programming: Simple Examples for All
Explore procedural programming with easy-to-follow examples and insights into its core principles. Learn how this step-by-step approach forms the basis of many programming paradigms.
Marek Pałys
Jul 05, 2024・10 min read
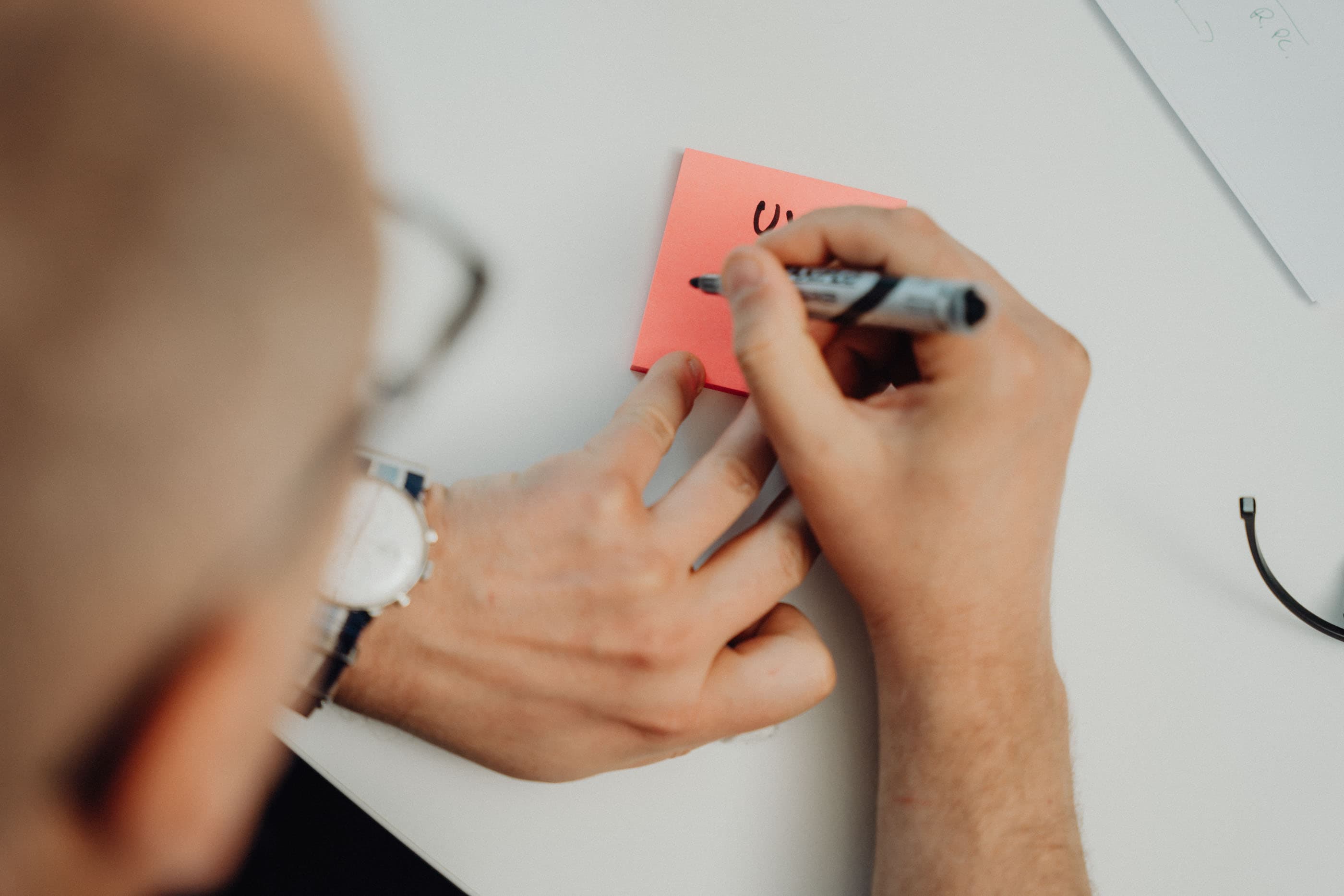
Understanding the Basics: Unravelling Functional and Object-Oriented Programming
Dive into the fundamentals of functional and object-oriented programming. Discover their unique features, benefits, and when to use each paradigm for effective coding solutions.
Alexander Stasiak
Jun 18, 2024・13 min read
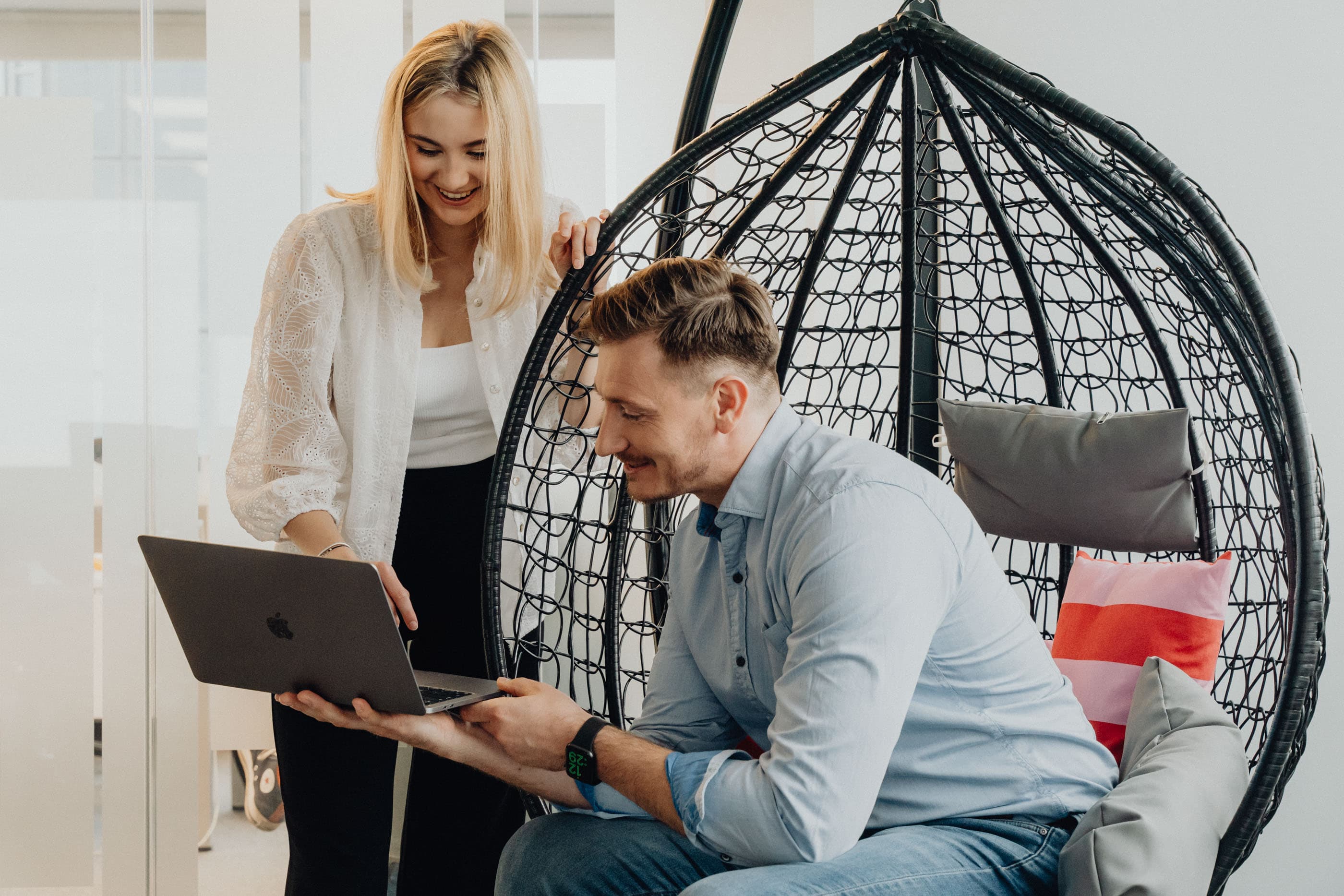
Understanding the Differences: BaseModel vs. ActiveRecord Validator in Ruby on Rails
BaseModel and ActiveRecord Validator in Ruby on Rails serve distinct purposes in application development. BaseModel provides a lightweight, database-independent option for handling data, while ActiveRecord Validator ensures data integrity through robust validation in database-backed models. This guide explores their differences, use cases, and benefits to help developers choose the right approach for their projects.
Alexander Stasiak
Nov 04, 2024・11 min read