🌍 All
About us
Digitalization
News
Startups
Development
Design
Getting Started with NestJS: A Modern Framework for Node.js
Viktor Kharchenko
Nov 14, 2023・5 min read
Table of Content
What is NestJS?
Modules and Providers
The MVC Architecture
TypeScript Support
Dependency Injection
Decorators
Getting Started with Your First NestJS Project
Conclusion
FAQs
Node.js has gained immense popularity in recent years for building server-side applications due to its performance and scalability. If you're looking to start your journey with Node.js and want a robust and organised framework to work with, NestJS is an excellent choice. In this article, we'll introduce you to NestJS and guide you through its fundamentals.
What is NestJS?
NestJS is a progressive Node.js framework designed for building efficient, scalable, and maintainable server-side applications. It combines the power of Node.js with TypeScript, making it a compelling choice for developers who prefer statically typed languages. Let's dive into the key aspects of NestJS:
Modules and Providers
NestJS applications are built using a modular approach. Modules and providers are fundamental building blocks of NestJS applications.
Modules:
A module is a way to organise your application into discrete and reusable parts. Each module encapsulates a closely related set of components, such as controllers, services, and other related files. Modules help in maintaining a clean and organised codebase.
Providers:
Providers are classes that can be injected into other components, such as controllers or other services. They play a crucial role in defining the business logic of your application. Providers are decorated with the @Injectable() decorator, making them injectable into other components that depend on them.
The MVC Architecture
NestJS follows the Model-View-Controller (MVC) architectural pattern, providing a structured and organised approach to building applications. This separation of concerns makes code maintainable and promotes scalability.
Model:
In NestJS, the Model represents the data and business logic of your application. It defines the structure of your data and how it interacts with the database. Here's an example of a simple user model using TypeScript:
// user.model.ts
export class User {
id: number;
username: string;
email: string;
// ... other properties and methods
}
View:
While NestJS primarily focuses on the backend, you can still create APIs that serve as the "view" for your frontend applications. The API endpoints defined in controllers act as the interface for your frontend to communicate with the server.
Controller:
Controllers handle incoming requests, process them by invoking services, and send back responses. They are responsible for defining the routes and request handling logic. Decorators play a crucial role in defining routes and linking controllers to specific endpoints. Here's an example of a simple controller in NestJS:
// app.controller.ts
import { Controller, Get } from '@nestjs/common';
@Controller('app')
export class AppController {
@Get()
getHello(): string {
return 'Hello, NestJS!';
}
}
TypeScript Support
TypeScript is a statically typed superset of JavaScript, and NestJS fully embraces it. This means you can write clean, type-safe code that catches errors at compile-time, improving your development workflow.
TypeScript provides features like interfaces and type checking, which are beneficial when defining data structures and contracts between different parts of your application. This ensures that you're working with consistent and well-defined data throughout your project.
Dependency Injection
Dependency Injection is a design pattern used in NestJS to manage component dependencies and improve the testability of your code. At its core, Dependency Injection is about providing the necessary dependencies (services or objects) to a component (e.g., a controller or another service) rather than having the component create them itself. This promotes code reusability and separation of concerns.
In NestJS, you can create services that encapsulate specific functionalities of your application. These services can then be injected into controllers or other services, promoting code modularity. Here's an example of a service and how it can be injected into a controller:
// cats.service.ts
import { Injectable } from '@nestjs/common';
@Injectable()
export class CatsService {
private readonly cats: string[] = [];
create(cat: string): void {
this.cats.push(cat);
}
findAll(): string[] {
return this.cats;
}
}
// cats.controller.ts
import { Controller, Post, Get, Body } from '@nestjs/common';
import { CatsService } from './cats.service';
@Controller('cats')
export class CatsController {
constructor(private readonly catsService: CatsService) {}
@Post()
async create(@Body() cat: string) {
this.catsService.create(cat);
}
@Get()
async findAll(): Promise<string[]> {
return this.catsService.findAll();
}
}
In this example, we have a CatsService that encapsulates the logic for managing a list of cats. This service is then injected into the CatsController, allowing the controller to utilise the functionality provided by the service.
Decorators
Decorators are a powerful feature in NestJS, allowing you to define metadata and create various decorators to handle routing, validation, and other functionalities. This simplifies code organisation and makes it more readable.
@Controller:
The @Controller decorator is used to define a controller class. It specifies the base route for all the routes defined within the controller.
@Get, @Post, @Put, @Delete:
These decorators are used within controller classes to specify the HTTP methods and routes associated with controller methods. For example, @Get() defines a GET route, and @Post() defines a POST route.
@Param, @Query, @Body:
These decorators are used to access request parameters, query parameters, and request bodies within controller methods. They simplify data retrieval and processing.
@Injectable:
The @Injectable() decorator is used to mark a class as a provider or service that can be injected into other components, such as controllers or other services.
Getting Started with Your First NestJS Project
Let's take a step-by-step approach to start your NestJS project:
1.Installation: Install NestJS globally using npm or yarn:
npm install -g @nestjs/cli
# OR
yarn global add @nestjs/cli
2.Create a New Project: Use the Nest CLI to create a new NestJS project:
nest new project-name
This command will generate the project structure and install the necessary dependencies.
Explore the Structure: After creating your project, navigate to the project folder. You'll find a well-organised project structure with folders for controllers, modules, services, and more.
3.Create Your First Module: Let's create a module, controller, and service. These are essential components in NestJS:
nest generate module module-name // nest g m module-name
nest generate controller controller-name // nest g c controller-name
nest generate service service-name // nest g s controller-name
Or make use of a set of shortcuts
nest g m module-name
nest g c controller-name
nest g s controller-name
Replace module-name, controller-name, and service-name with meaningful names for your project.
4.Define Routes: In your controller, use decorators like @Get(), @Post(), and others to define routes. Implement the business logic in your service.
5.Start the Application: Run your NestJS application with the following command:
npm run start
# OR
yarn start
By default, your NestJS application will listen on port 3000.
6. Test Your API: Open your browser or use tools like Postman to test your API endpoints. For example, if you have a GET route defined in your controller, you can access it at http://localhost:3000/controller-route.
Conclusion
NestJS is a powerful framework that simplifies Node.js development, providing a structured and efficient way to build server-side applications. Whether you're new to Node.js or an experienced developer, NestJS is worth exploring. With the resources mentioned here, you're well-equipped to embark on your NestJS journey. Happy coding!
FAQs
What is NestJS?
NestJS is a progressive Node.js framework for building efficient, scalable server-side applications.
What is the MVC architecture in NestJS?
MVC separates an app into Model, View, and Controller, promoting code organization and maintainability.
How does TypeScript support benefit NestJS?
TypeScript provides type safety, enhancing code quality and catching errors at compile-time.
What is Dependency Injection in NestJS?
Dependency Injection manages component dependencies, promoting code reusability and separation of concerns.
What are Modules and Providers in NestJS?
Modules organize the app into parts, while Providers are injectable classes used for defining business logic.
How do I create a new NestJS project?
Use nest new project-name to generate a new project structure with the Nest CLI.
What are decorators in NestJS?
Decorators are used to define metadata and simplify code organization, e.g., routing with @Get() and @Controller.
How can I start my NestJS application?
Run npm run start or yarn start to start your NestJS app, which listens on port 3000 by default.
How do I test API endpoints in NestJS?
Use tools like Postman or a web browser to test your API endpoints by accessing their URLs.
Can I create custom decorators in NestJS?
Yes, you can create custom decorators to add additional functionality or metadata to your code.
What is the role of the @Injectable() decorator?
The @Injectable() decorator marks a class as a provider, allowing it to be injected into other components.
How do I define routes in NestJS controllers?
Use decorators like @Get(), @Post(), etc., within controller classes to define routes.
Is NestJS suitable for both beginners and experienced developers?
Yes, NestJS is suitable for developers of all levels, offering a structured and efficient development experience.
Can I use NestJS for building RESTful APIs?
Yes, NestJS is an excellent choice for building RESTful APIs due to its routing and decorator features.
What databases are compatible with NestJS?
NestJS supports various databases, including MySQL, PostgreSQL, MongoDB, and more, via libraries like TypeORM.
Is NestJS suitable for real-time applications?
Yes, NestJS can be used for real-time applications with the integration of WebSocket libraries like Socket.io.
Does NestJS support microservices architecture?
Yes, NestJS supports microservices architecture and can be used to build distributed systems.
Can I use NestJS with a front-end framework like Angular?
Yes, NestJS can be used as a backend for Angular applications, offering a seamless integration experience.
Are there any official NestJS plugins or extensions?
Yes, NestJS has an active community and offers official and community-contributed plugins and extensions.
Where can I find NestJS documentation and tutorials?
You can find comprehensive documentation and tutorials on the official NestJS website and community forums.
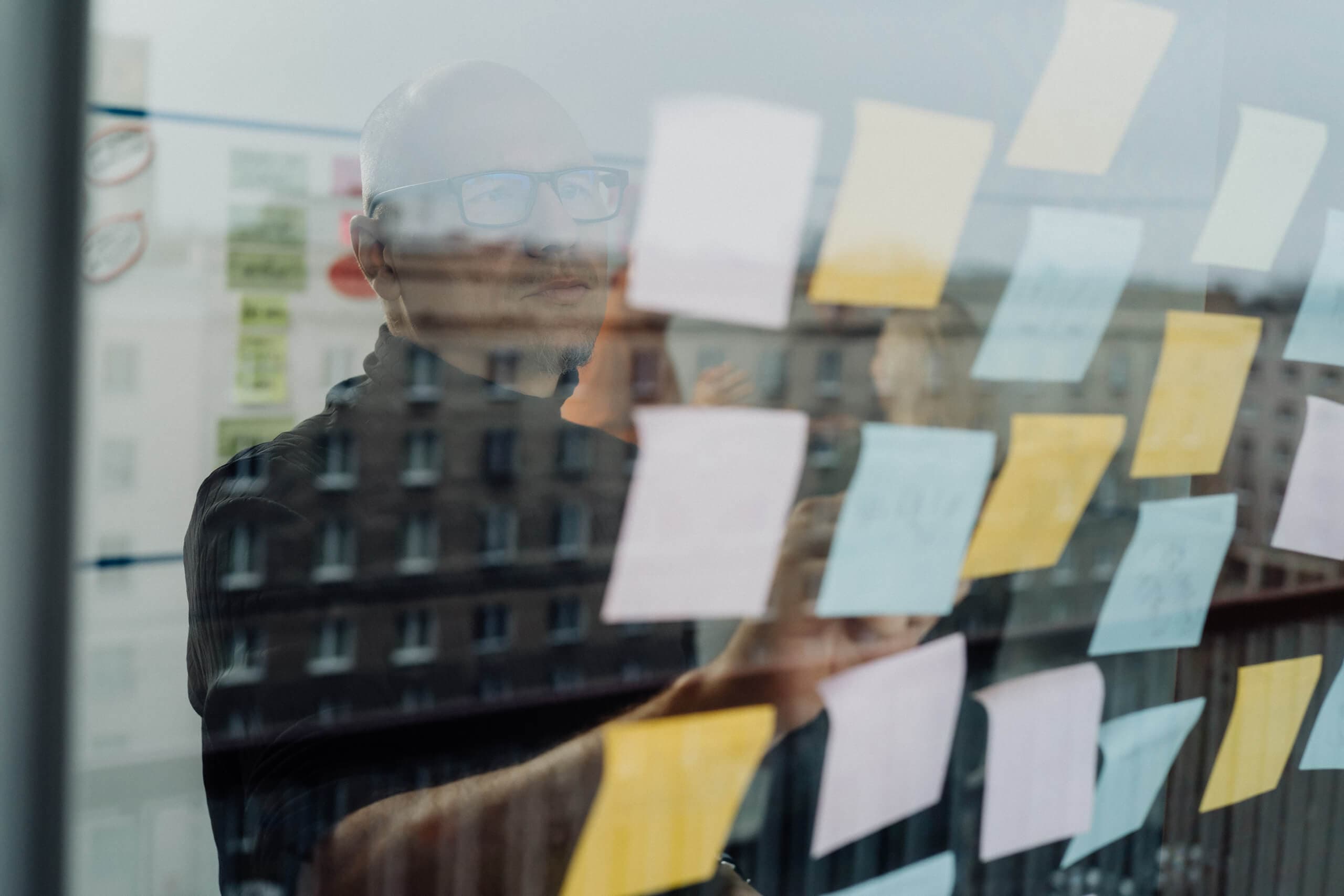
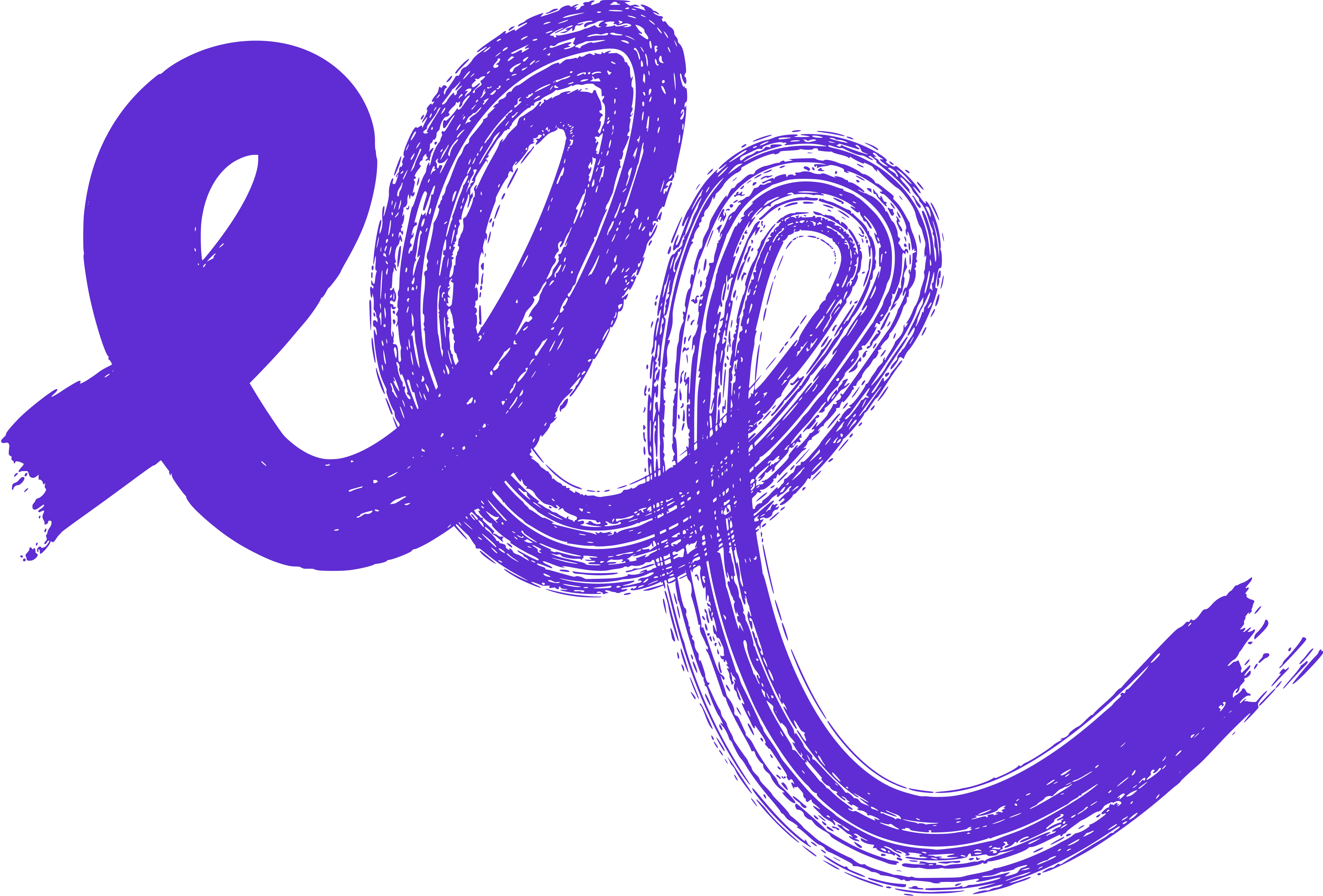
You may also
like...
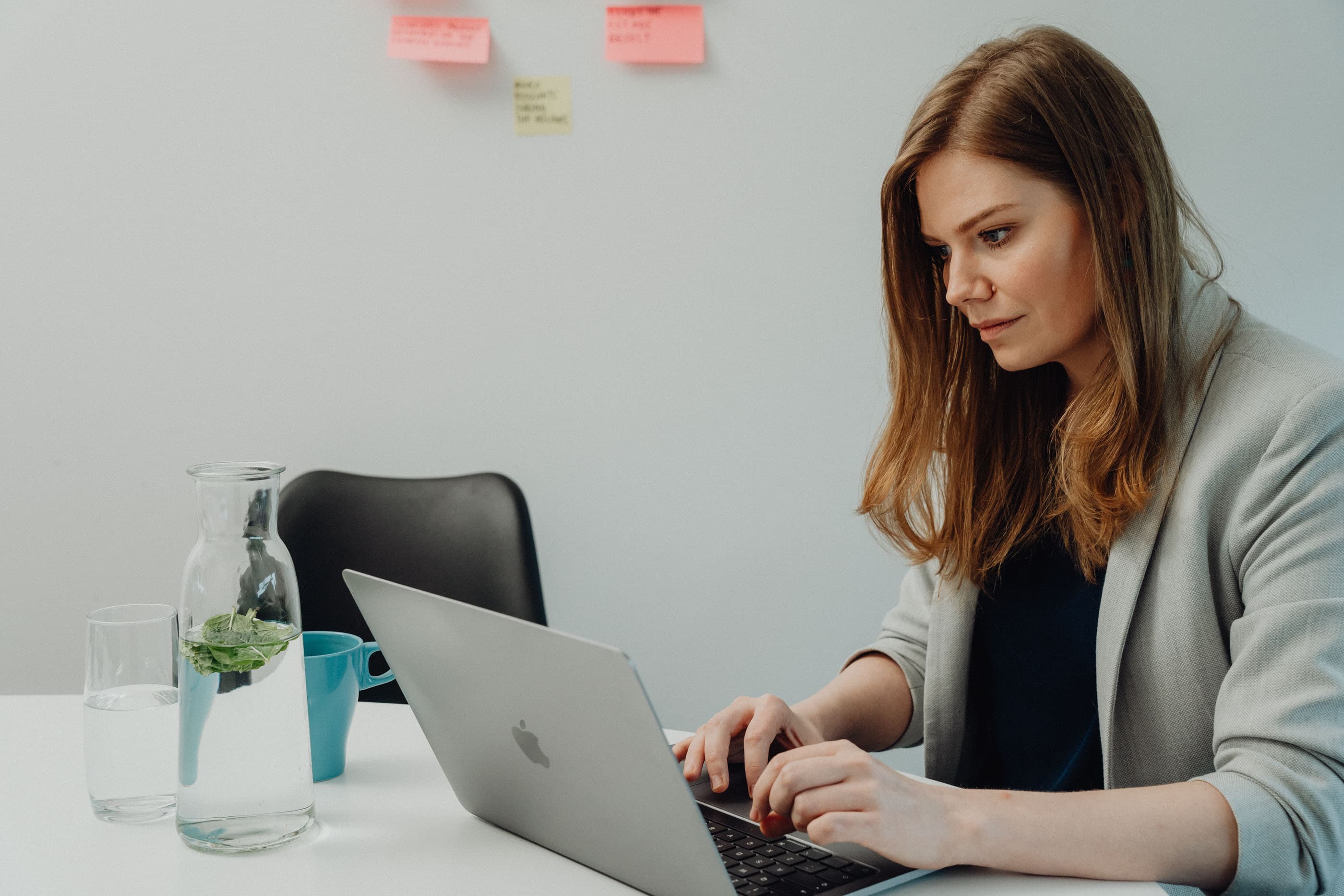
Understanding Event-Driven Programming: A Simple Guide for Everyone
Explore the essentials of event-driven programming. Learn how this responsive paradigm powers interactive applications with real-world examples and key concepts.
Marek Pałys
Apr 30, 2024・9 min read
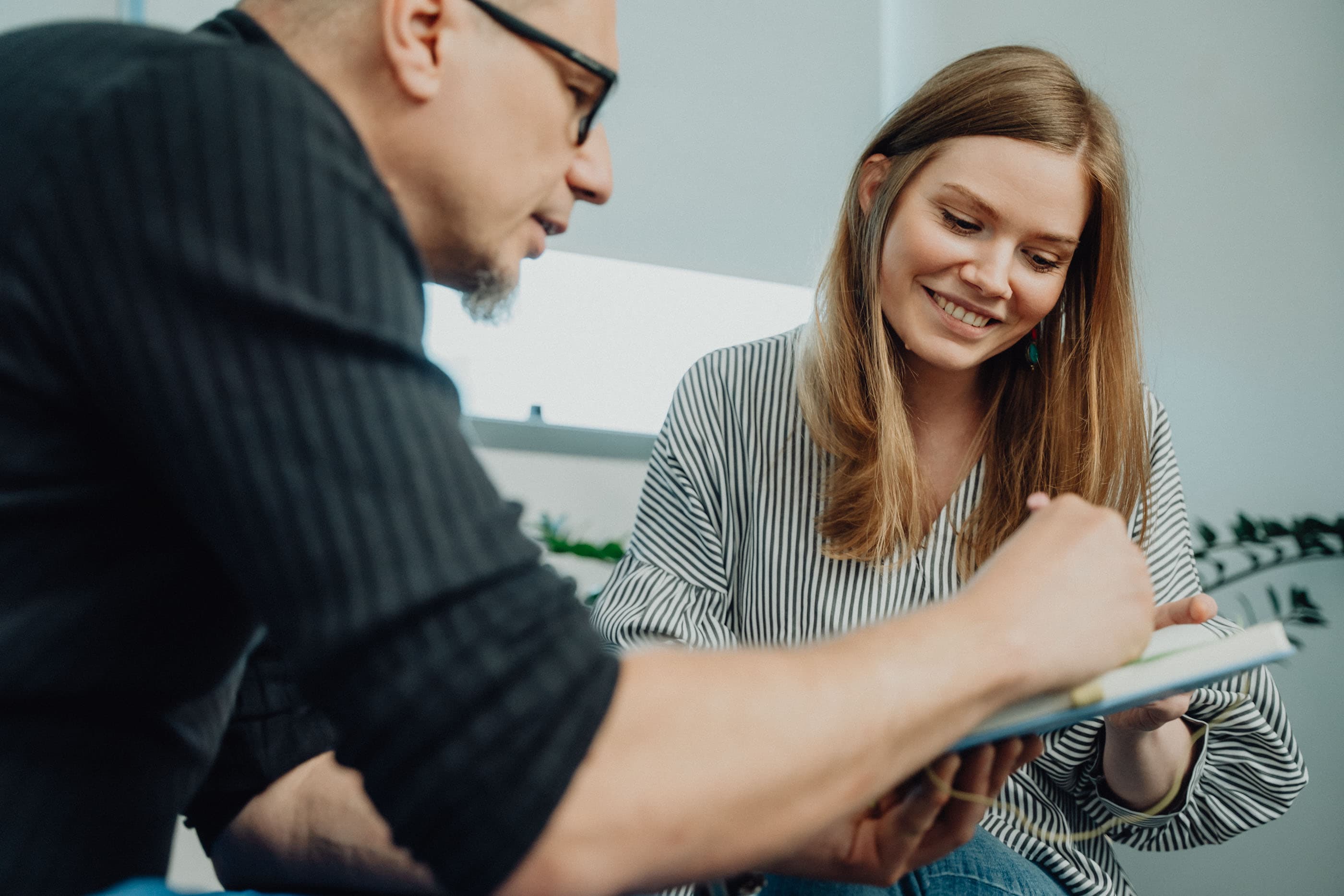
Navigating the Cloud: Understanding SaaS, PaaS, and IaaS
Discover the differences between SaaS, PaaS, and IaaS in cloud computing. This guide explains each model, their benefits, real-world use cases, and how to select the best option to meet your business goals.
Marek Pałys
Dec 12, 2024・11 min read
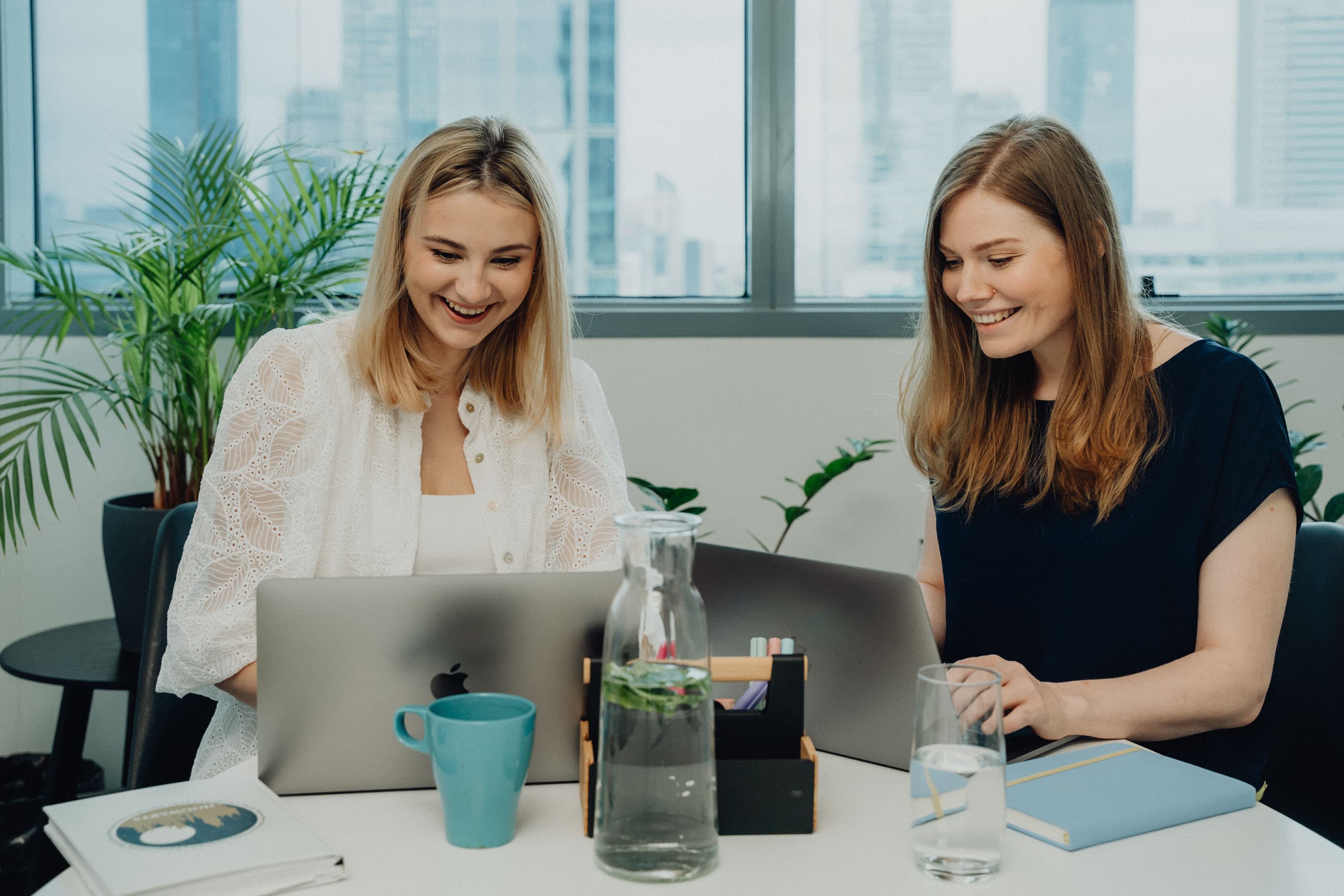
Cypress or Selenium: Making the Right Choice for Your Testing Needs
Cypress and Selenium are leading automated testing tools for web applications. Cypress offers speed, real-time feedback, and ease of setup, while Selenium supports multiple languages, browsers, and platforms for broader testing. Choosing the right tool depends on your project scope, testing needs, and environment.
Alexander Stasiak
Nov 26, 2024・5 min read