🌍 All
About us
Digitalization
News
Startups
Development
Design
Getting Started with Meteor: A Beginner's Guide to Web Development
Marek Majdak
Sep 03, 2024・10 min read
Table of Content
Introduction to Meteor
Setting Up Your Environment
Core Concepts of Meteor
Building Your First Application
Advanced Meteor Features
Embarking on a journey into web development can be both exciting and daunting, especially for those just starting out. One of the tools that can simplify this process is the Meteor web framework, which offers a streamlined approach to building modern web applications. Meteor stands out due to its full-stack JavaScript platform, enabling developers to craft both the client and server sides of an application using a single language. This guide aims to introduce you to the essentials of Meteor, breaking down its fundamental concepts and demonstrating how it can serve as a robust foundation for your web development projects. Whether you're a seasoned coder looking to expand your toolkit, or a newcomer eager to dive into the world of serious web app development, Meteor provides a user-friendly entry point to create dynamic and responsive applications.
Introduction to Meteor
What is Meteor?
Meteor is a full-stack JavaScript framework designed to simplify the process of building web and mobile applications. Unlike many other frameworks, Meteor allows you to use JavaScript for both the front-end and back-end components of your application. This unified approach reduces the complexity often associated with using multiple technologies.
Meteor comes with several built-in features, such as live reloading and a powerful data layer called Minimongo, which mimics MongoDB on the client side. This makes it easier to manage real-time data updates between the client and server. Additionally, Meteor integrates seamlessly with popular front-end libraries and frameworks like React and Angular, giving developers flexibility in their technology stack.
In essence, Meteor streamlines the development workflow, making it an excellent choice for both beginners and experienced developers looking for efficiency and simplicity in their projects.
Benefits of Using Meteor
Meteor offers several advantages that make it an appealing choice for web developers. One of its primary benefits is the ability to use a single programming language, JavaScript, across the entire application stack. This reduces the learning curve and allows developers to focus on building features rather than managing multiple languages and frameworks.
Another significant advantage is Meteor's real-time data capabilities. With built-in support for live data updates, applications remain responsive and engaging without requiring additional coding for synchronisation. This is particularly beneficial for mobile apps that demand real-time user interaction, such as chat applications or collaborative tools.
Meteor also simplifies deployment. With platforms like Galaxy, a cloud service tailored for Meteor applications, developers can easily deploy, scale, and manage their apps. This reduces the time and effort required to go from development to production.
Overall, Meteor's integrated approach and robust features make it a valuable tool for anyone looking to streamline their web development process.
Meteor vs Other Frameworks
When comparing Meteor to other popular frameworks like Angular, React, or Vue, its full-stack capabilities stand out. While frameworks like Angular focus mainly on the front-end, Meteor offers a complete package, handling both client-side and server-side operations. This full stack framework means you don't need to piece together various technologies to build a functioning application.
Another distinguishing feature is Meteor's live data updates. While other frameworks may require additional libraries or manual coding to achieve real-time functionality, Meteor provides this out of the box. This capability makes it particularly useful for apps that need instantaneous data changes or synchronisation.
Moreover, Meteor's ease of integration with other libraries gives it an edge in flexibility. Developers can seamlessly incorporate React or Angular for the front-end of meteor apps, gaining the benefits of these libraries while still leveraging Meteor's back-end strengths.
In summary, Meteor provides a more unified and efficient development experience compared to frameworks that focus on a specific part of the application stack.
Setting Up Your Environment
Installing Meteor
Installing Meteor is a straightforward process, making it accessible even for beginners. To get started, you need to ensure that you have Node.js and npm (Node Package Manager) installed on your system. Meteor requires these tools to manage dependencies and run its environment.
Once Node.js and npm are set up, you can install Meteor by running a simple command in your terminal or command prompt. For Windows, MacOS, and Linux, the installation command is:
curl https://install.meteor.com/ | sh
This command downloads and runs a script that installs Meteor and its dependencies. The process is usually quick and painless, setting up everything you need to start developing with Meteor.
After installation, you can verify that Meteor is correctly installed by typing meteor --version in your terminal. This should display the version number, confirming that Meteor is ready for use. With Meteor installed, you're now ready to create your first Meteor project.
Creating Your First Project
Once Meteor is installed, creating your first project is a breeze. Meteor's command-line tool simplifies the process, allowing you to set up a new project with minimal effort. To create a new Meteor application, open your terminal or command prompt and navigate to the directory where you want to store your project. Then, run the following command:
meteor create my-first-meteor-app
Replace my-first-meteor-app with your desired project name. This command generates a new directory with the basic structure of a Meteor application, including default files and folders. It provides you with a starting point, complete with a simple example to help you understand the framework's fundamentals.
To see your application in action, navigate into your project directory and start the development server by typing:
cd my-first-meteor-app meteor
Open a web browser and go to http://localhost:3000 to view your first Meteor app running. This quick setup allows you to dive straight into app development.
Understanding the Folder Structure
Understanding the folder structure of a Meteor project is crucial for effective development. When you create a new Meteor application, several key directories and files are generated by default. At the top level, you'll find the client, server, and imports folders, among others.
The client directory is where you place code that runs on the user's browser. This includes HTML, CSS, and client-side JavaScript files. It's responsible for the user interface and user interactions.
The server directory contains code that runs on the server. This is where you'll handle data processing, business logic, and any server-specific operations.
The imports directory is optional but recommended for organising code. By placing files here, you maintain a clear structure and take advantage of Meteor's module system, which supports lazy loading.
This structure helps separate concerns and keeps your codebase organised, making it easier to manage and scale your application as it grows.
Core Concepts of Meteor
Blaze Templates
Blaze is Meteor's built-in templating engine, designed to simplify the creation of dynamic user interfaces. Blaze allows you to define templates using HTML, which can then be populated with data and rendered reactively. This means any changes in the underlying data automatically update the user interface without requiring manual DOM manipulation.
A typical Blaze template consists of an HTML file where you define the template structure and a JavaScript file where you specify the template's behaviour. For example:
<template name="hello"> <h1>Hello, {{name}}!</h1> </template>
In the corresponding JavaScript file, you can define the data context:
Template.hello.helpers({ name() { return "World"; } });
This simplicity makes Blaze an excellent choice for beginners. It integrates seamlessly with Meteor's reactive data system, ensuring that your applications remain responsive and interactive. While Meteor supports other front-end frameworks like React and Angular, Blaze remains a straightforward and powerful option for building Meteor applications.
Collections and Data Management
In Meteor, collections are a fundamental concept for managing data. They serve as the primary mechanism for storing and retrieving data within your application. Meteor collections are built on top of MongoDB, a popular NoSQL database, enabling seamless integration and real-time data synchronisation.
To create a collection, you simply use the Mongo.Collection constructor in your JavaScript code. For example:
Tasks = new Mongo.Collection('tasks');
With collections, you can perform various operations such as inserting, updating, and removing data. These operations automatically propagate changes to all clients connected to your application, thanks to Meteor's reactivity.
Meteor also provides a mini version of MongoDB, called Minimongo, which runs on the client side. This allows for quick data access and manipulation without relying on server round-trips. The use of collections and reactivity simplifies data management, making Meteor an efficient choice for building applications that require up-to-date and responsive data handling.
Methods and Publications
Methods and publications are key components of data interaction in Meteor. Methods are functions that run on the server and allow clients to request changes to the database. They ensure data integrity by validating inputs and encapsulating business logic. For instance, a method to add a task might look like this:
Meteor.methods({ 'tasks.insert'(text) { check(text, String); Tasks.insert({ text, createdAt: new Date() }); } });
Publications, on the other hand, control the data that the server sends to the client. They define which subsets of data from the database clients can subscribe to. A simple publication might look like this:
Meteor.publish('tasks', function() { return Tasks.find(); });
Clients use Meteor.subscribe to access published data. This system allows for fine-grained control over data flow, ensuring efficient use of resources and maintaining data security. By using methods and publications, Meteor developers can build scalable and secure applications with responsive data handling.
Building Your First Application
Designing the User Interface
Designing the user interface (UI) is a crucial step in building a Meteor application. It involves creating a layout that is both functional and aesthetically pleasing. With Meteor, you can use Blaze, React, or Angular to construct your UI, offering flexibility in design choices.
Start by defining the structure of your UI using templates. These templates will serve as blueprints for your views. For instance, if you're using Blaze, you can create reusable components like navigation bars or footers, enhancing consistency across your application. Maintain a clean separation between your HTML, CSS, and JavaScript to ensure clarity and ease of maintenance.
Incorporate reactive data sources to keep your UI up-to-date with minimal effort. This means changes in data automatically reflect in the UI, providing a seamless user experience. Finally, pay attention to responsive design principles to ensure your application looks good on all devices. This comprehensive approach to UI design makes your Meteor app both user-friendly and robust.
Implementing Functionality
Once the user interface for simple app is in place, the next step is to implement the application's functionality. In Meteor, this involves using JavaScript to define interactive behaviours and handle user events. For example, you might want to add the ability for users to submit forms, click buttons, or navigate through different views.
Begin by setting up event handlers within your templates. These handlers respond to user actions and trigger the necessary logic. For instance, if a user submits a form, an event handler can capture the input, validate it, and call a Meteor method to update the database.
Additionally, leverage Meteor's reactive data sources to keep your application interactive. Use helpers to manage data context and ensure that changes in data automatically update the UI.
By focusing on clear and modular code, you can efficiently implement functionality that enhances user experience. This approach same code not only makes your application more robust but also easier to maintain and extend in the future.
Testing and Debugging
Testing and debugging are essential aspects of building any robust Meteor application. They ensure that your application functions correctly and provides a smooth user experience. Start by writing unit tests for individual components, such as methods and helpers, to verify their behaviour in isolation. Meteor integrates well with testing frameworks like Mocha and Jasmine, allowing you to automate this process efficiently.
In addition to unit testing, perform integration tests to check how different parts of your application work together. This helps identify issues that may arise from component interactions.
Debugging is equally important. Use Meteor's built-in tools and browser developer tools to inspect code, monitor network activities, and trace errors. Console logging is also a valuable technique to gain insights into your application's state during execution.
By systematically testing and debugging, you can identify and fix issues early in the development cycle, improving the reliability and performance of key features of your Meteor application. This proactive approach leads to a more stable and user-friendly product.
Advanced Meteor Features
Working with Packages
Meteor's package system is a powerful feature that allows developers to extend their applications with additional functionality. The Meteor ecosystem includes a wide range of packages that can be easily integrated into your project, from UI components to back-end services.
To add a package, use the Meteor command-line tool with a simple command. For instance, to add a package for user accounts, you might use:
meteor add accounts-ui accounts-password
This command installs the specified packages and makes them available in your application. Once added, the packages can be utilised in your code to simplify common tasks, such as authentication or data visualisation.
Additionally, you can create custom packages for reusable code across multiple projects, promoting code reusability and consistency.
Meteor's package system facilitates rapid development by reducing the amount of code you have to write from scratch. It also keeps your application modular, making it easier to maintain and scale over time.
Deployment Strategies
Deploying a Meteor application involves transferring your local development setup to a production environment where users can access it. Meteor offers several deployment strategies, giving you flexibility based on your project's needs and resources.
One straightforward option is deploying to Meteor's Galaxy hosting service. Galaxy is optimised for Meteor applications, offering seamless scaling and management tools. With simple commands, you can deploy your app to Galaxy and benefit from its robust infrastructure.
Alternatively, you can deploy your Meteor app to platforms like Heroku, AWS, or DigitalOcean. These platforms may require additional configuration, such as setting up a MongoDB instance and environment variables. However, they offer more control and potentially lower costs depending on your usage.
For smaller projects or testing purposes, you can also use free hosting services like Meteor's own free tier, although this may come with limitations on resources and uptime.
Selecting the right deployment strategy depends on your specific requirements for scalability, cost, and control.
Scaling Your Application
Scaling a Meteor application involves ensuring it can handle increased loads as your user base grows. One of the first steps in scaling is optimising your application's performance. This can be achieved by minimising database queries, using indexes effectively, and reducing the amount of data sent to clients.
Meteor's reactivity can also impact scalability. While it provides real-time updates, it can lead to performance bottlenecks if not managed properly. Consider using methods and publications judiciously to limit unnecessary data updates and only subscribe to data that's essential for the user.
For horizontal scaling issues, deploying your application on a cloud service that supports load balancing, like AWS or Galaxy, can distribute traffic across multiple instances. This approach ensures that no single server becomes a bottleneck.
Additionally, consider implementing a microservices architecture to further distribute the workload. By breaking your application into smaller, independent services, you can optimise each part for performance and scalability, enhancing the overall robustness of your application.
FAQs
- What is Meteor? Meteor is a full-stack JavaScript framework that allows developers to build both client and server-side applications using a single language.
- How is Meteor different from other frameworks? Unlike most frameworks, Meteor handles both front-end and back-end operations, simplifying the development process with real-time data updates.
- What makes Meteor a good choice for beginners? Meteor offers an easy setup, unified language, and built-in features like live data updates, making it accessible for new developers.
- Can I use React or Angular with Meteor? Yes, Meteor integrates seamlessly with React, Angular, and other front-end libraries for flexible application development.
- What is the main advantage of using Meteor for web development? Meteor allows developers to use JavaScript across the entire stack, reducing the complexity of managing multiple languages and frameworks.
- How do I install Meteor on my system? You can install Meteor by running a simple terminal command: curl https://install.meteor.com/ | sh.
- What are Blaze templates in Meteor? Blaze is Meteor's built-in templating engine, enabling developers to build dynamic and reactive user interfaces easily.
- How do Meteor's collections work? Meteor collections are built on MongoDB and allow real-time data management between the client and server.
- What are Meteor methods? Meteor methods are server-side functions that allow clients to interact with the database and perform data operations.
- How does Meteor handle data updates in real-time? Meteor automatically synchronizes data between the client and server using its reactive data system.
- Is Meteor good for building mobile apps? Yes, Meteor supports mobile app development by leveraging Apache Cordova to build hybrid applications.
- How can I scale a Meteor application? You can scale a Meteor application by using cloud services like Galaxy or AWS, which support load balancing and horizontal scaling.
- What is Minimongo in Meteor? Minimongo is a lightweight version of MongoDB that runs on the client side to provide fast, reactive data access.
- How do I deploy a Meteor app? You can deploy a Meteor app to platforms like Galaxy, Heroku, AWS, or DigitalOcean, depending on your scalability needs.
- What is Meteor's Galaxy service? Galaxy is a cloud platform specifically designed for deploying and scaling Meteor applications with ease.
- Can I use packages in Meteor? Yes, Meteor has a package system that allows you to easily add functionality like user authentication, data visualization, and more.
- What is the default folder structure in Meteor? A Meteor project has a clear structure with separate directories for client-side, server-side, and shared code.
- How does Meteor manage reactive data? Meteor's reactivity system automatically updates the UI when data changes, providing a smooth user experience.
- What are publications and subscriptions in Meteor? Publications define the data the server sends to the client, while subscriptions allow clients to request specific data sets.
- Is Meteor suitable for large-scale applications? Yes, with proper scaling strategies, Meteor can handle large applications by optimizing data flow and using cloud services for scalability.
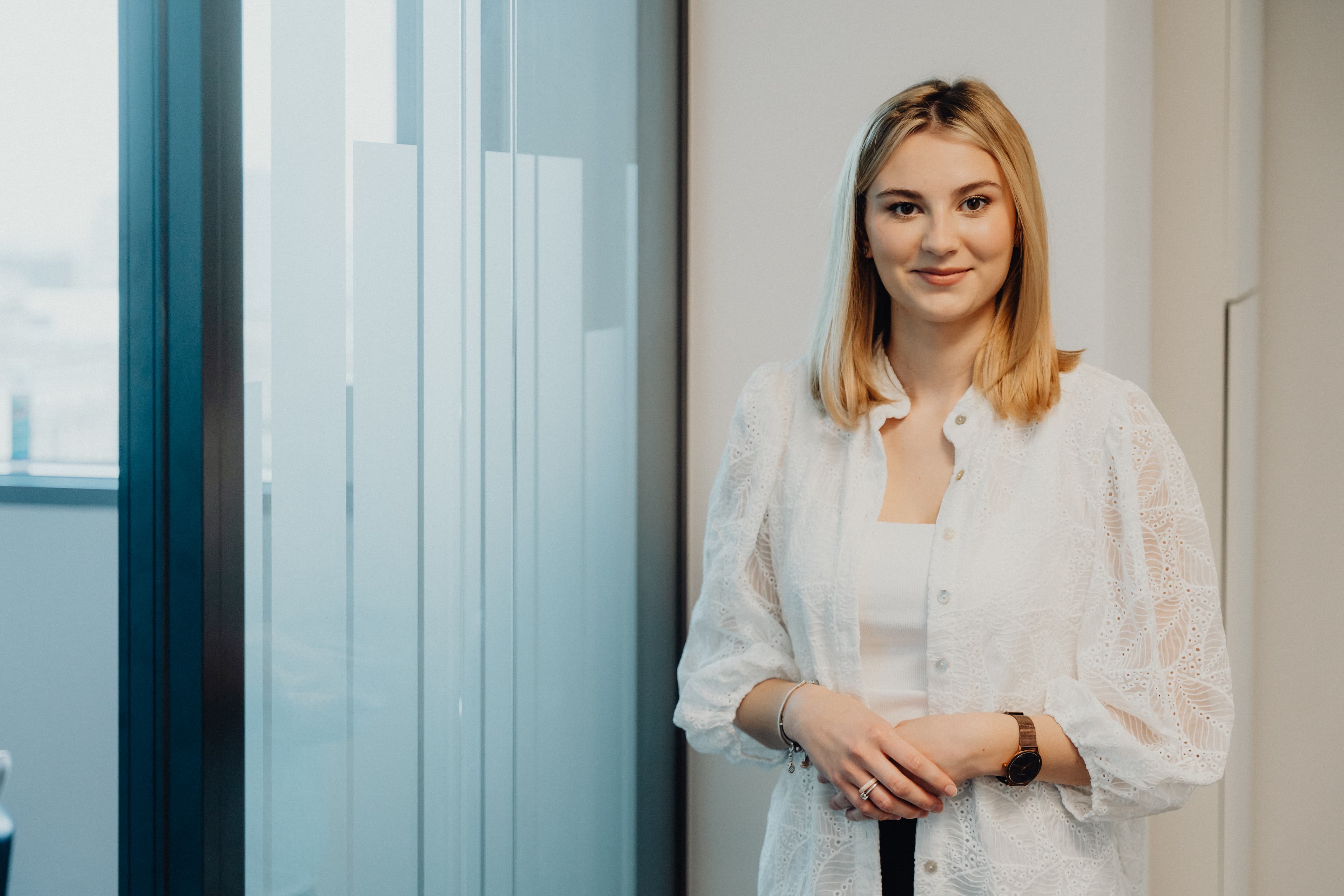
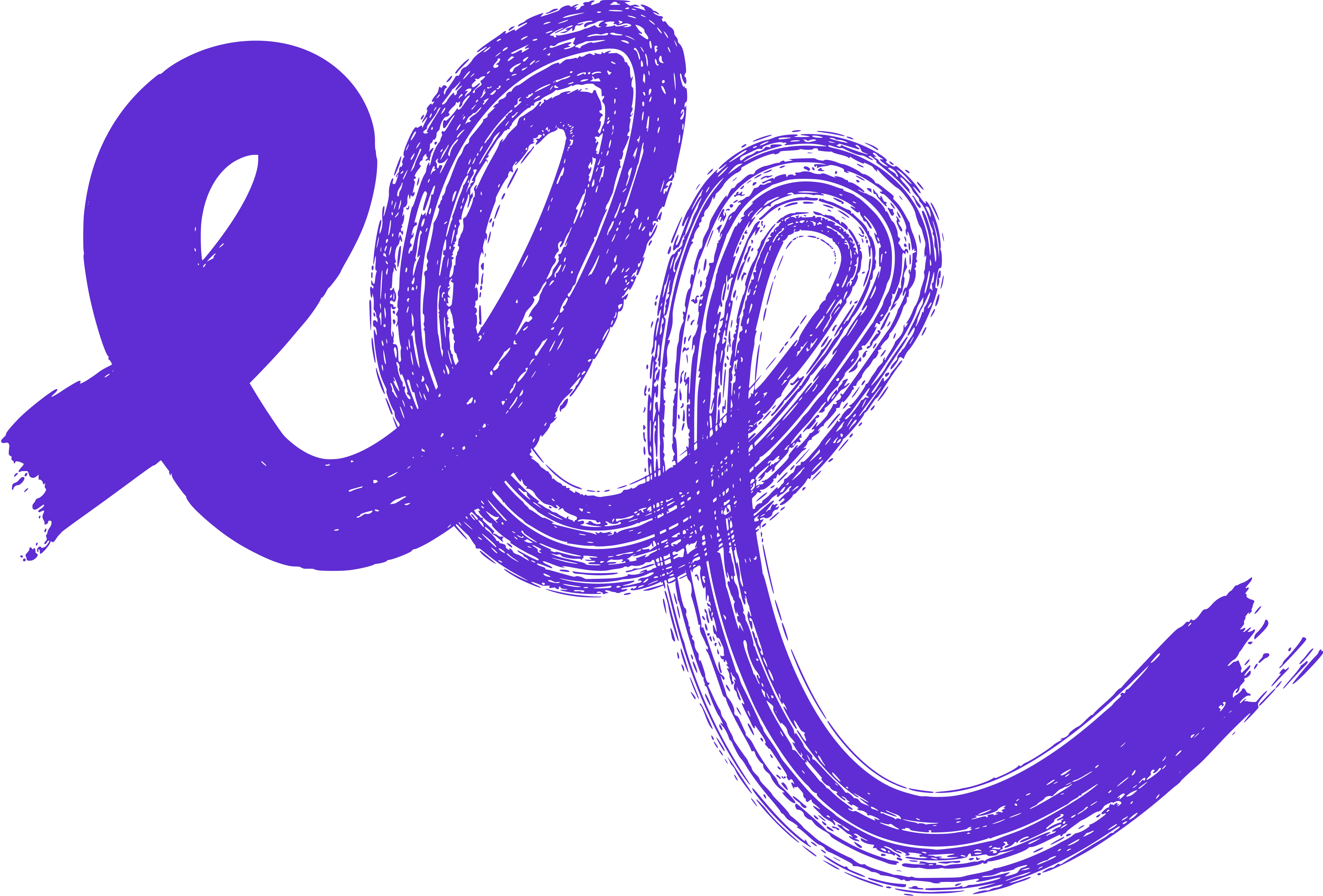
You may also
like...

Understanding Why Your App Rendered More Hooks Than During the Previous Render
This article explores the reasons why your application might render more hooks than during the previous render, a common issue faced by developers. By understanding the mechanics of React hooks and their rendering process, you'll gain practical insights to diagnose and resolve this problem. Enhancing your app's performance and stability is crucial for delivering a seamless user experience.
Marek Majdak
Apr 29, 2024・12 min read
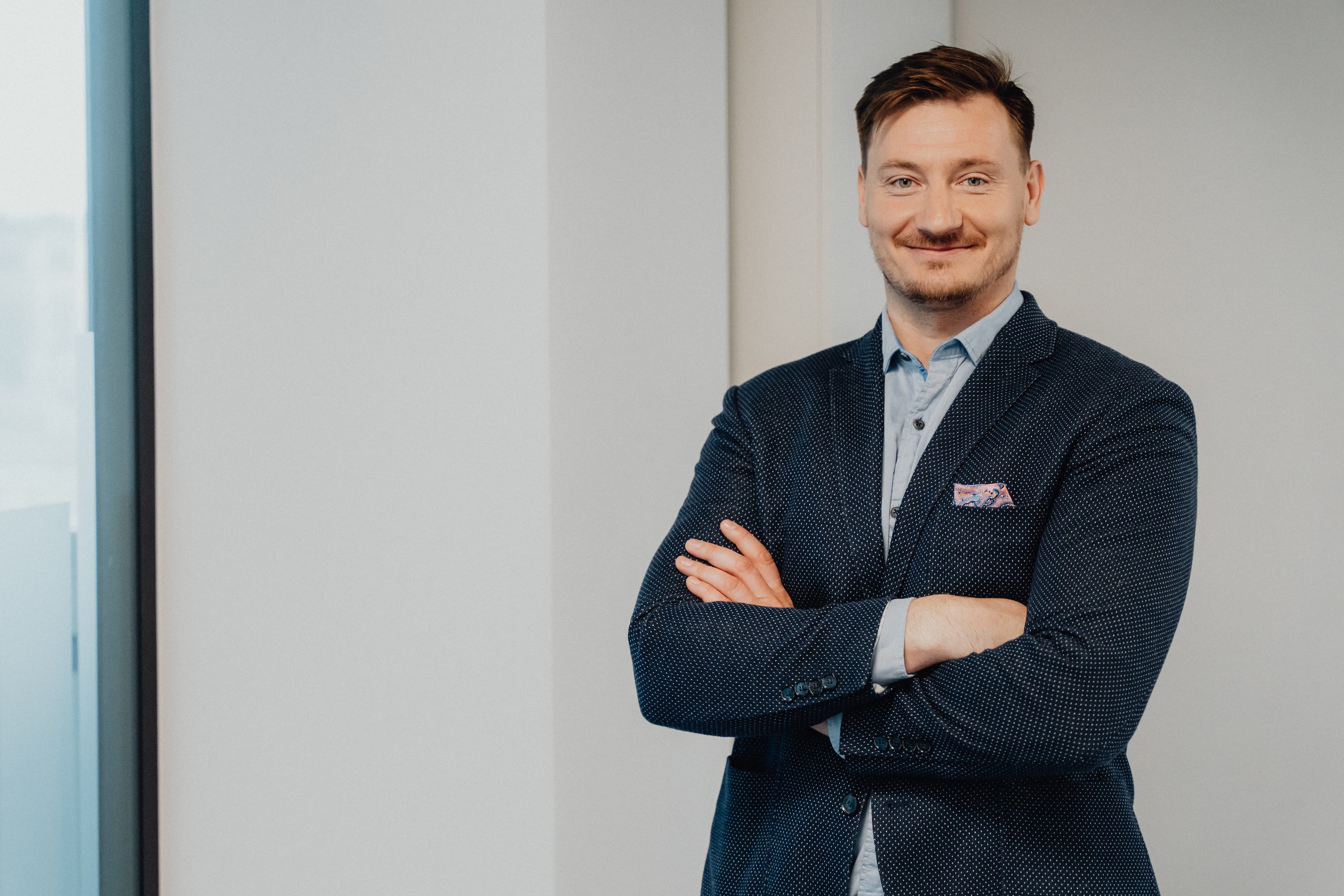
A Clear Perspective: The Python Language Advantages and Disadvantages
Python has emerged as one of the most popular programming languages, valued for its simplicity and versatility. This article examines its key advantages, such as ease of learning and extensive libraries, alongside potential drawbacks, including performance limitations and challenges in mobile computing. Understanding these factors can help developers make informed choices about using Python for their projects.
Marek Majdak
Jul 16, 2024・7 min read
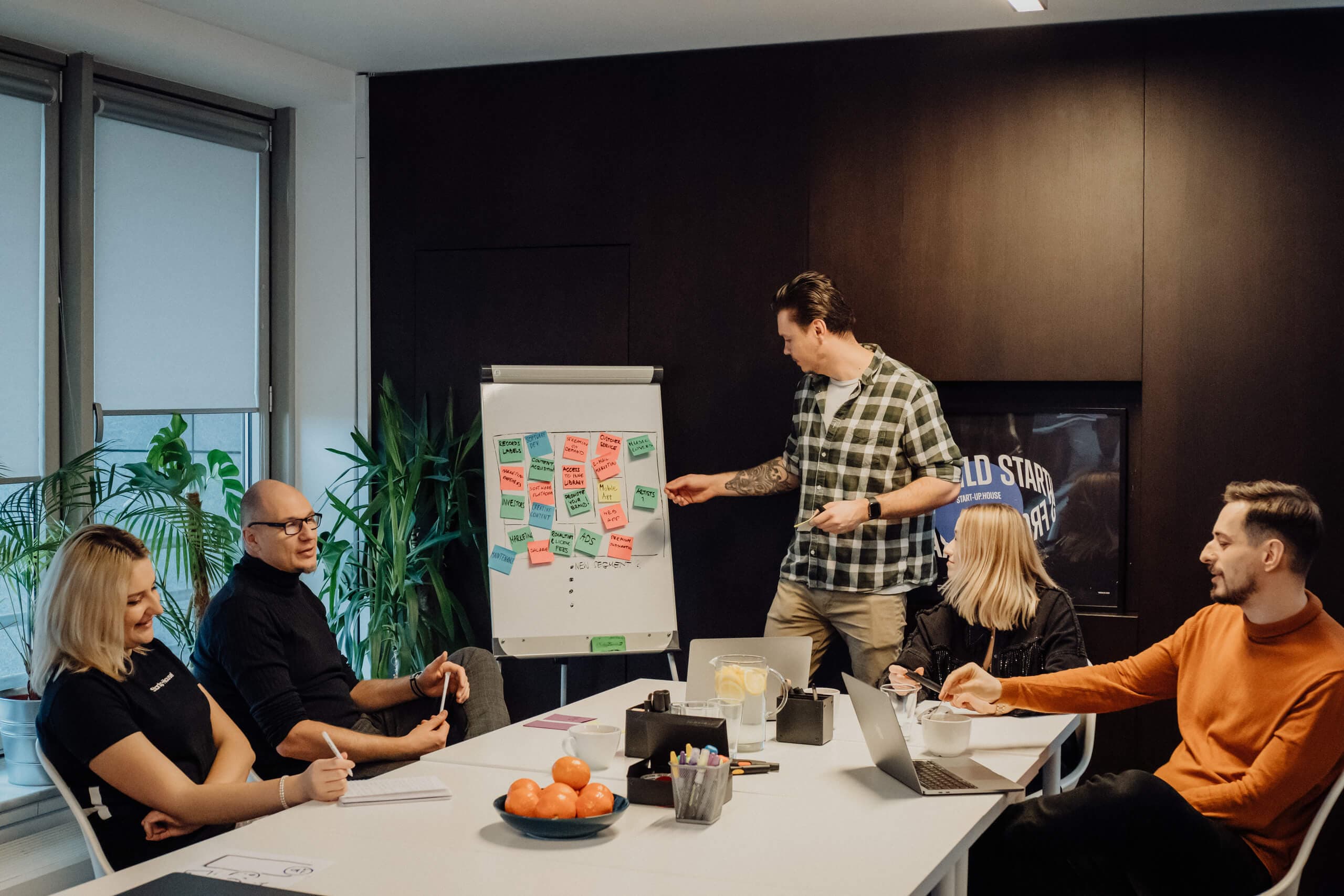
In-house vs Outsourcing Software Development: Making the Right Choice for Your Business
Choosing between in-house and outsourcing software development is a crucial decision that can significantly influence your business’s success. This article explores the distinct advantages and challenges of each approach, helping you assess your specific needs and make an informed choice. Whether you prioritize control and team cohesion or seek specialized skills and cost efficiency, understanding these factors is essential for strategic planning.
Alexander Stasiak
Aug 20, 2024・11 min read