🌍 All
About us
Digitalization
News
Startups
Development
Design
Mastering Dependency Injection in Python: Frameworks, Patterns, and Practical Insights
Alexander Stasiak
Feb 15, 2024・13 min read
Table of Content
FAQ
Dependency injection is more than just a trendy term; it's a design pattern that Python developers are increasingly adopting to improve code quality, flexibility, and testability. Python, as a versatile scripting language, makes implementing dependency injection straightforward and powerful.
What Is Dependency Injection?
Dependency injection (DI) is a design pattern where the dependencies of a class or function are defined and provided externally rather than being hardcoded. This pattern ensures that objects or components remain loosely coupled, making the application structure flexible and modular.
In Python, dependency injection works seamlessly thanks to its dynamic nature. Frameworks like Dependency Injector simplify the implementation of dependency injection patterns by offering DI containers, configuration file management, and constructor injection mechanisms.
Why Use Dependency Injection in Python?
- Improved Code Quality
Dependency injection reduces high coupling, ensuring your Python application adheres to the principles of high cohesion and inversion of control. - Enhanced Testing
With dependency injection, injecting mock dependencies for testing becomes simple, saving Python developers from rewriting all the code when testing new functionality. - Flexible Application Structure
DI frameworks allow you to define services and other objects in configuration files, making it easy to configure, reuse, and manage defined dependencies.
Implementing Dependency Injection in Python
Applying dependency injection in Python requires understanding DI frameworks and the dependency injection pattern. Below is a practical example using the Dependency Injector library:
from dependency_injector import containers, providers
class DatabaseService:
def __init__(self, db_url):
self.db_url = db_url
def connect(self):
return f"Connecting to {self.db_url}"
class AppContainer(containers.DeclarativeContainer):
config = providers.Configuration()
database_service = providers.Factory(DatabaseService, db_url=config.db_url)
# Configuration file
config = {"db_url": "sqlite:///:memory:"}
# Application structure
container = AppContainer()
container.config.from_dict(config)
db_service = container.database_service()
print(db_service.connect())
This snippet illustrates how dependency injection frameworks can create modular, loosely coupled components while maintaining high cohesion.
Practical Examples and Frameworks
Python’s ecosystem supports several DI frameworks to implement dependency injection effectively:
- Dependency Injector: A lightweight library for DI containers and configuration file management.
- Pinject: Ideal for Python developers who want simple dependency injections.
- DIpy: A beginner-friendly tool to inject dependencies into objects and functions.
FAQ
- How does dependency injection work in Python?
Dependency injection works in Python by defining dependencies externally and injecting them into components or objects during runtime. - What is a dependency injection framework?
A dependency injection framework simplifies applying dependency injection by providing tools like DI containers and configuration file management. - Why should Python developers use dependency injection?
Python developers should use dependency injection to improve code quality, reduce high coupling, and simplify testing. - What is the dependency injection pattern?
The dependency injection pattern is a design pattern where objects receive their dependencies from an external source. - What are defined dependencies in dependency injection?
Defined dependencies are the objects or components explicitly outlined for injection into a class or function. - What is a DI container?
A DI container is a component of dependency injection frameworks that helps manage and inject dependencies. - Can dependency injection in Python improve code quality?
Yes, dependency injection in Python enhances code quality by promoting loosely coupled and highly cohesive designs. - What is the purpose of constructor injection?
Constructor injection provides dependencies to objects during instantiation, ensuring modular and reusable application structures. - How do Python developers implement dependency injection?
Python developers implement dependency injection by using libraries like Dependency Injector or writing custom DI logic. - Is dependency injection suitable for all Python applications?
Dependency injection is suitable for Python applications requiring loosely coupled and testable components. - What is inversion of control in dependency injection?
Inversion of control refers to the principle of delegating control of object creation to a DI container or framework. - Are there dependency injection frameworks specific to Python?
Yes, frameworks like Dependency Injector and Pinject are designed specifically for dependency injection in Python. - What are practical examples of dependency injection?
Practical examples include injecting database connections, services, or configurations into Python applications. - How do configuration files support dependency injection?
Configuration files define dependencies and values that DI frameworks use to inject into application components. - What is a dependency injector in Python?
A dependency injector in Python is a tool or library that automates injecting dependencies into objects or classes. - What are the benefits of dependency injection frameworks?
Dependency injection frameworks provide DI containers, reduce boilerplate code, and streamline dependency management. - Can dependency injection reduce high coupling?
Dependency injection reduces high coupling by externalizing dependencies, allowing components to remain independent. - How does dependency injection enhance testing?
Dependency injection enhances testing by enabling the injection of mock dependencies without modifying production code. - What is a dependency injection container?
A dependency injection container manages and injects dependencies into classes or objects dynamically. - Why is dependency injection considered a silver bullet?
Dependency injection is considered a silver bullet for improving application structure, code quality, and testability in Python projects.
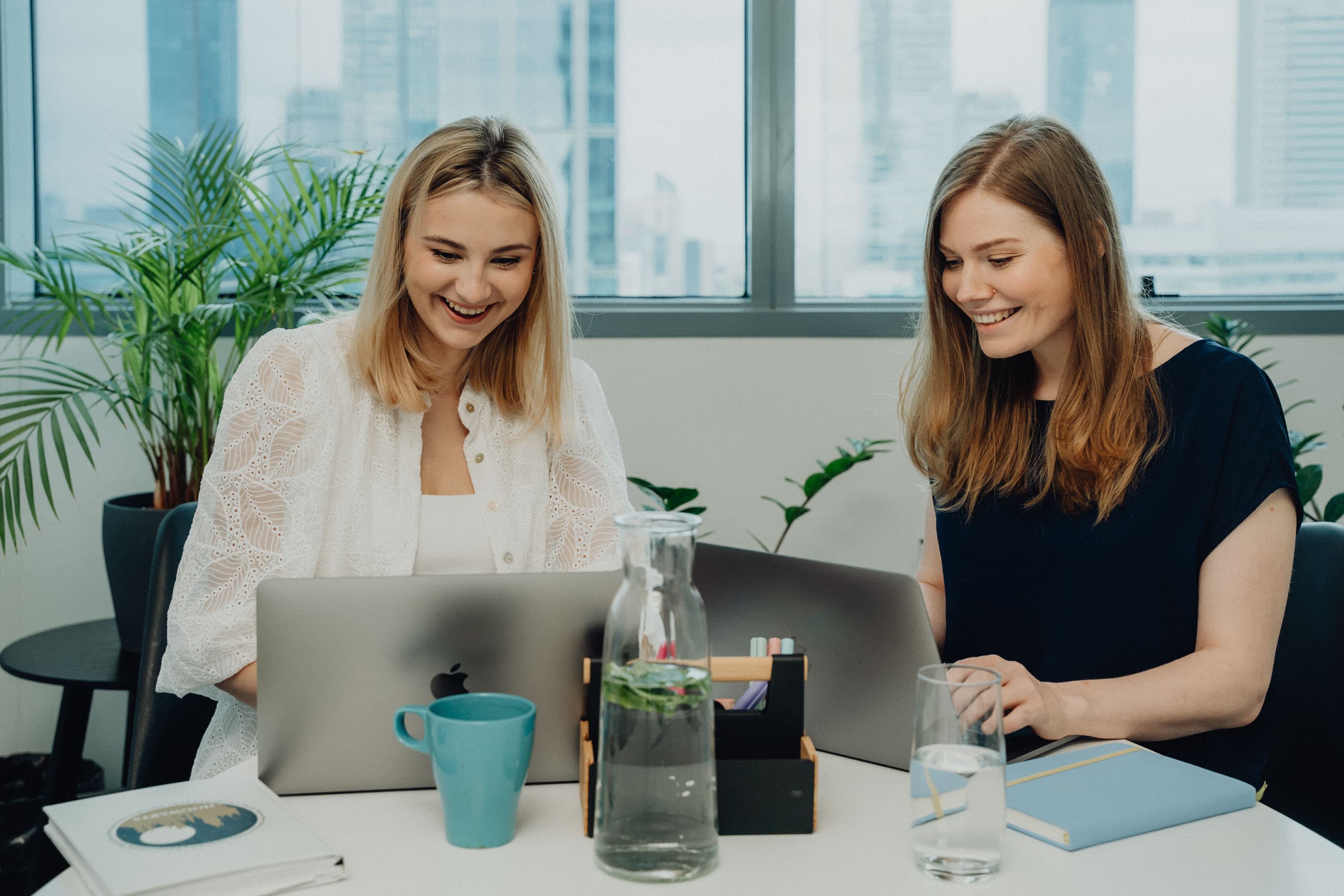
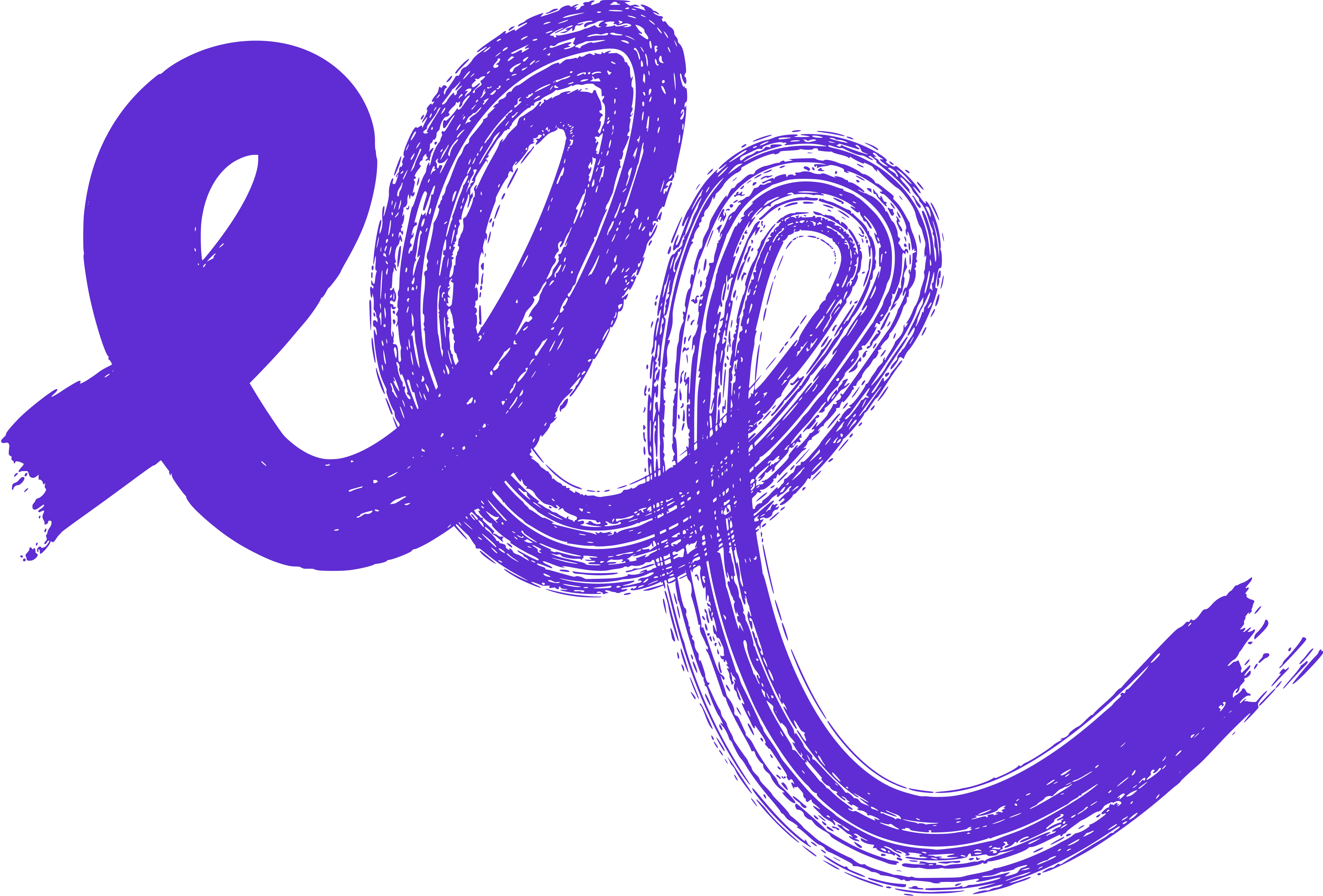
You may also
like...
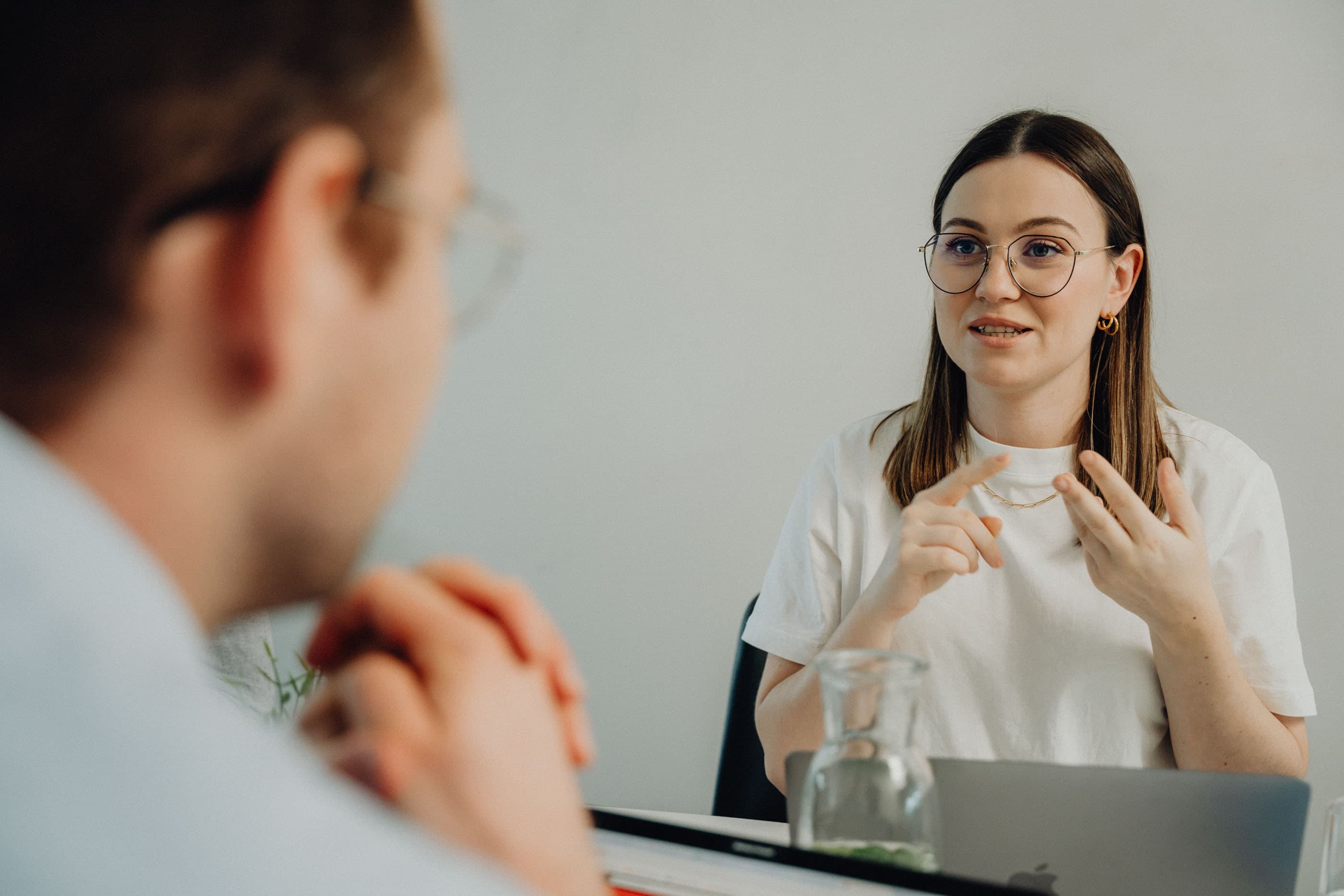
Choosing the Right Software Development Partner: A Practical Guide for Startups
The right software development partner can define your startup’s success. This guide shows how to choose one that fits your goals and needs.
Alexander Stasiak
Dec 30, 2024・10 min read
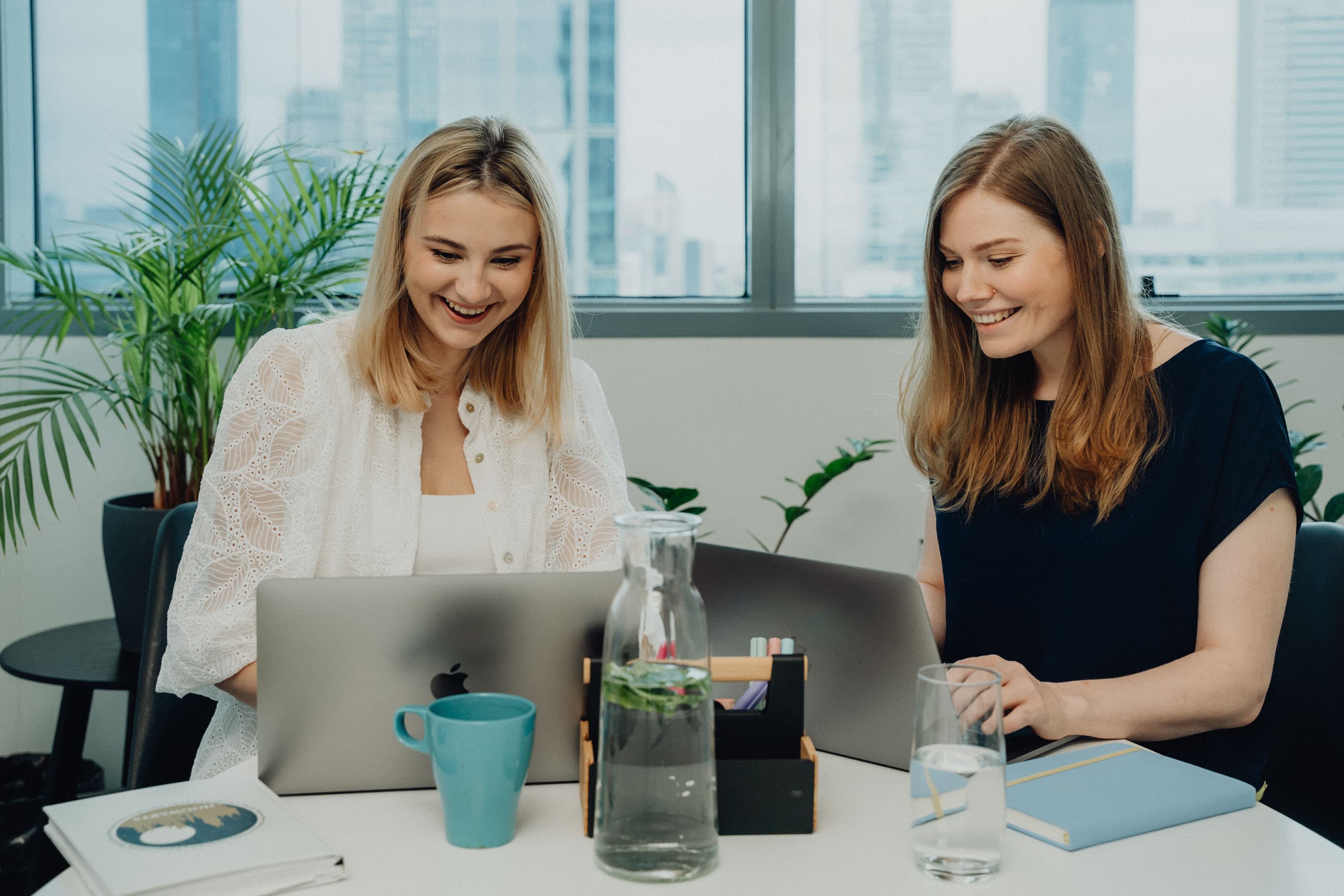
Mastering Declarative Programming: Essential Practices for Every Developer
Discover declarative programming essentials. This guide covers principles, tools, and best practices to simplify coding, enhance readability, and improve scalability.
Marek Pałys
Apr 16, 2024・11 min read
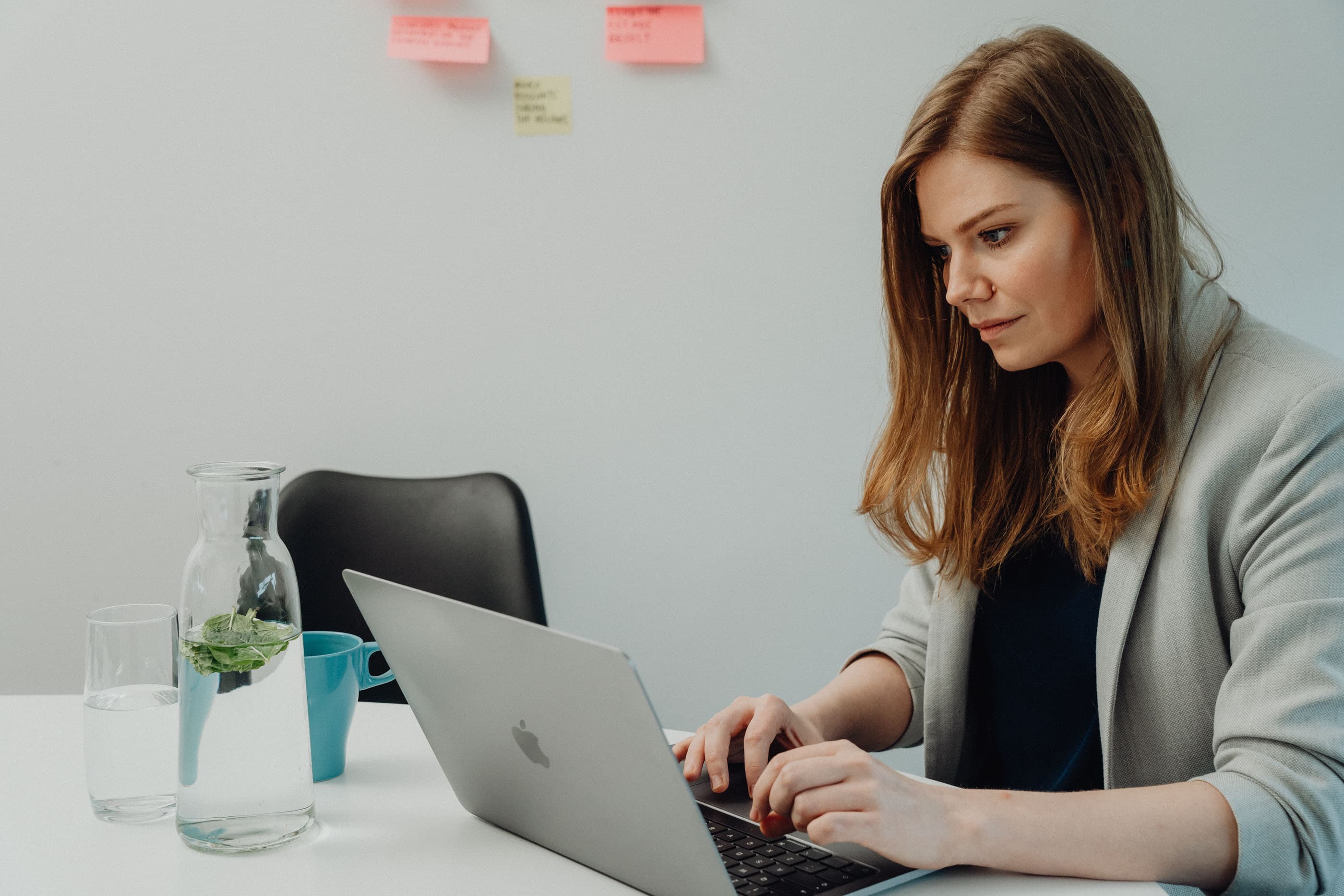
Understanding Event-Driven Programming: A Simple Guide for Everyone
Explore the essentials of event-driven programming. Learn how this responsive paradigm powers interactive applications with real-world examples and key concepts.
Marek Pałys
Apr 30, 2024・9 min read