🌍 All
About us
Digitalization
News
Startups
Development
Design
Data Validation with Pydantic: Simplify Your Python Data Models
Alexander Stasiak
Apr 12, 2024・14 min read
Table of Content
FAQ
Data validation is essential in Python applications to ensure accurate and reliable processing of user input and complex data structures. Pydantic, a Python library designed for defining and validating data models, offers a powerful solution by combining proper type checking, custom validators, and easy-to-read error messages. This blog post explores how Pydantic can transform your approach to data validation and parsing.
Why Use Pydantic for Data Validation?
Pydantic simplifies validating data by parsing input data against strict type annotations and raising informative validation errors when data types or structures don't match the defined schema. Whether you’re creating a user model or working with nested models, Pydantic ensures validated data aligns with expectations. By leveraging its powerful data validation capabilities, you can reduce errors, improve reliability, and enhance code quality in Python applications.
Key Features of Pydantic
- Data Models with Type Annotations
Pydantic models are Python classes that validate data based on type annotations. They ensure input data, such as integers, strings, or JSON files, conforms to the expected types and structures. - Default Values and Custom Validators
You can define default values for fields in your models and create custom validators to handle complex data structures, enabling flexibility and precision in data validation. - Nested Models for Complex Structures
Nested models allow you to validate data models containing other models, making it easy to parse and validate hierarchical data like JSON schemas. - User-Friendly Error Messages
When validation errors occur, Pydantic raises descriptive error messages that make debugging straightforward, saving developers time and effort. - ORM Mode for Database Integration
With ORM mode, Pydantic models can interact seamlessly with databases, simplifying data validation and serialization.
Example: Validating Data with Pydantic
Here’s a quick example of using Pydantic to validate input data:
from pydantic import BaseModel, ValidationError
class UserModel(BaseModel):
name: str
age: int
email: str
try:
user = UserModel(name="John Doe", age="twenty-five", email="john.doe@example.com")
except ValidationError as e:
print(e.json())
In this example, Pydantic raises a validation error for the age field because the input data is not a valid integer. The error message clearly indicates the issue, enabling developers to fix the input data or provide meaningful feedback to users.
Practical Applications of Pydantic
- Parsing JSON Files: Validate and parse complex data structures into Python objects.
- API Development: Ensure user input matches expected types in RESTful APIs.
- Database Operations: Validate data models before persisting them to databases.
- Configuration Management: Validate and parse configuration files with strict type checking.
FAQ
- What is data validation in Python?
Data validation ensures that input data matches the expected data types, structures, and values before processing it in Python applications. - How does Pydantic simplify data validation?
Pydantic simplifies data validation by combining type annotations, custom validators, and error messages for validated data. - What are Pydantic models used for?
Pydantic models are used for defining data models with proper type checking, validating data, and handling complex data structures. - How do you handle validation errors in Pydantic?
Validation errors in Pydantic are raised with detailed error messages that highlight issues in input data. - Can Pydantic validate nested models?
Yes, Pydantic can validate nested models, making it ideal for parsing complex data structures like JSON schemas. - What is the purpose of default values in Pydantic?
Default values in Pydantic allow you to define fields with fallback values when input data is missing. - How do you validate data models with Pydantic?
You validate data models with Pydantic by defining fields in a Pydantic model and passing input data to create a new instance. - What is the Pydantic import BaseModel?
The Pydantic import BaseModel is the foundation for creating Pydantic models, which validate data using type annotations. - What happens when Pydantic raises a validation error?
When Pydantic raises a validation error, it provides an error message detailing the issues in the input data. - How do you parse data with Pydantic?
You parse data with Pydantic by defining data models and using them to validate and structure input data into Python objects. - What is ORM mode in Pydantic?
ORM mode in Pydantic allows Pydantic models to work with object-relational mappers, validating data directly from databases. - Can you use Pydantic for JSON schemas?
Yes, Pydantic supports JSON schema generation and validation for complex data models. - What are custom validators in Pydantic?
Custom validators are helper functions in Pydantic that allow developers to define rules for validating data beyond type annotations. - Why is Pydantic considered a powerful data validation tool?
Pydantic is a powerful data validation tool because it combines strict type checking, nested models, and user-friendly error messages. - How do you validate user input with Pydantic?
You validate user input with Pydantic by creating a Pydantic model and passing user input as keyword arguments. - What is the use of Pydantic documentation?
Pydantic documentation provides guidelines, examples, and best practices for creating models and validating data. - How do you validate data types in Pydantic?
Pydantic validates data types by enforcing type annotations in Pydantic models and raising errors for mismatched types. - Can Pydantic handle complex data structures?
Yes, Pydantic handles complex data structures through nested models and custom validators. - What is the Pydantic import ValidationError?
The Pydantic import ValidationError allows developers to catch and handle validation errors when validating data models. - How do default values improve data validation in Pydantic?
Default values improve data validation in Pydantic by ensuring data models have fallback values when certain fields are not provided.
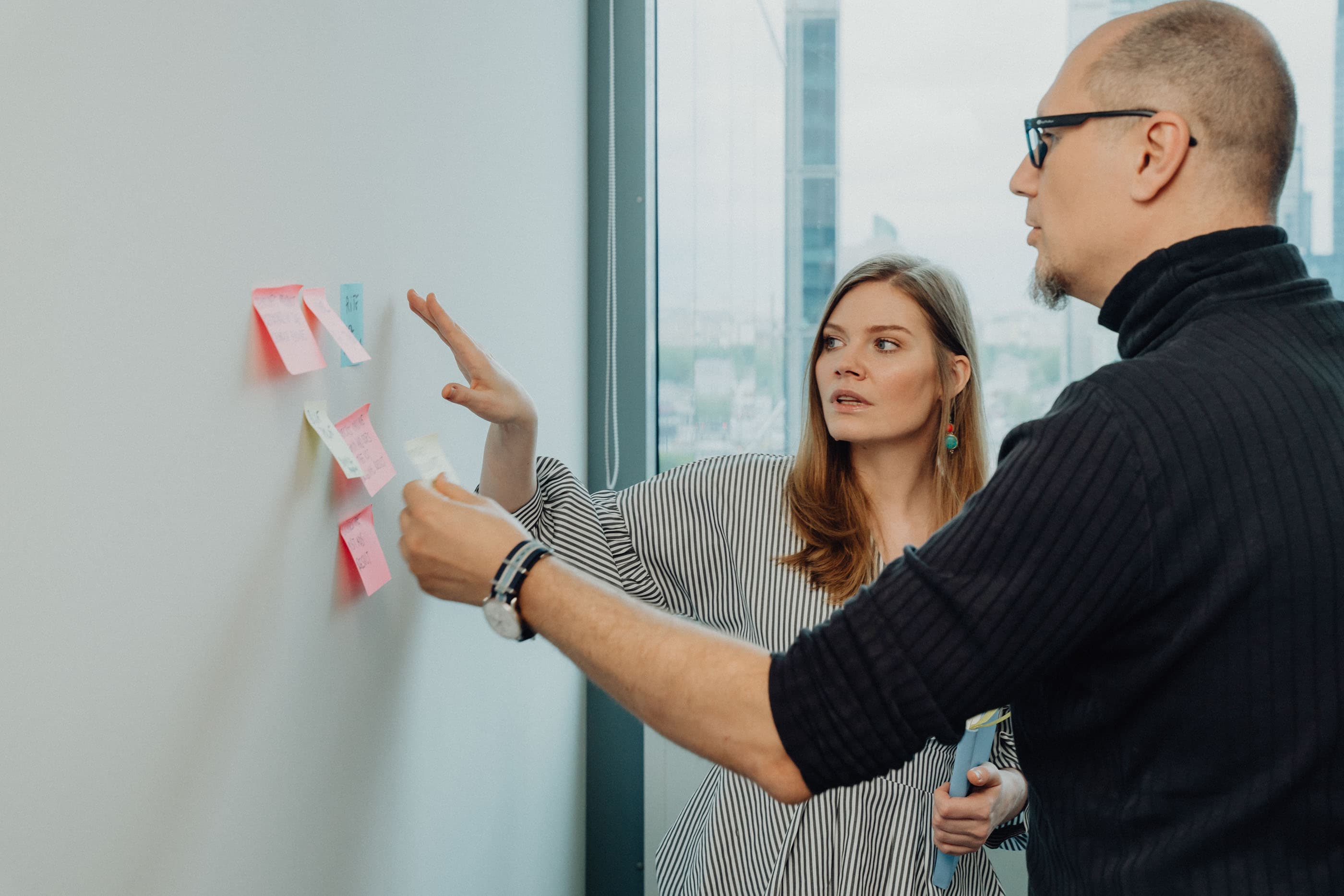
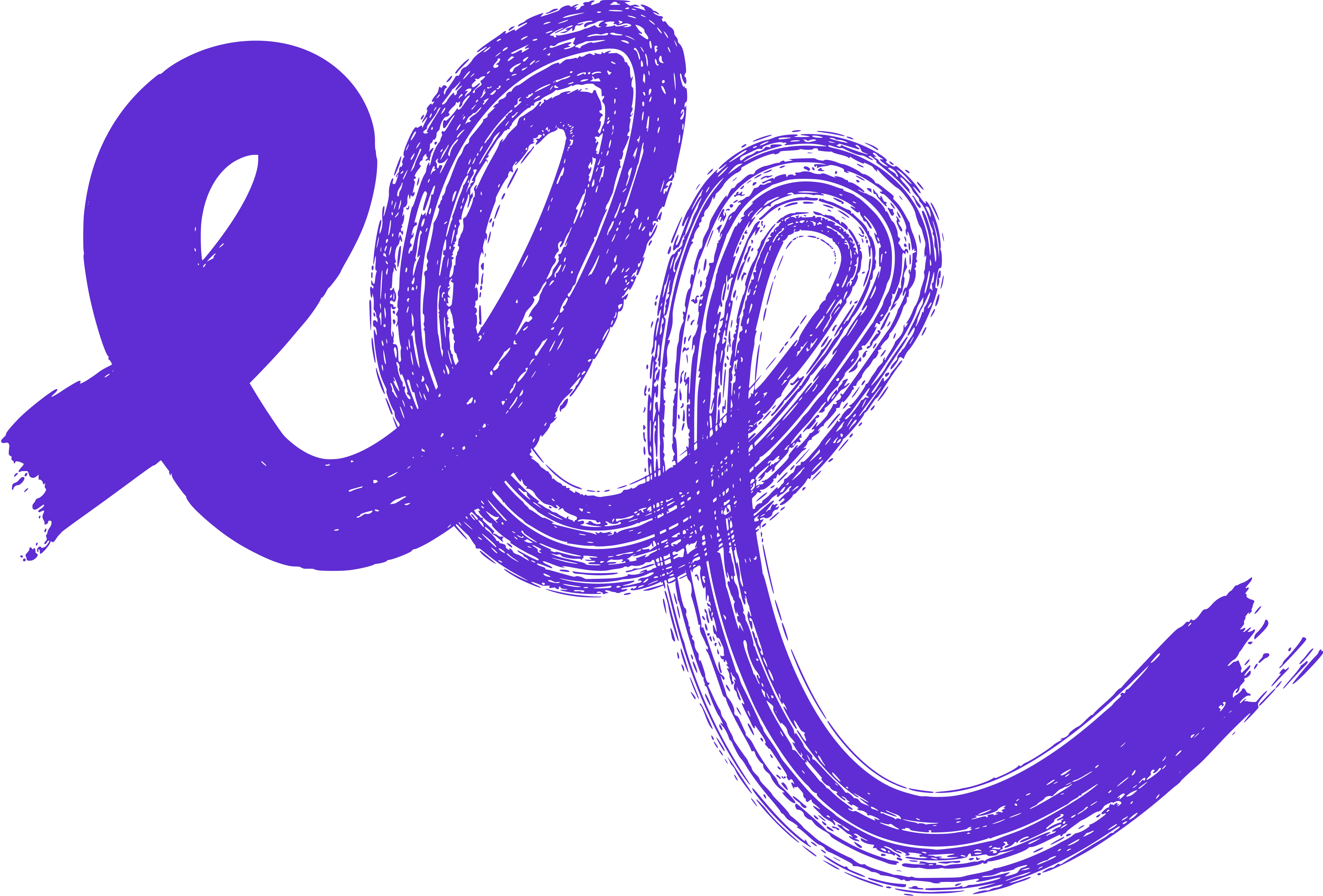
You may also
like...
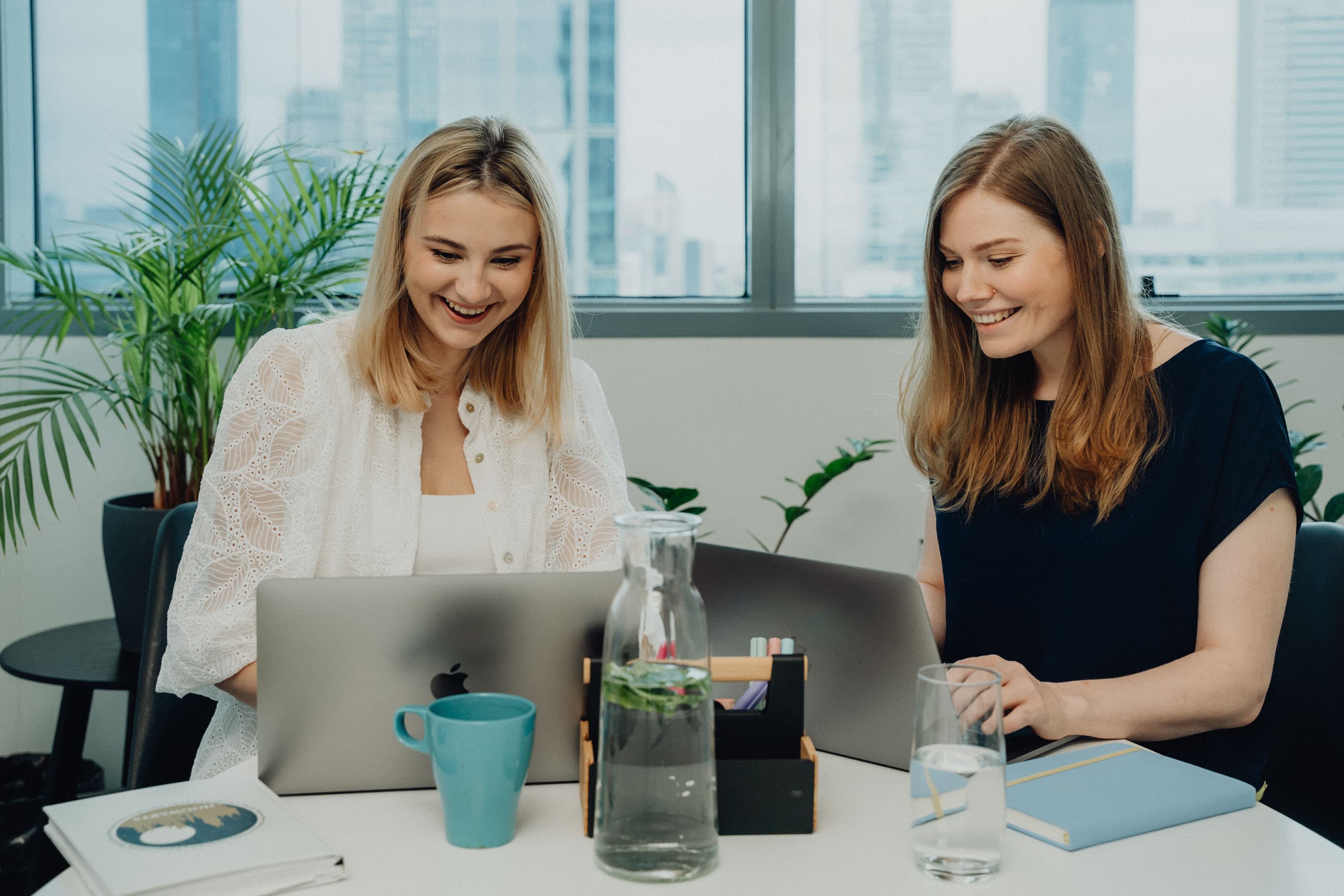
Mastering Declarative Programming: Essential Practices for Every Developer
Discover declarative programming essentials. This guide covers principles, tools, and best practices to simplify coding, enhance readability, and improve scalability.
Marek Pałys
Apr 16, 2024・11 min read
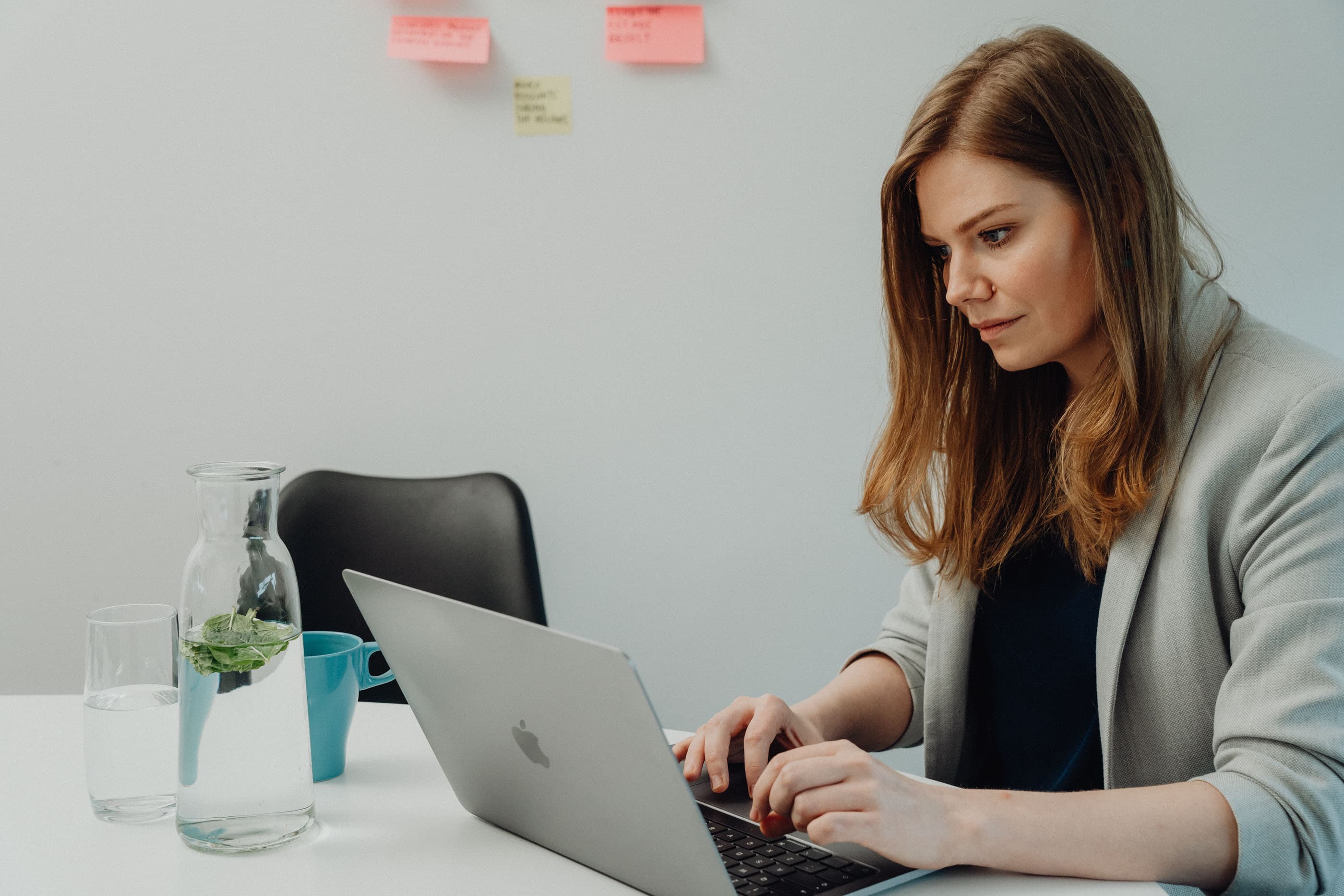
Understanding Event-Driven Programming: A Simple Guide for Everyone
Explore the essentials of event-driven programming. Learn how this responsive paradigm powers interactive applications with real-world examples and key concepts.
Marek Pałys
Apr 30, 2024・9 min read
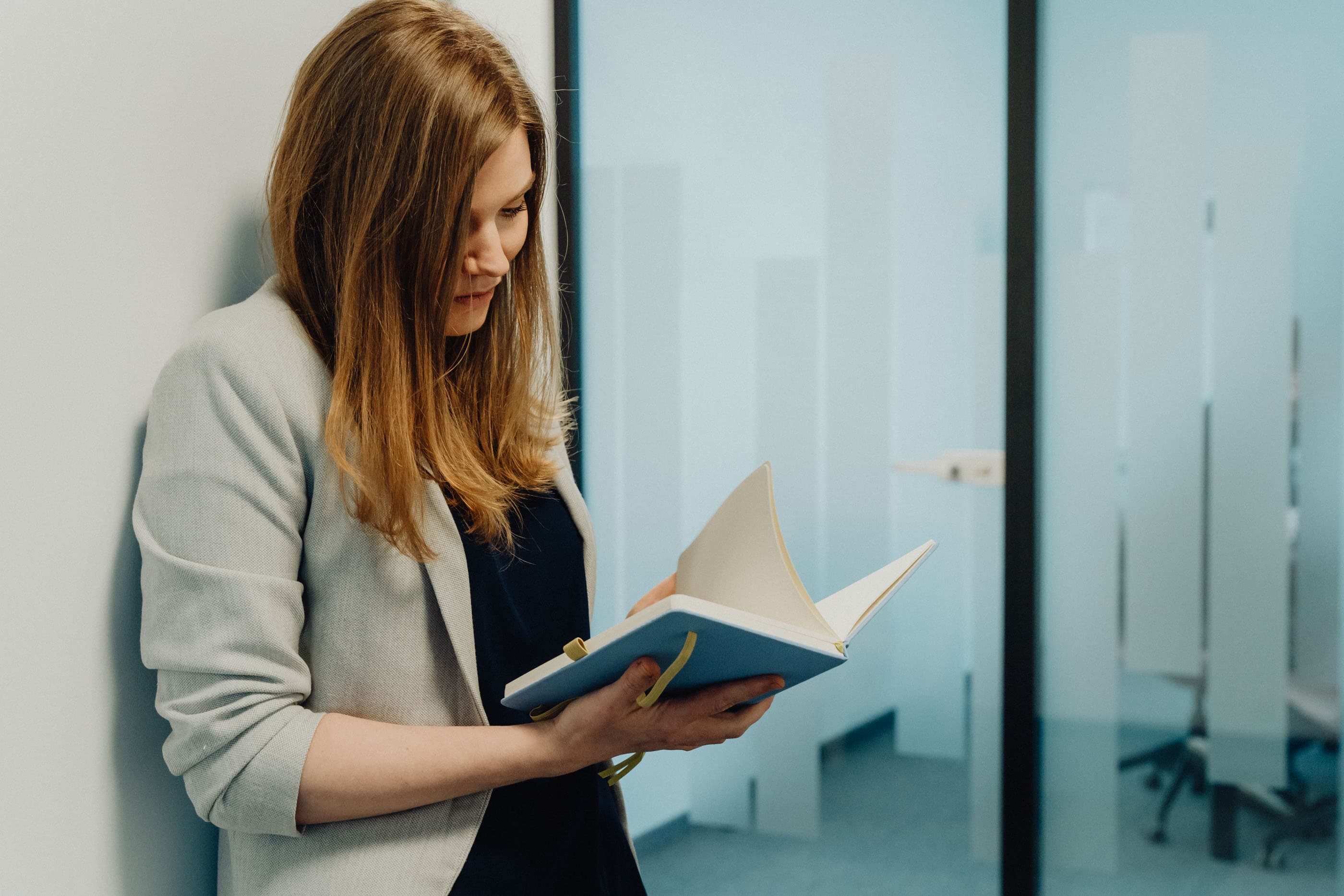
Demystifying Procedural Programming: Simple Examples for All
Explore procedural programming with easy-to-follow examples and insights into its core principles. Learn how this step-by-step approach forms the basis of many programming paradigms.
Marek Pałys
Jul 05, 2024・10 min read