🌍 All
About us
Digitalization
News
Startups
Development
Design
A Beginner's Guide to Building E-commerce Sites with Ruby on Rails
Marek Pałys
Aug 13, 2024・5 min read
Table of Content
Getting Started with Ruby on Rails
Essential Components of E-commerce Sites
Integrating Payment Gateways
Enhancing User Experience
Maintaining Your E-commerce Site
Building an e-commerce site can seem like a daunting task, particularly if you're new to web development. Fortunately, Ruby on Rails, a powerful and flexible web application framework, can make this process much more straightforward. Known for its simplicity and efficiency, Rails allows developers to create robust e-commerce platforms with relative ease. In this guide, we will delve into the essentials of setting up an e-commerce site using Ruby on Rails, providing you with practical steps and tips to get started. Whether you're a novice or someone looking to refine your skills, this guide will help you navigate the journey of building a successful online store.
Getting Started with Ruby on Rails
Setting Up Your Development Environment
Before you can start building your e-commerce site, you need to set up your development environment. Begin by installing Ruby, the programming language that Rails is built on. You can use a version manager like RVM or rbenv to manage different versions of Ruby. Next, install Rails by running the command gem install rails. This will install the latest version of Rails along with its dependencies.
Once Ruby and Rails are installed, you'll need to set up a database. SQLite is a good choice for development, though you might switch to PostgreSQL or MySQL for production. You'll also need a code editor; Visual Studio Code and Sublime Text are popular options. Finally, ensure Git is installed for version control. With these tools in place, you’re ready to start creating your e-commerce site with Ruby on Rails.
Installing Ruby on Rails
Installing Ruby on Rails is a straightforward process. First, ensure you have Ruby installed on your system. You can check this by running ruby -v in your terminal. If Ruby is not installed, you can download it from the official Ruby website or use a version manager like RVM or rbenv to install it.
Once Ruby is set up, installing Rails is simple. Open your terminal and run the command gem install rails. This command installs the latest version of Rails along with its dependencies. After the installation, verify it by running rails -v, which should display the installed Rails version.
It's also a good idea to install Bundler, a gem that manages dependencies for your Rails application. You can install Bundler by running gem install bundler. With Ruby, Rails, and Bundler installed, you are now ready to create and manage your Rails projects.
Creating Your First Rails Application
Creating your first Rails application is an exciting step. Begin by opening your terminal and navigating to the directory where you want to create your project. Then, run the command rails new my_ecommerce_site. Replace my_ecommerce_site with your desired application name. This command generates a new Rails application with a predefined directory structure, including folders for controllers, models, views, and more.
Once the command completes, navigate into your new application's directory using cd my_ecommerce_site. Before running the application, you need to set up the database. Run rails db:create to create the development and test databases. Next, start the Rails server with rails server or rails s. Open your web browser and go to http://localhost:3000, and you should see the Rails welcome page.
Congratulations, you've successfully created and run your first Rails application. Now you can start building the specific features for your e-commerce site.
Essential Components of E-commerce Sites
Understanding MVC Architecture
The Model-View-Controller (MVC) architecture is central to Ruby on Rails and helps structure your application effectively. MVC divides your application into three interconnected components: Models, Views, and Controllers.
The Model represents the data and business logic of your application. In an e-commerce site, models could include User, Product, and Order. Models interact with the database, managing the data.
The View is responsible for presenting data to the user. Views render the user interface and handle the display logic. In Rails, views are typically written in HTML with embedded Ruby (ERB) for dynamic content.
The Controller acts as the intermediary between models and views. Controllers handle incoming HTTP requests, process data through models, and pass the results to the views for rendering.
By following the MVC architecture, you can maintain a clean separation of concerns, making your codebase easier to manage and scale as your e-commerce site grows.
Setting Up User Authentication
User authentication is crucial for any e-commerce site, ensuring that only authorised users can access certain features. In Ruby on Rails, you can implement authentication easily using gems like Devise.
To get started, add Devise to your Gemfile by including gem 'devise', then run bundle install to install the gem. Next, run rails generate devise:install to set up the initial configuration. Follow the instructions provided by Devise to ensure proper setup.
Generate a User model with Devise by running rails generate devise User. This command creates a model and migration file for users. Run rails db:migrate to apply the changes to your database. Devise provides built-in views for user registration, login, and password recovery, which you can customise to match your site's design.
With user authentication in place, only registered users can perform actions like placing orders or accessing their account details, enhancing the security and user experience of your e-commerce site.
Managing Products and Inventory
Managing products and inventory is a core feature of any e-commerce site. In Ruby on Rails, you can create a Product model to handle the data related to your products. Start by generating a Product model with attributes such as name, description, price, and stock quantity using the command rails generate model Product name:string description:text price:decimal stock_quantity:integer.
After generating the model, run rails db:migrate to apply the changes to your database. You can then create a controller and views to manage the CRUD (Create, Read, Update, Delete) operations for your products. This allows you to add new products, update existing ones, and manage inventory levels.
Additionally, consider implementing validations in your Product model to ensure data integrity, such as validating the presence of a name and price. By effectively managing products and inventory, you can maintain an organised catalogue and provide a seamless shopping experience for your customers.
Integrating Payment Gateways
Choosing the Right Payment Gateway
Choosing the right payment gateway is essential for the success of your e-commerce site. A payment gateway facilitates online payments, ensuring that transactions are secure and efficient. When selecting a payment gateway, consider factors such as transaction fees, supported payment methods, and ease of integration with Ruby on Rails.
Popular payment gateways include Stripe, PayPal, and Square. Stripe is well-known for its developer-friendly API and extensive documentation, making it a popular choice for Rails applications. PayPal offers widespread recognition and supports various payment methods, including credit cards and direct bank transfers. Square provides a robust solution with competitive fees and easy integration.
Evaluate the needs of your business and your target audience when making your decision. Additionally, ensure that the payment gateway complies with security standards such as PCI-DSS. By choosing the right payment gateway, you can provide a seamless and secure checkout experience for your customers, boosting their confidence and increasing conversions.
Implementing Payment Processing
Implementing payment processing in your e-commerce site involves integrating your chosen payment gateway with your Ruby on Rails application. Let's use Stripe as an example due to its popularity and ease of use.
First, add the Stripe gem to your Gemfile by including gem 'stripe', then run bundle install. Next, configure your Stripe API keys in your Rails credentials by running rails credentials:edit and adding your stripe_publishable_key and stripe_secret_key.
Create a PaymentsController to handle the payment process. In your controller, set up actions to create a payment intent and process the payment. Use Stripe's JavaScript library to handle the payment form and securely collect payment details from your customers.
When a customer submits their payment information, your Rails application will communicate with Stripe's API to complete the transaction. Ensure you handle errors and provide feedback to the user.
By correctly implementing payment processing, you can facilitate secure and efficient transactions, enhancing the overall shopping experience for your customers.
Ensuring Secure Transactions
Ensuring secure transactions is paramount for any e-commerce site, safeguarding both your business and your customers. Start by choosing a payment gateway that complies with PCI-DSS (Payment Card Industry Data Security Standard), which ensures that credit card information is handled securely.
Encrypt sensitive data using SSL (Secure Socket Layer) to protect information transmitted between your server and the user's browser. Rails makes it easy to enforce SSL by enabling the force_ssl option in your application's configuration.
Use HTTPS for all pages where sensitive information is exchanged, such as checkout and account pages. Additionally, employ security measures such as two-factor authentication for user accounts to add an extra layer of protection.
Regularly update your Rails application and gems to patch any security vulnerabilities. Monitor your application for suspicious activities and consider using a Web Application Firewall (WAF) for added security.
By implementing these measures, you can ensure secure transactions, building trust with your customers and protecting your business from potential threats.
Enhancing User Experience
Designing User-Friendly Interfaces
Designing user-friendly interfaces is crucial for the success of your e-commerce site. A well-designed interface can significantly improve user experience, making it easier for customers to navigate your site and complete purchases. Start by prioritising simplicity and clarity in your design. Use a clean layout with intuitive navigation to help users find what they are looking for effortlessly.
Ensure that your site is responsive, providing a seamless experience across various devices, including desktops, tablets, and smartphones. Use larger buttons and touch-friendly elements for mobile users.
Incorporate visual hierarchy to guide users' attention to important elements, such as call-to-action buttons like "Add to Cart" and "Checkout." Employ consistent typography and colour schemes to create a cohesive look and feel.
Gather feedback from users through usability testing to identify and address any pain points. By focusing on designing user-friendly interfaces, you can enhance the overall shopping experience, encouraging repeat visits and increasing customer satisfaction.
Optimising Site Performance
Optimising site performance is vital for delivering a fast and smooth user experience, which can significantly impact customer satisfaction and conversion rates. Start by minimising the loading time of your e-commerce site. Compress images and other media files to reduce their size without compromising quality. Use tools like ImageMagick or online services to automate this process.
Implement caching strategies to store frequently accessed data, reducing the need for repeated database queries. Rails provides built-in support for caching, such as fragment caching and low-level caching, which can improve response times.
Use a Content Delivery Network (CDN) to distribute your content globally, ensuring faster load times for users regardless of their location.
Optimise your database queries to avoid unnecessary load and latency. Use indexes and optimise ActiveRecord queries to fetch only the required data.
Regularly monitor your site's performance using tools like Google PageSpeed Insights or GTmetrix to identify areas for improvement. By optimising site performance, you can provide a faster, more enjoyable shopping experience for your users.
Incorporating Responsive Design
Incorporating responsive design is essential for ensuring your e-commerce site provides a seamless user experience across all devices. A responsive design automatically adjusts the layout and elements of your site to fit different screen sizes, from desktops to smartphones.
Begin by using a mobile-first approach. Design your layout for smaller screens first, then progressively enhance it for larger screens. This ensures that essential content is prioritised and accessible on mobile devices.
Utilise flexible grid layouts and CSS media queries to create a fluid and adaptable design. Frameworks like Bootstrap or Foundation can simplify the implementation of responsive design elements.
Ensure touch-friendly navigation for mobile users by using larger buttons and avoiding hover-dependent interactions. Test your site on various devices and screen sizes to verify that it maintains usability and aesthetic appeal.
By incorporating responsive design, you can provide a consistent and enjoyable user experience, regardless of the device your customers use, which can lead to higher engagement and increased sales.
Maintaining Your E-commerce Site
Monitoring Site Analytics
Monitoring site analytics is crucial for understanding user behaviour, tracking performance, and making data-driven decisions to improve your e-commerce site. Begin by integrating an analytics tool such as Google Analytics or Matomo into your Rails application. These tools provide comprehensive insights into how users interact with your site.
Set up tracking for key metrics, including page views, bounce rates, conversion rates, and average session duration. Use these metrics to identify patterns and pinpoint areas for improvement. For instance, high bounce rates on specific pages may indicate issues with content or usability.
Create custom reports and dashboards to monitor the performance of marketing campaigns, product sales, and user engagement. Regularly review these reports to assess the effectiveness of your strategies and make necessary adjustments.
Additionally, consider setting up event tracking to capture specific user actions, such as button clicks or form submissions. By consistently monitoring site analytics, you can optimise your e-commerce site to meet user needs and achieve business goals.
Updating and Scaling Your Application
Updating and scaling your application are vital for ensuring long-term success and accommodating growth. Regularly update your Rails application and dependencies to incorporate the latest features, security patches, and performance improvements. Use bundle update to manage gem updates and address any deprecations or breaking changes.
To scale your application, start by optimising your existing codebase. Refactor inefficient code, improve database queries, and utilise caching to handle increased traffic and data loads efficiently. Consider horizontal scaling by adding more servers or vertical scaling by increasing the resources of your current server.
Implement load balancing to distribute traffic evenly across your servers, ensuring consistent performance. Use services like Amazon Web Services (AWS) or Heroku to manage scaling and deployment effortlessly.
Monitoring tools such as New Relic or Datadog can help track performance and identify bottlenecks. By staying proactive with updates and scalability, you can maintain a robust, high-performing e-commerce site that grows along with your business.
Handling Customer Support and Feedback
Handling customer support and feedback is essential for maintaining a positive relationship with your customers and improving your e-commerce site. Start by providing multiple channels for support, such as email, live chat, and social media. Ensure that your contact information is easily accessible on your site.
Implement a ticketing system to manage and prioritise customer inquiries efficiently. Tools like Zendesk or Freshdesk can help streamline the process, ensuring timely responses and resolution of issues.
Encourage customers to leave feedback and reviews. Positive reviews can boost your site's credibility, while constructive criticism can provide valuable insights into areas needing improvement. Use surveys and feedback forms to gather detailed information about customer experiences.
Regularly review and analyse feedback to identify common issues and trends. Use this data to make informed decisions and improve your products, services, and overall user experience.
By effectively handling customer support and feedback, you can build trust, enhance customer satisfaction, and foster loyalty, all of which are crucial for the sustained success of your e-commerce
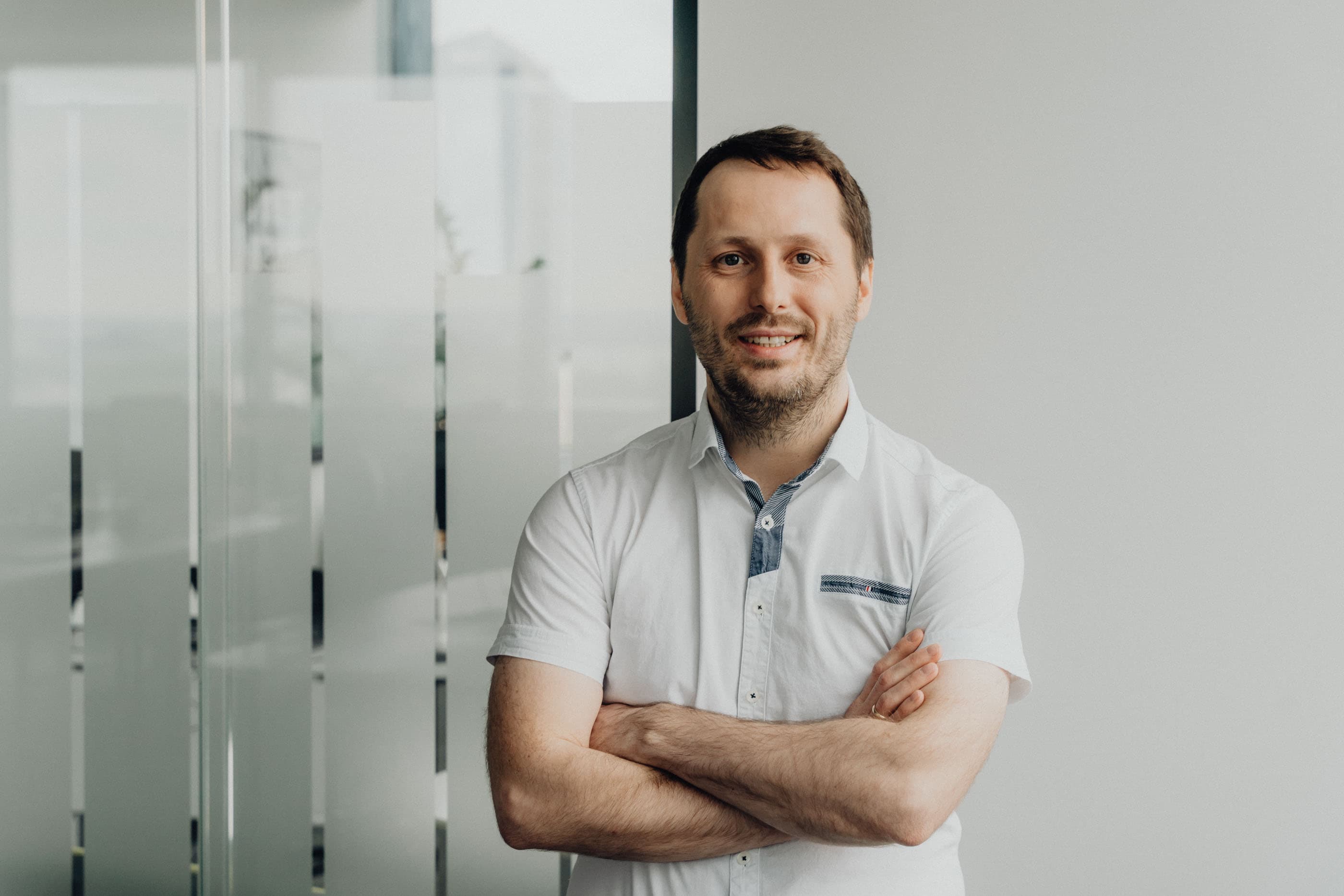
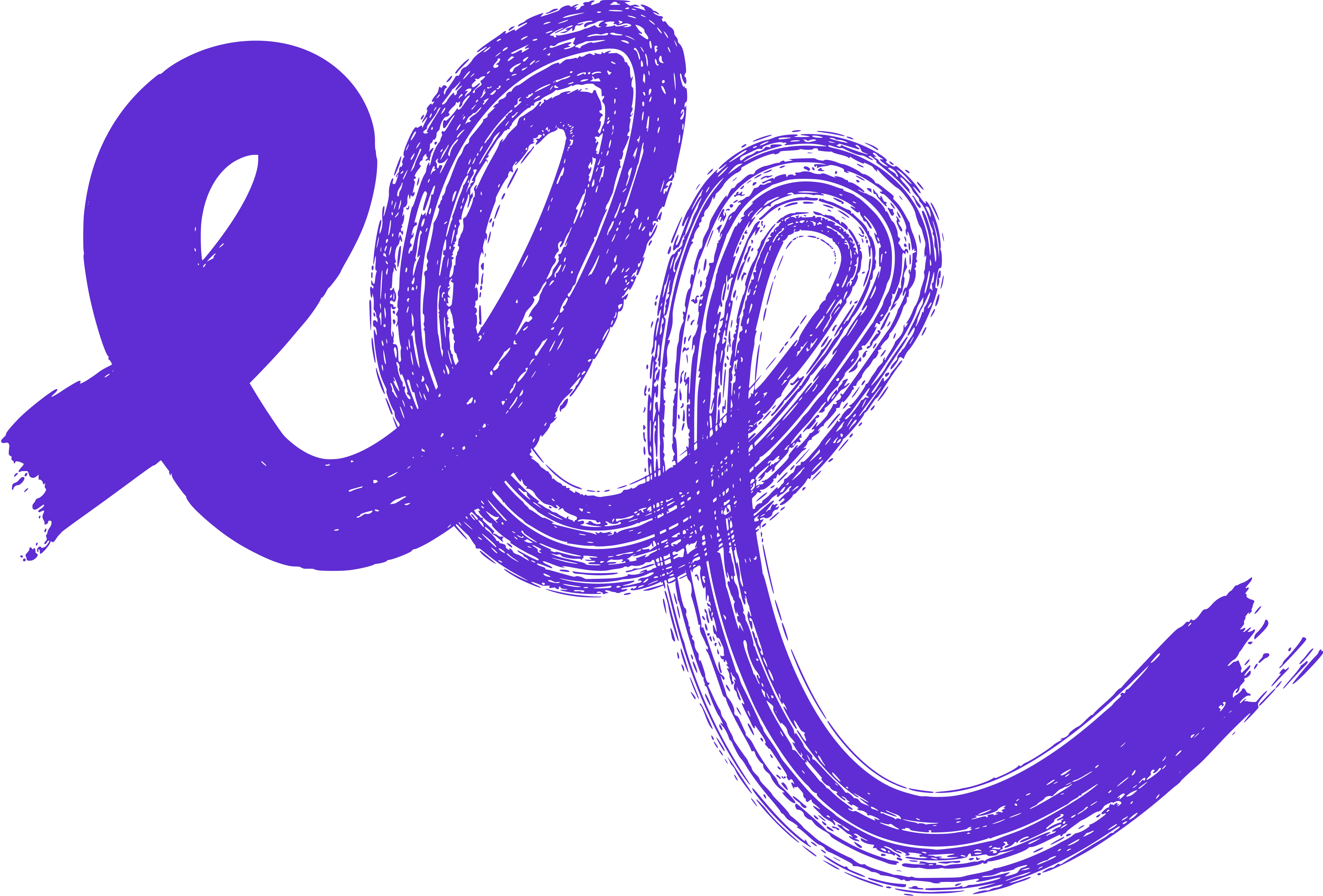
You may also
like...
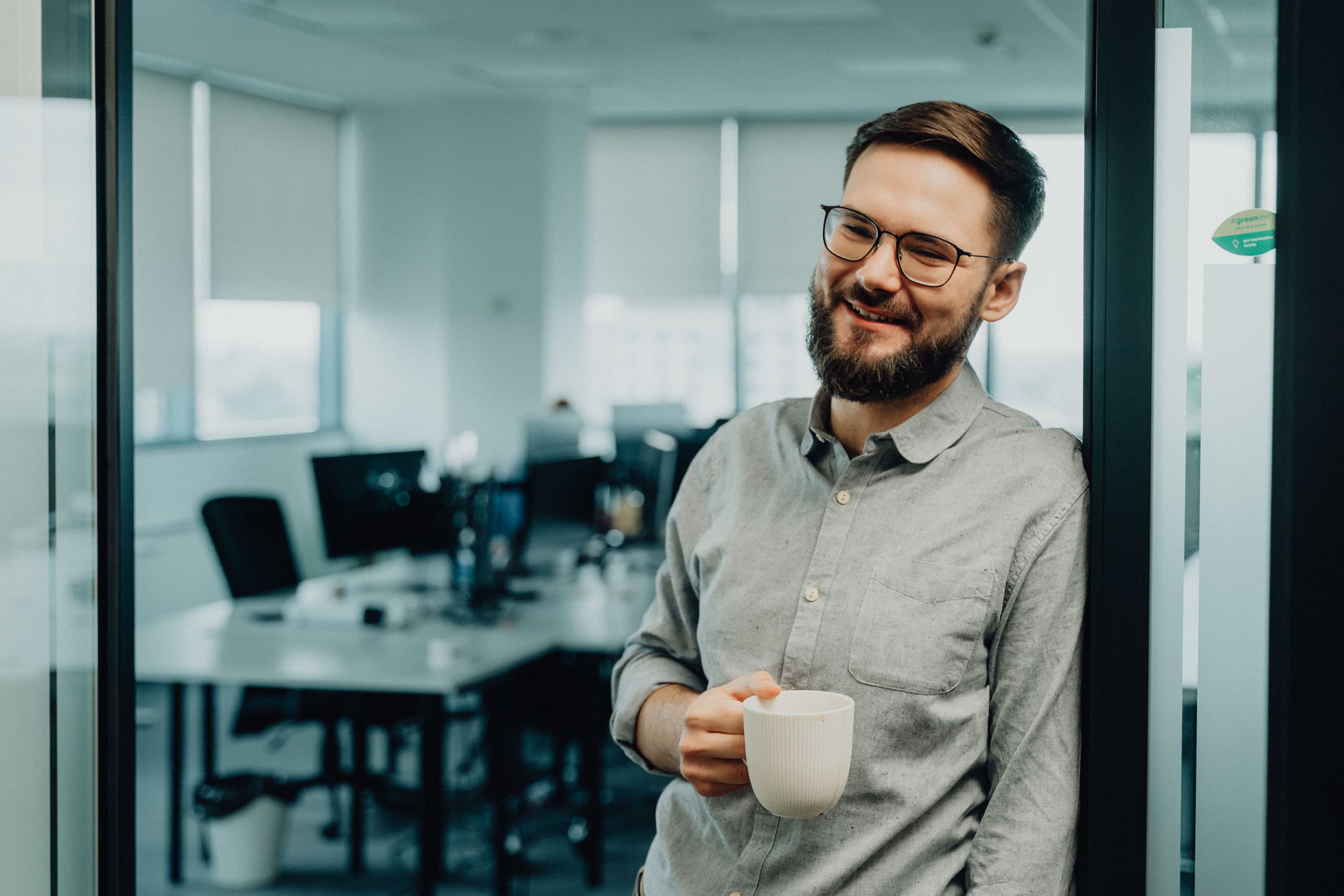
Mastering the Art of Hiring Remote Developers: A Comprehensive Guide
As the demand for skilled remote developers continues to grow, mastering the art of hiring remote teams is crucial for any business looking to stay competitive. This guide provides a step-by-step approach to hiring remote developers, from understanding the importance of remote work and its challenges to crafting the perfect job description and sourcing talent through the right platforms. Learn how to conduct effective remote interviews, assess technical skills, and evaluate cultural fit to build a strong and cohesive remote team. We also cover essential onboarding and integration practices to ensure your new hires are set up for success and fully integrated into your company culture. Whether you're a seasoned recruiter or new to remote hiring, this guide offers practical tips and insights to help you navigate the complexities of hiring remote developers efficiently and successfully.
Marek Pałys
Jun 11, 2024・5 min read
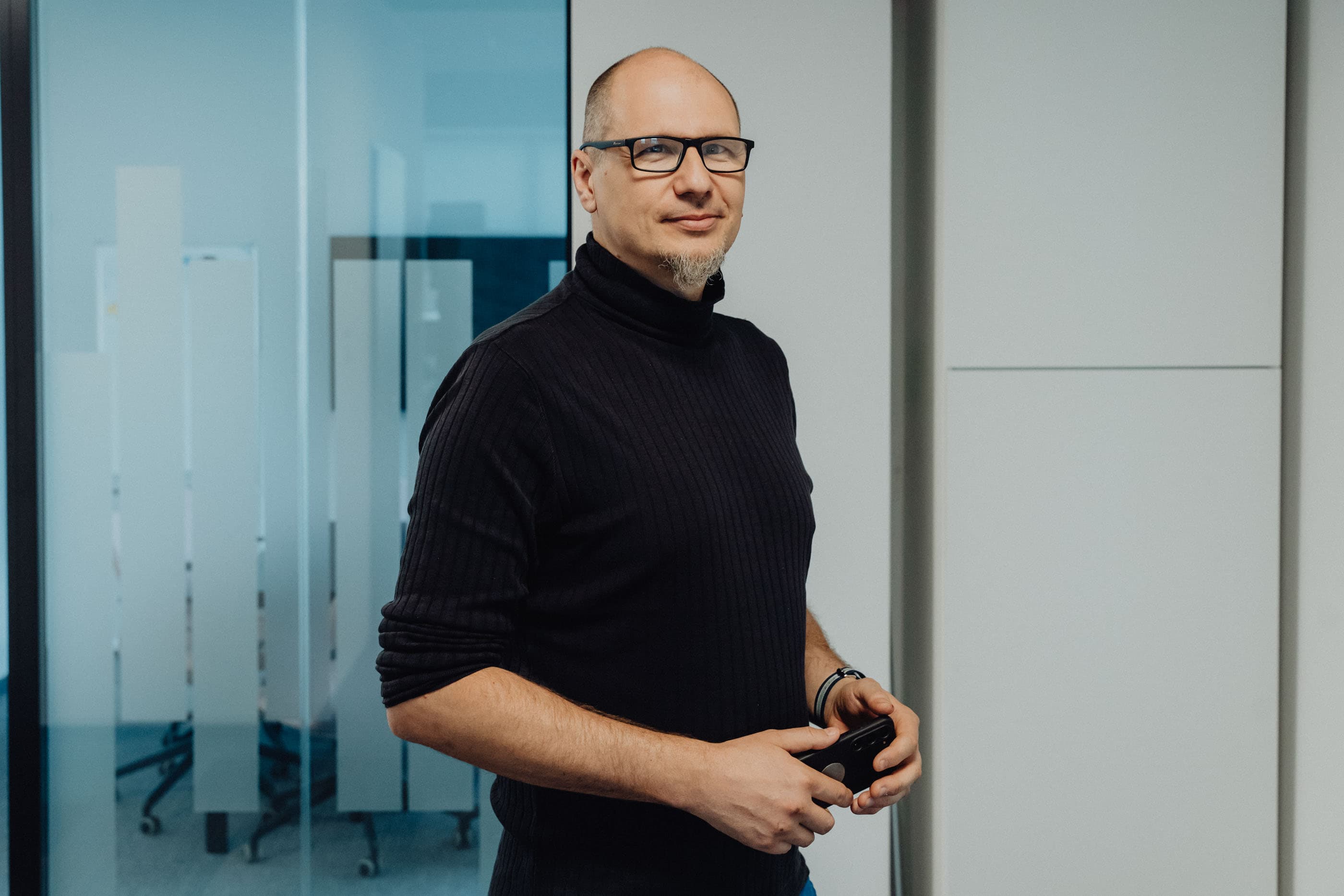
How to Hire Mobile App Developers: A Practical Guide for Businesses
Hiring the right mobile app developers is crucial for building a strong mobile presence. This guide provides a comprehensive overview of the steps involved in hiring skilled developers who can deliver on your business goals. From defining your project scope and identifying key skills to finding developers and evaluating their portfolios, we cover it all. Learn how to conduct effective interviews, assess technical skills, and evaluate cultural fit to ensure you make the right hiring decisions. Finally, discover tips on negotiating terms and successfully onboarding your new developers to set the stage for productive collaboration.
Marek Pałys
Mar 25, 2024・7 min read
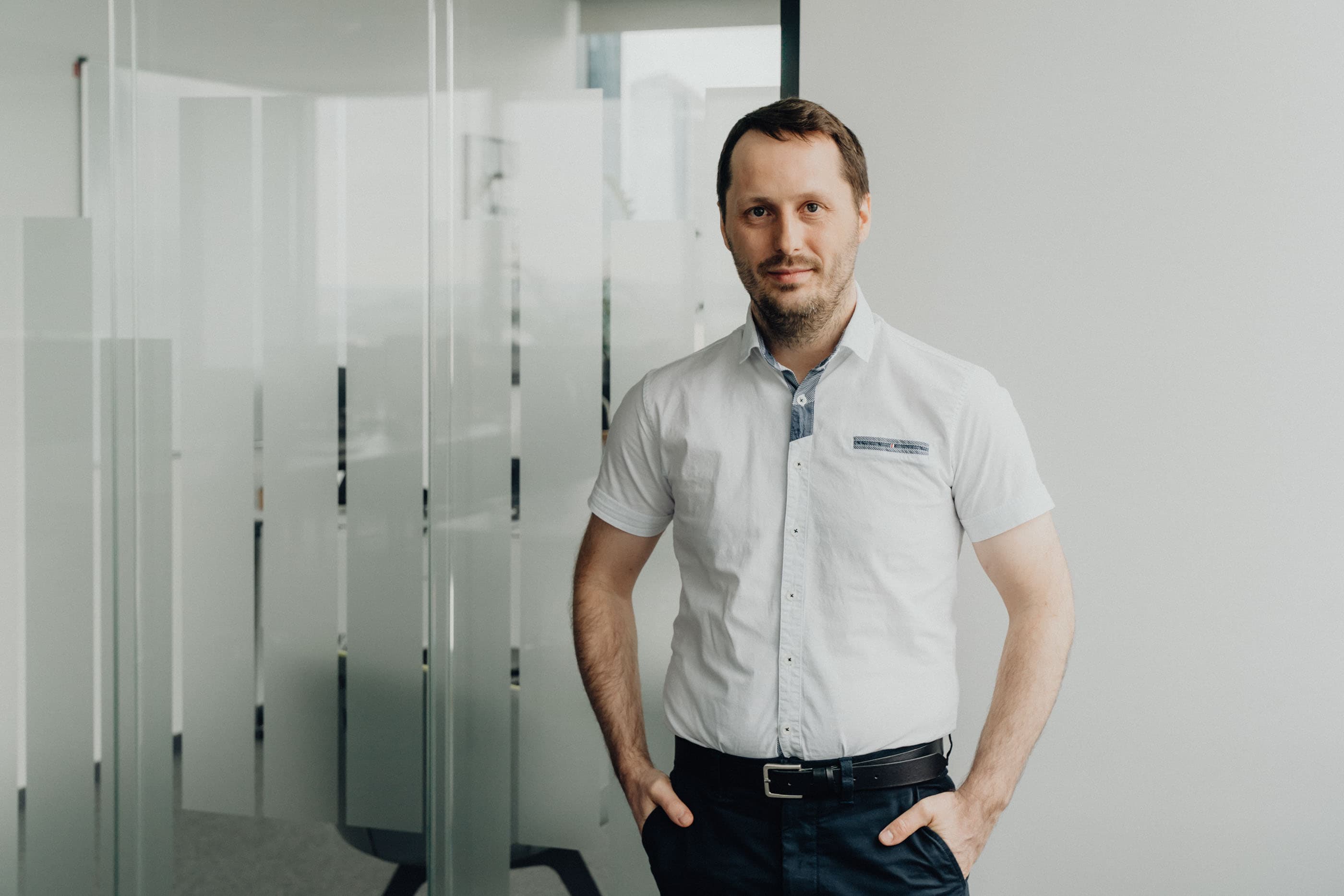
How to Hire Developers for a Startup: A Step-by-Step Guide
Hiring developers for a startup can be challenging but crucial for success. This guide offers practical steps to help you find and recruit the right talent. Whether you're defining project requirements, identifying the necessary skills, or assessing cultural fit, these strategies will ensure you build a technical team that aligns with your startup's vision. Learn how to leverage online platforms, conduct effective interviews, and craft competitive offers that attract top developers. Plus, discover tips on onboarding and fostering a collaborative environment to drive your startup's growth and innovation.
Marek Pałys
Apr 24, 2024・5 min read