🌍 All
About us
Digitalization
News
Startups
Development
Design
A Comprehensive Guide to Implementing BLoC Architecture in Flutter
Marek Majdak
Jul 13, 2023・4 min read
Table of Content
Components Involved in the BLoC Pattern
Benefits of the BLoC architecture
Building the Application Architecture
When to use the BLoC pattern
Final thoughts
Frequently Asked Questions
Flutter has emerged as one of the leading frameworks for mobile application development due to its simplicity, efficiency, and the power to create stunning user interfaces. As with any framework, the choice of an architectural pattern for structuring the app's business logic is paramount in crafting high-quality Flutter applications. In this context, the Business Logic Component (BLoC) pattern has gained widespread acceptance in the Flutter community.
Components
Involved in the BLoC Pattern
The main components involved in the BLoC pattern are Events, States, and the BLoC itself. Events are user interactions or network calls that instruct the BLoC to perform certain tasks. The BLoC then takes these incoming events, processes them, and produces an output, a new state. States are part of the app's state that are displayed in the user interface (UI). These components work together to decouple the presentation layer from the business logic, facilitating better code organization and ease of testing.
The local BLoC instance builder helps manage the BLoC instance for a particular part of the widget tree. This makes it easier to manage the lifecycle of the BLoC, avoiding memory leaks and redundant code inside your Flutter app's architecture.
Benefits of the BLoC architecture
Using the BLoC architecture provides several advantages. It separates the business logic component from the UI component, making it easier to maintain and test the code. By ensuring that all the business logic is housed within a single instance, changes in the data layer, such as an API response, do not directly affect the UI.
Reactive programming is at the heart of the BLoC architecture, and Dart Stream, a core component of Flutter, complements it perfectly. It allows multiple widgets to react to state changes concurrently, fostering a more dynamic and responsive user experience.
The BLoC architecture also reduces the amount of boilerplate code, thanks to the Flutter bloc library. This library simplifies the process of implementing BLoC and streamlines Flutter app development, contributing to cleaner, more maintainable code.
Building the Application Architecture
To construct a Flutter project using the BLoC architecture, it's essential to start by creating the necessary bloc classes and state classes. Using the Flutter package, 'flutter_bloc', greatly simplifies this process.
Remember to structure your code well by separating components into different files. For instance, creating a new file for each bloc class and state class enhances readability and maintainability.
To fetch data, say through a network request, or to handle user interactions, dispatch events to the BLoC. Within the BLoC, implement the mapEventToState and initialState methods to handle the incoming events and provide an initial state.
The following code can be placed inside the bloc class:
dartCopy code
class DecrementEvent extends IncomingEvent {...}
And, in the UI, implement the BlocBuilder to respond to state changes. The bloc argument links the bloc class to the UI, and the build method allows the UI to respond to state changes.
dartCopy code
BlocBuilder<BlocClass, StateClass>( bloc: blocArgument, builder: (BuildContext context, StateClass state) { //return scaffold with different UI for each state }, );
Remember to include the dispose method to avoid memory leaks, by calling it on the bloc instance.
dartCopy code
@override void dispose() { bloc.dispose(); super.dispose(); }
When to use the BLoC pattern
The BLoC pattern is a great choice when you have complex business logic that needs to be separated from the presentation layer. This architecture works excellently in real-world projects, as it aids in reducing code duplication by following the same patterns across the development process.
However, for simple app requirements, the overhead of setting up the BLoC architecture might be unnecessary. Alternatives such as Provider or Riverpod could be a better choice.
Final thoughts
BLoC stands for Business Logic Component, but in the Flutter universe, it's a superhero, streamlining Flutter app development and promoting maintainability, testability, and reusability. With proper understanding and implementation, the BLoC architecture can significantly enhance the quality of your Flutter apps. Happy coding!
Frequently Asked Questions
1. What are bloc classes in the BLoC architecture?
Bloc classes are key components in the BLoC architecture. They accept incoming events and convert them into states. The bloc class also includes the business logic components that handle events and create new states.
2. How active is the Flutter community in the development of the BLoC pattern?
The Flutter community is highly active and constantly contributing to the growth and refinement of the BLoC pattern. Numerous libraries, tutorials, and examples are available, developed by the Flutter community, to aid in implementing the BLoC pattern.
3. Can you explain the role of reactive programming in Flutter apps using BLoC?
Reactive programming is a crucial aspect of BLoC in Flutter apps. The BLoC architecture relies heavily on streams for state management, which is a fundamental concept in reactive programming. This makes it possible for multiple widgets to react to state changes concurrently, enhancing the user experience.
4. How does the BLoC architecture interact with the data layer of Flutter applications?
In BLoC architecture, any data change, such as a network call response, becomes an event that the BLoC class processes to create a new state. This keeps the data layer and presentation layer separate, contributing to a more maintainable and testable code.
5. Can the BLoC architecture reduce boilerplate code in Flutter projects?
Absolutely! The BLoC architecture, especially when using the flutter_bloc library, can significantly reduce boilerplate code in Flutter projects. It streamlines state management and creates a more maintainable code structure.
6. How do architectural patterns like BLoC affect error messages in Flutter development?
Using architectural patterns like BLoC in Flutter development can make error messages more comprehensible. By separating the app's logic into different bloc classes, it becomes easier to pinpoint where an error originates, and thus, more straightforward to resolve.
7. Why is it important to create a new file for each BLoC class and state class in Flutter apps?
Creating a new file for each BLoC class and state class helps to keep the code organized and maintainable. It makes it easier to navigate and locate specific parts of the code, especially in larger real-world projects.
8. What is the role of the presentation layer in the BLoC architecture?
In the BLoC architecture, the presentation layer, or the UI component, is entirely separated from the business logic. This layer only reacts to state changes and updates the UI accordingly. It allows for a clean codebase and promotes the reusability of UI components.
9. What is the significance of the dispose method in bloc classes?
The dispose method is critical in bloc classes to ensure proper resource management. It's called when the bloc is no longer needed, thus helping to avoid memory leaks and improving the overall performance of Flutter applications.
10. How are multiple widgets handled in the BLoC architecture?
In the BLoC architecture, multiple widgets can respond to state changes concurrently, thanks to the reactive programming pattern. The bloc instance can be accessed from multiple widgets within the widget tree, allowing for a responsive and dynamic user interface.
11. How is data fetched in the BLoC architecture?
Data fetching in the BLoC architecture is typically done through events. For example, a network call could be initiated by dispatching a fetch event to the bloc. The bloc class then handles this event, fetches the data, and emits a new state reflecting the updated data.
12. Can the BLoC architecture be used in simple apps as well as in real-world projects?
Yes, the BLoC architecture can be used in both simple apps and real-world projects. However, for very simple apps, the overhead of setting up the BLoC architecture might be unnecessary. For complex, real-world projects, BLoC can bring significant benefits in terms of maintainability, scalability, and testability.
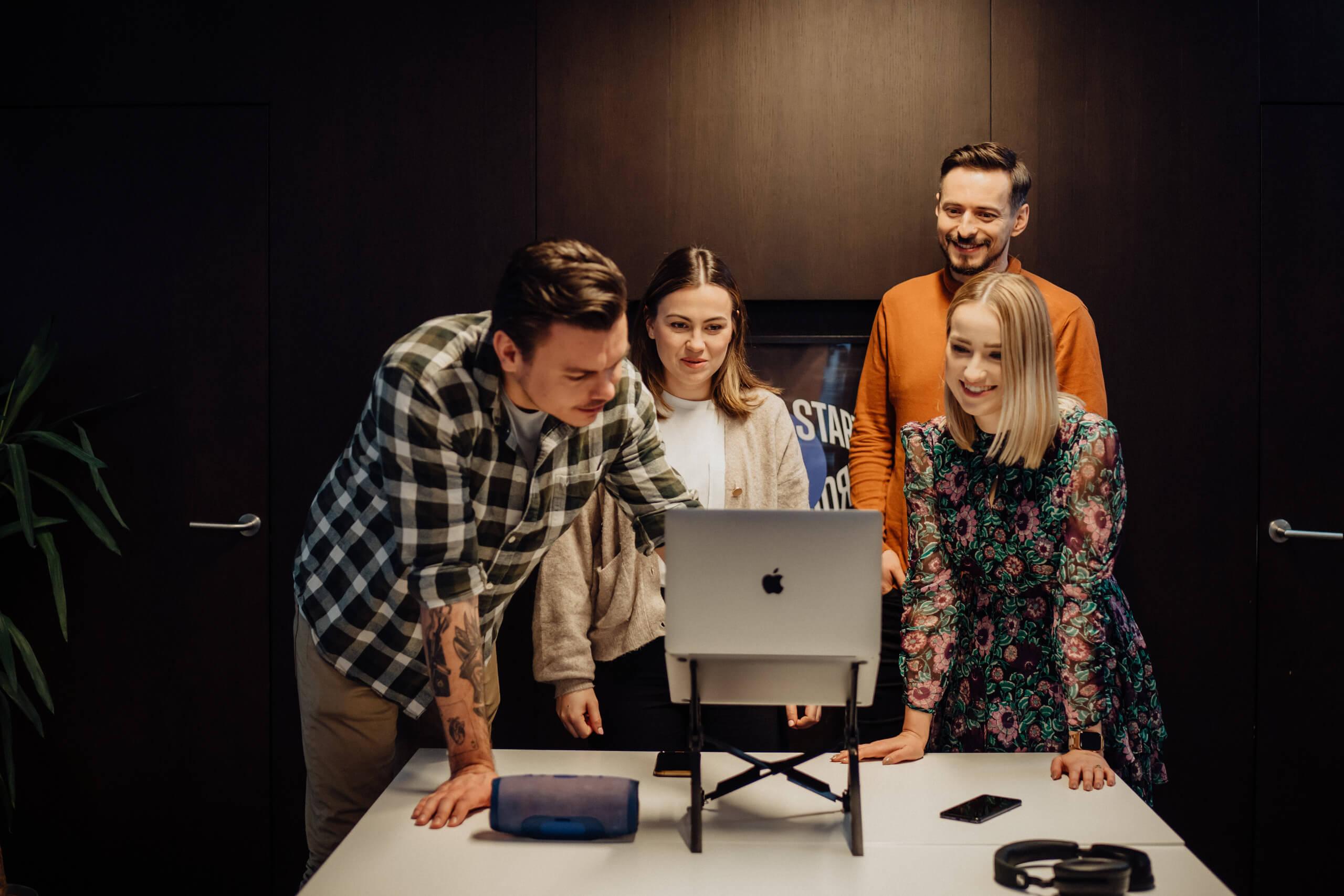
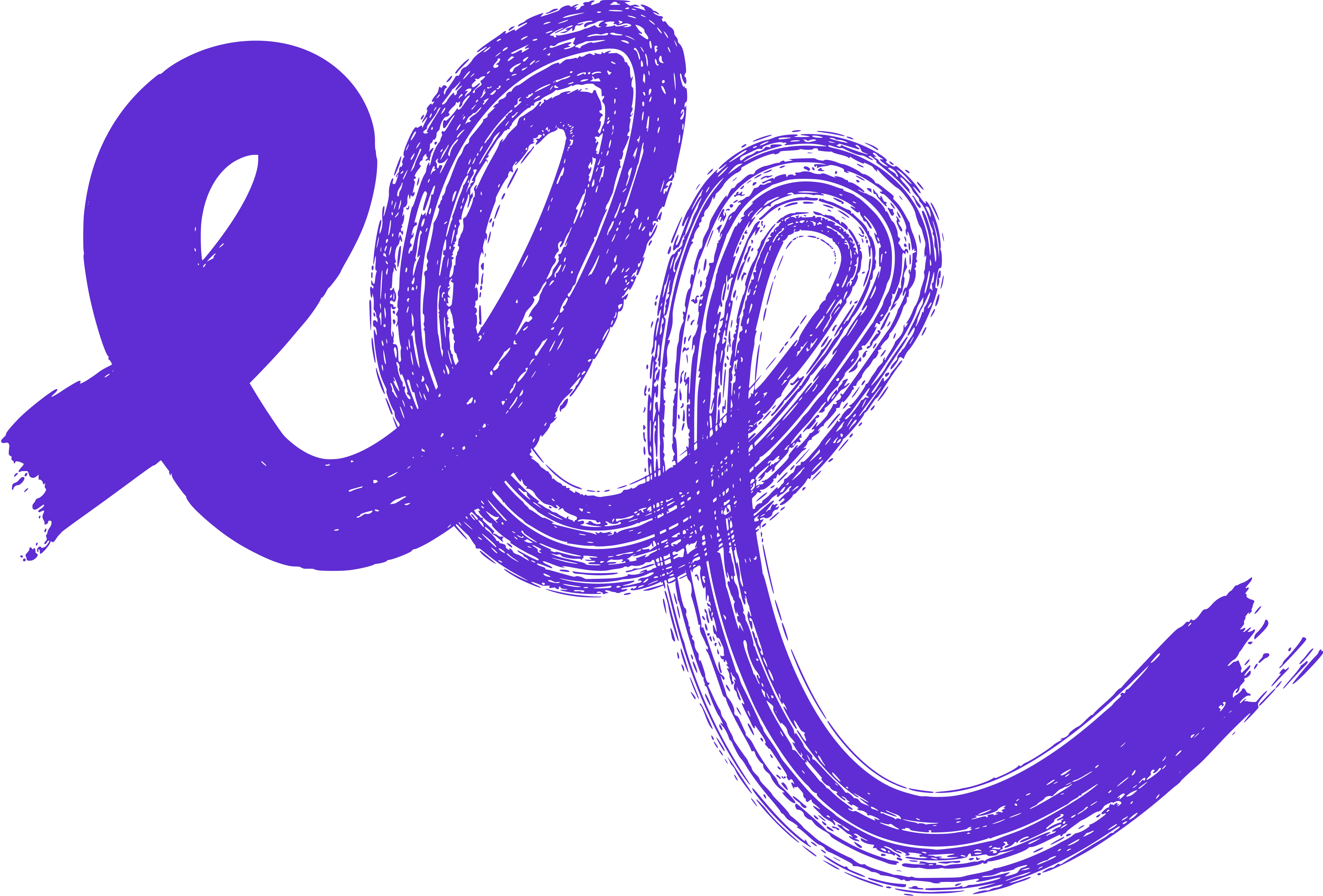
You may also
like...
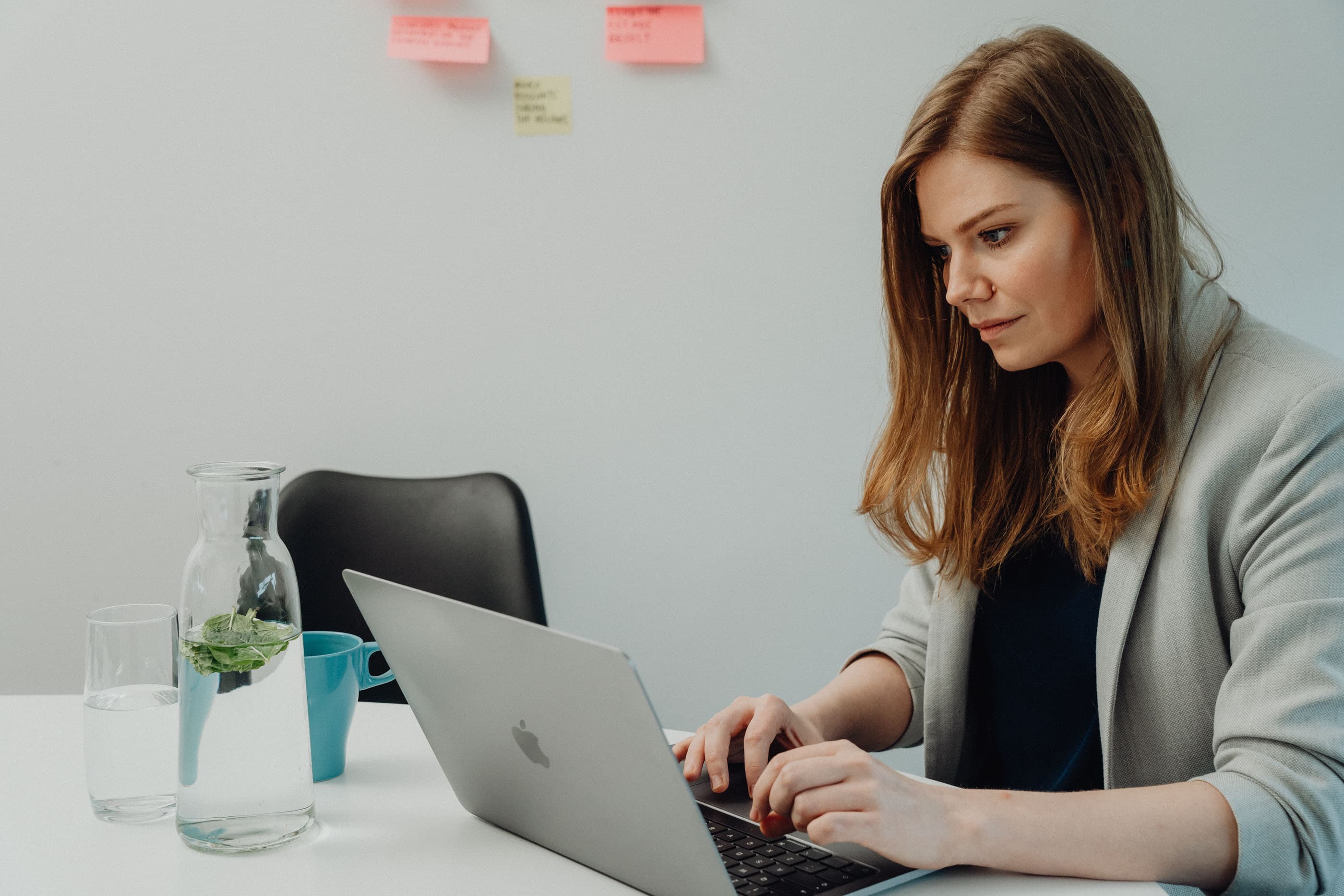
Understanding Event-Driven Programming: A Simple Guide for Everyone
Explore the essentials of event-driven programming. Learn how this responsive paradigm powers interactive applications with real-world examples and key concepts.
Marek Pałys
Apr 30, 2024・9 min read
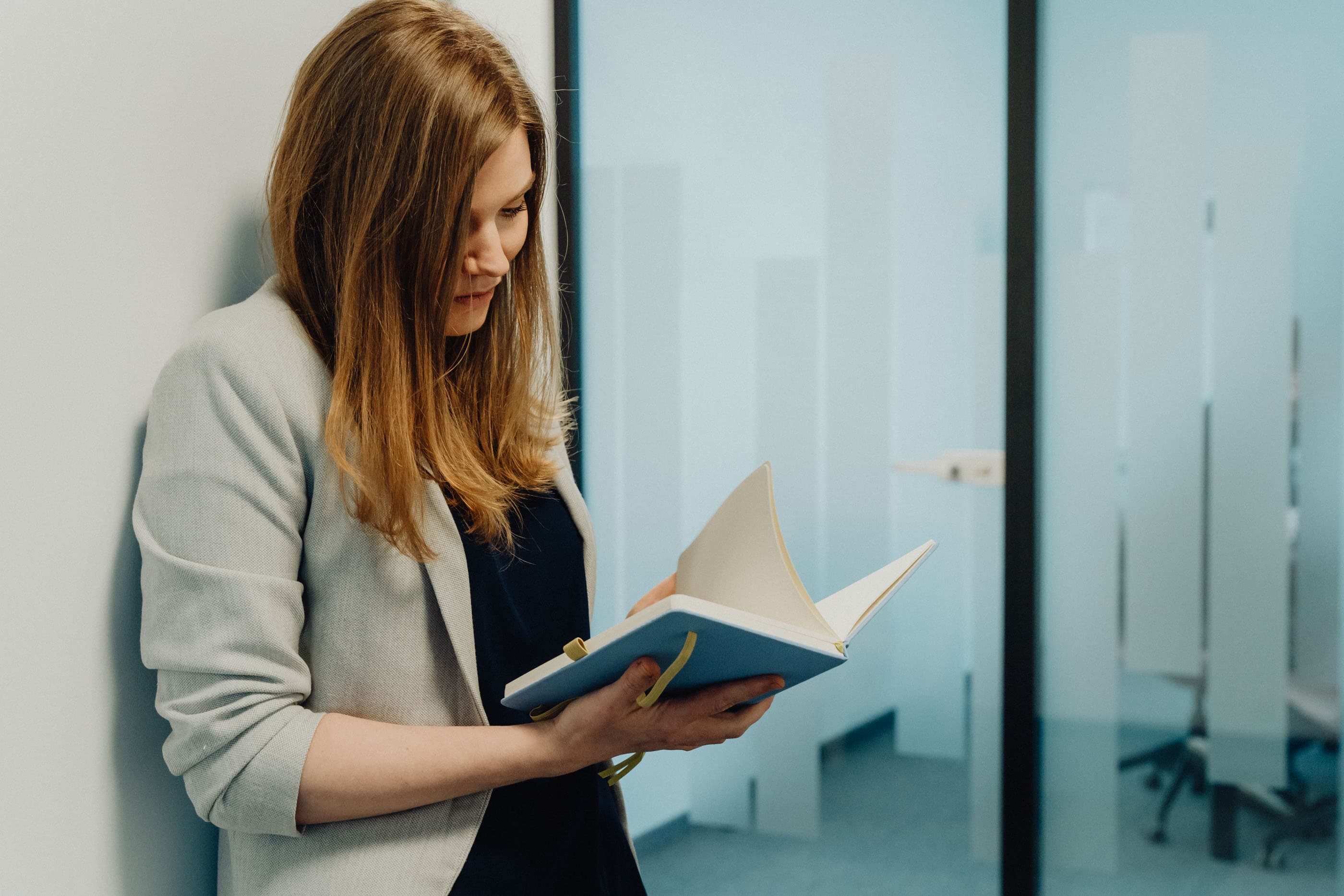
Unlocking Growth: How Serverless Architecture Can Transform Your Applications
Unlock the power of serverless architecture to build scalable and efficient applications. This guide explores the benefits of serverless, its implementation, cost-efficiency, and future trends, helping you innovate faster while reducing operational overhead.
Marek Majdak
Dec 27, 2023・12 min read
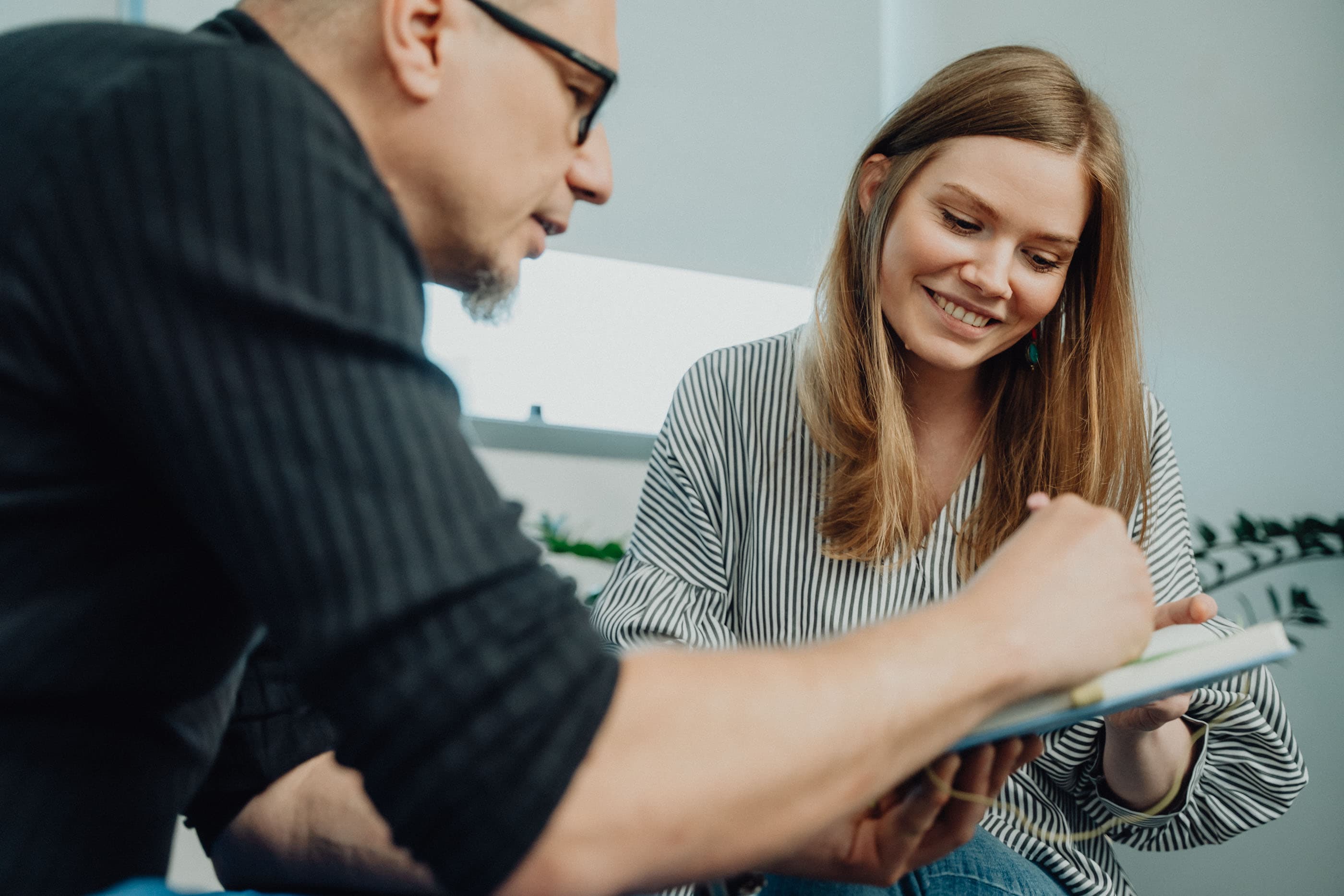
Navigating the Cloud: Understanding SaaS, PaaS, and IaaS
Discover the differences between SaaS, PaaS, and IaaS in cloud computing. This guide explains each model, their benefits, real-world use cases, and how to select the best option to meet your business goals.
Marek Pałys
Dec 12, 2024・11 min read