🌍 All
About us
Digitalization
News
Startups
Development
Design
Understanding the Basics: BaseModel vs ActiveRecord Validator in Rails
Marek Pałys
Oct 10, 2024・8 min read
Table of Content
Introduction to Rails Validators
Key Differences Explained
Implementing BaseModel in Your Project
Working with ActiveRecord Validator
Conclusion and Final Thoughts
Ensuring data integrity and validation is pivotal to building robust applications. One common area of focus for developers is the choice between using BaseModel and ActiveRecord Validator for these tasks. Understanding the distinctions, benefits, and appropriate use cases of BaseModel vs ActiveRecord Validator in Rails can significantly impact the effectiveness and maintainability of your code. This guide aims to demystify these core components, providing clear insights into their functionalities and practical applications. Let's delve into the basics and discern which approach best suits your development needs.
Introduction to Rails Validators
What is BaseModel?
In Rails, the term "BaseModel" often refers to an abstract class that provides a foundation for other models. It typically encapsulates shared logic and functionality that multiple models might require. By using a BaseModel, developers can centralise common methods and validations, promoting code reusability and consistency across the application. This abstraction layer allows for the implementation of custom validation logic that might not be feasible with ActiveRecord alone. In essence, a BaseModel acts as a parent class from which other models inherit, ensuring that they adhere to the same validation rules and logic. While it offers flexibility and control, using a BaseModel requires careful consideration to maintain readability and avoid overly complex code structures. Understanding when and how to implement a BaseModel is crucial for optimising your Rails application and ensuring robust data validation.
Understanding ActiveRecord Validator
ActiveRecord Validator is a built-in mechanism in Ruby on Rails that facilitates data validation within models. It provides a straightforward way to enforce rules and constraints on the data being saved to the database. Common validations include presence, uniqueness, numericality, and format checks. By leveraging ActiveRecord Validator, developers can ensure that only valid data persists, thereby maintaining data integrity and minimising errors. The validators are defined within the model class, making it simple to read and maintain. ActiveRecord Validator is highly customisable, allowing developers to create custom validation methods to cater to specific application needs. This tool is integral to the Rails ecosystem, as it seamlessly integrates with the database interaction layer, providing a cohesive and efficient validation solution. Understanding how to effectively use ActiveRecord Validator can significantly impact the robustness and reliability of your Rails application.
BaseModel vs ActiveRecord Validator in Rails
When comparing BaseModel vs ActiveRecord Validator in Rails, it is essential to understand their distinct roles and applications. BaseModel provides a means to abstract common logic and validations, promoting code reuse and consistency across multiple models. It allows for greater flexibility, enabling developers to implement complex validation logic that may not be directly supported by ActiveRecord. In contrast, ActiveRecord Validator offers a more straightforward approach, with built-in validation methods that are easy to implement and maintain. It is ideal for standard validation tasks, such as checking the presence or uniqueness of attributes. The choice between BaseModel and ActiveRecord Validator often depends on the complexity and specific requirements of your application. For projects needing extensive custom logic, a BaseModel might be more appropriate. However, for simpler, more standardised validation needs, ActiveRecord Validator is typically sufficient. Understanding these differences can help developers choose the most effective strategy for their Rails applications.
Key Differences Explained
Core Functionalities
The core functionalities of BaseModel and ActiveRecord Validator in Rails centre around their unique roles in application architecture. BaseModel primarily serves as an abstract class designed to house shared logic and custom validation methods. It facilitates the creation of reusable components that can be inherited by other models, promoting a DRY (Don't Repeat Yourself) philosophy. This makes it ideal for applications with complex validation needs that are shared across multiple models. On the other hand, ActiveRecord Validator is a built-in feature of Rails specifically tailored for data integrity. It provides a suite of predefined validation methods that can be easily integrated into any model. These methods cover a wide range of common validation scenarios, such as ensuring the presence of an attribute or verifying its uniqueness. The choice between these two hinges on the complexity of the validation logic required and the need for customisation versus ease of implementation. Understanding their core functionalities is pivotal in decision-making.
Use Cases and Examples
Understanding the appropriate use cases for BaseModel vs ActiveRecord Validator in Rails is crucial for effective implementation. BaseModel is particularly useful when multiple models share complex validation logic. For example, if several models require a custom validation that checks multiple attributes in tandem, encapsulating this logic within a BaseModel can centralise the code and ensure consistency. An example might be a validation that checks if a combination of date and time fields are within a permissible range across different event models.
Conversely, ActiveRecord Validator is well-suited for straightforward validations that apply to individual attributes. For instance, if you need to ensure that an email attribute is present and follows a specific format, ActiveRecord's built-in validates method can handle this efficiently. Here is an example:
class User < ApplicationRecord validates :email, presence: true, format: { with: URI::MailTo::EMAIL_REGEXP } end
This simplicity makes ActiveRecord Validator ideal for most common validation tasks, while BaseModel shines in more complex, shared scenarios.
Pros and Cons
Choosing between BaseModel and ActiveRecord Validator in Rails necessitates a clear understanding of their respective pros and cons.
BaseModel offers the advantage of centralising complex validation logic, which can reduce code duplication and promote consistency across models. It allows for flexible, custom solutions tailored to specific application needs. However, this flexibility can also lead to increased complexity, making the codebase harder to read and maintain if not well-managed. It may also demand more development effort upfront.
ActiveRecord Validator, on the other hand, is relatively easy to implement and maintain. It provides a range of built-in validation methods that cover most standard use cases, allowing developers to quickly enforce data integrity. This simplicity often leads to faster development times. Yet, it lacks the flexibility of BaseModel for handling more intricate validation scenarios, which might require cumbersome workarounds.
Balancing these pros and cons is key to selecting the most appropriate approach for your Rails application, ensuring both efficiency and maintainability.
Implementing BaseModel in Your Project
Step-by-Step Guide
Implementing a BaseModel in your Rails project involves a few systematic steps. First, create an abstract class that will serve as your BaseModel. This class should inherit from ActiveRecord::Base and include the abstract_class declaration.
class BaseModel < ActiveRecord::Base self.abstract_class = true # Add shared logic or validations here end
Next, define the shared validation logic within the BaseModel. For instance, you might want to validate a specific format across multiple models.
class BaseModel < ActiveRecord::Base self.abstract_class = true validates :example_attribute, format: { with: /regex_pattern/ } end
Then, ensure that other models inherit from this BaseModel rather than ActiveRecord::Base. This way, they automatically gain the shared validation logic.
class User < BaseModel # User model-specific logic here end class Admin < BaseModel # Admin model-specific logic here end
Finally, test your models to ensure that the validations are applied correctly. Implementing a BaseModel in this manner can significantly enhance code reusability and maintainability.
Common Pitfalls to Avoid
When implementing a BaseModel in your Rails project, avoiding common pitfalls is crucial to maintaining a clean and efficient codebase. One common mistake is overloading the BaseModel with too much logic. This can lead to a bloated base class, making it difficult to manage and understand. Instead, keep the BaseModel focused on shared logic that is genuinely common across multiple models.
Another pitfall is failing to test the BaseModel thoroughly. Since it serves as the foundation for other models, any issues within the BaseModel can propagate throughout your application. Ensure comprehensive testing to catch potential bugs early.
Additionally, watch out for tightly coupling your models to the BaseModel. This can make future changes cumbersome, especially if you decide to refactor or decouple certain functionalities. Maintain flexibility by ensuring that each model can override or extend BaseModel functionalities as needed.
Finally, avoid using BaseModel for validation logic that could be more simply handled by ActiveRecord Validator, to prevent unnecessary complexity.
Best Practices
Implementing a BaseModel in your Rails project requires adhering to best practices to ensure optimal performance and maintainability. First, keep the BaseModel lean by focusing on truly shared logic and validations. Avoid cramming it with business-specific logic that only a few models require.
Secondly, document the purpose and functionality of the BaseModel clearly within the code. This helps other developers understand its role and how to extend or override its methods properly.
Thirdly, make extensive use of unit tests. Validate that the shared logic in the BaseModel works as expected and doesn’t introduce conflicts with individual models. Comprehensive testing ensures that changes in the BaseModel do not inadvertently break other parts of your application.
Additionally, maintain flexibility by designing your BaseModel such that models inheriting from it can easily override or extend its methods. This approach facilitates future enhancements and refactoring.
Lastly, review and refactor your BaseModel periodically to remove any redundant or outdated logic, thereby keeping your codebase clean and efficient.
Working with ActiveRecord Validator
Setup and Configuration
Setting up ActiveRecord Validator in a Rails project is a straightforward process, leveraging the built-in capabilities of Rails. To begin, ensure your model inherits from ActiveRecord::Base, which gives access to the validation methods provided by ActiveRecord.
In your model class, use the validates method to define the validations. For example, to ensure an attribute is present and unique, you could write:
class User < ApplicationRecord validates :email, presence: true, uniqueness: true end
This configuration checks that the email attribute is not blank and that it is unique across all User records.
ActiveRecord Validator also allows custom error messages and conditions. You can specify these within the validates method options:
validates :username, presence: { message: "must be provided" }
This setup integrates seamlessly with Rails, triggering validations automatically when records are saved or updated. By following these steps, you can efficiently use ActiveRecord Validator to maintain the integrity of your data with minimal configuration effort.
Practical Examples
ActiveRecord Validator in Rails provides a versatile toolset for handling various data validation scenarios. Here are a few practical examples showcasing its application.
To validate numericality, you can ensure that an attribute is a number within a specific range:
class Product < ApplicationRecord validates :price, numericality: { greater_than: 0 } end
This validation ensures that the price attribute is a positive number.
For format validation, such as a phone number, you can use regular expressions:
class Contact < ApplicationRecord validates :phone_number, format: { with: /\A\d{10}\z/, message: "must be 10 digits" } end
Here, the phone_number is validated to contain exactly ten digits.
Conditional validations are also possible. For instance, you might only validate an attribute under certain conditions:
class Order < ApplicationRecord validates :discount_code, presence: true, if: :discount_applied? def discount_applied? discount.present? end end
These examples illustrate how ActiveRecord Validator can be tailored to meet diverse validation requirements, ensuring data integrity with minimal effort.
Troubleshooting Tips
When working with ActiveRecord Validator in Rails, encountering issues is not uncommon. Here are some troubleshooting tips to help resolve common problems.
First, ensure that your validations are correctly defined in the model. Typos or syntax errors can prevent validations from functioning as expected. Double-check the Rails documentation for proper usage.
If a validation isn't triggering, confirm that the attribute being validated is part of the model. Sometimes, missing database migrations or schema discrepancies can cause issues. Running rails db:migrate can help sync the schema.
When custom validation methods aren't working, make sure they are correctly referenced and defined within the model. Verify that these methods are accessible and properly scoped.
To debug, use ActiveModel::Errors to inspect validation error messages. For example, record.errors.full_messages will provide detailed error messages that can guide your troubleshooting.
Lastly, test your validations thoroughly. Automated tests can catch validation issues early, ensuring that your data integrity mechanisms are robust and reliable. Following these tips can streamline the debugging process and enhance your Rails application.
Conclusion and Final Thoughts
When to Use Each Validator
Choosing between BaseModel and ActiveRecord Validator in Rails depends on your application's specific needs. Use BaseModel when you have complex validation logic that is shared across multiple models. This approach promotes code reuse and consistency, making it ideal for large applications with intricate validation requirements. For example, if several models need to validate a unique combination of attributes or require extensive custom methods, BaseModel is the way to go.
On the other hand, ActiveRecord Validator is perfect for straightforward, attribute-level validations. It’s built into Rails, making it easy to implement and maintain. Use it when you need to ensure the presence, uniqueness, or format of individual attributes. This is particularly effective for smaller projects or scenarios where validations are not overly complex.
By understanding the strengths and limitations of each approach, you can make informed decisions that enhance the robustness and maintainability of your Rails application.
Future Trends in Rails Validators
The landscape of Rails validators is evolving, driven by the need for more robust, scalable solutions as applications grow in complexity. One emerging trend is the integration of machine learning algorithms to refine validation processes. Machine learning can adaptively learn from data patterns, offering predictive validation that evolves over time.
Another trend is the increasing use of microservices architectures, which necessitate validators that can operate across distributed systems. This requires validators to be more modular and independent, promoting interoperability between different services.
The community is also exploring enhanced customisation options within Rails validators, allowing for more granular control over validation logic. This includes extending validators to handle complex data types, such as JSON or XML, directly within the model layer.
Finally, as security concerns heighten, there's an emphasis on validators that incorporate security checks, ensuring data integrity and protection against vulnerabilities. These trends indicate a shift towards more intelligent, adaptable, and secure validation solutions in Rails.
Recap and Recommendations
In summary, understanding the distinctions between BaseModel and ActiveRecord Validator in Rails is crucial for effective application development. BaseModel offers a flexible approach for handling complex, shared validation logic across multiple models, making it ideal for larger applications with intricate requirements. Conversely, ActiveRecord Validator provides a simple and efficient way to enforce standard attribute-level validations, perfect for straightforward scenarios.
When deciding which to use, consider the complexity of your validation needs and the size of your project. For extensive, shared logic, a BaseModel helps maintain consistency and reduces code duplication. For simpler, individual attribute checks, ActiveRecord Validator is usually sufficient and easier to implement.
We recommend leveraging comprehensive testing to ensure that your chosen validation method performs as expected. Regularly review and refactor your validation logic to keep your codebase clean and efficient. By carefully selecting the appropriate validator, you can enhance your application's robustness and maintainability.
FAQs
- What is BaseModel in Rails?
BaseModel is an abstract class in Rails used to encapsulate shared logic and custom validations across multiple models. - How does ActiveRecord Validator work in Rails?
ActiveRecord Validator provides built-in methods to validate attributes directly within Rails models, ensuring data integrity during saves and updates. - What are the key differences between BaseModel and ActiveRecord Validator in Rails?
BaseModel centralizes complex, reusable logic across models, while ActiveRecord Validator focuses on simple, attribute-level validations within individual models. - When should I use BaseModel in Rails development?
Use BaseModel when multiple models share complex validation logic, requiring centralized management for consistency and maintainability. - When is ActiveRecord Validator the better choice in Rails?
ActiveRecord Validator is ideal for straightforward validations, such as presence or uniqueness, directly on individual model attributes. - How does BaseModel promote code reusability in Rails?
BaseModel allows developers to define shared methods and validations in a single class, which can then be inherited by multiple models. - What types of validations does ActiveRecord Validator support?
ActiveRecord Validator supports validations for presence, uniqueness, numericality, format, length, and custom logic through methods. - Can BaseModel and ActiveRecord Validator be used together in a Rails application?
Yes, BaseModel can centralize shared validations, while ActiveRecord Validator handles model-specific validations, creating a complementary setup. - What are the advantages of using ActiveRecord Validator?
ActiveRecord Validator is simple to use, integrates seamlessly with Rails, and supports a variety of common validation scenarios with minimal effort. - What are the challenges of using BaseModel in Rails?
Overloading BaseModel with excessive logic can make it complex and harder to maintain, requiring careful design and documentation. - How does BaseModel handle custom validation logic?
BaseModel enables developers to define and apply custom methods that extend beyond the built-in capabilities of ActiveRecord Validator. - What are practical examples of ActiveRecord Validator in use?
Examples include validating email format, ensuring numerical ranges for prices, or enforcing the uniqueness of usernames in a model. - How can BaseModel improve maintainability in Rails applications?
BaseModel reduces code duplication by centralizing shared logic, making it easier to update and maintain across related models. - How does ActiveRecord Validator handle errors in validations?
Validation errors are stored in an ActiveModel::Errors object, accessible via errors.full_messages for debugging or display purposes. - Can BaseModel be used for non-validation logic in Rails?
Yes, BaseModel can also house other shared functionalities, such as custom scopes or utility methods, beyond just validations. - What are common pitfalls when using ActiveRecord Validator?
Improper syntax, missing attributes, or neglecting to test validations can lead to unexpected errors or incomplete data protection. - How does the Rails community support ActiveRecord Validator?
The Rails community provides extensive documentation, examples, and forums to help developers effectively use ActiveRecord Validator. - Are there performance considerations when using BaseModel?
A poorly designed BaseModel can impact performance by introducing unnecessary complexity; efficient implementation is key. - How does ActiveRecord Validator contribute to Rails’ DRY principle?
ActiveRecord Validator keeps validation logic concise and reusable within models, reducing redundancy across the application. - What is the future of validation in Rails applications?
The future includes advanced tools like machine learning for adaptive validations and modular validators for microservices architectures.
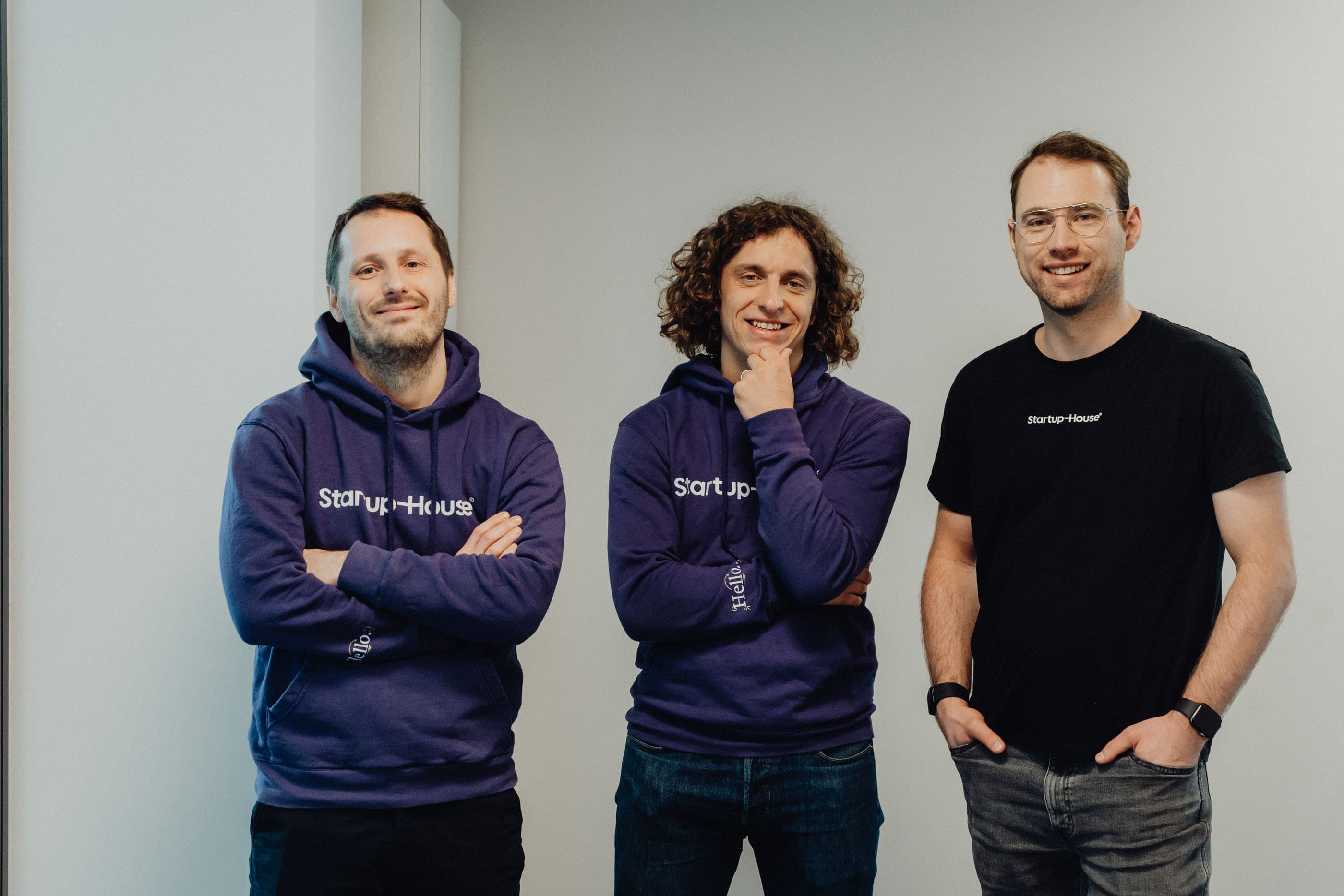
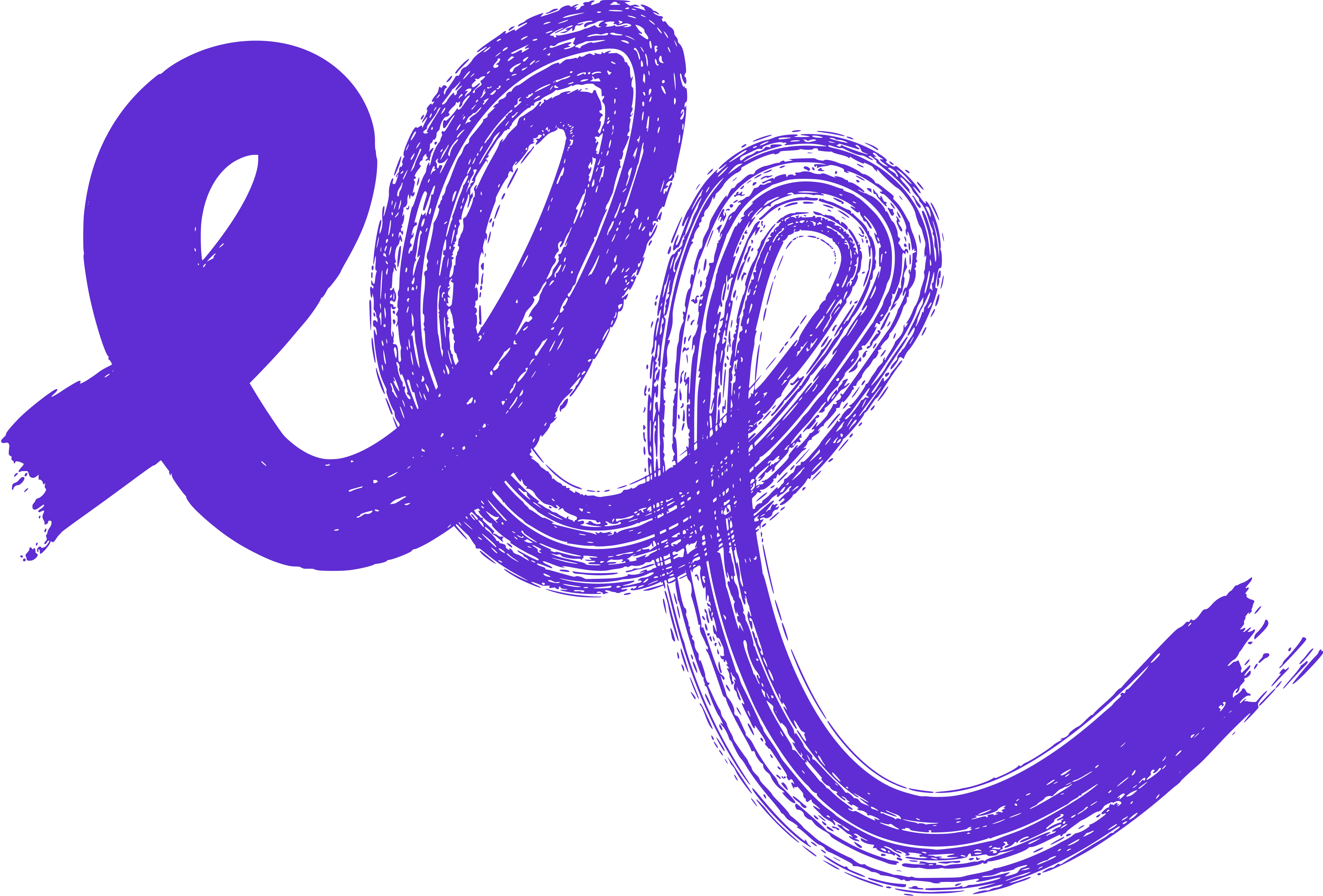
You may also
like...
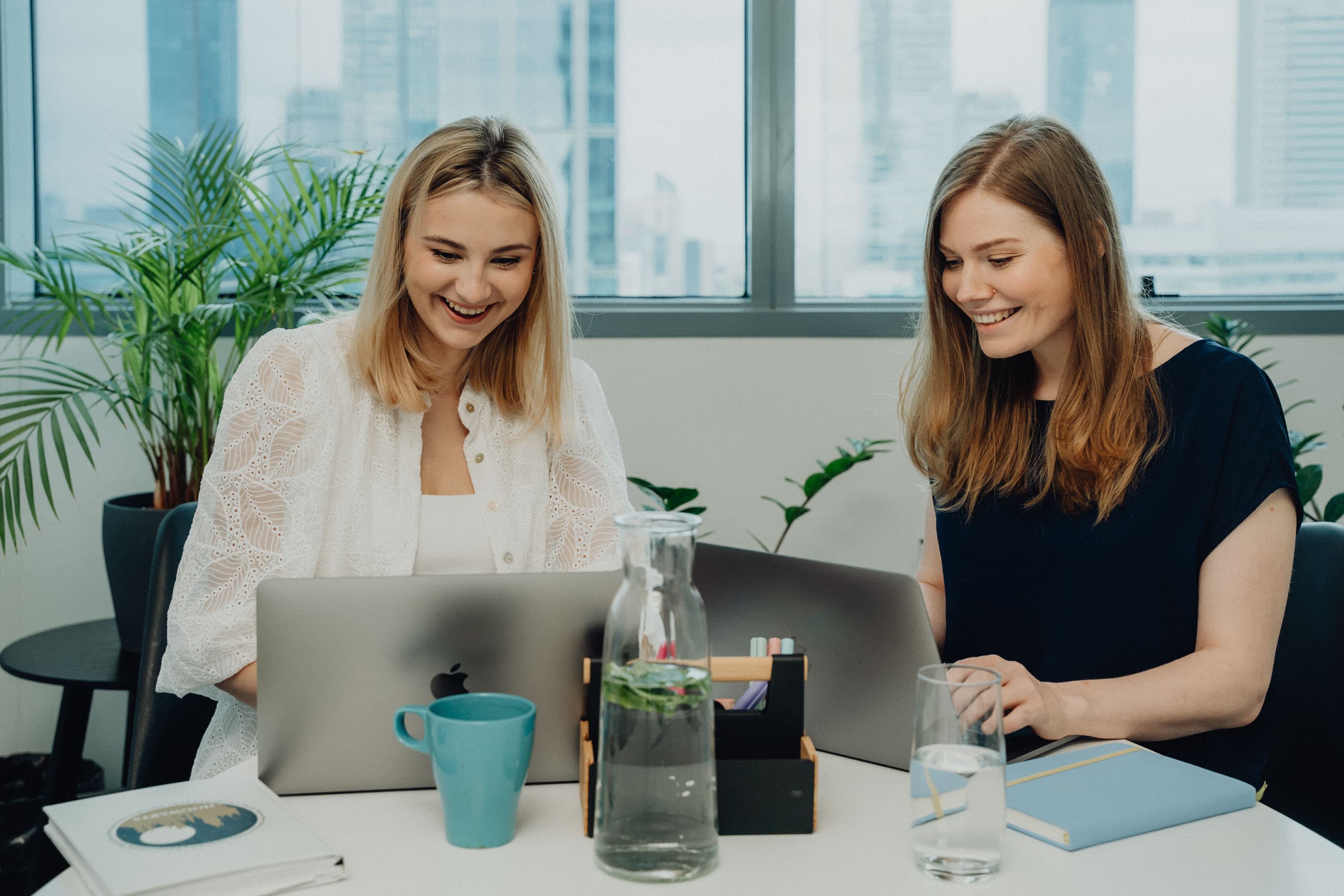
Mastering Declarative Programming: Essential Practices for Every Developer
Discover declarative programming essentials. This guide covers principles, tools, and best practices to simplify coding, enhance readability, and improve scalability.
Marek Pałys
Apr 16, 2024・11 min read
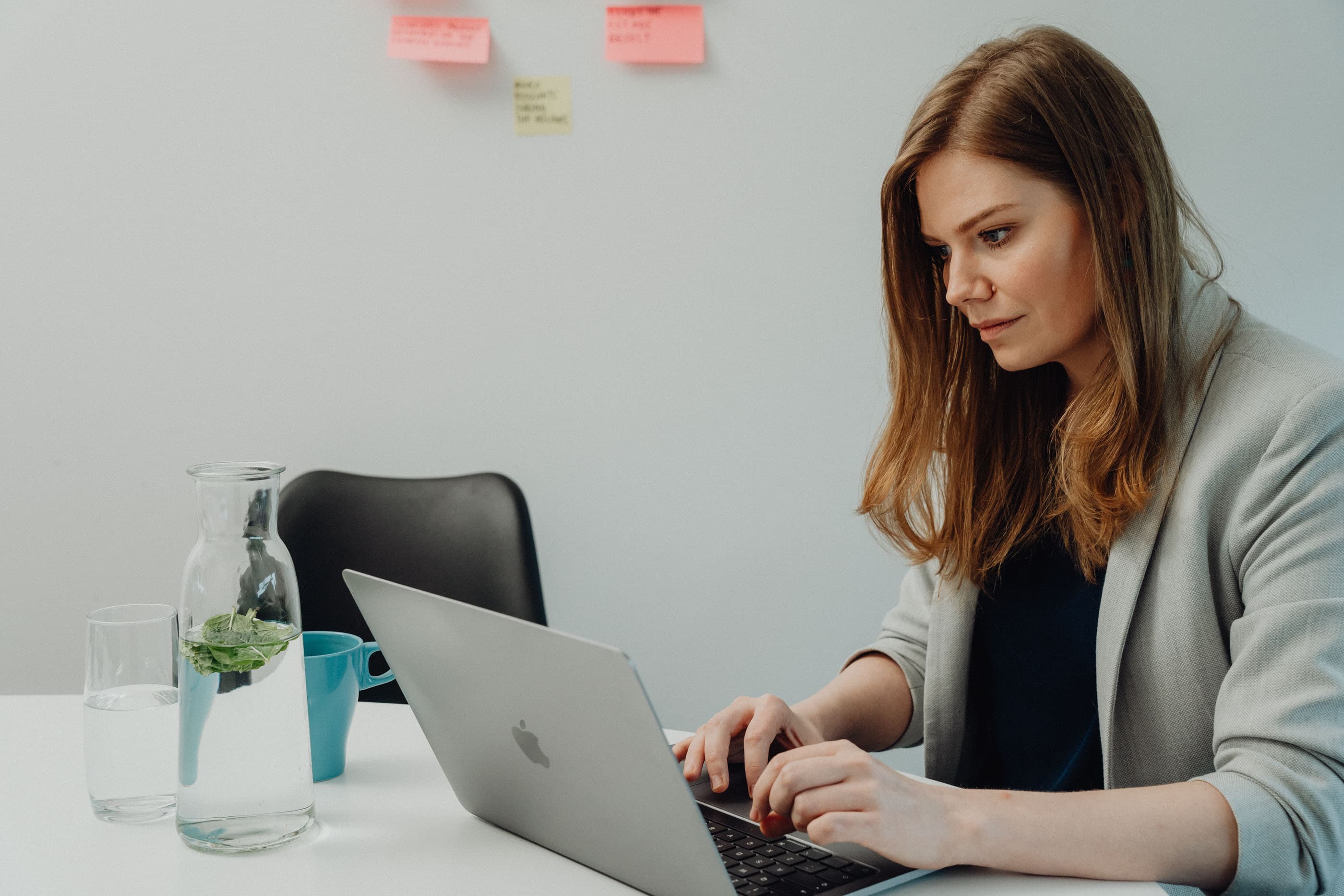
Understanding Event-Driven Programming: A Simple Guide for Everyone
Explore the essentials of event-driven programming. Learn how this responsive paradigm powers interactive applications with real-world examples and key concepts.
Marek Pałys
Apr 30, 2024・9 min read
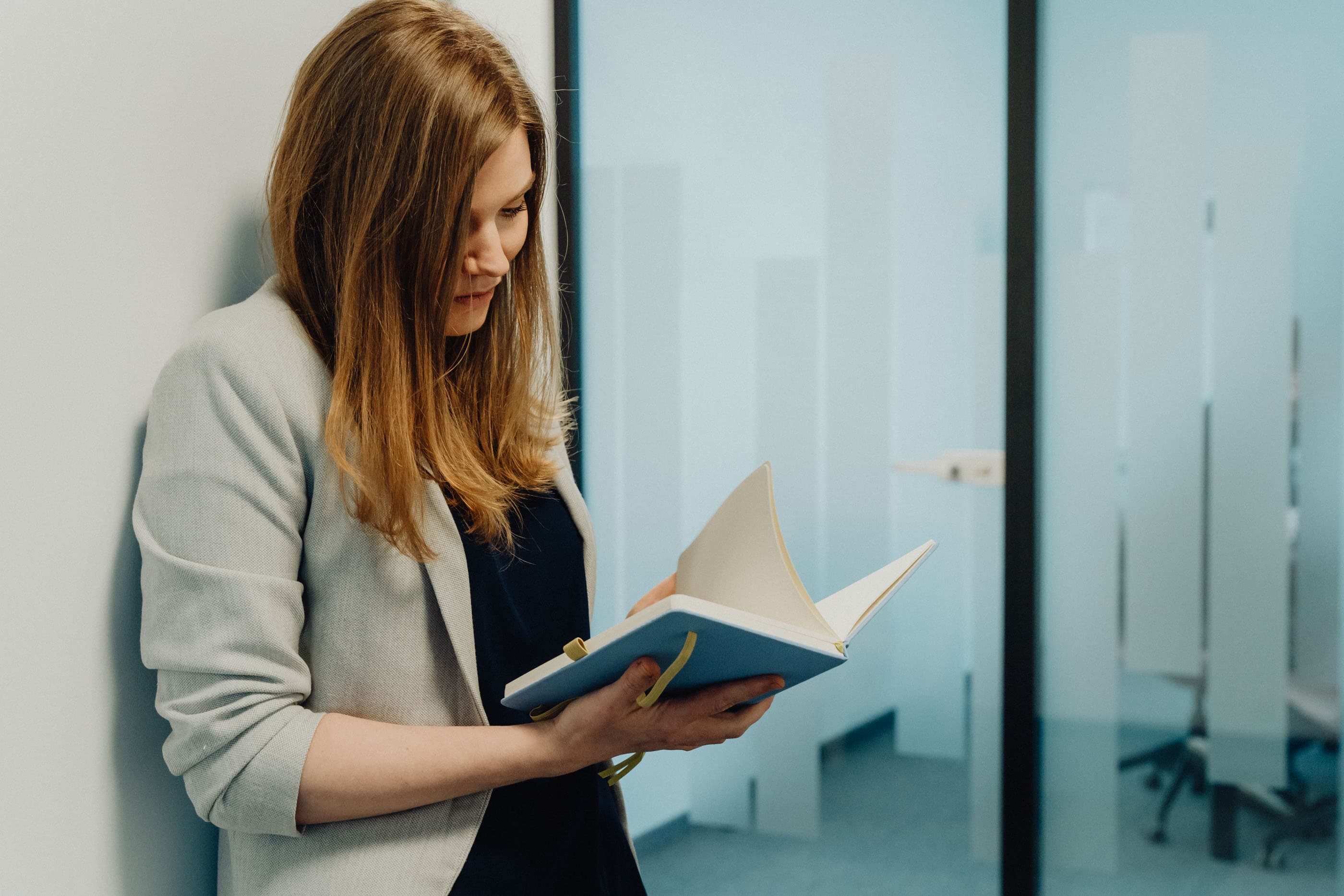
Demystifying Procedural Programming: Simple Examples for All
Explore procedural programming with easy-to-follow examples and insights into its core principles. Learn how this step-by-step approach forms the basis of many programming paradigms.
Marek Pałys
Jul 05, 2024・10 min read